Introduction
Welcome to the documentation of Enable Banking aggregation core!
This documentation focuses on the low-level interface of the core technology for aggregation of Open Banking APIs.
Get started by cloning our sample repository
$ git clone https://github.com/enablebanking/OpenBankingJavaExamples
$ git clone https://github.com/enablebanking/OpenBankingJSExamples
$ git clone https://github.com/enablebanking/OpenBankingPythonExamples
Enable Banking aggregation core provides native Java, Javascript and Python libraries for connecting directly from your code to a number of Open Banking APIs without intermediate aggregation services. The libraries provide unified interface for interacting with multiple ASPSPs (i.e. banks and similar financial institutions), harmonize data and implement all cryptographic functionality needed for interaction with PSD2 APIs, such as mTLS connection using eIDAS QWAC and request signing using eIDAS QSeal certificate.
The aggregation core powers Enable Banking API and its on-premise version.
It's the easiest to get started with the aggregation core by using our code samples. The complete code samples for Java, Javascript and Python are available under our Github account: https://github.com/enablebanking.
User guide
Authorization and user token refresh
This section describes a basic authorization flow and access token refreshing flow (if it is supported by an ASPSP).
In order to execute this flow correctly for a specific ASPSP connector, one should obtain connector's meta information and build your flow according to that information.
Below is the basic example of how such flow can be implemented.
0. Import library and choose connector
import com.enablebanking.ApiClient;
import com.enablebanking.api.AuthApi;
import com.enablebanking.api.MetaApi;
import com.enablebanking.model.*;
// Initializing meta interface.
MetaApi metaApi = new MetaApi(new ApiClient());
// Getting connectors for a country.
HalConnectors connectors = metaApi.getConnectors("FI");
// Finding meta data for a desired connector
Connector connectorMeta = null;
for (Connector conn : connectors.getConnectors()) {
if (conn.getName().equals('Sample')) {
connectorMeta = conn;
break;
}
}
const enablebanking = require('enablebanking');
// Initializing meta interface.
const metaApi = new enablebanking.MetaApi(new enablebanking.ApiClient());
// Getting connectors for a country.
const connectors = await metaApi.getConnectors({country: 'FI'});
// Finding meta data for a desired connector
let connectorMeta;
for (const conn of connectors.connectors) {
if (conn.name == 'Sample') {
connectorMeta = conn;
break;
}
}
import enablebanking
// Initializing meta interface.
meta_api = enablebanking.MetaApi(enablebanking.ApiClient())
// Getting connectors for a country.
connectors_info = meta_api.get_connectors(country='FI')
// Finding meta data for a desired connector
connector_meta = None
for conn in .connectors:
if conn.name == 'Sample':
connector_meta = conn
break
First of all we are getting the list of connectors for a country and choosing one connector, which we are going to used. In real-world case a list of ASPSPs accessed with the connectors, shall be shown to a user for making a choise. In the code sample we are just using 'Sample'.
1. Initialize API client and authentication interface
// Initializing settings; connector settings class to be replaced with a real one.
ConnectorSettings settings = new SampleConnectorSettings()
// other settings, including callback redirect URI, to be set here
.sandbox(true);
// Creating client instance.
ApiClient apiClient = new ApiClient(settings);
// Initializing authentication interface.
AuthApi authApi = new AuthApi(apiClient);
// Creating client instance; connector name to be replaced with a real one.
const apiClient = new enablebanking.ApiClient('Sample', {
sandbox: true,
/* other settings, including callback redirect URI, to be set here */
});
// Initializing authentication interface.
const authApi = new enablebanking.AuthApi(apiClient);
// Creating client instance; connector name to be replaced with a real one.
api_client = enablebanking.ApiClient(
"Sample",
{
"sandbox": True,
# other settings, including callback redirect URI, to be set here
})
// Initializing authentication interface.
auth_api = enablebanking.AuthApi(api_client)
After the choise has been made, we need to initialize API client for the chosen connector and create the authentication interface.
Connector settings (i.e. set of parameters used when initializing API client) differ from one connector to another. Description of settings for every connector can be found here.
2. Initiate user authentication
// Checking if user ID is required when initiating user authentication.
String userId = null;
if (connectorMeta.getAuthInfo().get(0).getInfo().isUserIdRequired()) {
// Setting userId; in real case it is coming from a user.
userId = "someUserId";
}
// Checking if user consent can be received while user authentication.
Access access = null;
if (connectorMeta.getAuthInfo().get(0).getInfo().isAccess()) {
// Initializing access, i.e. scope of access, which user needs to consent with.
access = new Access(/* user consent parameters */);
}
// Initiating user authentication
Auth auth = authApi.getAuth(
"test", // state returned to callback redirect URI
userId,
access);
// Sending user to this URL for authentication
String authUrl = auth.url;
// Checking if user ID is required when initiating user authentication.
let userId;
if (connectorMeta.authInfo[0].info.userIdRequired) {
// Setting userId; in real case it is coming from a user.
userId = "someUserId";
}
// Checking if user consent can be received while user authentication.
let access;
if (connectorMeta.authInfo[0].info.access) {
// Initializing access, i.e. scope of access, which user needs to consent with.
access = new Access(/* user consent parameters */);
}
// Initiating user authentication
const auth = await authApi.getAuth({
state: 'test', // state returned to callback redirect URI
access: access,
userId: userId
});
// Sending user to this URL for authentication
const authUrl = auth.url;
# Checking if user ID is required when initiating user authentication.
user_id = None
if connector_meta.auth_info[0].info.user_id_required:
# Setting userId; in real case it is coming from a user.
user_id = "some_user_id"
# Checking if user consent can be received while user authentication.
access = None
if connector_meta.auth_info[0].info.access:
# Initializing access, i.e. scope of access, which user needs to consent with.
access = enablebanking.Access(
# user consent parameters
)
# Initiating user authentication
auth = auth_api.get_auth(
state='test', # state returned to callback redirect URI
user_id=user_id,
access=access)
# Sending user to this URL for authentication
url = auth.url
Some connectors require user ID to be set when initiating user authentication. This information is available in connector meta and user ID shall be requested from a user.
Also scope of access, which user needs to consent with, shall be set. It will be used if user consent can be received while user authentication. If not, modify consents shall be used later on. By default widest possible scope will be requested.
And after the parameters are set, user authentication shall be initiated.
3. Obtain authorization code and get an access token
// Extracting data from the URL where user was redirected to after authentication.
AuthRedirect redirectInfo = authApi.parseRedirectUrl("https://your.domain/callback");
// Obtaining user access token (and possibly refresh token as well).
Token token = authApi.makeToken(
"authorization_code", // grant type
redirectInfo.code, // authorization code
auth.env // authentication environment
);
// Extracting data from the URL where user was redirected to after authentication.
AuthRedirect redirectInfo = authApi.parseRedirectUrl('https://your.domain/callback');
// Obtaining user access token (and possibly refresh token as well).
const token = await authApi.makeToken(
'authorization_code', // grant type
redirectInfo.code, // authorization code
auth.env // authentication environment
)
# Extracting data from the URL where user was redirected to after authentication.
redirect_info = auth_api.parse_redirect_url("https://your.domain/callback")
# Obtaining user access token (and possibly refresh token as well).
token = auth_api.make_token(
"authorization_code", # grant type
code, # authorization code
auth.env)
After the user has authenticated (and is redirected back to the desired URL), we are parsing this callback redirect URL to obtain an authorization code and other data.
The authorization code is to be used when making user access token. Along with the access token, returned Token data structure contains lifetime of the access token in seconds and refresh token (if it is supported).
4. Refresh access token (when needed)
Token refreshedToken = null;
if (connectorMeta.isRefreshToken()) {
refreshedToken = authApi.makeToken(
"refresh_token", // grant type
token.getRefreshToken(), // refresh token
null // environment is not needed for token refresh
);
}
let refreshedToken;
if (connectorMeta.refreshToken) {
refreshedToken = authApi.makeToken(
"refresh_token", // grant type
token.refresh_token // refresh token
);
}
refreshed_token = None
if connector_meta.refresh_token:
refreshed_token = auth_api.make_token(
"refresh_token",
token.refresh_token
)
When supported, refresh token can be used for obtaining new user access token.
Information on whether refresh tokens are supported by a connector can be seen in the connector meta.
Reference
Select a language for code samples from the tabs above or the mobile navigation menu.
Enable Banking aggregation core consists of 4 interfaces:
- Meta provides information about available connectors;
- Auth provides PSU (bank user) authentication and token creation functionality;
- AISP provides functions for accessing account information, such as transactions and balances, on behalf of a PSU;
- PISP provides functions for initiating and conforming payment requests.
The same calls and data structures are used for interacting with different ASPSPs. In order to use each of the interfaces corresponding instance needs to be created. When instantiating any of the interfaces except Meta, ASPSP connector name and settings need to be supplied. The settings differ from one connector to another (although many of them have the same properties) and are described in the Connectors section of the documentation.
Enable Banking aggregation core interfaces were originally based on STET PSD2 specification, but have been heavily modified and extended in order to support usage scenarious beyond the original specification.
License: LicenseRef-LICENSE
Meta API
getConnectors
Code samples
const enablebanking = require('enablebanking');
const metaApi = new enablebanking.MetaApi(new enablebanking.ApiClient());
const connectors = await metaApi.getConnectors({
country: 'FI' // requesting connectors for Finland
});
import enablebanking
meta_api = enablebanking.MetaApi(enablebanking.ApiClient())
connectors = meta_api.get_connectors(
country='FI') # requesting connectors for Finland
import com.enablebanking.*;
import com.enablebanking.model.*;
import com.enablebanking.api.MetaApi;
MetaApi metaApi = new MetaApi(new ApiClient());
HalConnectors connectors = metaApi.getConnectors("FI"); // connectors for Finland
Retrieval of the available connectors
Parameters
Name | Type | Required | Description |
---|---|---|---|
country | string | false | Country ISO 3166-1 alpha-2 code |
connector | string | false | Connector name |
Returned value
Data type: HalConnectors
List of connectors
Auth API
getAuth
Code samples
const enablebanking = require('enablebanking');
const authApi = new enablebanking.AuthApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const { url } = await authApi.getAuth(
{
state: 'test' // state to pass to redirect URL
});
import enablebanking
auth_api = enablebanking.AuthApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
})
url = auth_api.get_auth(
state='test', # state to pass to redirect URL
).url
import com.enablebanking.ApiClient;
import com.enablebanking.model.*;
import com.enablebanking.api.AuthApi;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AuthApi authApi = new AuthApi(new ApiClient(connectorSettings));
String url = authApi.getAuth(
"test", // state to pass to redirect URL
null, // user id
null, // password
null // not passing access request (bank's defaults will be used)
).url;
Request Authorization Data
Parameters
Name | Type | Required | Description |
---|---|---|---|
state | string | false | Arbitrary String to be returned from to redirect URI |
credentials | array[string] | false | Array of user credentials which are required to log in to the bank (e.g. user ID, password, phone number etc) |
method | string | false | Authentication method |
access | Access | false | Request for access to account information |
Detailed descriptions
access: Request for access to account information This parameter should be set to override defaults for the banks, which allow PSUs to give consent for account information sharing right after authentication.
Returned value
Data type: Auth
Authorization Data
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
makeToken
Code samples
const enablebanking = require('enablebanking');
const authApi = new enablebanking.AuthApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const token = await authApi.makeToken(
'authorization_code', // grant type, MUST be set to "authorization_code"
'so43ls-3ldg03sd-hl4saa3l2sl5czfhl3'); // The code received in the query string when redirected from authorization
import enablebanking
auth_api = enablebanking.AuthApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
})
token = auth_api.make_token(
'authorization_code', # grant type, MUST be set to "authorization_code"
'so43ls-3ldg03sd-hl4saa3l2sl5czfhl3') # The code received in the query string when redirected from authorization
import com.enablebanking.ApiClient;
import com.enablebanking.model.*;
import com.enablebanking.api.AuthApi;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AuthApi authApi = new AuthApi(new ApiClient(connectorSettings));
Token token = authApi.makeToken(
"authorization_code", // grant type, MUST be set to "authorization_code"
"so43ls-3ldg03sd-hl4saa3l2sl5czfhl3" // The code received in the query string when redirected from authorization
);
Request Access Token
Parameters
Name | Type | Required | Description |
---|---|---|---|
grant_type | string | true | Value should be set to authorization_code or refresh_token depending on what |
code | string | false | Value of either the code received in the query string when redirected from |
auth_env | string | false | Additional environment data for makeToken. This parameter is only required for some connectors. |
Detailed descriptions
grant_type: Value should be set to authorization_code
or refresh_token
depending on what
passed to code
parameter.
code: Value of either the code received in the query string when redirected from authorization page or the refresh token used for renewing access token.
Enumerated Values
grant_type
Value | Description |
---|---|
authorization_code | Used to exchange an authorization code for an access token. After the user returns to the client via the redirect URL, the application will get the authorization code from the URL and use it to request an access token. |
refresh_token | Used to exchange a refresh token for an access token when the access token has expired. This allows clients to continue to have a valid access token without further interaction with the user. Please note that refresh token is likely to be changed when calling `makeToken` with `refresh_token` grant type. |
Returned value
Data type: Token
Authorisation token (Bearer)
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
getCurrentToken
Code samples
const enablebanking = require('enablebanking');
const authApi = new enablebanking.AuthApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const token = await authApi.getCurrentToken();
import enablebanking
auth_api = enablebanking.AuthApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
})
token = auth_api.get_current_token()
import com.enablebanking.ApiClient;
import com.enablebanking.model.*;
import com.enablebanking.api.AuthApi;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AuthApi authApi = new AuthApi(new ApiClient(connectorSettings));
Token token = authApi.getCurrentToken();
Get current token data
Returned value
Data type: Token
Current token. Values for some fields might be missing if not used.
parseRedirectUrl
Parsing of redirect url during authorization
Parameters
Name | Type | Required | Description |
---|---|---|---|
redirectUrl | string | true | Redirect url |
Returned value
Data type: AuthRedirect
Structured data extracted from redirect URL
validateCredentials
Parsing of redirect url during authorization
Parameters
Name | Type | Required | Description |
---|---|---|---|
credentials | array[string] | false | Array of user credentials which are required to log in to the bank (e.g. user ID, password, phone number etc) |
method | string | false | Authentication method |
Returned value
Data type: AuthRedirect
Structured data extracted from redirect URL
setClientInfo
Set extra PSU headers
Parameters
Name | Type | Required | Description |
---|---|---|---|
clientInfo | ClientInfo | false | ClientInfo object with PSU headers |
Returned value
Data type: None
Client Info was successfully set.
AISP API
getAccounts
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const accounts = await aispApi.getAccounts();
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
accounts = aisp_api.get_accounts()
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
HalAccounts accounts = aispApi.getAccounts();
Retrieval of the PSU accounts (AISP)
Description
This call returns all payment accounts that are relevant the PSU on behalf of whom the AISP is connected.Prerequisites
- Valid PSU access token shall be set in the connector.
- For ASPSPs requiring PSU consent for listing the accounts, consent ID shall be set in the connector.
Business flow
The TPP sends a request to the ASPSP for retrieving the list of the PSU payment accounts. The ASPSP computes the relevant PSU accounts and builds the answer as an accounts list. Each payment account will be provided with its characteristics returned by ASPSP, which may vary between ASPSPs and depending on the consent given by the PSU.Returned value
Data type: HalAccounts
Returned value consists of the list of the accounts that have been made available to the AISP by the PSU.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
AccountNotAccessibleException | Raised when non accessible or inexsitent account transactions are requested. |
InvalidResponseSignatureException | none |
getAccountBalances
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const balances = await aispApi.getAccountBalances('203059694928560295396833');
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
balances = aisp_api.get_account_balances('203059694928560295396833')
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
HalBalances balances = aispApi.getAccountBalances("203059694928560295396833")
Retrieval of an account balances report (AISP)
Description
This call returns a set of balances for a given PSU account that is specified by the AISP through an account resource identification.- ASPSPs provide at least the accounting balance on the account.
- ASPSPs can provide other balance restitutions, e.g. instant balance, as well, if possible.
- Actually, from the PSD2 perspective, any other balances that are provided through the Web-Banking service of the ASPSP must also be provided by this ASPSP through the API.
Prerequisites
- Valid PSU access token shall be set in the connector.
- Consent ID shall be set in the connector.
- The TPP has previously retrieved the list of available accounts for the PSU.
Business flow
The AISP requests the ASPSP on one of the PSU’s accounts.The ASPSP answers by providing a list of balances on this account.
Parameters
Name | Type | Required | Description |
---|---|---|---|
accountResourceId | string | true | Identification of account resource to fetch |
Returned value
Data type: HalBalances
Returned value consists of the list of account balances.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
AccountNotAccessibleException | Raised when non accessible or inexsitent account transactions are requested. |
InvalidResponseSignatureException | none |
getAccount
Retrieval of an account details (AISP)
Description
This call returns the details of a given PSU account that is specified by the AISP through an account resource identification.Prerequisites
- Valid PSU access token shall be set in the connector.
- Consent ID shall be set in the connector.
- The TPP has previously retrieved the list of available accounts for the PSU.
Business flow
The AISP requests the ASPSP on one of the PSU’s accounts.The ASPSP answers by providing the details of this account.
Parameters
Name | Type | Required | Description |
---|---|---|---|
accountResourceId | string | true | Identification of account resource to fetch |
Returned value
Data type: AccountResource
Returned value consists of the account details.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
AccountNotAccessibleException | Raised when non accessible or inexsitent account transactions are requested. |
InvalidResponseSignatureException | none |
getAccountTransactions
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const transactions = await aispApi.getAccountTransactions('203059694928560295396833');
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
transactions = aisp_api.get_account_transactions('203059694928560295396833')
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
HalTransactions transactions = aispApi.getAccountTransactions("203059694928560295396833");
Retrieval of an account transaction set (AISP)
Description
This call returns transactions for an account for a given PSU account that is specified by the AISP through an account resource identification. The request may use some filter parameter in order to restrict the query.Prerequisites
- Valid PSU access token shall be set in the connector.
- Consent ID shall be set in the connector.
- The TPP has previously retrieved the list of available accounts for the PSU.
Business flow
The AISP requests the ASPSP on one of the PSU’s accounts. It may specify some selection criteria. The ASPSP answers by a set of transactions that matches the query.Parameters
Name | Type | Required | Description |
---|---|---|---|
accountResourceId | string | true | Identification of account resource to fetch |
dateFrom | string(date-time) | false | Inclusive minimal imputation date of the transactions. |
dateTo | string(date-time) | false | Exclusive maximal imputation date of the transactions. |
continuationKey | string | false | Specifies the value on which the result has to be computed. |
transactionStatus | TransactionStatus | false | Transactions having a transactionStatus equal to this parameter are included within the result. |
Detailed descriptions
dateFrom: Inclusive minimal imputation date of the transactions.
Transactions having an imputation date equal to this parameter are included within the result.
dateTo: Exclusive maximal imputation date of the transactions.
Transactions having an imputation date equal to this parameter are not included within the result.
continuationKey: Specifies the value on which the result has to be computed.
Enumerated Values
transactionStatus
Value | Description |
---|---|
BOOK | (ISO20022 ClosingBooked) Accounted transaction |
CNCL | Cancelled transaction |
HOLD | Account hold |
OTHR | Transaction with unknown status or not fitting the other options |
PDNG | (ISO20022 Expected) Instant Balance Transaction |
RJCT | Rejected transaction |
SCHD | Scheduled transaction |
Returned value
Data type: HalTransactions
Complete transactions response
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
TransactionPeriodException | Raised when wrong or invalid account transactions period is requested. |
AccountNotAccessibleException | Raised when non accessible or inexsitent account transactions are requested. |
InvalidResponseSignatureException | none |
getTransactionDetails
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const transactions = await aispApi.getTransactionDetails('203059694928560295396833', '797272616');
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
transactions = aisp_api.get_transaction_details('203059694928560295396833', '797272616')
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
HalTransactions transactions = aispApi.getTransactionDetails("203059694928560295396833","797272616");
Retrieval of an account transaction details (AISP)
Description
This call enables you to retrieve transaction details for a specific transaction, as designated by the Account Information Service Provider (AISP), using an account resource identification. The returned information provides comprehensive insights into the transactions associated with the specified account.Prerequisites
- Valid PSU access token shall be set in the connector.
- Consent ID shall be set in the connector.
- The TPP has previously retrieved the list of available accounts for the PSU.
Business flow
The AISP requests the ASPSP on the details of one of the PSU’s account transactions.The ASPSP answers by providing the details of this transaction.
Parameters
Name | Type | Required | Description |
---|---|---|---|
accountResourceId | string | true | Identification of account resource to fetch |
transactionResourceId | string | true | Identification of a transaction resource to fetch |
Returned value
Data type: Transaction
Complete transactions details response
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
TransactionPeriodException | Raised when wrong or invalid account transactions period is requested. |
AccountNotAccessibleException | Raised when non accessible or inexsitent account transactions are requested. |
InvalidResponseSignatureException | none |
modifyConsents
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const consent = await aispApi.modifyConsents({
access: {
balances: ['203059694928560295396833'],
transactions: [],
trustedBeneficiaries: false,
psuIdentity: true
}
});
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
consent = aisp_api.modify_consents(
access=enablebanking.Access(
# default access to balances
balances=enablebanking.BalancesAccess(),
# default access to transactions
transactions=enablebanking.TransacrionsAccess(),
trusted_beneficiaries=False,
psu_identity=True,
),
)
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
Consent consent = aispApi.modifyConsents(
new Access()
.balances(new BalancesAccess()) // default access to balances
.transactions(new TransactionsAccess()) // default access to transactions
.trustedBeneficiaries(false)
.psuIdentity(true));
Forwarding the PSU consent (AISP)
Description
This call requests ASPSP to set the consent of an authenticated PSU. In the mixed detailed consent on accounts- the AISP captures the consent of the PSU
- then it forwards this consent to the ASPSP
Prerequisites
- Valid PSU access token shall be set in the connector.
- For ASPSPs allowing consent to be give only for specified accounts, the list of accounts shall be retrieved.
Business flow
The PSU specifies to the AISP which of his/her accounts will be accessible and which functionalities should be available. The AISP forwards these settings to the ASPSP.Parameters
Name | Type | Required | Description |
---|---|---|---|
state | string | false | Arbitrary String to be returned from to redirect URI |
access | Access | false | List of consents granted to the AISP by the PSU. |
Returned value
Data type: Consent
Created or modified consent
Exceptions
Data type | Description |
---|---|
ResponseValidationException | Raised when ASPSP API responses with unspecified error. |
deleteConsent
Deletes consent (AISP)
Description
Revokes PSU consent. After the consent is revoked, it becomes invalid and can not be used for futher API requests.Business flow
The PSU signals that he/she wants to revoke his consent for TPP to access account information. The TPP makes a request to the ASPSP to revoke PSU consent. The consent becomes invalid and its ID can not be used for consequent calls.Returned value
Data type: None
Consent successfully revoked
getCurrentConsent
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
const consent = await aispApi.getCurrentConsent();
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
consent = aisp_api.get_current_consent()
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
Consent consent = aispApi.getCurrentConsent();
Returns current consent (AISP)
Description
This call returns the consent current being used by the ASPSP connector, i.e. either explicitly set when the connector is initialized or as side effect produced by `getAuth` call.Returned value
Data type: Consent
Current consent used for accessing AIS APIs.
PISP API
makePaymentRequest
Code samples
const enablebanking = require('enablebanking');
const pispApi = new enablebanking.PISPApi(
new enablebanking.ApiClient('ConnectorName', { /* Connector settings */ }));
// Making payment request with credit transafer transaction of one element
const requestCreation = await pispApi.makePaymentRequest({
creditTransferTransaction: [{
instructedAmount: {
amount: '12.25', // amount
currency: 'EUR' // currency code
},
beneficiary: {
creditor: {
name: 'Creditor Name' // payee (merchant) name
},
creditorAccount: {
iban: 'FI4966010005485495' // payee account number
}
}
}],
debtor: {
name: 'Debtor Name' // payer (account holder) name
},
debtorAccount: {
iban: 'FI3839390001384700' // payer account number
}
}, null, null, null);
import enablebanking
pisp_api = enablebanking.PISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
# Making payment request with credit transafer transaction of one element
request_creation = pisp_api.make_payment_request(
enablebanking.PaymentRequestResource(
credit_transfer_transaction=[
enablebanking.CreditTransferTransaction(
instructed_amount=enablebanking.AmountType(
amount='12.25', # amount
currency='EUR'), # currency code
beneficiary=enablebanking.Beneficiary(
creditor=enablebanking.PartyIdentification(
name='Creditor Name'), # payee (merchant) name
creditor_account=enablebanking.AccountIdentification(
iban='FI4966010005485495'), # payee account number
),
),
],
debtor=enablebanking.PartyIdentification(
name='Debtor Name'), # payer (account holder) name
debtor_account=enablebanking.AccountIdentification(
iban='FI3839390001384700'), # payer account number
))
import java.math.BigDecimal;
import java.util.Arrays;
import com.enablebanking.ApiClient;
import com.enablebanking.api.PispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
PispApi pispApi = new PispApi(new ApiClient(connectorSettings));
// Making payment request with credit transafer transaction of one element
HalPaymentRequestCreation requestCreation = pispApi.makePaymentRequest(
new PaymentRequestResource()
.creditTransferTransaction(Arrays.asList(
new CreditTransferTransaction()
.instructedAmount(
new AmountType()
.currency("EUR")
.amount(new BigDecimal("12.25")))
.beneficiary(
new Beneficiary()
.creditor(
new PartyIdentification()
.name("Creditor Name"))
.creditorAccount(
new AccountIdentification()
.iban("FI4966010005485495")))))
.debtor(
new PartyIdentification()
.name("Debtor Name"))
.debtorAccount(
new AccountIdentification()
.iban("FI3839390001384700")), null, null, null);
Payment request initiation (PISP)
Description
The following use cases can be applied:- payment request on behalf of a merchant
- transfer request on behalf of the account's owner
- standing-order request on behalf of the account's owner
Data content
A payment request or a transfer request might embed several payment instructions having- one single execution date or multiple execution dates,
- one single beneficiary or multiple beneficiaries.
Business flow
Payment Request use case
The PISP forwards a payment request on behalf of a merchant.The PSU buys some goods or services on an e-commerce website held by a merchant. Among other payment method, the merchant suggests the use of a PISP service. As there is obviously a contract between the merchant and the PISP, there is no need of such a contract between the PSU and this PISP to initiate the process.
Case of the PSU that chooses to use the PISP service:
- The merchant forwards the requested payment characteristics to the PISP and redirects the PSU to the PISP portal.
- The PISP requests from the PSU which ASPSP will be used.
- The PISP prepares the Payment Request and sends this request to the ASPSP.
- The Request can embed several payment instructions having different requested execution date.
- The beneficiary, as being the merchant, is set at the payment level.
Transfer Request use case
The PISP forwards a transfer request on behalf of the owner of the account.- The PSU provides the PISP with all information needed for the transfer.
- The PISP prepares the Transfer Request and sends this request to the relevant ASPSP that holds the debtor account.
- The Request can embed several payment instructions having different beneficiaries.
- The requested execution date, as being the same for all instructions, is set at the payment level.
Standing Order Request use case
The PISP forwards a Standing Order request on behalf of the owner of the account.- The PSU provides the PISP with all information needed for the Standing Order.
- The PISP prepares the Standing Order Request and sends this request to the relevant ASPSP that holds the debtor account.
- The Request embeds one single payment instruction with
- The requested execution date of the first occurrence
- The requested execution frequency of the payment in order to compute further execution dates
- An execution rule to handle cases when the computed execution dates cannot be processed (e.g. bank holydays)
- An optional end date for closing the standing Order
Authentication flows for all use cases
As the request posted by the PISP to the ASPSP needs a PSU authentication before execution, this request will include:- The specification of the authentication approaches that are supported by both the PISP and the ASPSP (any combination of "REDIRECT", "EMBEDDED" and "DECOUPLED" values).
- In case of possible REDIRECT or DECOUPLED authentication approach, one or two callback URLs will be passed to the ASPSP at the finalisation of the authentication and consent process. The URLs are set in the connector settings.
- In case of possible "EMBEDDED" or "DECOUPLED" approaches, the PSU identifier that can be processed by the ASPSP for PSU recognition must have been set within the [debtor] structure.
- A resource identification of the accepted request that can be further used to retrieve the request and its status information.
- The specification of the chosen authentication approach taking into account both the PISP and the PSU capabilities.
- In case of chosen REDIRECT authentication approach, the URL to be used by the PISP for redirecting the PSU in order to perform a authentication.
Redirect authentication approach
When the authentication approach within the ASPSP answer is set to "REDIRECT":- The PISP redirects the PSU to the ASPSP which authenticates the PSU.
- The ASPSP asks the PSU to give (or deny) his/her consent to the payment request
- The PSU chooses or confirms which of his/her accounts shall be used by the ASPSP for the future credit transfer.
- The ASPSP is then able to initiate the subsequent credit transfer.
- The ASPSP redirects the PSU to the PISP using one of the callback URLs.
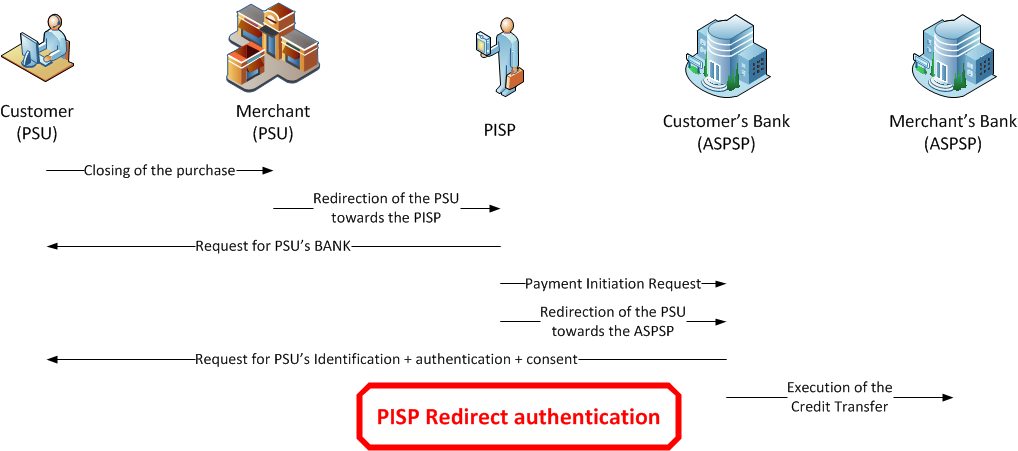
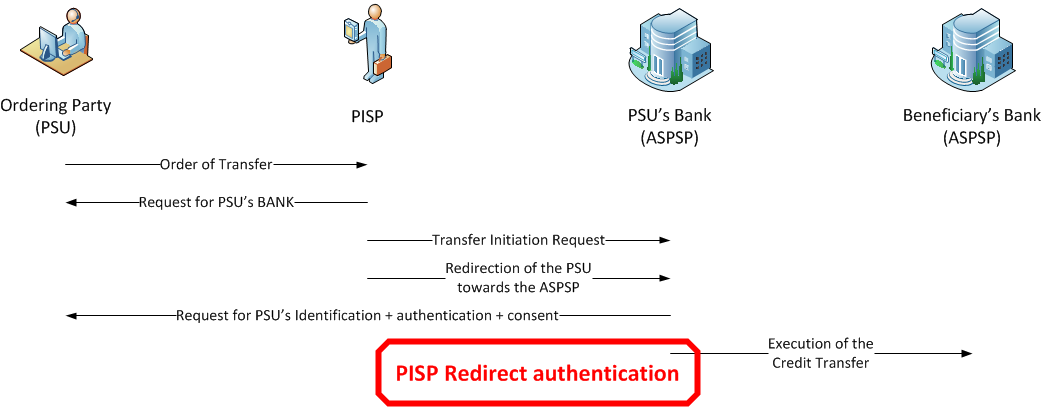
Decoupled authentication approach
When the authentication approach is "DECOUPLED":- Based on the PSU identifier provided within the payment request by the PISP, the ASPSP gives the PSU with the payment request details and challenges the PSU for a Strong Customer Authentication on a decoupled device or application.
- The PSU chooses or confirms which of his/her accounts shall be used by the ASPSP for the future credit transfer.
- The ASPSP is then able to initiate the subsequent credit transfer.
- The ASPSP notifies the PISP about the finalisation of the authentication and consent process by using one of the callback URLs.
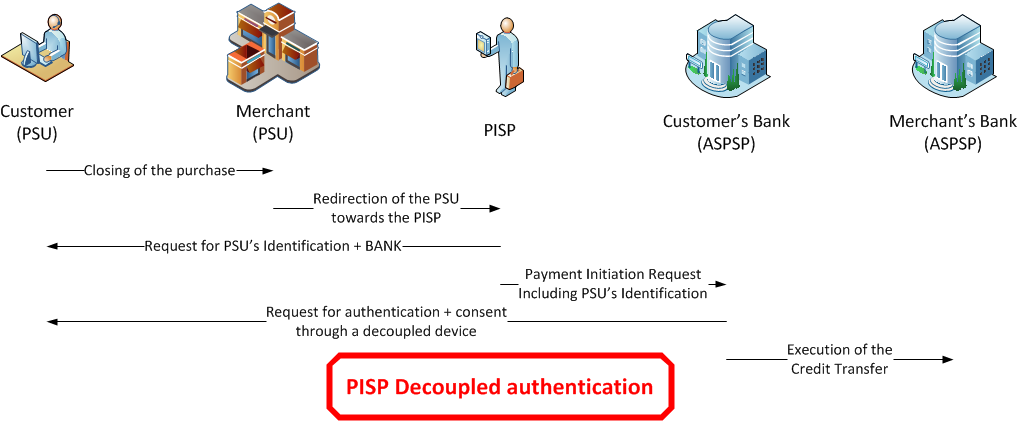
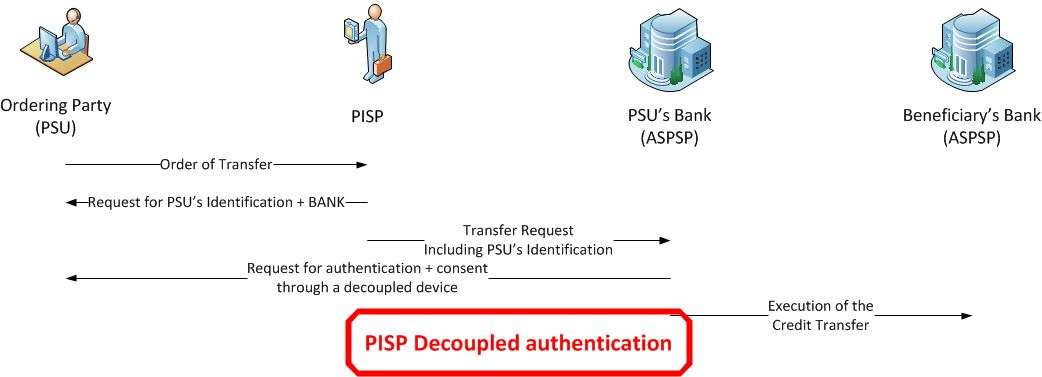
Embedded authentication approach
When the authentication approach within the ASPSP answers is set to "EMBEDDED":- The TPP informs the PSU that a challenge is needed for completing the Payment Request processing. This challenge will be one of the following:
- A One-Time-Password sent by the ASPSP to the PSU on a separate device or application.
- A response computed by a specific device on base of a challenge sent by the ASPSP to the PSU on a separate device or application.
- The PSU unlocks the device or application through a "knowledge factor" and/or an "inherence factor" (biometric), retrieves the payment request details and processes the data sent by the ASPSP.
- The PSU might choose to confirm which of his/her accounts shall be used by the ASPSP for the future credit transfer when the device or application allows it.
- When agreeing the payment request, the PSU enters the resulting authentication factor through the PISP interface.
- The PISP shall confirm the payment request using the authentication factor received from the PSU.
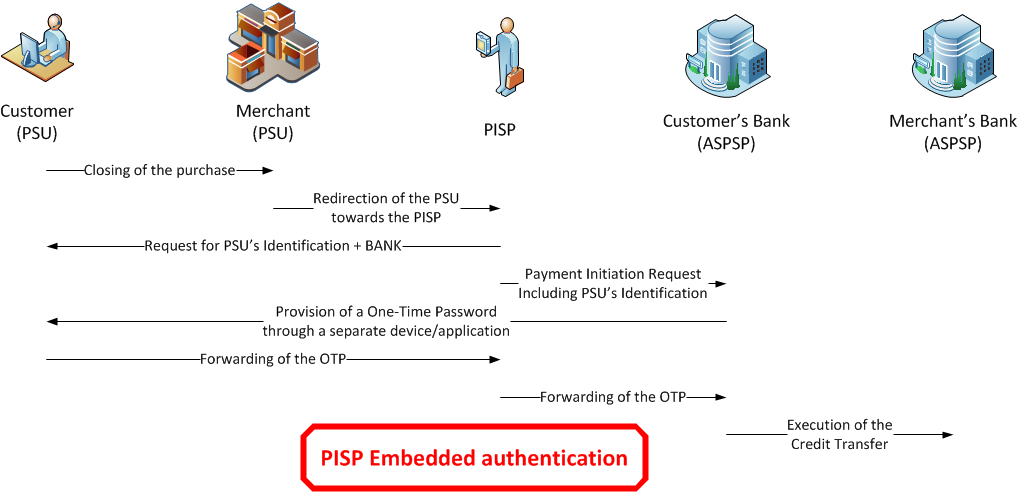
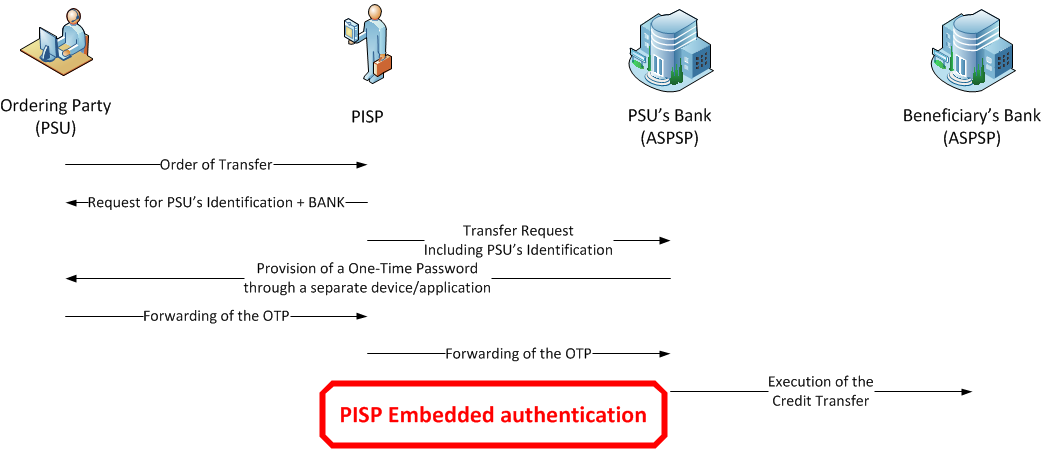
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentType | string | true | Payment type. Can be either SEPA, INST_SEPA, DOMESTIC or CROSSBORDER |
state | string | false | Arbitrary String to be returned from to redirect URI |
credentials | array[string] | false | Array of user credentials which are required to log in to the bank (e.g. user ID, password, phone number etc) |
method | string | false | Authentication method |
paymentRequest | PaymentRequestResource | true | ISO20022 based payment Initiation Request |
Returned value
Data type: HalPaymentRequestCreation
The request has been created as a resource. The ASPSP must authenticate the PSU.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
initiatePaymentRequestCancellation
Payment request cancellation (PISP)
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
state | string | false | Arbitrary String to be returned from to redirect URI |
credentials | array[string] | false | Array of user credentials which are required to log in to the bank (e.g. user ID, password, phone number etc) |
method | string | false | Authentication method |
Returned value
Data type: HalPaymentRequestCancellation
Cancellation successful
executePaymentRequestCancellation
Confirmation of a payment request or a modification request (PISP)
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
confirmation | PaymentRequestConfirmation | false | Data needed for confirmation of the Payment Request, especially in EMBEDDED approach |
Detailed descriptions
confirmation: Data needed for confirmation of the Payment Request, especially in EMBEDDED approach
Returned value
Data type: HalPaymentReuqestCancellationResult
retrieval of the Payment Cancellation enriched with the status report
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
validatePaymentRequest
Payment request initiation (PISP)
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentType | string | true | Payment type. Can be either SEPA, INST_SEPA, DOMESTIC or CROSSBORDER |
skipDebtorAccountValidation | boolean | false | Flag whether to skip debtor account validation |
paymentRequest | PaymentRequestResource | true | ISO20022 based payment Initiation Request |
Returned value
Data type: None
Validation successful
getPaymentRequest
Retrieval of a payment request (PISP)
Description
The following use cases can be applied:- retrieval of a payment request on behalf of a merchant
- retrieval of a transfer request on behalf of the account's owner
- retrieval of a standing-order request on behalf of the account's owner
The ASPSP has registered the request, updated if necessary the relevant identifiers in order to avoid duplicates and returned the updated request.
The PISP gets the request that might have been updated with the resource identifiers, the status of the payment/transfer request and the status of the subsequent credit transfer.
Prerequisites
- The TPP has previously posted a payment request which has been accepted by the ASPSP.
Business flow
The PISP asks to retrieve the payment/transfer request that has been accepted by the ASPSP. The PISP uses the payment request identifier provided by the ASPSP in response to the payment request.The ASPSP returns the previously accepted payment/transfer request, which is enriched with:
- The resource identifiers given by the ASPSP
- The status information of the Payment Request and of the subsequent credit transfer
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
Returned value
Data type: HalPaymentRequest
Retrieval of the previously posted Payment Request
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
getPaymentRequestTransaction
Retrieval of a payment request transaction (PISP)
Description
The PISP retrieves the transaction that has been initiated by the payment request.Prerequisites
- The TPP has previously posted a payment request which has been accepted by the ASPSP.
- The TPP has retrieved the accepted payment request in order to get the relevant resource IDs.
Business flow
The PISP asks to retrieve the transaction that has been initiated by the payment request. The PISP uses the payment request identifier provided by the ASPSP in response to the payment request.The ASPSP returns the previously initiated transaction.
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
paymentRequestTransactionResourceId | string | true | Identification of the Payment Request Transaction Resource |
Returned value
Data type: HalPaymentRequestTransaction
Retrieval of the previously initiated transaction
makePaymentRequestConfirmation
Confirmation of a payment request or a modification request (PISP)
Description
The PISP confirms one of the following requests- payment request on behalf of a merchant
- transfer request on behalf of the account's owner
- standing-order request on behalf of the account's owner
Prerequisites
- The TPP has previously set a request which has been accepted by the ASPSP.
- The TPP has retrieved the accpeted request in order to get the relevant resource IDs.
- The TPP provided the PSU necessary information and means for SCA required before the confirmation.
Business flow
Once the PSU has been authenticated, it is the due to the PISP to confirm the Request to the ASPSP in order to complete the process flow.In REDIRECT and DECOUPLED approach, this confirmation is not a prerequisite to the execution of the Credit Transfer.
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
confirmation | PaymentRequestConfirmation | false | Data needed for confirmation of the Payment Request, especially in EMBEDDED approach |
Detailed descriptions
confirmation: Data needed for confirmation of the Payment Request, especially in EMBEDDED approach
Returned value
Data type: HalPaymentRequest
retrieval of the Payment Request enriched with the status report
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
CBPII API
makeFundsConfirmation
Payment coverage check request (CBPII)
Description
The CBPII can ask an ASPSP to check if a given amount can be covered by the liquidity that is available on a PSU cash account or payment card.Business flow
The CBPII requests the ASPSP for a payment coverage check against either a bank account or a card primary identifier.The ASPSP answers with a structure embedding the original request and the result as a Boolean.
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentCoverage | PaymentCoverageRequestResource | true | parameters of a payment coverage request |
Returned value
Data type: HalPaymentCoverageReport
payment coverage request
Data types
Access
Requested access services.
Fields
Name | Type | Required | Description |
---|---|---|---|
accounts |
false |
List of accounts access to which is requested. If not set behaviour depends on the bank: some banks allow users to choose list of accessible accounts through their access consent UI, while other may provide access to all accounts or just access to the list of accounts. |
|
balances |
boolean |
false |
Defines whether balance information is requested. |
transactions |
boolean |
false |
Defines whether transactions information is requested. |
transactionsLimitDays |
integer |
false |
Defines the maximum number of days for which transactions are requested. Zero means no explicit limit. |
paymentInitiation |
boolean |
false |
Defines whether access to payments initiation is requested. |
trustedBeneficiaries |
boolean |
false |
Indicator that access to the trusted beneficiaries list was requested or not to the AISP by the PSU
|
recurringIndicator |
boolean |
false |
|
frequencyPerDay |
integer |
false |
This field indicates the requested maximum frequency for an access without PSU involvement per day. For a one-off access, this attribute is set to "1". The frequency needs to be greater equal to one. |
validUntil |
string(date-time) |
false |
This parameter is requesting a valid until date for the requested consent. The content is the local ASPSP date in ISO-Date Format, e.g. 2017-10-30. Future dates might get adjusted by ASPSP. |
AccountIdentification
Unique and unambiguous identification for the account between the account owner and the account servicer.
Fields
Name | Type | Required | Description |
---|---|---|---|
iban |
string |
false |
ISO20022: International Bank Account Number (IBAN) - identification used internationally by financial institutions to uniquely identify the account of a customer. Further specifications of the format and content of the IBAN can be found in the standard ISO 13616 "Banking and related financial services - International Bank Account Number (IBAN)" version 1997-10-01, or later revisions. |
other |
false |
ISO20022: Unique identification of an account, a person or an organisation, as assigned by an issuer. |
AccountResource
PSU account that is made available to the TPP
Fields
Name | Type | Required | Description |
---|---|---|---|
resourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
accountServicer |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
accountId |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
allAccountIds |
false |
All account identifiers provided by ASPSPs (including primary identifier available in the accountId field) |
|
name |
string |
false |
Account holder(s) name |
details |
string |
false |
Account description set by PSU or provided by ASPSP |
usage |
string |
false |
Specifies the usage of the account |
cashAccountType |
string |
true |
Specifies the type of the account |
product |
string |
false |
Product Name of the Bank for this account, proprietary definition |
currency |
true |
Currency used for the account |
|
creditLimit |
false |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
balances |
false |
list of balances provided by the ASPSP |
|
psuStatus |
string |
false |
Relationship between the PSU and the account - Account Holder - Co-account Holder - Attorney |
postalAddress |
false |
ISO20022 : Information that locates and identifies a specific address, as defined by postal services. |
Enumerated Values
usage
Value | Description |
---|---|
PRIV |
private personal account |
ORGA |
professional account |
cashAccountType
Value | Description |
---|---|
CACC |
Account used to post debits and credits when no specific account has been nominated |
CASH |
Account used for the payment of cash |
CARD |
Account used for card payments only |
LOAN |
Account used for loans |
SVGS |
Account used for savings |
OTHR |
Account not otherwise specified |
AddressType
ISO20022: Identifies the nature of the postal address.
Base type
string
Enumerated Values
Value | Description |
---|---|
Business |
— |
Correspondence |
— |
DeliveryTo |
— |
MailTo |
— |
POBox |
— |
Postal |
— |
Residential |
— |
Statement |
— |
AmountType
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used.
API: only instructed amount can be used
Fields
Name | Type | Required | Description |
---|---|---|---|
currency |
true |
Specifies the currency of the amount or of the account. |
|
amount |
string(number) |
true |
ISO20022: Amount of money to be moved between the debtor and creditor, before deduction of charges, expressed in the currency as ordered by the initiating party. |
Auth
Fields
Name | Type | Required | Description |
---|---|---|---|
url |
string |
false |
URL for authorization. Used for REDIRECT authorization flow |
env |
string |
false |
Additional authorization environment data. To be passed to makeToken. |
authData |
false |
[structure used for returning auth parameters to be passed to user] |
AuthDataItem
structure used for returning auth parameters to be passed to user
Fields
Name | Type | Required | Description |
---|---|---|---|
type |
string |
false |
Type of the auth data item |
value |
string |
false |
Value of auth data item |
Enumerated Values
type
Value | Description |
---|---|
otpIndex |
— |
message |
— |
APP |
— |
IMAGE_LINK |
— |
IMAGE_RENDER |
— |
IMAGE_B64 |
— |
AuthInfo
Information about usage of getAuth
method
Fields
Name | Type | Required | Description |
---|---|---|---|
access |
boolean |
true |
Shows if access parameter is supported in |
credentials |
false |
Shows necessary credentials needed to provide in |
|
authData |
boolean |
false |
Shows if |
authUrlHints |
true |
Shows which actions can be done with url returned from |
|
brands |
[Brand] |
false |
List of brands used by the ASPSP (in a certain country) |
AuthRedirect
structure used for returning parameters of redirect url
Fields
Name | Type | Required | Description |
---|---|---|---|
code |
string |
false |
redirect code received from a bank |
id_token |
string |
false |
Id of token received from a bank |
state |
string |
false |
state specified by TPP |
error |
string |
false |
error message |
error_description |
string |
false |
error description |
consent_id |
string |
false |
consent id |
AuthUrlHint
Auxiliary data structure binding information about usage of an url returned from getAuth
method
Fields
Name | Type | Required | Description |
---|---|---|---|
authType |
false |
Action which can be done with url returned from |
|
refresh |
boolean |
true |
Shows if the url needs to be refreshed |
refreshFrequency |
integer |
false |
Shows how often the url needs to be refreshed. Applicable only if refresh is true |
AuthUrlType
Action which can be done with url returned from getAuth
Base type
string
Enumerated Values
Value | Description |
---|---|
REDIRECT |
PSU needs to be redirected to the URL |
IFRAME |
the url needs to be displayed in an iframe |
APP |
the url needs to be opened in a mobile app |
IMAGE_LINK |
Link to an image which needs to be displayed |
IMAGE_RENDER |
Image data which needs to be displayed |
IMAGE_B64 |
Image data in base64 format which needs to be displayed |
AuthenticationApproach
The ASPSP, based on the authentication approaches proposed by the PISP, choose the one that it can processed, in respect with the preferences and constraints of the PSU and indicates in this field which approach has been chosen
Base type
string
Enumerated Values
Value | Description |
---|---|
REDIRECT |
the PSU is redirected by the TPP to the ASPSP which processes identification and authentication |
DECOUPLED |
the TPP identifies the PSU and forwards the identification to the ASPSP which processes the authentication through a decoupled device |
EMBEDDED |
the TPP identifies the PSU and forwards the identification to the ASPSP which starts the authentication. The TPP forwards one authentication factor of the PSU (e.g. OTP or response to a challenge) |
BalanceResource
Structure of an account balance
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
true |
Label of the balance |
balanceAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
balanceType |
true |
Type of balance |
|
lastChangeDateTime |
string(date-time) |
false |
Timestamp of the last change of the balance amount |
referenceDate |
string(date) |
false |
Reference date for the balance |
lastCommittedTransaction |
string |
false |
Identification of the last committed transaction. This is actually useful for instant balance. |
BalanceStatus
Type of balance
Base type
string
Enumerated Values
Value | Description |
---|---|
CLAV |
(ISO20022 Closing Available) Closing available balance |
CLBD |
(ISO20022 ClosingBooked) Accounting Balance |
FWAV |
(ISO20022 ForwardAvailable) Balance of money that is at the disposal of the account owner on the date specified |
INFO |
(ISO20022 Information) Balance for informational purposes |
ITAV |
(ISO20022 InterimAvailable) Available balance calculated in the course of the day |
ITBD |
(ISO20022 InterimBooked) Booked balance calculated in the course of the day |
OPAV |
(ISO20022 OpeningAvailable) Opening balance of amount of money that is at the disposal of the account owner on the date specified |
OPBD |
(ISO20022 OpeningBooked) Book balance of the account at the beginning of the account reporting period. It always equals the closing book balance from the previous report |
PRCD |
(ISO20022 PreviouslyClosedBooked) Balance of the account at the end of the previous reporting period |
OTHR |
Other Balance |
VALU |
Value-date balance |
XPCD |
(ISO20022 Expected) Instant Balance |
BankTransactionCode
ISO20022: Allows the account servicer to correctly report a transaction, which in its turn will help account owners to perform their cash management and reconciliation operations.
Fields
Name | Type | Required | Description |
---|---|---|---|
description |
string |
false |
Transaction type description |
code |
string |
false |
ISO20022: Specifies the family of a transaction within the domain |
subCode |
string |
false |
ISO20022: Specifies the sub-product family of a transaction within a specific family |
Beneficiary
Specification of a beneficiary
Fields
Name | Type | Required | Description |
---|---|---|---|
creditorAgent |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
creditor |
false |
API : Description of a Party which can be either a person or an organization. |
|
creditorAccount |
true |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
creditorCurrency |
string |
false |
Creditor account currency code |
BookingInformation
indicator that the payment can be immediately booked or not
- true: payment is booked
- false: payment is not booked
Base type
boolean
Brand
Information about ASPSP end-user recognizable brand
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
true |
End-customer facing name |
logos |
[Logo] |
false |
List of available logos |
bic |
string |
false |
ASPSP BIC |
CategoryPurposeCode
ISO20022: Specifies the high level purpose of the instruction based on a set of pre-defined categories. This is used by the initiating party to provide information concerning the processing of the payment. It is likely to trigger special processing by any of the agents involved in the payment chain.
Base type
string
Enumerated Values
Value | Description |
---|---|
BONU |
BonusPayment Transaction is the payment of a bonus. |
CASH |
CashManagementTransfer Transaction is a general cash management instruction. |
CBLK |
Card Bulk Clearing A Service that is settling money for a bulk of card transactions, while referring to a specific transaction file or other information like terminal ID, card acceptor ID or other transaction details. |
CCRD |
Credit Card Payment Transaction is related to a payment of credit card. |
CORT |
TradeSettlementPayment Transaction is related to settlement of a trade, eg a foreign exchange deal or a securities transaction. |
DCRD |
Debit Card Payment Transaction is related to a payment of debit card. |
DIVI |
Dividend Transaction is the payment of dividends. |
DVPM |
DeliverAgainstPayment Code used to pre-advise the account servicer of a forthcoming deliver against payment instruction. |
EPAY |
Epayment Transaction is related to ePayment. |
FCOL |
Fee Collection A Service that is settling card transaction related fees between two parties. |
GOVT |
GovernmentPayment Transaction is a payment to or from a government department. |
HEDG |
Hedging Transaction is related to the payment of a hedging operation. |
ICCP |
Irrevocable Credit Card Payment Transaction is reimbursement of credit card payment. |
IDCP |
Irrevocable Debit Card Payment Transaction is reimbursement of debit card payment. |
INTC |
IntraCompanyPayment Transaction is an intra-company payment, ie, a payment between two companies belonging to the same group. |
INTE |
Interest Transaction is the payment of interest. |
LOAN |
Loan Transaction is related to the transfer of a loan to a borrower. |
MP2B |
Commercial Mobile P2B Payment |
MP2P |
Consumer Mobile P2P Payment |
OTHR |
OtherPayment Other payment purpose. |
PENS |
PensionPayment Transaction is the payment of pension. |
RPRE |
Represented Collection used to re-present previously reversed or returned direct debit transactions. |
RRCT |
ReimbursementReceivedCreditTransfer Transaction is related to a reimbursement for commercial reasons of a correctly received credit transfer. |
RVPM |
ReceiveAgainstPayment Code used to pre-advise the account servicer of a forthcoming receive against payment instruction. |
SALA |
SalaryPayment Transaction is the payment of salaries. |
SECU |
Securities Transaction is the payment of securities. |
SSBE |
SocialSecurityBenefit Transaction is a social security benefit, ie payment made by a government to support individuals. |
SUPP |
SupplierPayment Transaction is related to a payment to a supplier. |
TAXS |
TaxPayment Transaction is the payment of taxes. |
TRAD |
Trade Transaction is related to the payment of a trade finance transaction. |
TREA |
TreasuryPayment Transaction is related to treasury operations. E.g. financial contract settlement. |
VATX |
ValueAddedTaxPayment Transaction is the payment of value added tax. |
WHLD |
WithHolding Transaction is the payment of withholding tax. |
ChargeBearerCode
ISO20022: Specifies which party/parties will bear the charges associated with the processing of the payment transaction.
Base type
string
Enumerated Values
Value | Description |
---|---|
SLEV |
Service level. Charges are to be applied following the rules agreed in the service level and/or scheme. |
SHAR |
Shared. |
DEBT |
The Payer (sender of the payment) will bear all of the payment transaction fees. |
CRED |
The Payee (recipient of the payment) will incur all of the payment transaction fees. |
ClearingSystemMemberIdentification
ISO20022: Information used to identify a member within a clearing system. API: to be used for some specific international credit transfers in order to identify the beneficiary bank
Fields
Name | Type | Required | Description |
---|---|---|---|
clearingSystemId |
string |
false |
ISO20022: Specification of a pre-agreed offering between clearing agents or the channel through which the payment instruction is processed. |
memberId |
string |
false |
ISO20022: Identification of a member of a clearing system. |
ClientInfo
Client Data.
Fields
Name | Type | Required | Description |
---|---|---|---|
psuIpAddress |
string |
false |
IP address used by the PSU's terminal when connecting to the TPP |
psuIpPort |
string |
false |
IP port used by the PSU's terminal when connecting to the TPP |
psuHttpMethod |
string |
false |
Http method for the most relevant PSU’s terminal request to the TTP |
psuDate |
string |
false |
Timestamp of the most relevant PSU’s terminal request to the TTP |
psuUserAgent |
string |
false |
"User-Agent" header field sent by the PSU terminal when connecting to the TPP |
psuReferer |
string |
false |
"Referer" header field sent by the PSU terminal when connecting to the TPP. Notice that an initial typo in RFC 1945 specifies that "referer" (incorrect spelling) is to be used. The correct spelling "referrer" can be used but might not be understood. |
psuAccept |
string |
false |
"Accept" header field sent by the PSU terminal when connecting to the TPP |
psuAcceptCharset |
string |
false |
"Accept-Charset" header field sent by the PSU terminal when connecting to the TPP |
psuAcceptEncoding |
string |
false |
"Accept-Encoding" header field sent by the PSU terminal when connecting to the TPP |
psuAcceptLanguage |
string |
false |
"Accept-Language" header field sent by the PSU terminal when connecting to the TPP |
psuGeoLocation |
string |
false |
Geographical location of the PSU as provided by the PSU mobile terminal if any to the TPP |
psuDeviceId |
string |
false |
UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of installation identification this ID need to be unaltered until removal from device. |
psuLastLoggedTime |
string |
false |
The time when the PSU last logged in with the TPP. |
Connector
Information about the connector used for interaction with ASPSPs
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
true |
Name of the connector |
environments |
[string] |
true |
List of supported environments (sandbox/production) |
countries |
[string] |
true |
List of supported countries |
languages |
[string] |
false |
List of supported languages |
scopes |
[string] |
true |
List of supported APIs (aisp/pisp) supported |
modifyConsentsInfo |
true |
Details about usage of |
|
authInfo |
true |
Details about usage of |
|
transactionsInfo |
false |
Details about usage and limitations of |
|
consentRevocation |
boolean |
true |
Flag showing if the consent revocation is supported in the connector |
maximumConsentValidity |
integer |
true |
Maximum consent validity in seconds |
refreshToken |
boolean |
true |
Flag showing if the |
payments |
true |
Supported payment types by country |
|
aspspIds |
[string] |
true |
List of all ASPSP IDs supported in the connector |
requiredPsuHeaders |
[string] |
true |
List of required PSU headers |
psuTypes |
[string] |
true |
List of supported PSU types (personal/business) |
authMethods |
true |
List of supported authentication methods by country |
|
betaAisp |
boolean |
true |
Flag showing if the connector's AISP implementation is in beta |
betaPisp |
boolean |
true |
Flag showing if the connector's PISP implementation is in beta |
psuIpAddressV6Supported |
boolean |
true |
Flag showing if the connector supports IPv6 as PSU-IP-Address |
deprecated |
boolean |
true |
Flag showing if the connector is deprecated |
transactionHistoryDays |
integer |
false |
Number of days for which transaction history is available |
pisAccountsAvailable |
boolean |
true |
Flag showing if the connector supports fetching list of accounts with only PIS scope |
ConnectorSettings
Base settings for all connectors
Fields
Name | Type | Required | Description |
---|---|---|---|
sandbox |
boolean |
true |
Defines whether connect to sandbox or production APIs |
consentId |
string |
true |
User consent identifier |
accessToken |
string |
true |
User access token |
refreshToken |
string |
true |
User refresh token |
redirectUri |
string |
true |
URI where clients are redirected to after giving consent. |
connector |
string |
true |
Connector identifier (discriminator) |
Consent
User consent
Fields
Name | Type | Required | Description |
---|---|---|---|
consentStatus |
string |
false |
none |
consentId |
string |
false |
none |
env |
string |
false |
Additional authorization environment data. To be passed to makeToken. |
authData |
false |
[structure used for returning auth parameters to be passed to user] |
|
_links |
false |
none |
ContactDetails
Contact details of a person.
Fields
Name | Type | Required | Description |
---|---|---|---|
emailAddress |
string |
false |
Email address of a person |
phoneNumber |
string |
false |
Phone number of a person |
CountryAuthInfo
Auxiliary data structure binding information about usage of getAuth
method to a country and authentication method
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code |
method |
string |
false |
Authentication method |
hiddenMethod |
boolean |
true |
Flag showing whether this authentication method is hidden from the list of authentication methods This method still can be chosen and expected to work |
title |
string |
false |
Authentication method human readable name |
info |
true |
Information about usage of |
|
approach |
true |
The ASPSP, based on the authentication approaches proposed by the PISP, choose the one that it can processed, in respect with the preferences and constraints of the PSU and indicates in this field which approach has been chosen |
CountryAuthMethods
Auxiliary data structure binding information about usage of getAuthMethods
method to a country
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code |
info |
[string] |
true |
Information about available auth methods |
CountryModifyConsentsInfo
Auxiliary data structure binding information about usage of modifyConsents
method
to a country
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code |
info |
true |
Information about usage of |
CountryPaymentTypes
Auxiliary data structure containing binding information about supported payment types to a country
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code |
paymentTypes |
true |
List of payment types for the country |
CreationDateTime
ISO20022: Date and time at which a (group of) payment instruction(s) was created by the instructing party.
Base type
string(date-time)
Credential
User credentials to provide in getAuth
call
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
true |
Name of user credential. (userId, password, companyId, phoneNumber, personalCode, etc) |
title |
string |
true |
Human readable and formatted name of user credential. (User ID, Password, Company ID, Phone Number, Personal Code, etc) |
required |
boolean |
true |
Shows if it is necessary to provide credential in |
description |
string |
false |
Description of the field. |
template |
string |
false |
Shows required format for the credential (if any) |
CreditTransferTransaction
Payment instruction to transfer instructed amount of money to the specified beneficiary.
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentId |
false |
ISO20022: Set of elements used to reference a payment instruction. |
|
requestedExecutionDate |
false |
ISO20022: Date at which the initiating party requests the clearing agent to process the payment. |
|
referenceNumber |
false |
ISO20022: This field specifies the reference assigned by the sender to unambiguously identify the message. |
|
endDate |
false |
The last applicable day of execution for a given standing order. |
|
executionRule |
false |
Execution date shifting rule for standing orders |
|
frequency |
false |
Frequency rule for standing orders. |
|
instructedAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
ultimateDebtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
beneficiary |
true |
Specification of a beneficiary |
|
ultimateCreditor |
false |
API : Description of a Party which can be either a person or an organization. |
|
regulatoryReporting |
false |
[Information needed due to regulatory and statutory requirements.] |
|
remittanceInformation |
false |
Payment details. For credit transfers may contain free text, reference number or both at the same time (in case Extended Remittance Information is supported). When it is known that remittance information contains a reference number (either based on ISO 11649 or a local scheme), the reference number is also available via the |
CreditTransferTransactionDetails
Payment instruction to transfer instructed amount of money to the specified beneficiary.
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentId |
false |
ISO20022: Set of elements used to reference a payment instruction. |
|
resourceId |
false |
ISO20022 : Reference assigned by a sending party to unambiguously identify the transaction information block within the message. |
|
transactionStatus |
false |
ISO20022: Specifies the status of the payment information. |
|
requestedExecutionDate |
false |
ISO20022: Date at which the initiating party requests the clearing agent to process the payment. |
|
referenceNumber |
false |
ISO20022: This field specifies the reference assigned by the sender to unambiguously identify the message. |
|
endDate |
false |
The last applicable day of execution for a given standing order. |
|
executionRule |
false |
Execution date shifting rule for standing orders |
|
frequency |
false |
Frequency rule for standing orders. |
|
instructedAmount |
false |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
ultimateDebtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
beneficiary |
false |
Specification of a beneficiary |
|
ultimateCreditor |
false |
API : Description of a Party which can be either a person or an organization. |
|
regulatoryReporting |
false |
[Information needed due to regulatory and statutory requirements.] |
|
remittanceInformation |
false |
Payment details. For credit transfers may contain free text, reference number or both at the same time (in case Extended Remittance Information is supported). When it is known that remittance information contains a reference number (either based on ISO 11649 or a local scheme), the reference number is also available via the |
CurrencyCode
Specifies the currency of the amount or of the account. A code allocated to a currency by a Maintenance Agency under an international identification scheme, as described in the latest edition of the international standard ISO 4217 "Codes for the representation of currencies and funds".
Base type
string
CurrencyCodeEnum
Specifies the currency of the amount or of the account. A code allocated to a currency by a Maintenance Agency under an international identification scheme, as described in the latest edition of the international standard ISO 4217 "Codes for the representation of currencies and funds".
Base type
string
Enumerated Values
Value | Description |
---|---|
AED |
United Arab Emirates dirham |
AFN |
Afghan afghani |
ALL |
Albanian lek |
AMD |
Armenian dram |
ANG |
Netherlands Antillean guilder |
AOA |
Angolan kwanza |
ARS |
Argentine peso |
AUD |
Australian dollar |
AWG |
Aruban florin |
AZN |
Azerbaijani manat |
BAM |
Bosnia and Herzegovina convertible mark |
BBD |
Barbados dollar |
BDT |
Bangladeshi taka |
BGN |
Bulgarian lev |
BHD |
Bahraini dinar |
BIF |
Burundian franc |
BMD |
Bermudian dollar |
BND |
Brunei dollar |
BOB |
Boliviano |
BOV |
Bolivian Mvdol (funds code) |
BRL |
Brazilian real |
BSD |
Bahamian dollar |
BTN |
Bhutanese ngultrum |
BWP |
Botswana pula |
BYN |
Belarusian ruble |
BZD |
Belize dollar |
CAD |
Canadian dollar |
CDF |
Congolese franc |
CHE |
WIR euro (complementary currency) |
CHF |
Swiss franc |
CNH |
Chinese yuan |
CHW |
WIR franc (complementary currency) |
CLF |
Unidad de Fomento (funds code) |
CLP |
Chilean peso |
CNY |
Chinese yuan |
COP |
Colombian peso |
COU |
Unidad de Valor Real |
CRC |
Costa Rican colon |
CUC |
Cuban convertible peso |
CUP |
Cuban peso |
CVE |
Cape Verdean escudo |
CZK |
Czech koruna |
DJF |
Djiboutian franc |
DKK |
Danish krone |
DOP |
Dominican peso |
DZD |
Algerian dinar |
EGP |
Egyptian pound |
ERN |
Eritrean nakfa |
ETB |
Ethiopian birr |
EUR |
Euro |
FJD |
Fiji dollar |
FKP |
Falkland Islands pound |
GBP |
Pound sterling |
GEL |
Georgian lari |
GHS |
Ghanaian cedi |
GIP |
Gibraltar pound |
GMD |
Gambian dalasi |
GNF |
Guinean franc |
GTQ |
Guatemalan quetzal |
GYD |
Guyanese dollar |
HKD |
Hong Kong dollar |
HNL |
Honduran lempira |
HRK |
Croatian kuna |
HTG |
Haitian gourde |
HUF |
Hungarian forint |
IDR |
Indonesian rupiah |
ILS |
Israeli new shekel |
INR |
Indian rupee |
IQD |
Iraqi dinar |
IRR |
Iranian rial |
ISK |
Icelandic króna |
JMD |
Jamaican dollar |
JOD |
Jordanian dinar |
JPY |
Japanese yen |
KES |
Kenyan shilling |
KGS |
Kyrgyzstani som |
KHR |
Cambodian riel |
KMF |
Comoro franc |
KPW |
North Korean won |
KRW |
South Korean won |
KWD |
Kuwaiti dinar |
KYD |
Cayman Islands dollar |
KZT |
Kazakhstani tenge |
LAK |
Lao kip |
LBP |
Lebanese pound |
LKR |
Sri Lankan rupee |
LRD |
Liberian dollar |
LSL |
Lesotho loti |
LYD |
Libyan dinar |
MAD |
Moroccan dirham |
MDL |
Moldovan leu |
MGA |
Malagasy ariary |
MKD |
Macedonian denar |
MMK |
Myanmar kyat |
MNT |
Mongolian tögrög |
MOP |
Macanese pataca |
MRU |
Mauritanian ouguiya |
MUR |
Mauritian rupee |
MVR |
Maldivian rufiyaa |
MWK |
Malawian kwacha |
MXN |
Mexican peso |
MXV |
Mexican Unidad de |
MYR |
Malaysian ringgit |
MZN |
Mozambican metical |
NAD |
Namibian dollar |
NGN |
Nigerian naira |
NIO |
Nicaraguan córdoba |
NOK |
Norwegian krone |
NPR |
Nepalese rupee |
NZD |
New Zealand dollar |
OMR |
Omani rial |
PAB |
Panamanian balboa |
PEN |
Peruvian sol |
PGK |
Papua New Guinean kina |
PHP |
Philippine peso |
PKR |
Pakistani rupee |
PLN |
Polish złoty |
PYG |
Paraguayan guaraní |
QAR |
Qatari riyal |
RON |
Romanian leu |
RSD |
Serbian dinar |
RUB |
Russian ruble |
RWF |
Rwandan franc |
SAR |
Saudi riyal |
SBD |
Solomon Islands dollar |
SCR |
Seychelles rupee |
SDG |
Sudanese pound |
SEK |
Swedish krona/kronor |
SGD |
Singapore dollar |
SHP |
Saint Helena pound |
SLL |
Sierra Leonean leone |
SOS |
Somali shilling |
SRD |
Surinamese dollar |
SSP |
South Sudanese pound |
STN |
São Tomé and Príncipe dobra |
SVC |
Salvadoran colón |
SYP |
Syrian pound |
SZL |
Swazi lilangeni |
THB |
Thai baht |
TJS |
Tajikistani somoni |
TMT |
Turkmenistan manat |
TND |
Tunisian dinar |
TOP |
Tongan paʻanga |
TRY |
Turkish lira |
TTD |
Trinidad and Tobago dollar |
TWD |
New Taiwan dollar |
TZS |
Tanzanian shilling |
UAH |
Ukrainian hryvnia |
UGX |
Ugandan shilling |
USD |
United States dollar |
USN |
United States dollar (next day) (funds code) United States |
UYI |
Uruguay Peso en Unidades Indexadas (URUIURUI) (funds code) |
UYU |
Uruguayan peso |
UYW |
Unidad previsional |
UZS |
Uzbekistan som |
VES |
Venezuelan bolívar soberano |
VND |
Vietnamese đồng |
VUV |
Vanuatu vatu |
WST |
Samoan tala |
XAF |
CFA franc BEAC |
XCD |
East Caribbean dollar |
XOF |
CFA franc BCEAO |
XPF |
CFP franc (franc Pacifique) |
YER |
Yemeni rial |
ZAR |
South African rand |
ZMW |
Zambian kwacha |
ZWL |
Zimbabwean dollar |
EndDate
The last applicable day of execution for a given standing order. If not given, the standing order is considered as endless.
Base type
string(date-time)
EpirusBankConnectorSettings
Settings for initializing ApiClient for Epirus Bank API
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL certificate in PEM format |
ExchangeRate
Provides details on the currency exchange rate and contract.
Fields
Name | Type | Required | Description |
---|---|---|---|
unitCurrency |
false |
Currency in which the rate of exchange is expressed in a currency exchange. In the example 1GBP = xxxCUR, the unit currency is GBP. |
|
instructedAmount |
false |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
exchangeRate |
string(number) |
false |
The factor used for conversion of an amount from one currency to another. This reflects the price at which one currency was bought with another currency. |
rateType |
string |
false |
none |
contractIdentification |
string |
false |
Unique and unambiguous reference to the foreign exchange contract agreed between the initiating party/creditor and the debtor agent. |
Enumerated Values
rateType
Value | Description |
---|---|
SPOT |
Spot – Exchange rate applied is the spot rate. |
SALE |
Sale – Exchange rate applied is the market rate at the time of the sale. |
AGRD |
Agreed – Exchange rate applied is the rate agreed between the parties. |
ExecutionRule
Execution date shifting rule for standing orders This data attribute defines the behaviour when recurring payment dates falls on a weekend or bank holiday. The payment is then executed either the "preceding" or "following" working day. ASPSP might reject the request due to the communicated value, if rules in Online-Banking are not supporting this execution rule.
Base type
string
Enumerated Values
Value | Description |
---|---|
FWNG |
following |
PREC |
preceding |
FinancialInstitutionIdentification
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme.
Fields
Name | Type | Required | Description |
---|---|---|---|
bicFi |
string |
false |
ISO20022: Code allocated to a financial institution by the ISO 9362 Registration Authority as described in ISO 9362 "Banking - Banking telecommunication messages - Business identification code (BIC)". |
clearingSystemMemberId |
false |
ISO20022: Information used to identify a member within a clearing system. |
|
name |
string |
false |
Name of the financial institution |
postalAddress |
false |
ISO20022 : Information that locates and identifies a specific address, as defined by postal services. |
FrequencyCode
Frequency rule for standing orders. The following codes from the "EventFrequency7Code" of ISO 20022 are supported. However, each ASPSP might restrict these values into a subset.
Base type
string
Enumerated Values
Value | Description |
---|---|
DAIL |
Daily |
WEEK |
Weekly |
TOWK |
EveryTwoWeeks |
MNTH |
Monthly |
TOMN |
EveryTwoMonths |
QUTR |
Quarterly |
SEMI |
SemiAnnual |
YEAR |
Annual |
FundsAvailabilityInformation
indicator that the payment can be covered or not by the funds available on the relevant account
- true: payment is covered
- false: payment is not covered
Base type
boolean
GenericIdentification
ISO20022: Unique identification of an account, a person or an organisation, as assigned by an issuer. API: The ASPSP will document which account reference type it will support.
Fields
Name | Type | Required | Description |
---|---|---|---|
identification |
string |
true |
API Identifier |
schemeName |
true |
Name of the identification scheme. Partially based on ISO20022 external code list |
|
issuer |
string |
false |
ISO20022: Entity that assigns the identification. this could a country code or any organisation name or identifier that can be recognized by both parties |
HalAccounts
HYPERMEDIA structure used for returning the list of the available accounts to the AISP
Fields
Name | Type | Required | Description |
---|---|---|---|
connectedPsu |
string |
false |
Last name and first name that has granted access to the AISP on the accounts data This information can be retrieved based on the PSU's authentication that occurred during the OAUTH2 access token initialisation. |
accounts |
true |
List of PSU account that are made available to the TPP |
HalBalances
HYPERMEDIA structure used for returning the list of the relevant balances for a given account to the AISP
Fields
Name | Type | Required | Description |
---|---|---|---|
balances |
true |
List of account balances |
HalBeneficiaries
HYPERMEDIA structure used for returning the list of the whitelisted beneficiaries
Fields
Name | Type | Required | Description |
---|---|---|---|
beneficiaries |
true |
List of trusted beneficiaries |
HalConnectors
HYPERMEDIA structure used for returning the list of the connectors for given countries
Fields
Name | Type | Required | Description |
---|---|---|---|
connectors |
true |
List of connectors |
HalPaymentCoverageReport
HYPERMEDIA structure used for returning the payment coverage report to the CBPII
Fields
Name | Type | Required | Description |
---|---|---|---|
request |
true |
Payment coverage request structure. The request must rely either on a cash account or a payment card. |
|
result |
boolean |
true |
Result of the coverage check :
|
HalPaymentRequest
HYPERMEDIA structure used for returning the original Payment Request to the PISP
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
paymentRequest |
false |
ISO20022: The PaymentRequestResourceDetails message is sent by the Creditor sending party to the Debtor receiving party, directly or through agents. It is used by a Creditor to request movement of funds from the debtor account to a creditor. |
|
paymentInformationStatus |
false |
Status of the payment request resource NULL value means that the status is not yet available from the bank |
|
statusReasonInformation |
false |
ISO20022: Provides detailed information on the status reason. Can only be used in status equal to "RJCT". |
|
_links |
false |
links that can be used for further navigation when having post a Payment Request in order |
|
authData |
false |
[structure used for returning auth parameters to be passed to user] |
HalPaymentRequestCancellation
data forwarded by the ASPSP to the PISP after creation of the Payment Request resource creation
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
_links |
false |
Links that can be used for further navigation, especially in REDIRECT approach |
|
authData |
false |
[structure used for returning auth parameters to be passed to user] |
HalPaymentRequestCreation
data forwarded by the ASPSP to the PISP after creation of the Payment Request resource creation
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
_links |
false |
Links that can be used for further navigation, especially in REDIRECT approach |
|
authData |
false |
[structure used for returning auth parameters to be passed to user] |
HalPaymentRequestTransaction
HYPERMEDIA structure used for returning the original Payment Request Transaction to the PISP
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
paymentRequestTransaction |
false |
Payment instruction to transfer instructed amount of money to the specified beneficiary. |
HalPaymentReuqestCancellationResult
HYPERMEDIA structure used for returning the original Payment Request to the PISP
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
paymentInformationStatus |
false |
Status of the payment request resource NULL value means that the status is not yet available from the bank |
|
_links |
false |
links that can be used for further navigation when having post a Payment Request in order |
|
authData |
false |
[structure used for returning auth parameters to be passed to user] |
HalTransactions
HYPERMEDIA structure used for returning the list of the transactions for a given account to the AISP
Fields
Name | Type | Required | Description |
---|---|---|---|
transactions |
true |
List of transactions |
|
continuationKey |
string |
false |
Value to be passed to getAccountTransactions to retrieve next page of transactions. Null if there are no more pages. Only valid in current session. |
LocalInstrumentCode
ISO20022: User community specific instrument. Usage: This element is used to specify a local instrument, local clearing option and/or further qualify the service or service level.
Base type
string
Logo
ASPSP logo, which can be used for showing to an end-user
Fields
Name | Type | Required | Description |
---|---|---|---|
url |
string |
true |
Logo URL |
ModifyConsentsInfo
Information about usage of modifyConsents
method
Fields
Name | Type | Required | Description |
---|---|---|---|
beforeAccounts |
boolean |
true |
Shows if |
extendsDetails |
boolean |
true |
Shows if bank returns extended information about accounts from method |
accountsRequired |
boolean |
true |
Shows if |
PartyIdentification
API : Description of a Party which can be either a person or an organization.
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
false |
ISO20022: Name by which a party is known and which is usually used to identify that party. |
postalAddress |
false |
ISO20022 : Information that locates and identifies a specific address, as defined by postal services. |
|
organisationId |
false |
Unique and unambiguous way to identify an organisation. |
|
privateId |
false |
Unique and unambiguous identification of a person. |
|
contactDetails |
false |
Contact details of a person. |
PaymentCoverageRequestResource
Payment coverage request structure. The request must rely either on a cash account or a payment card.
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentCoverageRequestId |
string |
true |
Identification of the payment Coverage Request |
payee |
string |
false |
The merchant where the card is accepted as information to the PSU. |
instructedAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
accountId |
true |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
PaymentIdentification
ISO20022: Set of elements used to reference a payment instruction.
Fields
Name | Type | Required | Description |
---|---|---|---|
instructionId |
string |
false |
ISO20022: Unique identification as assigned by an instructing party for an instructed party to unambiguously identify the instruction. API: Unique identification shared between the PISP and the ASPSP |
endToEndId |
string |
false |
ISO20022: Unique identification assigned by the initiating party to unambiguously identify the transaction. This identification is passed on, unchanged, throughout the entire end-to-end chain. API: Unique identification shared between the merchant and the PSU |
PaymentInformationId
ISO20022 : Reference assigned by a sending party to unambiguously identify the payment information block within the message.
Base type
string
PaymentInformationStatusCode
ISO20022: Specifies the status of the payment information.
Base type
string
Enumerated Values
Value | Description |
---|---|
ACCC |
AcceptedCreditSettlementCompleted. Settlement on the creditor's account has been completed. |
ACCP |
AcceptedCustomerProfile. Preceding check of technical validation was successful. Customer profile check was also successful. |
ACSC |
AcceptedSettlementCompleted. Settlement on the debtor's account has been completed. |
ACSP |
AcceptedSettlementInProcess. All preceding checks such as technical validation and customer profile were successful. Dynamic risk assessment is now also successful and therefore the Payment Request has been accepted for execution. |
ACTC |
AcceptedTechnicalValidation. Authentication and syntactical and semantical validation are successful. |
ACWC |
AcceptedWithChange. Instruction is accepted but a change will be made, such as date or remittance not sent. |
ACWP |
AcceptedWithoutPosting. Payment instruction included in the credit transfer is accepted without being posted to the creditor's account. |
PART |
PartiallyAccepted. A number of transactions have been accepted, whereas another number of transactions have not yet achieved 'accepted' status. |
RCVD |
Received. Payment initiation has been received by the receiving agent. |
PDNG |
Pending. Payment request or individual transaction included in the Payment Request is pending. Further checks and status update will be performed. |
RJCT |
Rejected. Payment request has been rejected. |
ACPT |
Accepted. Request is accepted. |
ACCR |
AcceptedCancellationRequest. Cancellation is accepted. |
RJCR |
RejectedCancellationRequest. Cancellation request is rejected. |
PACR |
PartiallyAcceptedCancellationRequest. Cancellation is partially accepted. |
PDCR |
PendingCancellationRequest. Cancellation request is pending. |
CNCL |
PaymentCancelled. Payment is cancelled. |
null |
NoCancellationProcess. There is no cancellation process ongoing. |
PaymentRequestConfirmation
Contains data for confirmation of payment request
Fields
Name | Type | Required | Description |
---|---|---|---|
psuAuthenticationFactor |
string |
false |
Authentication factor forwarded by the TPP to the ASPSP in order to fulfil the strong customer authentication process |
PaymentRequestResource
ISO20022: The PaymentRequestResource message is sent by the Creditor sending party to the Debtor receiving party, directly or through agents. It is used by a Creditor to request movement of funds from the debtor account to a creditor. API: Information about the creditor (Id, account and agent) might be placed either at payment level or at instruction level. Thus multi-beneficiary payments can be handled. The requested execution date can be placed either at payment level when all instructions are requested to be executed at the same date or at instruction level. The latest case includes:
- multiple instructions having different requested execution dates
- standing orders settings
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentInformationId |
false |
ISO20022 : Reference assigned by a sending party to unambiguously identify the payment information block within the message. |
|
paymentTypeInformation |
false |
ISO20022: Set of elements used to further specify the type of transaction. |
|
debtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
debtorAccount |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
debtorAgent |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
debtorCurrency |
string |
false |
Debtor account currency code |
purpose |
false |
ISO20022: Underlying reason for the payment transaction, as published in an external purpose code list. |
|
chargeBearer |
false |
ISO20022: Specifies which party/parties will bear the charges associated with the processing of the payment transaction. |
|
creditTransferTransaction |
true |
Payment instructions to be executed towards one or multiple beneficiaries in the payment process. Maximum number of transactions depend on the ASPSP and type of the payment taking into accounts its specificities about payment request handling. |
PaymentRequestResourceDetails
ISO20022: The PaymentRequestResourceDetails message is sent by the Creditor sending party to the Debtor receiving party, directly or through agents. It is used by a Creditor to request movement of funds from the debtor account to a creditor. API: Information about the creditor (Id, account and agent) might be placed either at payment level or at instruction level. Thus multi-beneficiary payments can be handled. The requested execution date can be placed either at payment level when all instructions are requested to be executed at the same date or at instruction level. The latest case includes:
- multiple instructions having different requested execution dates
- standing orders settings
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentInformationId |
false |
ISO20022 : Reference assigned by a sending party to unambiguously identify the payment information block within the message. |
|
paymentTypeInformation |
false |
ISO20022: Set of elements used to further specify the type of transaction. |
|
debtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
debtorAccount |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
debtorAgent |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
debtorCurrency |
string |
false |
Debtor account currency code |
purpose |
false |
ISO20022: Underlying reason for the payment transaction, as published in an external purpose code list. |
|
chargeBearer |
false |
ISO20022: Specifies which party/parties will bear the charges associated with the processing of the payment transaction. |
|
creditTransferTransaction |
true |
Payment instructions to be executed towards one or multiple beneficiaries in the payment process. Maximum number of transactions depend on the ASPSP and type of the payment taking into accounts its specificities about payment request handling. |
PaymentTransactionResourceId
ISO20022 : Reference assigned by a sending party to unambiguously identify the transaction information block within the message.
Base type
string
PaymentType
Payment type info
Base type
string
Enumerated Values
Value | Description |
---|---|
SEPA |
SEPA credit transfers |
INST_SEPA |
Instant SEPA credit transfers |
BULK_SEPA |
SEPA bulk credit transfers |
CROSSBORDER |
Crossborder credit transfers |
DOMESTIC |
Domestic credit transfers |
DOMESTIC_SE_GIRO |
Swedish domestic Giro payments (BankGiro/PlusGiro) |
INTERNAL |
Transfer made within the same aspsp |
PaymentTypeInfo
Information about available payment types
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentType |
true |
Payment type info |
|
maxTransactions |
integer |
false |
Maximum number of transactions |
currencies |
false |
List of supported currencies |
|
debtorNameRequired |
boolean |
false |
States if debtor name is required |
debtorAccountRequired |
boolean |
false |
States if debtor account is required during payment initiation request. |
debtorAccountSchemas |
false |
List of supported debtor account schemas |
|
debtorCountryRequired |
boolean |
false |
States if debtor country is required |
creditorAccountSchemas |
false |
List of supported creditor account schemas |
|
priorityCodes |
false |
List of supported priority codes |
|
chargeBearerValues |
false |
List of supported charge bearer codes |
|
creditorCountryRequired |
boolean |
false |
States if creditor country is required |
creditorNameRequired |
boolean |
false |
States if creditor name is required |
creditorPostalAddressRequired |
boolean |
false |
States if creditor postal address (addressLine and townName) is required |
remittanceInformationRequired |
boolean |
false |
States if remittance information is required |
remittanceInformationLines |
false |
Properties of remittance information. Each item of the array correspond to the remittance information line with the same index. When provided, the number of lines in the remittance information should be the same as the length of this array. |
|
debtorCurrencyRequired |
boolean |
false |
States if debtor currency is required |
debtorContactEmailRequired |
boolean |
false |
States if debtor's contact email is required when a payment this type is being initiated |
debtorContactPhoneRequired |
boolean |
false |
States if debtor's contact phone is required when a payment this type is being initiated |
creditorAgentBicFiRequired |
boolean |
false |
States if creditor agent bicFi is required |
creditorAgentClearingSystemMemberIdRequired |
boolean |
false |
States if creditor agent clearing system member ID is required |
allowedAuthMethods |
[string] |
false |
List of supported auth methods for this payment type |
regulatoryReportingCodes |
false |
List of supported codes for regulatory reporting details |
|
regulatoryReportingCodeRequired |
boolean |
false |
States if regulatory reporting shall be provided for credit transfer transactions. |
referenceNumberSupported |
boolean |
false |
States if reference number can be provided for credit transfer transactions. |
referenceNumberSchemas |
false |
List of reference number schemas supported by a payment method. |
|
requestedExecutionDateSupported |
boolean |
false |
States if requested execution date supported by a payment method. |
requestedExecutionDateMaxPeriod |
integer |
false |
Maximum requested execution date interval in the future. |
PaymentTypeInformation
ISO20022: Set of elements used to further specify the type of transaction.
Fields
Name | Type | Required | Description |
---|---|---|---|
instructionPriority |
false |
ISO20022: Indicator of the urgency or order of importance that the instructing party would like the instructed party to apply to the processing of the instruction. |
|
serviceLevel |
false |
ISO20022: Agreement under which or rules under which the transaction should be processed. Specifies a pre-agreed service or level of service between the parties, as published in an external service level code list. |
|
categoryPurpose |
false |
ISO20022: Specifies the high level purpose of the instruction based on a set of pre-defined categories. This is used by the initiating party to provide information concerning the processing of the payment. It is likely to trigger special processing by any of the agents involved in the payment chain. |
|
localInstrument |
false |
ISO20022: User community specific instrument. |
PostalAddress
ISO20022 : Information that locates and identifies a specific address, as defined by postal services.
Fields
Name | Type | Required | Description |
---|---|---|---|
addressType |
false |
ISO20022: Identifies the nature of the postal address. |
|
department |
string |
false |
Identification of a division of a large organisation or building. |
subDepartment |
string |
false |
Identification of a sub-division of a large organisation or building. |
streetName |
string |
false |
Name of a street or thoroughfare. |
buildingNumber |
string |
false |
Number that identifies the position of a building on a street. |
postCode |
string |
false |
Identifier consisting of a group of letters and/or numbers that is added to a postal address to assist the sorting of mail. |
townName |
string |
false |
Name of a built-up area, with defined boundaries, and a local government. |
countrySubDivision |
string |
false |
Identifies a subdivision of a country such as state, region, county. |
country |
string |
false |
ISO20022: Country in which a person resides (the place of a person's home). In the case of a company, it is the country from which the affairs of that company are directed. |
addressLine |
[string] |
false |
Unstructured address. The two lines must embed zip code and town name |
PriorityCode
ISO20022: Indicator of the urgency or order of importance that the instructing party would like the instructed party to apply to the processing of the instruction.
Base type
string
Enumerated Values
Value | Description |
---|---|
HIGH |
High priority |
NORM |
Normal priority |
EXPR |
Express priority. Polish-specific priority code |
PurposeCode
ISO20022: Underlying reason for the payment transaction, as published in an external purpose code list.
Base type
string
Enumerated Values
Value | Description |
---|---|
ACCT |
Funds moved between 2 accounts of same account holder at the same bank. |
CASH |
general cash management instruction, may be used for Transfer Initiation. |
COMC |
Transaction is related to a payment of commercial credit or debit. |
CPKC |
General Carpark Charges Transaction is related to carpark charges. |
TRPT |
Transport RoadPricing Transaction is for the payment to top-up pre-paid card and electronic road pricing for the purpose of transportation. |
ReferenceNumber
ISO20022: This field specifies the reference assigned by the sender to unambiguously identify the message.
Base type
string
ReferenceNumberScheme
Name of the reference number scheme.
Base type
string
Enumerated Values
Value | Description |
---|---|
SEBG |
Swedish Bankgiro OCR. |
NORF |
Norwegian KID (OCR). |
FIRF |
Finnish reference number. |
INTL |
International reference number (starting with RF). |
RegulatoryAuthority
Entity requiring the regulatory reporting information.
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country in which the regulatory authority operates. |
name |
string |
false |
Name of the regulatory authority. |
RegulatoryReporting
Information needed due to regulatory and statutory requirements.
Fields
Name | Type | Required | Description |
---|---|---|---|
authority |
false |
Regulatory authority to which reporting shall be made. |
|
details |
true |
Details to provide on the regulatory reporting information. |
RegulatoryReportingCode
Regulatory reporting code made available by a certain regulatory authority.
Fields
Name | Type | Required | Description |
---|---|---|---|
value |
string |
true |
Value of the code, i.e. what needs to be passed as a code when filling in regulatory reporting details. |
description |
string |
false |
Regulatory authority to which reporting shall be made. |
RegulatoryReportingDetails
Set of elements used to provide details on the regulatory reporting information.
Fields
Name | Type | Required | Description |
---|---|---|---|
amount |
false |
Amount of money to be reported. If not provided the total instructed amount of the transaction is assumed. |
|
code |
string |
false |
A code specifying the nature, purpose, and/or reason for the transaction. Codes to be used depend on the regulatory authority, to which they are being reported. |
information |
string |
false |
Additional details that cater for specific domestic regulatory requirements. |
RemittanceInformationLineInfo
Information about expected properties of the remittance information line
Fields
Name | Type | Required | Description |
---|---|---|---|
minLength |
integer |
false |
Minimum possible length of the remittance information line |
maxLength |
integer |
false |
Maximum possible length of the remittance information line |
pattern |
string |
false |
Perl compatible regular expression used for check of the remittance information line format |
RequestedExecutionDate
ISO20022: Date at which the initiating party requests the clearing agent to process the payment. API: This date can be used in the following cases:
- the single requested execution date for a payment having several instructions. In this case, this field must be set at the payment level.
- the requested execution date for a given instruction within a payment. In this case, this field must be set at each instruction level.
- The first date of execution for a standing order. When the payment cannot be processed at this date, the ASPSP is allowed to shift the applied execution date to the next possible execution date for non-standing orders. For standing orders, the [executionRule] parameter helps to compute the execution date to be applied.
Base type
string(date-time)
ResourceId
Identifier assigned by the ASPSP for further use of the created resource through API calls
Base type
string
SchemeName
Name of the identification scheme. Partially based on ISO20022 external code list
Base type
string
Enumerated Values
Value | Description |
---|---|
CHID |
Clearing Identification Number |
GS1G |
GS1GLNIdentifier |
DUNS |
Data Universal Numbering System |
BANK |
BankPartyIdentification. Unique and unambiguous assignment made by a specific bank or similar financial institution to identify a relationship as defined between the bank and its client. |
TXID |
TaxIdentificationNumber |
CUST |
CorporateCustomerNumber |
EMPL |
EmployerIdentificationNumber |
OTHC |
OtherCorporate. |
DRLC |
DriversLicenseNumber |
SOSE |
SocialSecurityNumber |
ARNU |
AlienRegistrationNumber |
CCPT |
PassportNumber |
OTHI |
OtherIndividual |
COID |
CountryIdentificationCode. Country authority given organisation identification (e.g., corporate registration number) |
SREN |
The SIREN number is a 9 digit code assigned by INSEE, the French National Institute for Statistics and Economic Studies, to identify an organisation in France. |
SRET |
The SIRET number is a 14 digit code assigned by INSEE, the French National Institute for Statistics and Economic Studies, to identify an organisation unit in France. It consists of the SIREN number, followed by a five digit classification number, to identify the local geographical unit of that entity. |
NIDN |
NationalIdentityNumber. Number assigned by an authority to identify the national identity number of a person. |
OAUT |
OAUTH2 access token that is owned by the PISP being also an AISP and that can be used in order to identify the PSU |
CPAN |
Card PAN (masked or plain) |
BBAN |
Basic Bank Account Number. Represents a country-specific bank account number. |
IBAN |
International Bank Account Number (IBAN) - identification used internationally by financial institutions to uniquely identify the account of a customer. |
MIBN |
Masked IBAN |
BGNR |
Swedish BankGiro account number. Used in domestic swedish giro payments |
PGNR |
Swedish PlusGiro account number. Used in domestic swedish giro payments |
ServiceLevelCode
ISO20022: Agreement under which or rules under which the transaction should be processed. Specifies a pre-agreed service or level of service between the parties, as published in an external service level code list.
Base type
string
Enumerated Values
Value | Description |
---|---|
BKTR |
Book Transaction – Payment through internal book transfer. |
G001 |
TrackedCustomerCreditTransfer – Tracked Customer Credit Transfer |
G002 |
TrackedStopAndRecall – Tracked Stop and Recall |
G003 |
TrackedCorporateTransfer – Tracked Corporate Transfer |
G004 |
TrackedFinancialInstitutionTransfer – Tracked Financial Institution Transfer |
NUGP |
Non-urgent Priority Payment – Payment must be executed as a non-urgent transaction with priority settlement. |
NURG |
Non-urgent Payment – Payment must be executed as a non-urgent transaction, which is typically identified as an ACH or low value transaction. |
PRPT |
EBAPriorityService – Transaction must be processed according to the EBA Priority Service. |
SDVA |
SameDayValue – Payment must be executed with same day value to the creditor. |
SEPA |
SingleEuroPaymentsArea – Payment must be executed following the Single Euro Payments Area scheme. |
SVDE |
Domestic Cheque Clearing and Settlement – Payment execution following the cheque agreement and traveller cheque agreement of the German Banking Industry Committee (Die Deutsche Kreditwirtschaft - DK) and Deutsche Bundesbank – Scheck Verrechnung Deutschland |
URGP |
Urgent Payment – Payment must be executed as an urgent transaction cleared through a real-time gross settlement system, which is typically identified as a wire or high value transaction. |
URNS |
Urgent Payment Net Settlement – Payment must be executed as an urgent transaction cleared through a real-time net settlement system, which is typically identified as a wire or high value transaction. |
StatusReasonInformation
ISO20022: Provides detailed information on the status reason. Can only be used in status equal to "RJCT".
Base type
string
Enumerated Values
Value | Description |
---|---|
AC01 |
IncorectAccountNumber. the account number is either invalid or does not exist |
AC04 |
ClosedAccountNumber. the account is closed and cannot be used |
AC06 |
BlockedAccount. the account is blocked and cannot be used |
AG01 |
Transaction forbidden. Transaction forbidden on this type of account |
CH03 |
RequestedExecutionDateOrRequestedCollectionDateTooFarInFuture. The requested execution date is too far in the future |
CUST |
RequestedByCustomer. The reject is due to the debtor (refusal or lack of liquidity) |
DS02 |
OrderCancelled. An authorized user has cancelled the order |
FF01 |
InvalidFileFormat. The reject is due to the original Payment Request which is invalid (syntax, structure or values) |
FRAD |
FraudulentOriginated. the Payment Request is considered as fraudulent |
MS03 |
NotSpecifiedReasonAgentGenerated. No reason specified by the ASPSP |
NOAS |
NoAnswerFromCustomer. The PSU has neither accepted nor rejected the Payment Request and a time-out has occurred |
RR01 |
MissingDebtorAccountOrIdentification. The Debtor account and/or Identification are missing or inconsistent |
RR03 |
MissingCreditorNameOrAddress. Specification of the creditor’s name and/or address needed for regulatory requirements is insufficient or missing. |
RR04 |
RegulatoryReason. Reject from regulatory reason |
RR12 |
InvalidPartyID. Invalid or missing identification required within a particular country or payment type. |
Token
Fields
Name | Type | Required | Description |
---|---|---|---|
access_token |
string |
false |
The access token value |
expires_in |
integer(int32) |
false |
The lifetime in seconds of the access token |
refresh_token |
string |
false |
The refresh token value |
scope |
string |
false |
Scopes of the token |
Transaction
structure of a transaction
Fields
Name | Type | Required | Description |
---|---|---|---|
resourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
entryReference |
string |
false |
Unique transaction identifier provided by ASPSP. This identifier is both unique and immutable and can be used for matching transactions across multiple PSU authentication sessions. Usually the same identifier is available for transactions in ASPSP's online/mobile interface and is called archive ID or similarly. |
transactionAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
creditor |
false |
API : Description of a Party which can be either a person or an organization. |
|
creditorAccount |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
creditorAgent |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
debtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
debtorAccount |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
debtorAgent |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
bankTransactionCode |
false |
ISO20022: Allows the account servicer to correctly report a transaction, which in its turn will help account owners to perform their cash management and reconciliation operations. |
|
creditDebitIndicator |
string |
true |
Accounting flow of the transaction |
status |
true |
Type of Transaction |
|
bookingDate |
string(date) |
false |
Booking date of the transaction on the account |
valueDate |
string(date) |
false |
Value date of the transaction on the account |
transactionDate |
string(date) |
false |
Date used for specific purposes:
|
balanceAfterTransaction |
false |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
referenceNumber |
string |
false |
Credit transfer reference number (also known as the creditor reference or the structured creditor reference). The value is set when it is known that the transaction data contains a reference number (in either ISO 11649 or a local format). |
remittanceInformation |
false |
Payment details. For credit transfers may contain free text, reference number or both at the same time (in case Extended Remittance Information is supported). When it is known that remittance information contains a reference number (either based on ISO 11649 or a local scheme), the reference number is also available via the |
|
merchantCategoryCode |
string |
false |
Category code conform to ISO 18245, related to the type of services or goods the merchant provides for the transaction |
exchangeRate |
false |
Provides details on the currency exchange rate and contract. |
|
debtorAccountAdditionalIdentification |
false |
All other debtor account identifiers provided by ASPSPs |
|
creditorAccountAdditionalIdentification |
false |
All other creditor account identifiers provided by ASPSPs |
|
note |
string |
false |
The internal note made by PSU |
Enumerated Values
creditDebitIndicator
Value | Description |
---|---|
CRDT |
Credit type transaction |
DBIT |
Debit type transaction |
TransactionIndividualStatusCode
ISO20022: Specifies the status of the payment information group.
Base type
string
Enumerated Values
Value | Description |
---|---|
RCVD |
Payment request or individual transaction included in the Payment Request has been received by the receiving agent. |
RJCT |
Payment request or individual transaction included in the Payment Request has been rejected. |
PDNG |
Pending. Payment request or individual transaction included in the Payment Request is pending. Further checks and status update will be performed. |
ACSP |
All preceding checks such as technical validation and customer profile were successful and therefore the Payment Request has been accepted for execution. |
ACSC |
Settlement on the debtor's account has been completed. |
TransactionStatus
Type of Transaction
Base type
string
Enumerated Values
Value | Description |
---|---|
BOOK |
(ISO20022 ClosingBooked) Accounted transaction |
CNCL |
Cancelled transaction |
HOLD |
Account hold |
OTHR |
Transaction with unknown status or not fitting the other options |
PDNG |
(ISO20022 Expected) Instant Balance Transaction |
RJCT |
Rejected transaction |
SCHD |
Scheduled transaction |
TransactionsInfo
Meta information about usage and limitations of getAccountTransactions
method
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code. Country to which transactions information applies. |
psuType |
string |
true |
PSU Type (personal/business). PSU type to which transactions information applies. |
scaRequired |
boolean |
false |
Flag showing whether dedicated SCA Required to fetch transactions |
oneOff |
boolean |
false |
Flag showing whether limitations is applicable to a one-off fetching |
description |
string |
false |
Any additional information related to transactions fetching |
dateLimit |
string(date) |
false |
If set then transactions are available up to a specified date |
daysPastLimit |
integer |
false |
Limit of days in the past for which transactions are available |
daysSingleRequestLimit |
integer |
false |
Limit of days in a single request for which transactions are available (interval between dateTo and dateFrom) |
entriesLimit |
integer |
false |
Limit of transaction entries which can be looked in the past |
entriesSingleRequestLimit |
integer |
false |
Limit of transaction entries which can be returned in a single request |
UnstructuredRemittanceInformation
Payment details. For credit transfers may contain free text, reference number or both at the same time (in case Extended Remittance Information is supported). When it is known that remittance information contains a reference number (either based on ISO 11649 or a local scheme), the reference number is also available via the referenceNumber
field of the Transaction
data structure.
Fields
Name | Type | Required | Description |
---|---|---|---|
remittanceLine |
string |
false |
Relevant information to the transaction |
HAL
ConsentLinks
Fields
Name | Type | Required | Description |
---|---|---|---|
redirect |
false |
hypertext reference |
GenericLink
hypertext reference
Fields
Name | Type | Required | Description |
---|---|---|---|
href |
string |
true |
URI to be used |
templated |
boolean |
false |
specifies "true" if href is a URI template, i.e. with parameters. Otherwise, this property is absent or set to false |
PaymentRequestLinks
links that can be used for further navigation when having post a Payment Request in order to get the relevant status report.
Fields
Name | Type | Required | Description |
---|---|---|---|
consentApproval |
false |
URL to be used by the PISP in order to continue the ASPSP authentication and consent management process |
PaymentRequestResourceCreationLinks
Links that can be used for further navigation, especially in REDIRECT approach
Fields
Name | Type | Required | Description |
---|---|---|---|
consentApproval |
false |
URL to be used by the PISP in order to start the ASPSP authentication and consent management process |
Exceptions
AccountNotAccessibleException
This exception is raised when PSU does not have access to the requested account or it doesn't exist.
Base type
AuthorizationCancelledException
This exception is raised when authorization was cancelled either by ASPSP or by user.
Base type
CoreException
Base Exception class in SDK, raised for unexpected exceptions.
Fields
Name | Type | Required | Description |
---|---|---|---|
code |
integer(int32) |
true |
Error code |
message |
string |
true |
Error description |
DataRetrievalException
This exception is raised when unable to retrieve data requested from ASPSP API.
Base type
ExpiredConsentException
This exception is raised when ASPSP API response shows that used consent is no longer valid and
cannot be refreshed or restored.
This also raised when both access and refresh (if supported) tokens are expired.
Base type
HttpException
Code samples
import com.enablebanking.ApiClient;
import com.enablebanking.api.AuthApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AuthApi authApi = new AuthApi(new ApiClient(connectorSettings));
try {
Auth auth = authApi.getAuth("state", null, null, null); // Let's say Unexpected HTTP exception occured
} catch (HttpException e) {
List> headers = httpException.getResponseHeaders();
String body = httpException.getResponseBody();
int code = httpException.getCode();
}
This exception is raised when ASPSP API responses with unspecified error. Base exception for HTTP related errors
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
responseHeaders |
[object] |
true |
Headers of http response |
responseBody |
string |
true |
Body of http response |
InsufficientScopeException
This exception is raised when consent given by the PSU does not allow to retrieve
requested data.
Base type
InvalidCredentialsException
This exception is raised when ASPSP API response shows that provided credentials are invalid.
Base type
InvalidResponseIdException
This exception is raised when ASPSP API response id does not match request id in.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
requestId |
string |
true |
Request id |
InvalidResponseSignatureException
This exception is raised when ASPSP API response signature verification failed.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
jws |
string |
true |
JSON web signature |
MakeTokenException
This exception is raised when ASPSP API is not ready yet. Flag retry
shows if it is required to retry method call.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
env |
string |
true |
Environment |
retry |
string |
true |
Flag showing whether it is possible to retry the request |
url |
string |
false |
URL to retry |
authData |
false |
[structure used for returning auth parameters to be passed to user] |
ModelValidationException
Code samples
import com.enablebanking.ApiClient;
import com.enablebanking.api.PispApi;
import com.enablebanking.model.*;
PispApi pispApi = new PispApi(apiClient);
PaymentRequestResource prr = new PaymentRequestResource()
.creditTransferTransaction(Arrays.asList(
new CreditTransferTransaction()
.instructedAmount(new AmountType()
.currency("EUR")
.amount(new BigDecimal("1.25")))
.frequency(FrequencyCode.DAIL)
.beneficiary(
new Beneficiary()
.creditor(
new PartyIdentification()
.name("Creditor name")
.postalAddress(
new PostalAddress()
.addressLine(Arrays.asList(
"Creditor Name ",
"Creditor Address 1",
"Creditor Address 2"))
.country("RO")))
.creditorAccount(new AccountIdentification()
.iban("Some iban")))
.paymentTypeInformation(new PaymentTypeInformation()
.instructionPriority(PriorityCode.NORM)
.serviceLevel(ServiceLevelCode.SEPA)
.categoryPurpose(CategoryPurposeCode.CASH))
.debtor(new PartyIdentification()
.name("Debtor name")
.postalAddress(new PostalAddress()
.addressLine(Arrays.asList(
"Debtor Name",
"Debtor Address 1",
"Debtor Address 2"))
.country("PL")))
.debtorAccount(new AccountIdentification().iban("Some Iban")); // Remittance information required but not set
try {
PaymentRequestResource prr = pispApi.makePaymentRequest(prr);
} catch (ModelValidationException e) {
String key = e.getKey(); // "remittanceInformation"
String path = e.getPath() // "paymentRequestResource.creditTransferTransaction.remittanceInformation"
}
This exception is raised when model validation failed.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
key |
string |
true |
Reference to a field |
path |
string |
true |
Path to a field |
NotImplementedException
This exception is raised when called method is not implemented in SDK(either not supported by ASPSP API or integration is not ready yet).
Base type
ParameterValidationException
Code samples
import com.enablebanking.ApiClient;
import com.enablebanking.api.AuthApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AuthApi authApi = new AuthApi(new ApiClient(connectorSettings));
try {
Auth auth = authApi.getAuth("state", null, null, null); // userId (2nd parameter) is required but not set
} catch (ParameterValidationError e) {
String key = e.getKey(); // "userId"
}
This exception is raised when function parameter validation failed. Key attribute is reference to parameter.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
key |
string |
true |
Reference to a parameter |
PsuActionRequiredException
This exception is raised when ASPSP API response shows that there are additional actions
required from a PSU.
For example, a PSU need to contact their bank customer service to enable necessary
permissions or they need to sign necessary agreements in their online bank.
Base type
RateLimitException
This exception is raised when maximum daily access exceeded.
Base type
ResourceNotFoundException
This exception is raised when unable to load certificate/private key from path.
Base type
ResponseValidationException
This exception is raised when ASPSP API response validation failed.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
responseHeaders |
[object] |
true |
Headers of http response |
responseBody |
string |
true |
Body of http response |
errorDescription |
string |
false |
User-friendly error message |
TransactionPeriodException
This exception is raised when wrong or invalid account transactions period is requested.
Base type
TransactionsFetchingException
This exception is raised when ASPSP API responses with error related to transactions fetching
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
retry |
string |
true |
Flag showing whether it is possible to retry the request |
continuationKey |
string |
false |
Continuation key to provide in the following requests |
scaRedirect |
string |
false |
If additional SCA is required, then this field contains link |
UnauthorizedException
This exception is raised when unauthorized to retrieve data requested from ASPSP API (e.g. access token expired).
Base type
ValidationException
Base class for validation related exceptions.
Base type
Connectors
ABNAMROBusinessConnectorSettings
Settings for initializing ApiClient for ABN AMRO
Credentials can obtained at ABN AMRO Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. Possible values: |
clientId |
string |
true |
API client ID (obtained from ABN AMRO Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
apiKey |
string |
true |
API key |
ABNAMROConnectorSettings
Settings for initializing ApiClient for ABN AMRO
Credentials can obtained at ABN AMRO Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. Possilbe values: |
brand |
string |
true |
Denotes the bank where the account is held. If omitted, ABN AMRO account in NL or commercial ABN AMRO account in BE, GB, or DE will be used. |
clientId |
string |
true |
API client ID (obtained from ABN AMRO Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
apiKey |
string |
true |
API key |
AIBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AIBConnectorSettings;
ApiClient apiClient = new ApiClient(
new AIBConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.business(false)
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AIBConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
client_id="your-client-id",
client_secret="your-client-secret",
business="false"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
clientId: "your-client-id",
clientSecret: "your-client-secret",
business: "false",
}
const apiClient = new enablebanking.ApiClient('AIB', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AIB
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
ASNBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ASNBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new ASNBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ASNBankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('ASNBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for ASN Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
AXABanqueConnectorSettings
Settings for initializing ApiClient for AXA Banque (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
AXAConnectorSettings
Settings for initializing ApiClient for AXA Bank Connector.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
NCA id obtained from QWAC certificate. Use "VALID_CLIENT_ID" value for sandbox |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
AasenSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AasenSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new AasenSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Aasen Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
AbancaBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AbancaBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new AbancaBusinessConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.apiKey("your-api-key")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AbancaBusinessConnectorSettings(
client_id="your-client-id",
api_key="your-api-key",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
apiKey: "your-api-key",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('AbancaBusiness', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AbancaBusiness
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
apiKey |
string |
true |
API client key. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
AbancaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AbancaConnectorSettings;
ApiClient apiClient = new ApiClient(
new AbancaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.apiKey("your-apiKey")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("qseal-cert-public-serial-here")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AbancaConnectorSettings(
client_id="your-client-id",
apiKey="your-apiKey",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
apiKey: "your-apiKey",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "qseal-cert-public-serial-here"
}
const apiClient = new enablebanking.ApiClient('Abanca', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Abanca
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
apiKey |
string |
true |
none |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
AbancaPTConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AbancaPTConnectorSettings;
ApiClient apiClient = new ApiClient(
new AbancaPTConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.apiKey("your-apiKey")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AbancaPTConnectorSettings(
client_id="your-client-id",
apiKey="your-apiKey",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
apiKey: "your-apiKey",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('AbancaPT', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AbancaPT
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
apiKey |
string |
true |
none |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ActivoBankConnectorSettings
Settings for initializing ApiClient for BANCO ACTIVOBANK, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
ActivoBankESConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ActivoBankESConnectorSettings;
ApiClient apiClient = new ApiClient(
new ActivoBankESConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.signKeyPath("your-sign-key-path")
.signCertPath("your-sign-cert-path")
.certPath("your-cert-path")
.signPubKeySerial("your-sign-pub-key-serial")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ActivoBankESConnectorSettings(
client_id="your-client-id",
sign_key_path="your-sign-key-path",
sign_cert_path="your-sign-cert-path",
cert_path="your-cert-path",
sign_pub_key_serial="your-sign-pub-key-serial",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
signKeyPath: "your-sign-key-path",
signCertPath: "your-sign-cert-path",
certPath: "your-cert-path",
signPubKeySerial: "your-sign-pub-key-serial",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('ActivoBankES', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for ActivoBankES
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
AddikoBankHRConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AddikoBankHRConnectorSettings;
ApiClient apiClient = new ApiClient(
new AddikoBankHRConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AddikoBankHRConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('AddikoBankHR', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AddikoBankHR
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
AddikoConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AddikoConnectorSettings;
ApiClient apiClient = new ApiClient(
new AddikoConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AddikoConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Addiko', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Addiko
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
AionBank2ConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AionBank2ConnectorSettings;
ApiClient apiClient = new ApiClient(
new AionBank2ConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.country("your-country")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AionBank2ConnectorSettings(
client_id="your-client-id",
country="your-country",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
country: "your-country",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('AionBank2', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AionBank2
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
country |
string |
true |
Bank`s country, two-letter country code. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
AktiaConnectorSettings
Settings for initializing ApiClient for Aktia (Finland).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Aktia Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Aktia Developer Portal) |
sandboxPaymentId |
string |
false |
ID of the payment request used in sandbox (sandbox only) |
sandboxSafeguard |
boolean |
false |
Flag whether to fix malformed sandbox data or not (sandbox only) |
AktiaContingencyConnectorSettings
Settings for initializing ApiClient for Aktia Contingency API (Finland).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
authorizationHeader |
string |
true |
Authorization header obtained from Aktia onboarding(without "Basic") |
AlandsbankenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AlandsbankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new AlandsbankenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.xApiKey("put-your-x-api-key-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.sandbox(true));
Settings for initializing ApiClient for Ålandsbanken (Finland).
Credentials can obtained at Open Banking Market operated by Crosskey Banking Solutions.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
true |
Language of authentication user interface. |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
xApiKey |
string |
true |
API client key (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. Test certificate (for accessing sandbox environment) can be downloaded from Open Banking Market. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be presented as a decimal string. The following command can be use for extracting it from QSeal certificate in PEM format: |
AliorConnectorSettings
Settings for initializing ApiClient for Alior Bank (Poland).
Credentials can obtained at Alior Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Alior Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Alior Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded with padding) |
signCertUrl |
string |
true |
Public URL where QSeal certificate can be found |
tppId |
string |
true |
ID assigned to the TPP by an authority (format: PSDXX-YYYY-ZZZZZZZZ) |
AlisaBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AlisaBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new AlisaBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.signPubKeySerial("your-sign-pub-key-serial")
.apiKey("your-api-key")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AlisaBankConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
sign_pub_key_serial="your-sign-pub-key-serial",
api_key="your-api-key"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
signPubKeySerial: "your-sign-pub-key-serial",
apiKey: "your-api-key"
}
const apiClient = new enablebanking.ApiClient('AlisaBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AlisaBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
apiKey |
string |
true |
API client key. |
AllianzBankFinancialAdvisorsConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AllianzBankFinancialAdvisorsConnectorSettings;
ApiClient apiClient = new ApiClient(
new AllianzBankFinancialAdvisorsConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.business(false)
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AllianzBankFinancialAdvisorsConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
business=False
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
business: false
}
const apiClient = new enablebanking.ApiClient('AllianzBankFinancialAdvisors', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AllianzBankFinancialAdvisors
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
AllianzBanqueConnectorSettings
Settings for initializing ApiClient for Allianz Banque (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
AlphaBankCYConnectorSettings
Settings for initializing ApiClient for AlphaBankCY
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ocpApimSubscriptionKey |
string |
true |
none |
pispOcpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Developer Portal) |
AlphaBankGRConnectorSettings
Settings for initializing ApiClient for AlphaBankGR
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Developer Portal) |
pispOcpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Developer Portal) |
AlphaBankROConnectorSettings
Settings for initializing ApiClient for AlphaBankRO
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ocpApimSubscriptionKey |
string |
true |
none |
pispOcpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Developer Portal) |
AmExConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AmExConnectorSettings;
ApiClient apiClient = new ApiClient(
new AmExConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AmExConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('AmEx', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AmEx
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
AmExNewConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AmExNewConnectorSettings;
ApiClient apiClient = new ApiClient(
new AmExNewConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.AmExNewConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('AmExNew', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for AmExNew
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
AndbankConnectorSettings
Settings for initializing ApiClient for [Andbank]
Credentials can be obtained at Andbank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
AndbankLUConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
AndelskassenFaelleskassenConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
ApoBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ApoBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new ApoBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ApoBankConnectorSettings(
country="your-country",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('ApoBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for ApoBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ArbejdernesLandsbankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
ArgentaConnectorSettings
Settings for initializing ApiClient for Argenta Bank (Poland).
Credentials can obtained at Argenta API Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from the API Portal) |
apiSecret |
string |
true |
API secret (obtained from the API Portal) |
apiKey |
string |
true |
API key (obtained from the API Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
ArionBankiConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ArionBankiConnectorSettings;
ApiClient apiClient = new ApiClient(
new ArionBankiConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.apiKey("your-api-key")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ArionBankiConnectorSettings(
client_id="your-client-id",
api_key="your-api-key",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
apiKey: "your-api-key",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('ArionBanki', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Arion banki
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
language |
string |
false |
Language of authentication user interface. |
clientId |
string |
true |
API client ID. |
apiKey |
string |
true |
API key (obtained from the API Portal) |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ArkeaBankingServicesConnectorSettings
Settings for initializing ApiClient for Arkea Banking Services (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
ArkeaBanqueEntreprisesConnectorSettings
Settings for initializing ApiClient for Arkea Banque Entreprises (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
ArkeaBanquePriveeConnectorSettings
Settings for initializing ApiClient for Arkea Banque Privee (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
ArquiaConnectorSettings
Settings for initializing ApiClient for [Arquia]
Credentials can be obtained at Arquia Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
AskimOgSpydebergSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AskimOgSpydebergSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new AskimOgSpydebergSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Askim og Spydeberg Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
AtlanticoEuropaConnectorSettings
Settings for initializing ApiClient for BANCO ATLANTICO EUROPA, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
AtruviaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AtruviaConnectorSettings;
ApiClient apiClient = new ApiClient(
new AtruviaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true)
.brand("Berliner Atruvia branches");
import enablebanking
connector_settings = enablebanking.AtruviaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
brand="Berliner Atruvia branches"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
brand: "Berliner Atruvia branches"
}
const apiClient = new enablebanking.ApiClient('Atruvia', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Atruvia
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
brand |
string |
true |
Name of Atruvia branches |
AurlandSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AurlandSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new AurlandSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Aurland Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BAWAGConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BAWAGConnectorSettings;
ApiClient apiClient = new ApiClient(
new BAWAGConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BAWAGConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BAWAG', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BAWAG
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BBVAConnectorSettings
Settings for initializing ApiClient for [BBVA]
Credentials can be obtained at BBVA Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
BIBConnectorSettings
Settings for initializing ApiClient for [Baltic International Bank] (https://www.bib.eu/)
Credentials can be obtained at Baltic International Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BKSBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BKSBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new BKSBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BKSBankConnectorSettings(
country="your-country",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BKSBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BKS Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BKSBankSIConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BKSBankSIConnectorSettings;
ApiClient apiClient = new ApiClient(
new BKSBankSIConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BKSBankSIConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BKSBankSI', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BSK Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BKSHRConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BKSHRConnectorSettings;
ApiClient apiClient = new ApiClient(
new BKSHRConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BKSHRConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BKSHR', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BKSHR
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BNBankBusinessConnectorSettings
Settings for initializing ApiClient for BN Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BNBankConnectorSettings
Settings for initializing ApiClient for BN Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BNGConnectorSettings
Settings for initializing ApiClient for BNG Bank (Netherlands).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from BNG Bank Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
BNLBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BNLBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new BNLBusinessConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BNLBusinessConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('BNLBusiness', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BNLBusiness
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
BNLConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BNLConnectorSettings;
ApiClient apiClient = new ApiClient(
new BNLConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.brand("brands connected through BNL's end point")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BNLConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
brand="brands connected through BNL's end point"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
brand: "brands connected through BNL's end point"
}
const apiClient = new enablebanking.ApiClient('BNL', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BNL
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
brand |
string |
true |
Brands connected through BNL's end point |
BNPParibasFRBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BNPParibasFRBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new BNPParibasFRBusinessConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BNPParibasFRBusinessConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('BNPParibasFRBusiness', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BNPParibasFRBusiness
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
BNPParibasFRPersonalConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BNPParibasFRPersonalConnectorSettings;
ApiClient apiClient = new ApiClient(
new BNPParibasFRPersonalConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BNPParibasFRPersonalConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('BNPParibasFRPersonal', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BNPParibasFRPersonal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
BNPParibasFortisBusinessConnectorSettings
Settings for initializing ApiClient for BNP Paribas Fortis (Belgium).
Credentials can obtained at BNP Paribas Fortis Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from BNP Paribas Fortis Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from BNP Paribas Fortis Developer Portal) |
brand |
string |
false |
Bank brand. Allowed values are "BNP Paribas Fortis", "Hello Bank" and "Fintro" |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BNPParibasFortisConnectorSettings
Settings for initializing ApiClient for BNP Paribas Fortis (Belgium).
Credentials can obtained at BNP Paribas Fortis Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from BNP Paribas Fortis Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from BNP Paribas Fortis Developer Portal) |
brand |
string |
false |
Bank brand. Allowed values are "BNP Paribas Fortis", "Hello Bank" and "Fintro" |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BNPParibasPolandConnectorSettings
Settings for initializing ApiClient for BNP Paribas Bank Polska (Poland).
Credentials can obtained at BNP Paribas Poland Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from BNP Paribas Poland Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from BNP Paribas Poland Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded without padding) |
signCertUrl |
string |
true |
Public URL where QSeal certificate can be found |
tppId |
string |
true |
ID assigned to the TPP by an authority (format: PSDXX-YYYY-ZZZZZZZZ) |
BOIConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BOIConnectorSettings;
ApiClient apiClient = new ApiClient(
new BOIConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.clientId("your-client-id")
.business(false)
.sandbox(true);
Settings for initializing ApiClient for BOI
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
BPCEConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BPCEConnectorSettings;
ApiClient apiClient = new ApiClient(
new BPCEConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.clientId("your-client-id")
.brand("bpce-brands")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BPCEConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
client_id="your-client-id",
brand="bpce-brands"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
clientId: "your-client-id",
brand: "bpce-brands"
}
const apiClient = new enablebanking.ApiClient('BPCE', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BPCE Group
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
clientId |
string |
true |
API client ID. |
brand |
string |
true |
BPCE brands. |
BPEConnectorSettings
Settings for initializing ApiClient for BPE / Louvre Banque Privée (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
BPERBancaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BPERBancaConnectorSettings;
ApiClient apiClient = new ApiClient(
new BPERBancaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.brand("brands connected through BPER Banca's end point")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BPERBancaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
brand="brands connected through BPER Banca's end point"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
brand: "brands connected through BPER Banca's end point"
}
const apiClient = new enablebanking.ApiClient('BPERBanca', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BPERBanca
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
brand |
string |
true |
Brands connected through BPER Banca's end point |
BPIConnectorSettings
Settings for initializing ApiClient for BANCO BPI, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
BRDBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BRDBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new BRDBusinessConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BRDBusinessConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
signCertPath="your-sign-cert-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path"
}
const apiClient = new enablebanking.ApiClient('BRDBusiness', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BRDBusiness
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
BRDConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BRDConnectorSettings;
ApiClient apiClient = new ApiClient(
new BRDConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BRDConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BRD', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BRD
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BancaComercialaRomanaConnectorSettings
Settings for Erste&Steiermärkische Bank (Bank in Croatia Republic)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API key (obtained from the API Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BancaCredifarmaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancaCredifarmaConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancaCredifarmaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancaCredifarmaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('BancaCredifarma', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancaCredifarma
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
BancaFideuramConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancaFideuramConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancaFideuramConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancaFideuramConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('BancaFideuram', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancaFideuram
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
BancaMarchConnectorSettings
Settings for initializing ApiClient for [BancaMarch]
Credentials can be obtained at Banca March Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
BancaMediolanumConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancaMediolanumConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancaMediolanumConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancaMediolanumConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BancaMediolanum', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Banca Mediolanum
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BancaPassadoreConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancaPassadoreConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancaPassadoreConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancaPassadoreConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('BancaPassadore', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CBIGlobe
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
BancaPopolareDellAltoAdigeConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancaPopolareDellAltoAdigeConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancaPopolareDellAltoAdigeConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancaPopolareDellAltoAdigeConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('BancaPopolareDellAltoAdige', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancaPopolareDellAltoAdige
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
BancaPopolareDiSondrioConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancaPopolareDiSondrioConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancaPopolareDiSondrioConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancaPopolareDiSondrioConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('BancaPopolareDiSondrio', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancaPopolareDiSondrio
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
BancoBPMConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancoBPMConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancoBPMConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.brand("Brands connected through BPM's end point")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancoBPMConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
brand="Brands connected through BPM's end point"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
brand: "Brands connected through BPM's end point"
}
const apiClient = new enablebanking.ApiClient('BancoBPM', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancoBPM
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
brand |
string |
true |
Brands connected through BPM's end point |
BancoCaminosConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancoCaminosConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancoCaminosConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.signKeyPath("your-sign-key-path")
.signCertPath("your-sign-cert-path")
.certPath("your-cert-path")
.signPubKeySerial("your-sign-pub-key-serial")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancoCaminosConnectorSettings(
client_id="your-client-id",
sign_key_path="your-sign-key-path",
sign_cert_path="your-sign-cert-path",
cert_path="your-cert-path",
sign_pub_key_serial="your-sign-pub-key-serial",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
signKeyPath: "your-sign-key-path",
signCertPath: "your-sign-cert-path",
certPath: "your-cert-path",
signPubKeySerial: "your-sign-pub-key-serial",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BancoCaminos', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancoCaminos
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BancoCetelemConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancoCetelemConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancoCetelemConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.signKeyPath("your-sign-key-path")
.signCertPath("your-sign-cert-path")
.certPath("your-cert-path")
.signPubKeySerial("your-sign-pub-key-serial")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancoCetelemConnectorSettings(
client_id="your-client-id",
sign_key_path="your-sign-key-path",
sign_cert_path="your-sign-cert-path",
cert_path="your-cert-path",
sign_pub_key_serial="your-sign-pub-key-serial",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
signKeyPath: "your-sign-key-path",
signCertPath: "your-sign-cert-path",
certPath: "your-cert-path",
signPubKeySerial: "your-sign-pub-key-serial",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BancoCetelem', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancoCetelem
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BancoEuropeoDeFinanzasConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancoEuropeoDeFinanzasConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancoEuropeoDeFinanzasConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.signKeyPath("your-sign-key-path")
.signCertPath("your-sign-cert-path")
.certPath("your-cert-path")
.signPubKeySerial("your-sign-pub-key-serial")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancoEuropeoDeFinanzasConnectorSettings(
client_id="your-client-id",
sign_key_path="your-sign-key-path",
sign_cert_path="your-sign-cert-path",
cert_path="your-cert-path",
sign_pub_key_serial="your-sign-pub-key-serial",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
signKeyPath: "your-sign-key-path",
signCertPath: "your-sign-cert-path",
certPath: "your-cert-path",
signPubKeySerial: "your-sign-pub-key-serial",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BancoEuropeoDeFinanzas', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancoEuropeoDeFinanzas
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BancoInversisConnectorSettings
Settings for initializing ApiClient for [Banco Inversis]
Credentials can be obtained at BancoInversis Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
BancoInvestimentoGlobalConnectorSettings
Settings for initializing ApiClient for BANCO DE INVESTIMENTO GLOBAL ESPANHA, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
BancoMediolanumConnectorSettings
Settings for initializing ApiClient for [BancoMediolanum]
Credentials can be obtained at Banco Mediolanum Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
BancoPichinchaConnectorSettings
Settings for initializing ApiClient for [BancoPichincha]
Credentials can be obtained at Banco Pichincha Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
BancoSantanderConnectorSettings
Settings for initializing ApiClient for [BancoSantander]
Credentials can be obtained at Banco Santander Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
BancoSantanderCorporateConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancoSantanderCorporateConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancoSantanderCorporateConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.signKeyPath("your-sign-key-path")
.signCertPath("your-sign-cert-path")
.certPath("your-cert-path")
.signPubKeySerial("your-sign-pub-key-serial")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancoSantanderCorporateConnectorSettings(
client_id="your-client-id",
sign_key_path="your-sign-key-path",
sign_cert_path="your-sign-cert-path",
cert_path="your-cert-path",
sign_pub_key_serial="your-sign-pub-key-serial",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
signKeyPath: "your-sign-key-path",
signCertPath: "your-sign-cert-path",
certPath: "your-cert-path",
signPubKeySerial: "your-sign-pub-key-serial",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BancoSantanderCorporate', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BancoSantanderCorporate
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BancofarConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BancofarConnectorSettings;
ApiClient apiClient = new ApiClient(
new BancofarConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.signKeyPath("your-sign-key-path")
.signCertPath("your-sign-cert-path")
.certPath("your-cert-path")
.signPubKeySerial("your-sign-pub-key-serial")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BancofarConnectorSettings(
client_id="your-client-id",
sign_key_path="your-sign-key-path",
sign_cert_path="your-sign-cert-path",
cert_path="your-cert-path",
sign_pub_key_serial="your-sign-pub-key-serial",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
signKeyPath: "your-sign-key-path",
signCertPath: "your-sign-cert-path",
certPath: "your-cert-path",
signPubKeySerial: "your-sign-pub-key-serial",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Bancofar', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Bancofar
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BankDeKremerConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BankDeKremerConnectorSettings;
ApiClient apiClient = new ApiClient(
new BankDeKremerConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BankDeKremerConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BankDeKremer', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BankDeKremer
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BankNordikDenmarkConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BankNordikDenmarkConnectorSettings;
ApiClient apiClient = new ApiClient(
new BankNordikDenmarkConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for BankNordik Denmark.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BankNordikFaroeIslandsConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BankNordikFaroeIslandsConnectorSettings;
ApiClient apiClient = new ApiClient(
new BankNordikFaroeIslandsConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for BankNordik Faroe Islands.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BankNorwegianConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BankNorwegianConnectorSettings;
ApiClient apiClient = new ApiClient(
new BankNorwegianConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("NO")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BankNorwegianConnectorSettings(
country="NO",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "NO",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('BankNorwegian', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Bank Norwegian
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
BankVanBredaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BankVanBredaConnectorSettings;
ApiClient apiClient = new ApiClient(
new BankVanBredaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BankVanBredaConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BankVanBreda', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BankVanBreda
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BankhausFaisstConnectorSettings
Settings for initializing ApiClient for BankhausFaisst (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
BankhausLampeConnectorSettings
Settings for initializing ApiClient for BankhausLampe (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
BankhausWerhahnConnectorSettings
Settings for initializing ApiClient for BankhausWerhahn (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
BankinterConnectorSettings
Settings for initializing ApiClient for [Bankinter]
Credentials can be obtained at Bankinter Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
BankinterLUConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BankinterSucursalConnectorSettings
Settings for initializing ApiClient for BANKINTER, SA - SUCURSAL EM PORTUGAL (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
BanqueCPHConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BanqueCPHConnectorSettings;
ApiClient apiClient = new ApiClient(
new BanqueCPHConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BanqueCPHConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BanqueCPH', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Banque CPH
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BanqueDeLuxembourgBEConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BanqueDeLuxembourgConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BanqueDePatrimoinesPrivesConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BanqueEuropeenneFRConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BanqueEuropeenneFRConnectorSettings;
ApiClient apiClient = new ApiClient(
new BanqueEuropeenneFRConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BanqueEuropeenneFRConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('BanqueEuropeenneFR', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Banque Européenne
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
BanqueTransatlantiqueBEConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BanqueTransatlantiqueConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BanqueTransatlantiqueConnectorSettings;
ApiClient apiClient = new ApiClient(
new BanqueTransatlantiqueConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BanqueTransatlantiqueConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('BanqueTransatlantique', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Banque Transatlantique
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
BanqueTransatlantiqueLUConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
BasisbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BasisbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new BasisbankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Basisbank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BelfiusConnectorSettings
Settings for initializing ApiClient for Belfius (BE)
Credentials can obtained at Belfius (BE) Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Belfius Developer Portal). |
clientSecret |
string |
false |
API client Secret (obtained from Belfius Developer Portal). Required for production, not used in sandbox. |
certPath |
string |
false |
Client secret obtained from SDC in onboarding process. Required for production, not used in sandbox. |
keyPath |
string |
false |
Path to QWAC certificate in PEM format. Required for production, not used in sandbox. |
language |
string |
true |
Language of authentication user interface. The default language is |
BeobankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BeobankConnectorSettings;
ApiClient apiClient = new ApiClient(
new BeobankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BeobankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('Beobank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Beobank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
BerenbergConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BerenbergConnectorSettings;
ApiClient apiClient = new ApiClient(
new BerenbergConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BerenbergConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Berenberg', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Berenberg
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BethmannBankConnectorSettings
Settings for initializing ApiClient for BethmannBank (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
BetriFOConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BetriFOConnectorSettings;
ApiClient apiClient = new ApiClient(
new BetriFOConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Betri (FO).
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BicPortuguesConnectorSettings
Settings for initializing ApiClient for BANCO BIC PORTUGUES, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
BorbjergSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BorbjergSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new BorbjergSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Borbjerg Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BoursoramaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BoursoramaConnectorSettings;
ApiClient apiClient = new ApiClient(
new BoursoramaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BoursoramaConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('Boursorama', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Boursorama
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
BpostBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BpostBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new BpostBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BpostBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BpostBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for BpostBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BrabankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BrabankConnectorSettings;
ApiClient apiClient = new ApiClient(
new BrabankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Brabank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BrabankSverigeConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BrabankSverigeConnectorSettings;
ApiClient apiClient = new ApiClient(
new BrabankSverigeConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Brabank Sverige.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BredBanquePopulaireConnectorSettings
Settings for initializing ApiClient for LCL
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
xApiKey |
string |
true |
API client key (obtained from the Developer Portal) |
clientId |
string |
true |
API client ID. |
BroagerSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BroagerSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new BroagerSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Broager Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
BuddyBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BuddyBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new BuddyBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.BuddyBankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('BuddyBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Buddy Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
BundesbankConnectorSettings
Settings for initializing ApiClient for Deutsche Bundesbank (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
BunqConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.BunqConnectorSettings;
ApiClient apiClient = new ApiClient(
new BunqConnectorSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.sandbox(true));
Settings for initializing ApiClient for Bunq.
Credentials can obtained at Bunq Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client ID |
clientSecret |
string |
true |
Client secret |
CIBBankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CIBBankBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new CIBBankBusinessConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CIBBankBusinessConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('CIBBankBusiness', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CIB Bank Business
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CIBBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CIBBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new CIBBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CIBBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('CIBBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CIB Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CICBanquePriveeConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CICBanquePriveeConnectorSettings;
ApiClient apiClient = new ApiClient(
new CICBanquePriveeConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CICBanquePriveeConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('CICBanquePrivee', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CIC Banque Privée
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
CICConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CICConnectorSettings;
ApiClient apiClient = new ApiClient(
new CICConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CICConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('CIC', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CIC
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
CSOBCZConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CSOBCZConnectorSettings;
ApiClient apiClient = new ApiClient(
new CSOBCZConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.apiKey("your-api-key")
.certPath("your-cert-path")
.keyPath("your-key-path")
.companyName("your-company-name")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CSOBCZConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
api_key="your-api-key",
cert_path="your-cert-path",
key_path="your-key-path",
company_name="your-company-name"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
apiKey: "your-api-key",
certPath: "your-cert-path",
keyPath: "your-key-path",
companyName: "your-company-name"
}
const apiClient = new enablebanking.ApiClient('CSOBCZ', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CSOBCZ
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API client key. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
companyName |
string |
true |
none |
CSOBSKConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CSOBSKConnectorSettings;
ApiClient apiClient = new ApiClient(
new CSOBSKConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CSOBSKConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('CSOBSK', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CSOBSK
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CTTConnectorSettings
Settings for initializing ApiClient for BANCO CTT, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaisseDEpargneConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CaisseDEpargneConnectorSettings;
ApiClient apiClient = new ApiClient(
new CaisseDEpargneConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.clientId("your-client-id")
.brand("your-brand")
.business(false)
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CaisseDEpargneConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
client_id="your-client-id",
brand="your-brand",
business=False
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
clientId: "your-client-id",
brand: "your-brand",
business: "false"
}
const apiClient = new enablebanking.ApiClient('CaisseDEpargne', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CaisseDEpargne
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
clientId |
string |
true |
API client ID. |
brand |
string |
true |
Denotes the bank where the account is held. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
CaixaBankConnectorSettings
Settings for initializing ApiClient for [CaixaBank]
Credentials can be obtained at CaixaBank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
CaixaCentralCreditoAgricolaMutuoConnectorSettings
Settings for initializing ApiClient for CAIXA CENTRAL - CAIXA CENTRAL DE CREDITO AGRICOLA MUTUO, CRL (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaCreditoAgricolaMutuoBombarralConnectorSettings
Settings for initializing ApiClient for Caixa De Credito Agricola Mutuo De Bombarral Crl (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaCreditoAgricolaMutuoChamuscaConnectorSettings
Settings for initializing ApiClient for Caixa De Credito Agricola Mutuo Da Chamusca, C.r.l (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaCreditoAgricolaMutuoLeiriaConnectorSettings
Settings for initializing ApiClient for CAIXA DE CREDITO AGRICOLA MUTUO DE LEIRIA, CRL (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaCreditoAgricolaMutuoMafraConnectorSettings
Settings for initializing ApiClient for Caixa De Credito Agricola Mutuo De Mafra, C.R.L. (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaCreditoAgricolaMutuoTorresConnectorSettings
Settings for initializing ApiClient for Caixa De Credito Agricola Mutuo De Torres Vedras,c.r.l (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaEconomicaMisericordiaAngraHeroismoConnectorSettings
Settings for initializing ApiClient for CAIXA ECONOMICA DA MISERICORDIA DE ANGRA DO HEROISMO (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaEconomicaMontepioGeralConnectorSettings
Settings for initializing ApiClient for CAIXA ECONOMICA MONTEPIO GERAL (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaGeralDepositosConnectorSettings
Settings for initializing ApiClient for CAIXA GERAL DE DEPOSITOS, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CaixaGeralDepositosFranceConnectorSettings
Settings for initializing ApiClient for CAIXA GERAL DE DEPOSITOS FRANCE (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CajaRuralConnectorSettings
Settings for initializing ApiClient for [CajaRural]
Credentials can be obtained at Caja Rural Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
brand |
string |
true |
Name of rural bank. e.g. (Caja Rural Del Sur, Caixa Rural Benicarlo, etc) |
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
CajamarCajaRuralConnectorSettings
Settings for initializing ApiClient for [CajamarCajaRural]
Credentials can be obtained at Cajamar Caja Rural Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
CajasurBancoConnectorSettings
Settings for initializing ApiClient for [CajasurBanco]
Credentials can be obtained at Cajasur Banco Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
CecabankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CecabankConnectorSettings;
ApiClient apiClient = new ApiClient(
new CecabankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CecabankConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Cecabank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Cecabank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CeskaSporitelnaConnectorSettings
Settings for Ceska Sporitelna (Bank in Czech Republic)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API key (obtained from the API Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CheBancaCorporateConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CheBancaCorporateConnectorSettings;
ApiClient apiClient = new ApiClient(
new CheBancaCorporateConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CheBancaCorporateConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('CheBancaCorporate', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CheBancaCorporate
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
CitadeleConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
false |
Bank`s country |
language |
string |
false |
Language of authentication user interface. |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
sandboxConsentId |
string |
false |
Static consent id with approved status used in sandbox |
CitiHandlowyConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CitiHandlowyConnectorSettings;
ApiClient apiClient = new ApiClient(
new CitiHandlowyConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CitiHandlowyConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('CitiHandlowy', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for citiHandlowy
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CofidisConnectorSettings
Settings for initializing ApiClient for COFIDIS (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
ColonyaConnectorSettings
Settings for initializing ApiClient for [Colonya]
Credentials can be obtained at Colonya Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
ComdirectConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ComdirectConnectorSettings;
ApiClient apiClient = new ApiClient(
new ComdirectConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ComdirectConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Comdirect', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Comdirect
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ComercialPortuguesConnectorSettings
Settings for initializing ApiClient for BANCO COMERCIAL PORTUGUES, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
CommerzbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CommerzbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new CommerzbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CommerzbankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Commerzbank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Commerzbank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ConnectPayConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ConnectPayConnectorSettings;
ApiClient apiClient = new ApiClient(
new ConnectPayConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ConnectPayConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('ConnectPay', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for ConnectPay
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
ConsorsBankConnectorSettings
Settings for initializing Consorsbank and BNP Paribas Wealth Management.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ConsorsFinanzConnectorSettings
Settings for initializing ApiClient for Consors Finanz, BNP Paribas S.A. Niederlassung Deutschland (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
CoopBankDenmarkConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SydbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SydbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
CoopPankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
xApiKey |
string |
true |
API client key (obtained in developer portal) |
certPath |
string |
false |
Path to QWAC certificate in PEM format |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
CreatisConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreatisConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreatisConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreatisConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('Creatis', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Creatis
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
CreditAgricoleFRConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditAgricoleFRConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditAgricoleFRConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.brand("credit-agricole-branches")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditAgricoleFRConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
brand="credit-agricole-branches"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
brand: "credit-agricole-branches"
}
const apiClient = new enablebanking.ApiClient('CreditAgricoleFR', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CreditAgricoleFR
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
brand |
string |
true |
One of available brands. |
tppName |
string |
true |
Name of the TPP using the connector. |
CreditAgricoleITBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditAgricoleITBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditAgricoleITBusinessConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditAgricoleITBusinessConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('CreditAgricoleITBusiness', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CreditAgricoleITBusiness
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
CreditAgricoleITPersonalConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditAgricoleITPersonalConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditAgricoleITPersonalConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditAgricoleITPersonalConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('CreditAgricoleITPersonal', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CreditAgricoleITPersonal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
CreditAgricolePolandConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditAgricolePolandConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditAgricolePolandConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditAgricolePolandConnectorSettings(
client_id="your-client-id",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('CreditAgricolePoland', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CreditAgricolePoland
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Alior Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded with padding) |
CreditEuropeBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditEuropeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditEuropeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditEuropeBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('CreditEuropeBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Credit Europe Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CreditMunicipalConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditMunicipalConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditMunicipalConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditMunicipalConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('CreditMunicipal', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Credit Municipal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
CreditMutuelBretagneConnectorSettings
Settings for initializing ApiClient for Credit Mutuel de Bretagne (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
CreditMutuelConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditMutuelConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditMutuelConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditMutuelConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('CreditMutuel', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Credit Mutuel
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
CreditMutuelSudOuestConnectorSettings
Settings for initializing ApiClient for Credit Mutuel SudOuest (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
CreditoEmilianoConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CreditoEmilianoConnectorSettings;
ApiClient apiClient = new ApiClient(
new CreditoEmilianoConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.brand("brands connected through Credito Emiliano's end point)
.sandbox(true);
import enablebanking
connector_settings = enablebanking.CreditoEmilianoConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
brand="brands connected through Credito Emiliano's end point"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
brand: "brands connected through Credito Emiliano's end point"
}
const apiClient = new enablebanking.ApiClient('CreditoEmiliano', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for CreditoEmiliano
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
brand |
string |
true |
Brands connected through Credito Emiliano's end point |
CrelanConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client id (obtained from Crelan developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
CulturaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.CulturaConnectorSettings;
ApiClient apiClient = new ApiClient(
new CulturaConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Cultura.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
DABBNPParibasConnectorSettings
Settings for initializing DAB BNP Paribas (Gernamy).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DBSConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DBSConnectorSettings;
ApiClient apiClient = new ApiClient(
new DBSConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DBSConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DBS', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DBS
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DKBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DKBConnectorSettings;
ApiClient apiClient = new ApiClient(
new DKBConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DKBConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DKB', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DKB
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DNBBusinessConnectorSettings
Settings for initializing ApiClient for DNB Bank (Norway).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to a QWAC public certificate |
keyPath |
string |
true |
Path to a QWAC private key |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
DNBConnectorSettings
Settings for initializing ApiClient for DNB Bank (Norway).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to a QWAC public certificate |
keyPath |
string |
true |
Path to a QWAC private key |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
DanskeAndelskassersBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
DanskeBankBusinessConnectorSettings
Settings for initializing ApiClient for DanskeBank
Credentials can obtained at Danske Bank Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
false |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
DanskeBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DanskeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new DanskeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.sandbox(true));
Settings for initializing ApiClient for DanskeBank
Credentials can obtained at Danske Bank Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
false |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
business |
boolean |
false |
Account type to access to. If true business, if false personal. |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
DanskeBankPersonalConnectorSettings
Settings for initializing ApiClient for DanskeBank
Credentials can obtained at Danske Bank Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
false |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
DegussaBankConnectorSettings
Settings for initializing ApiClient for Degussa Bank AG (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
DelavskaHranilnicaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DelavskaHranilnicaConnectorSettings;
ApiClient apiClient = new ApiClient(
new DelavskaHranilnicaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DelavskaHranilnicaConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DelavskaHranilnica', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Delavska Hranilnica
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DenLilleBikubeConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DenLilleBikubeConnectorSettings;
ApiClient apiClient = new ApiClient(
new DenLilleBikubeConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Den Lille Bikube.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
DeutscheBankBEConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankBEConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankBEConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankBEConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankBE', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DeutscheBankBE
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DeutscheBankBusinessESConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankBusinessESConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankBusinessESConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankBusinessESConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankBusinessES', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DeutscheBankBusinessES
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DeutscheBankBusinessEUConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankBusinessEUConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankBusinessEUConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankBusinessEUConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankBusinessEU', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DeutscheBankBusinessEU
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DeutscheBankDEConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankDBConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankDBConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankDBConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankDB', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DeutscheBankDE
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DeutscheBankESConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankESConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankESConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankESConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankES', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DeutscheBankES
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DeutscheBankITConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankITConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankITConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true)
.business(false);
import enablebanking
connector_settings = enablebanking.DeutscheBankITConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
business: False
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
business: false
}
const apiClient = new enablebanking.ApiClient('DeutscheBankIT', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DeutscheBankIT
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
business |
boolean |
true |
none |
DeutscheBankLUConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankLUConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankLUConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankLUConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankLU', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DeutscheBankLU
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DiPocketConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DiPocketConnectorSettings;
ApiClient apiClient = new ApiClient(
new DiPocketConnectorSettings()
.redirectUri("https://your-redirect-uri")
.brand("your-brand")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DiPocketConnectorSettings(
brand="your-brand",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
brand: "your-brand",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DiPocket', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for DiPocket
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country where PSU using the integration resides. |
brand |
string |
true |
Denotes the brand used by the PSU as DiPocket operates under multiple. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DinersClubConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DinersClubConnectorSettings;
ApiClient apiClient = new ApiClient(
new DinersClubConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DinersClubConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DinersClub', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Diners Club
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
DjurslandsBankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DjurslandsBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new DjurslandsBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for DjurslandsBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
DjurslandsBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DjurslandsBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new DjurslandsBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for DjurslandsBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
DragsholmSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DragsholmSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new DragsholmSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Dragsholm Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
DrangedalSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DrangedalSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new DrangedalSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Drangedal Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
DronninglundSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DronninglundSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new DronninglundSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Dronninglund Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
DynamicMockASPSPConnectorSettings
Settings for initializing ApiClient for Mock ASPSP using data input from Enable Banking Control Panel
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
origin |
string |
true |
Origin of the API providing data for the connector. |
cpOrigin |
string |
true |
Origin of the Control Panel used for editing mock data. |
EVOBancoConnectorSettings
Settings for initializing ApiClient for [EVOBanco]
Credentials can be obtained at EVO Banco Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
EastWestUnitedBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
EasybankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.EasybankConnectorSettings;
ApiClient apiClient = new ApiClient(
new EasybankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Easybank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
EihbankConnectorSettings
Settings for initializing ApiClient for Europäisch-Iranische Handelsbank AG (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
EikaBusinessConnectorSettings
Settings for initializing ApiClient for Sparebank 1
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
brand |
string |
true |
Denotes the bank where the account is held. |
EikaPersonalConnectorSettings
Settings for initializing ApiClient for Sparebank 1
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
brand |
string |
true |
Denotes the bank where the account is held. |
EkobankenMedlemsbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.EkobankenMedlemsbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new EkobankenMedlemsbankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Ekobanken Medlemsbank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
EllouConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.EllouConnectorSettings;
ApiClient apiClient = new ApiClient(
new EllouConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.EllouConnectorSettings(
country="your-country",
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Ellou', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Ellou
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ErsteBankATConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ErsteBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new ErsteBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.apiKey("your-api-key")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ErsteBankConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
api_key="your-api-key",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
apiKey: "your-api-key",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('ErsteBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Erste Bank (Austria)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API key (obtained from the API Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ErsteBankHUCorporateConnectorSettings
Settings for initializing ApiClient for the corporate business of ErsteBank in Hungary
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API key (obtained from the API Portal). |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ErsteBankHURetailConnectorSettings
Settings for initializing ApiClient for the retail business of ErsteBank in Hungary
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API key (obtained from the API Portal). |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ErsteSteiermarkischeBankConnectorSettings
Settings for Erste&Steiermärkische Bank (Bank in Croatia Republic)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API key (obtained from the API Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
EtneSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Etne Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
EtneSparebankConnectorSettings
Settings for initializing ApiClient for Etne Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
EurobankConnectorSettings
Settings for initializing ApiClient for Eurobank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Developer Portal) |
pispOcpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Developer Portal) |
EurobankPrivateBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
EurocajaRuralConnectorSettings
Settings for initializing ApiClient for [EurocajaRural]
Credentials can be obtained at Eurocaja Rural Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
EuropabankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.EuropabankConnectorSettings;
ApiClient apiClient = new ApiClient(
new EuropabankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.EuropabankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Europabank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Europabank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
FacitBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FacitBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new FacitBankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Facit bank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
FacitbankNOConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FacitbankNOConnectorSettings;
ApiClient apiClient = new ApiClient(
new FacitbankNOConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Facitbank NO.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
FanaSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Fana Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
FanaSparebankConnectorSettings
Settings for initializing ApiClient for Fana Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
FanoeSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FanoeSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new FanoeSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Fanø Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
FasterAndelskasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
FerratumBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FerratumBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new FerratumBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.hmac("your-hmac")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.FerratumBankConnectorSettings(
country="your-country",
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
hmac="your-hmac"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
hmac: "your-hmac"
}
const apiClient = new enablebanking.ApiClient('FerratumBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for FerratumBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
hmac |
string |
true |
none |
FiareBancaEticaConnectorSettings
Settings for initializing ApiClient for [FiareBancaEtica]
Credentials can be obtained at Fiare Banca Etica Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
FideuramBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
FindomesticConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FindomesticConnectorSettings;
ApiClient apiClient = new ApiClient(
new FindomesticConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.FindomesticConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('Findomestic', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Findomestic
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
FinecobankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FinecobankConnectorSettings;
ApiClient apiClient = new ApiClient(
new FinecobankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.FinecobankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Finecobank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Finecobank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
FlekkefjordSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Flekkefjord Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
FlekkefjordSparebankConnectorSettings
Settings for initializing ApiClient for Flekkefjord Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
FolkesparekassenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FolkesparekassenConnectorSettings;
ApiClient apiClient = new ApiClient(
new FolkesparekassenConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Folkesparekassen.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
ForbrugsforeningenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ForbrugsforeningenConnectorSettings;
ApiClient apiClient = new ApiClient(
new ForbrugsforeningenConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Forbrugsforeningen.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
FortuneoConnectorSettings
Settings for initializing ApiClient for Fortuneo (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
FroesSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.FroesSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new FroesSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Frøs Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
FrorupAndelskasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
FroslevMollerupSparekasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
FynskeBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
GBKRConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.GBKRConnectorSettings;
ApiClient apiClient = new ApiClient(
new GBKRConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.GBKRConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('GBKR', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for GBKR
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
GranitBankConnectorSettings
Settings for initializing ApiClient for Granit Bank(Hungary)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
GronlandsBANKENConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
HPBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.HPBConnectorSettings;
ApiClient apiClient = new ApiClient(
new HPBConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.HPBConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('HPB', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for HPB
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
HSBCConnectorSettings
Settings for initializing ApiClient for HSBC.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
HSBCTrinkausConnectorSettings
Settings for initializing ApiClient for HSBC Trinkaus & Burkhardt AG (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
HandelsbankenBusinessConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country |
clientId |
string |
true |
API client id (obtained from Handelsbanken developer portal) |
certPath |
string |
true |
Path to a QWAC public certificate |
keyPath |
string |
true |
Path to a QWAC private key |
HandelsbankenConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country |
clientId |
string |
true |
API client id (obtained from Handelsbanken developer portal) |
certPath |
string |
true |
Path to a QWAC public certificate |
keyPath |
string |
true |
Path to a QWAC private key |
HandelsbankenDenmarkConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
HandelsbankenNorwayBusinessConnectorSettings
Settings for initializing ApiClient for Handelsbanken Norway
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
HandelsbankenNorwayConnectorSettings
Settings for initializing ApiClient for Handelsbanken Norway
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
HaugesundSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Haugesund Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
HaugesundSparebankConnectorSettings
Settings for initializing ApiClient for Haugesund Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
HelloBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.HelloBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new HelloBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.HelloBankConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('HelloBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for HelloBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
HelloBankFRConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.HelloBankFRConnectorSettings;
ApiClient apiClient = new ApiClient(
new HelloBankFRConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.HelloBankFRConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('HelloBankFR', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for HelloBankFR
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
HoenefossSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.HoenefossSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new HoenefossSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Hønefoss Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
HolviConnectorSettings
Settings for initializing ApiClient for Holvi (Finland).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Holvi onboarding) |
clientSecret |
string |
true |
API client secret (obtained from Holvi onboarding) |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
HvidbjergBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
HypoTirolConnectorSettings
Settings for initializing ApiClient for Hypo Tirol (Austra)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
HypoVereinsbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.HypoVereinsbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new HypoVereinsbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.business(false)
.country("DE")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.HypoVereinsbankConnectorSettings(
business=False,
country="DE",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
business: false,
country: "DE",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('HypoVereinsbank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for HypoVereinsbank (DE, GB)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
business |
boolean |
true |
none |
country |
string |
true |
Bank`s country |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ICABankenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ICABankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new ICABankenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.sandbox(true));
Settings for initializing ApiClient for ICA Banken.
Credentials can obtained at ICA Banken Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from ICA Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from ICA Developer Portal) |
certPath |
string |
false |
Path to QWAC certificate in PEM format. |
keyPath |
string |
false |
Path to QWAC certificate in PEM format. |
INGConnectorSettings
Settings for initializing ApiClient for ING Bank
Credentials can obtained at ING Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
INGPolandConnectorSettings
Settings for initializing ApiClient for ING Silesian Bank (Poland).
Credentials can obtained at ING Silesian Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from ING Silesian Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded without padding) |
signCertUrl |
string |
true |
Public URL where QSeal certificate can be found |
tppId |
string |
true |
ID assigned to the TPP by an authority (format: PSDXX-YYYY-ZZZZZZZZ) |
INGWholesaleBankingConnectorSettings
Settings for initializing ApiClient for ING Wholesale Banking Credentials can obtained at ING Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
ISPSloveniaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ISPSloveniaConnectorSettings;
ApiClient apiClient = new ApiClient(
new ISPSloveniaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ISPSloveniaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('ISPSlovenia', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for ISP Slovenia
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
IbercajaBancoConnectorSettings
Settings for initializing ApiClient for [IbercajaBanco]
Credentials can be obtained at Ibercaja Banco Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
IccreaBancaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.IccreaBancaConnectorSettings;
ApiClient apiClient = new ApiClient(
new IccreaBancaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret").
.brand("brands connected through Iccrea Banca's end point")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.IccreaBancaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
brand="brands connected through Iccrea Banca's end point"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
brand: "brands connected through Iccrea Banca's end point"
}
const apiClient = new enablebanking.ApiClient('IccreaBanca', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for IccreaBanca
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
brand |
string |
true |
Brands connected through Credit Agricole's end point |
IgeaDigitalBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.IgeaDigitalBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new IgeaDigitalBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.IgeaDigitalBankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('IgeaDigitalBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for IgeaDigitalBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
IkanoBankConnectorSettings
Settings for initializing ApiClient for Ikano Bank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
IkanoBankDKConnectorSettings
Settings for initializing ApiClient for Ikano Bank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
B Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process. |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
IndreSognSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.IndreSognSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new IndreSognSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Indre Sogn Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
IntesaSanpaoloConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.IntesaSanpaoloConnectorSettings;
ApiClient apiClient = new ApiClient(
new IntesaSanpaoloConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.IntesaSanpaoloConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('IntesaSanpaolo', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for IntesaSanpaolo
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
IntesaSanpaoloPrivateConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.IntesaSanpaoloPrivateConnectorSettings;
ApiClient apiClient = new ApiClient(
new IntesaSanpaoloPrivateConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.IntesaSanpaoloPrivateConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('IntesaSanpaoloPrivate', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for IntesaSanpaoloPrivate
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
IntesaSanpaoloWealthManagementConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
country |
string |
false |
Country code of the bank. Possilbe values: |
InvestimentoGlobalConnectorSettings
Settings for initializing ApiClient for BANCO DE INVESTIMENTO GLOBAL, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
IsbankConnectorSettings
Settings for initializing ApiClient for Isbank AG Zentrale Frankfurt (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
IslandsbankiConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.IslandsbankiConnectorSettings;
ApiClient apiClient = new ApiClient(
new IslandsbankiConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.IslandsbankiConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Islandsbanki', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Islandsbanki
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
IsybankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.IsybankConnectorSettings;
ApiClient apiClient = new ApiClient(
new IsybankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.IsybankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
}
const apiClient = new enablebanking.ApiClient('Isybank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Isybank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
JAKAndelskasseOesterVraaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.JAKAndelskasseOesterVraaConnectorSettings;
ApiClient apiClient = new ApiClient(
new JAKAndelskasseOesterVraaConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for J.A.K Andelskasse Øster Vrå.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
JAKMedlemsbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.JAKMedlemsbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new JAKMedlemsbankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for JAK Medlemsbank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
JTBankaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.JTBankaConnectorSettings;
ApiClient apiClient = new ApiClient(
new JTBankaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.tppName("your-tpp-name"),
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.JTBankaConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
tppName="your-tpp-name",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('JTBanka', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for JTBanka
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
tppName |
string |
true |
The name of the original TPP that created the request. Eg. ‘Star corporation, a.s.’. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
JuliusBaerConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
JutlanderBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.JutlanderBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new JutlanderBankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Jutlander Bank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
JyskeBankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.JyskeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new JyskeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for JyskeBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
JyskeBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.JyskeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new JyskeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for JyskeBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
KBCConnectorSettings
Settings for initializing ApiClient for KBC/CBC Bank
Credentials can obtained at KBC Developer Portla
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from the QWAC certificate) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
brand |
string |
true |
Name of brand. If not set defaults to KBC. Allowed values are "KBC", "CBC", "KBC Brussels" |
KHConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KHConnectorSettings;
ApiClient apiClient = new ApiClient(
new KHConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.KHConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
client_id: "your-client-id"
}
const apiClient = new enablebanking.ApiClient('KH', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for KH
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
KIRConnectorSettings
Setting for the connector to PolishAPI implementation provided by KIR.
Originally implemented for openbankinghackathon.pl
Sandbox only
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
origin |
string |
true |
API origin (scheme://domain:port) |
clientId |
string |
true |
TPP ID to be put here. KIR sandbox certificates use PSDPL-KNF-1234567890 |
certPath |
string |
true |
Path to sandbox QWAC certificate |
keyPath |
string |
true |
Path to sandbox key used for QWAC |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. If not set request signing will be |
signSubjectKeyIdentifier |
string |
false |
Subject Key Identifier (2.5.29.14) field of QSeal certificate as hex string |
signFingerprint |
string |
false |
Fingerprint of the QSeal certificate (base64 encoded without padding) |
signCertUrl |
string |
false |
Public URL where QSeal certificate can be found |
KLPBankenBusinessConnectorSettings
Settings for initializing ApiClient for KLP Banken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
KLPBankenConnectorSettings
Settings for initializing ApiClient for KLP Banken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
KeytradeBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KeytradeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new KeytradeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.KeytradeBankConnectorSettings(
country="your-country"
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country"
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('KeytradeBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for KeytradeBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
KlarnaConnectorSettings
Settings for initializing ApiClient for Klarna (Sweden and Germany)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
KlimSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KlimSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new KlimSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Klim Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
KnabConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KnabConnectorSettings;
ApiClient apiClient = new ApiClient(
new KnabConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.KnabConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Knab', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Knab
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
KomercniBankaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KomercniBankaConnectorSettings;
ApiClient apiClient = new ApiClient(
new KomercniBankaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.KomercniBankaConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('KomercniBanka', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Komercni Banka
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
KontistConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KontistConnectorSettings;
ApiClient apiClient = new ApiClient(
new KontistConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.KontistConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('Kontist', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Kontist
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
KreditbankenBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KreditbankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new KreditbankenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Kreditbanken
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
KreditbankenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KreditbankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new KreditbankenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Kreditbanken
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
KutxabankConnectorSettings
Settings for initializing ApiClient for [Kutxabank]
Credentials can be obtained at Kutxabank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
KvikaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KvikaConnectorSettings;
ApiClient apiClient = new ApiClient(
new KvikaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.KvikaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('Kvika', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Kvika
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
LCLConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LCLConnectorSettings;
ApiClient apiClient = new ApiClient(
new LCLConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.clientId("api-client-id")
.signCertUrl("your-public-qseal-certificate")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.LCLConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
client_id="api-client-id",
sign_cert_url=("your-public-qseal-certificate")
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
clientId: "api-client-id",
sign_cert_url: "your-public-qseal-certificate"
}
const apiClient = new enablebanking.ApiClient('LCL', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for LCL
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
clientId |
string |
true |
API client ID. |
signCertUrl |
string |
true |
Public URL where QSeal certificate can be found |
LHVBusinessConnectorSettings
Settings for initializing ApiClient for LHV Business (Estonia).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
clientId |
string |
true |
TPP ID (extracted from certificate, for example PSDEE-LHVTEST-01AAA) |
LHVConnectorSettings
Settings for initializing ApiClient for LHV (Estonia).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
clientId |
string |
true |
TPP ID (extracted from certificate, for example PSDEE-LHVTEST-01AAA) |
LKUConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LKUConnectorSettings;
ApiClient apiClient = new ApiClient(
new LKUConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.LKUConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('LKU', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for LKU
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
LONConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LONConnectorSettings;
ApiClient apiClient = new ApiClient(
new LONConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.LONConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('LON', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for LON
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
LPBConnectorSettings
Settings for initializing ApiClient for LPB
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
LaBanquePostaleConnectorSettings
Settings for initializing ApiClient for La Banque Postale (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
LaBanquePostaleV2ConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LaBanquePostaleV2ConnectorSettings;
ApiClient apiClient = new ApiClient(
new LaBanquePostaleV2ConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.LaBanquePostaleV2ConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('LaBanquePostaleV2', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for LaBanquePostaleV2
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
LaanAndSparBankDKConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LaanAndSparBankDKConnectorSettings;
ApiClient apiClient = new ApiClient(
new LaanAndSparBankDKConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Lån & Spar Bank DK.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
LaanAndSparBankSEConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LaanAndSparBankSEConnectorSettings;
ApiClient apiClient = new ApiClient(
new LaanAndSparBankSEConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Lån & Spar Bank SE.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
LaboralKutxaConnectorSettings
Settings for initializing ApiClient for [LaboralKutxa]
Credentials can be obtained at Laboral Kutxa Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
LaegernesBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
LandbobankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LandbobankConnectorSettings;
ApiClient apiClient = new ApiClient(
new LandbobankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Landbobank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
LandbobankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LandbobankConnectorSettings;
ApiClient apiClient = new ApiClient(
new LandbobankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Landbobank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
LandkredittBankBusinessConnectorSettings
Settings for initializing ApiClient for Landkreditt Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
LandkredittBankConnectorSettings
Settings for initializing ApiClient for Landkreditt Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
LandsbankinnBusinessConnectorSettings
Settings for initializing ApiClient for Landsbankinn Business (Iceland).
Credentials can obtained at https://psd2.landsbanki.is/docs/berlingroup/landsbankinn_sandbox.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
LandsbankinnConnectorSettings
Settings for initializing ApiClient for Landsbankinn (Iceland).
Credentials can obtained at https://psd2.landsbanki.is/docs/berlingroup/landsbankinn_sandbox.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
LangaaSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LangaaSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new LangaaSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Langå Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
LansforsakringarBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LansforsakringarConnectorSettings;
ApiClient apiClient = new ApiClient(
new LansforsakringarConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.sandbox(true));
Settings for initializing ApiClient for Lansforsakringar.
Credentials can obtained at Lansforsakringar Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from ICA Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from ICA Developer Portal) |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Optional in sandbox, but required in production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Optional in sandbox, but required in production. |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. Required for production. |
LansforsakringarConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LansforsakringarConnectorSettings;
ApiClient apiClient = new ApiClient(
new LansforsakringarConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.sandbox(true));
Settings for initializing ApiClient for Lansforsakringar.
Credentials can obtained at Lansforsakringar Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from ICA Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from ICA Developer Portal) |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Optional in sandbox, but required in production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Optional in sandbox, but required in production. |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. Required for production. |
LazardFreresBanqueConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LazardFreresBanqueConnectorSettings;
ApiClient apiClient = new ApiClient(
new LazardFreresBanqueConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.LazardFreresBanqueConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('LazardFreresBanque', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Lazard Freres Banque
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
LillesandsSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Lillesands Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
LillesandsSparebankConnectorSettings
Settings for initializing ApiClient for Lillesands Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
LillestroemSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LillestroemSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new LillestroemSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Lillestrøm Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
LollandsBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
LuminorConnectorSettings
Settings for initializing ApiClient for Luminor Estonia, Luminor Latvia, Luminor Lithuania.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
false |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required for production, not used in sandbox. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required for production, not used in sandbox. |
clientId |
string |
true |
API client ID |
clientSecret |
string |
false |
API client secret. Required for sandbox, not used in production |
companyName |
string |
true |
Company name displayed to the PSU during authorization |
LunarConnectorSettings
Settings for initializing ApiClient for Lunar (Denmark).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
LusterSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Luster Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
LusterSparebankConnectorSettings
Settings for initializing ApiClient for Luster Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
MBHBankConnectorSettings
Settings for initializing ApiClient for MBH Bank(Hungary)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
MBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new MBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.business(false)
.country("your-country")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.signFingerprint("your-sign-fingerprint")
.signCertUrl("your-sign-cert-url")
.tppId("your-tpp-id")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.MBankConnectorSettings(
business=False,
country="your-country",
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
sign_fingerprint="your-sign-fingerprint",
sign_cert_url="your-sign-cert-url",
tpp_idl="your-tpp-id"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
business: false,
country: "your-country",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
signFingerprint: "your-sign-fingerprint",
signCertUrl: "your-sign-cert-url",
tppId: "your-tpp-id"
}
const apiClient = new enablebanking.ApiClient('MBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for mBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded without padding). |
signCertUrl |
string |
true |
none |
tppId |
string |
false |
none |
MPSConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MPSConnectorSettings;
ApiClient apiClient = new ApiClient(
new MPSConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.business(false)
.sandbox(true);
import enablebanking
connector_settings = enablebanking.MPSConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
business=False
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
business: false
}
const apiClient = new enablebanking.ApiClient('MPS', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for MPS
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
MagNetBankConnectorSettings
Settings for initializing ApiClient for MagNet Magyar Közösségi Bank(Hungary).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
clientId |
string |
true |
API client ID. |
MajBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
MarginalenBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MarkerSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new MarkerSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Marker Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
signCertPath |
string |
false |
Path to QWAC certificate in PEM format. |
signKeyPath |
string |
false |
Path to QWAC certificate private key in PEM format. |
signPubKeySerial |
string |
false |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
false |
Client key obtained from the Marginalen Bank developer portal. Used only in the sandbox |
clientSecret |
string |
false |
Client secret obtained from the Marginalen Bank developer portal. Used only in the sandbox |
MaritimeOgMerchantBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MaritimeOgMerchantBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new MaritimeOgMerchantBankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Maritime og Merchant Bank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
MedicinosBankasConnectorSettings
Settings for initializing ApiClient for [Medicinos Bankas] (https://www.medbank.lt/)
Credentials can be obtained at Medicinos Bankas Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
postbackUri |
string |
true |
URL where the user is going to be redirected using the POST method |
MediobancaPremierConnectorSettings
Settings for initializing ApiClient for MediobancaPremier
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
MerckfinckConnectorSettings
Settings for initializing ApiClient for Merck Finck Privatbankiers AG (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
MerkurAndelskasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
MiddelfartSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MiddelfartSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new MiddelfartSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Middelfart Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
MilleisBanqueConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MilleisBanqueConnectorSettings;
ApiClient apiClient = new ApiClient(
new MilleisBanqueConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.MilleisBanqueConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('MilleisBanque', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Milleis Banque
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
MillenniumBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MillenniumBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new ST1FinanceConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.signFingerprint("qseal-cert-fingerprint")
.sandbox(true));
import enablebanking
connector_settings = enablebanking.MillenniumBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
sign_finger_print="qseal-cert-fingerprint"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
signFingerprint: "qseal-cert-fingerprint",
}
const apiClient = new enablebanking.ApiClient('MillenniumBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for MillenniumBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded with padding). |
MockASPSPBusinessConnectorSettings
Settings for initializing ApiClient for MockASPSP Business Connector. - country
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
MockASPSPConnectorSettings
Settings for initializing ApiClient for MockASPSP Connector. - country
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
MonabanqConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MonabanqConnectorSettings;
ApiClient apiClient = new ApiClient(
new MonabanqConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.MonabanqConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('Monabanq', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Monabanq
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
MonsBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
MooneyConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MooneyConnectorSettings;
ApiClient apiClient = new ApiClient(
new MooneyConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.MooneyConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('Mooney', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Mooney
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
MorrowConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate) |
language |
string |
true |
User interface desired language |
clientId |
string |
true |
none |
xApiKey |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
MufgBankConnectorSettings
Settings for initializing ApiClient for MUFG Bank (Europe) N. V. Germany Branch (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
MyBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.MyBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new MyBankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for MyBank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
N26ConnectorSettings
Settings for initializing ApiClient for N26 (Germany and Spain).
Credentials are not required for this bank.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID |
certPath |
string |
true |
The path to the cert for authorization |
keyPath |
string |
true |
The path to the key correcponding to the certificate |
NBGConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NBGConnectorSettings;
ApiClient apiClient = new ApiClient(
new NBGConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandboxId("your-sandbox-id")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.NBGConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sandbox_id="your-sandbox-id"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
sandboxId: "your-sandbox-id"
}
const apiClient = new enablebanking.ApiClient('NBG', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for NBG
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
sandboxId |
string |
true |
none |
NEXIConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NEXIConnectorSettings;
ApiClient apiClient = new ApiClient(
new NEXIConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.NEXIConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('NEXI', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for NEXI
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
NLBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NLBConnectorSettings;
ApiClient apiClient = new ApiClient(
new NLBConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.NLBConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('NLB', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for NLB
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
NWBBankConnectorSettings
Settings for initializing ApiClient for NWBBank (Poland).
Credentials can obtained at NWBBank API Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from the API Portal) |
apiSecret |
string |
true |
API secret (obtained from the API Portal) |
apiKey |
string |
true |
API key (obtained from the API Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
NaeringsbankenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NaeringsbankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new NaeringsbankenConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Næringsbanken.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
NagelmackersConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NagelmackersConnectorSettings;
ApiClient apiClient = new ApiClient(
new NagelmackersConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.NagelmackersConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Nagelmackers', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Nagelmackers
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
NationalBankConnectorSettings
Settings for initializing ApiClient for National-Bank AG (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
NeuflizeOBCConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NeuflizeOBCConnectorSettings;
ApiClient apiClient = new ApiClient(
new NeuflizeOBCConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.NeuflizeOBCConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('NeuflizeOBC', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Neuflize OBC
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
NidarosSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NidarosSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new NidarosSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Nidaros Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
NoerreNebelSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NoerreNebelSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new NoerreNebelSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Nørre Nebel Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
NordeaBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordeaBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordeaBusinessConnectorSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.signKeyPath("path/to/qseal-key.pem")
.country("FI")
.language("fi")
.redirectUri("https://your-redirect-uri")
.sandbox(true));
Settings for initializing ApiClient for Nordea (all countries).
Credentials can obtained at Nordea Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. If country is |
clientId |
string |
true |
API client ID (obtained from Nordea Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Nordea Developer Portal) |
signKeyPath |
string |
true |
Path to Qseal private key in PEM format. |
NordeaCommercialCardsConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordeaCommercialCardsSettings;
ApiClient apiClient = new ApiClient(
new NordeaCommercialCardsSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.signKeyPath("path/to/qseal-key.pem")
.country("FI")
.language("fi")
.redirectUri("https://your-redirect-uri")
.sandbox(true));
Settings for initializing ApiClient for Nordea Commercial Cards (all countries).
Credentials can obtained at Nordea Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. If country is |
clientId |
string |
true |
API client ID (obtained from Nordea Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Nordea Developer Portal) |
signKeyPath |
string |
true |
Path to Qseal private key in PEM format. |
NordeaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordeaConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordeaConnectorSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.signKeyPath("path/to/qseal-key.pem")
.country("FI")
.language("fi")
.redirectUri("https://your-redirect-uri")
.sandbox(true));
Settings for initializing ApiClient for Nordea (all countries).
Credentials can obtained at Nordea Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. If country is |
clientId |
string |
true |
API client ID (obtained from Nordea Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Nordea Developer Portal) |
signKeyPath |
string |
true |
Path to Qseal private key in PEM format. |
NordeaCorporateConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordeaBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordeaBusinessConnectorSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.signKeyPath("path/to/qseal-key.pem")
.country("FI")
.language("fi")
.redirectUri("https://your-redirect-uri")
.sandbox(true));
Settings for initializing ApiClient for Nordea Corporate (all countries).
Credentials can obtained at Nordea Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. If country is |
clientId |
string |
true |
API client ID (obtained from Nordea Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Nordea Developer Portal) |
signKeyPath |
string |
true |
Path to Qseal private key in PEM format. |
NordeaDirectConnectorSettings
Settings for initializing ApiClient for Nordea Direct
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
NordfynsBankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordfynsBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordfynsBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for NordfynsBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
NordfynsBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordfynsBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordfynsBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for NordfynsBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
NordicCorporateBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordicCorporateBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordicCorporateBankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Nordic Corporate Bank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
NordoyarSparikassiConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordoyarSparikassiConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordoyarSparikassiConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Nordoyar Sparikassi.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
NorisbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankNorisConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankNorisConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankNorisConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankNoris', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Norisbank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
NovaKBMConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NovaKBMConnectorSettings;
ApiClient apiClient = new ApiClient(
new NovaKBMConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.NovaKBMConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('NovaKBM', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for NovaKBM
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
NovoBancoConnectorSettings
Settings for initializing ApiClient for NOVO BANCO, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
NovoBancoDosAcoresConnectorSettings
Settings for initializing ApiClient for NOVO BANCO DOS ACORES, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
NykreditBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
OBOSBankenBusinessConnectorSettings
Settings for initializing ApiClient for OBOS Banken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
OBOSBankenConnectorSettings
Settings for initializing ApiClient for OBOS Banken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
OKQ8BankConnectorSettings
Settings for initializing ApiClient for OKQ8 Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
OPBalticsEEConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OPBalticsEEConnectorSettings;
ApiClient apiClient = new ApiClient(
new OPBalticsEEConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.apiKey("your-api-key")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.OPBalticsEEConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
api_key="your-api-key"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
apiKey: "your-api-key"
}
const apiClient = new enablebanking.ApiClient('OPBalticsEE', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for OPBaltics
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
apiKey |
string |
true |
API client key. |
OPBalticsLTConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OPBalticsLTConnectorSettings;
ApiClient apiClient = new ApiClient(
new OPBalticsLTConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.apiKey("your-api-key")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.OPBalticsLTConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
api_key="your-api-key"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
apiKey: "your-api-key"
}
const apiClient = new enablebanking.ApiClient('OPBalticsLT', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for OPBaltics
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
apiKey |
string |
true |
API client key. |
OPBalticsLVConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OPBalticsLVConnectorSettings;
ApiClient apiClient = new ApiClient(
new OPBalticsLVConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.apiKey("your-api-key")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.OPBalticsLVConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
api_key="your-api-key"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
apiKey: "your-api-key"
}
const apiClient = new enablebanking.ApiClient('OPBalticsLV', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for OPBalticsLV
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
apiKey |
string |
true |
API client key. |
OPConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
apiKey |
string |
true |
API key |
clientId |
string |
true |
Client id |
clientSecret |
string |
true |
Client secret |
certPath |
string |
true |
Path to a QWAC |
keyPath |
string |
true |
Path to a QWAC private key |
signKeyPath |
string |
true |
Path to a public QSEAL certificate |
signPubKeySerial |
string |
true |
Public QSEAL certificate serial number |
OTPBankConnectorSettings
Settings for initializing ApiClient for OTP Bank (France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
tppId |
string |
true |
The Id of TPP. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
OberbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OberbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new OberbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.OberbankConnectorSettings(
country="your-country",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Oberbank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Oberbank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
OddobhfBankConnectorSettings
Settings for initializing ApiClient for ODDO BHF-Bank AG (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
OerlandSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OerlandSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new OerlandSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Ørland Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
OfotenSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OfotenSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new OfotenSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Ofoten Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
OikosAndelskasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OikosAndelskasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new OikosAndelskasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Oikos Andelskasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
OmaSpConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Samlink Developer Portal) |
clientSecret |
string |
false |
API client secret (sandbox client secret obtained from Samlink Developer Portal, not used in production) |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. Required for production, not used in sandbox. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath (not used in sandbox, required for production) |
signCertPath |
string |
false |
Path to QSEAL public certificate in PEM format. Required for production, not used in sandbox. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Samlink Developer Portal, "Ocp-Apim-Subscription-Key" in sandbox, "Api Key" in production) |
OpenBankConnectorSettings
Settings for initializing ApiClient for [OpenBank]
Credentials can be obtained at OpenBank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
OptimabankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OptimabankConnectorSettings;
ApiClient apiClient = new ApiClient(
new OptimabankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.OptimabankConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('Optimabank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Optimabank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
OrangeBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.OrangeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new OrangeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.OrangeBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('OrangeBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Orange Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
PBZConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PBZConnectorSettings;
ApiClient apiClient = new ApiClient(
new PBZConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.PBZConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('PBZ', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for PBZ
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
PHVConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PHVConnectorSettings;
ApiClient apiClient = new ApiClient(
new PHVConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.PHVConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('PHV', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for PHV
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
PKOConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PKOConnectorSettings;
ApiClient apiClient = new ApiClient(
new PKOConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-key.pem")
.signPubKeySerial("qseal-cert-serial")
.signFingerprint("qseal-cert-fingerprint")
.signCertUrl("qseal-cert-public-url")
.tppId("your-tpp-id")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
none |
signFingerprint |
string |
true |
none |
signCertUrl |
string |
true |
none |
tppId |
string |
true |
none |
POPPankkiConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Samlink Developer Portal) |
clientSecret |
string |
false |
API client secret (sandbox client secret obtained from Samlink Developer Portal, not used in production) |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. Required for production, not used in sandbox. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath (not used in sandbox, required for production) |
signCertPath |
string |
false |
Path to QSEAL public certificate in PEM format. Required for production, not used in sandbox. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Samlink Developer Portal, "Ocp-Apim-Subscription-Key" in sandbox, "Api Key" in production) |
POSTEPAYConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.POSTEPAYConnectorSettings;
ApiClient apiClient = new ApiClient(
new POSTEPAYConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.business(false)
.sandbox(true);
import enablebanking
connector_settings = enablebanking.POSTEPAYConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
business=False
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
business: false
}
const apiClient = new enablebanking.ApiClient('POSTEPAY', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for POSTEPAY
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
ParetoBankBusinessConnectorSettings
Settings for initializing ApiClient for Pareto Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
ParetoBankConnectorSettings
Settings for initializing ApiClient for Pareto Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
PayPalBusinessConnectorSettings
Settings for initializing ApiClient for PayPal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
User country code. |
clientId |
string |
true |
API client ID. Obtained during onboarding. |
clientSecret |
string |
true |
API client secret. Obtained during onboarding. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
PayPalConnectorSettings
Settings for initializing ApiClient for PayPal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
User country code. |
clientId |
string |
true |
API client ID. Obtained during onboarding. |
clientSecret |
string |
true |
API client secret. Obtained during onboarding. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
PayseraConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PayseraConnectorSettings;
ApiClient apiClient = new ApiClient(
new PayseraConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.language("your-language")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.PayseraConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
language="your-language"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
language: "your-language"
}
const apiClient = new enablebanking.ApiClient('Paysera', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Paysera
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
language |
string |
false |
Language of authentication user interface. |
PekaoConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PekaoConnectorSettings;
ApiClient apiClient = new ApiClient(
new PekaoConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-key.pem")
.signPubKeySerial("qseal-cert-serial")
.signFingerprint("qseal-cert-fingerprint")
.signCertUrl("qseal-cert-public-url")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
none |
clientSecret |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
none |
signFingerprint |
string |
true |
none |
signCertUrl |
string |
true |
none |
serverSignCertPath |
string |
false |
Path/URI for a server public pem certificate which is used for verification of a signature in a response received from a server. |
serverSignCertThumbprint |
string |
false |
Thimbprint of a server signature pem certificate provided in the |
PenSamConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
PermanentTSBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PermanentTSBConnectorSettings;
ApiClient apiClient = new ApiClient(
new PermanentTSBConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.PermanentTSBConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('PermanentTSB', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for PermanentTSB
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
PiraeusBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PiraeusBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new PiraeusBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.PiraeusBankConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('PiraeusBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for PiraeusBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
PleoConnectorSettings
Settings for initializing ApiClient for Pleo.
Credentials can obtained at https://priora.saltedge.com/docs/berlingroup/pleo.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
PortuguesGestaoConnectorSettings
Settings for initializing ApiClient for BANCO PORTUGUES DE GESTAO, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
PostLUConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
PostbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DeutscheBankPostbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new DeutscheBankPostbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.DeutscheBankPostbankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('DeutscheBankPostbank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Postbank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
PostovabankaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PostovabankaConnectorSettings;
ApiClient apiClient = new ApiClient(
new PostovabankaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.serverSignCertPath("your-server-sign-cert-path")
.brand("your-brand")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.PostovabankaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
server_sign_cert_path="your-server-sign-cert-path",
brand="your-brand"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
serverSignCertPath: "your-server-sign-cert-path",
brand: "your-brand"
}
const apiClient = new enablebanking.ApiClient('Postovabanka', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Postovabanka
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
serverSignCertPath |
string |
true |
none |
brand |
string |
true |
Denotes the bank where the account is held. |
ProCreditBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ProCreditBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new ProCreditBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ProCreditBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('ProCreditBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for ProCreditBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from the bank Developer Portal, "Ocp-Apim-Subscription-Key" in sandbox) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
QontoConnectorSettings
Settings for initializing ApiClient for Qonto (Online bank based in France).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
RBSInternationalConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RBSInternationalConnectorSettings;
ApiClient apiClient = new ApiClient(
new RBSInternationalConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RBSInternationalConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('RBSInternational', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for RBSInternational
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
RabobankConnectorSettings
Settings for initializing ApiClient for Rabobank (NL)
Credentials can obtained at Rabobank (NL) Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Rabobank Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Rabobank Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
RaiffeisenBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RaiffeisenBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new RaiffeisenBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandboxClientSecret("sanbox-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RaiffeisenBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sandbox_client_secret="sandbox-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
sandbox_client_secret: "sandbox-client-secret"
}
const apiClient = new enablebanking.ApiClient('RaiffeisenBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for RaiffeisenBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
sandboxClientSecret |
string |
true |
Static clientSecret used in sandbox |
RaiffeisenCZConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RaiffeisenCZConnectorSettings;
ApiClient apiClient = new ApiClient(
new RaiffeisenCZConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.tppName("your-tpp-name")
.tppId("your-tpp-id")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RaiffeisenCZConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
tpp_name="your-tpp-name",
tpp_id="your-tpp-id"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
tppName: "your-tpp-name",
tppId: "your-tpp-id"
}
const apiClient = new enablebanking.ApiClient('RaiffeisenCZ', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for RaiffeisenCZ
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
tppName |
string |
true |
The name of the original TPP that created the request. Eg. ‘Star corporation, a.s.’. |
tppId |
string |
true |
The identification (licence number) of the original TPP that created the request. Eg. ‘CZ013574-15’. |
RaiffeisenHRConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RaiffeisenHRConnectorSettings;
ApiClient apiClient = new ApiClient(
new RaiffeisenHRConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RaiffeisenHRConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('RaiffeisenHR', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for RaiffeisenHR
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
RaiffeisenHUConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RaiffeisenHUConnectorSettings;
ApiClient apiClient = new ApiClient(
new RaiffeisenHUConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RaiffeisenHUConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('RaiffeisenHU', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for RaiffeisenHU
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
RaiffeisenITConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RaiffeisenITConnectorSettings;
ApiClient apiClient = new ApiClient(
new RaiffeisenITConnectorSettings()
.brand("brand")
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("qseal-cert-public-serial-here")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RaiffeisenITConnectorSettings(
brand="brand"
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
brand: "brand",
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "qseal-cert-public-serial-here"
}
const apiClient = new enablebanking.ApiClient('RaiffeisenIT', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for RaiffeisenIT
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
brand |
string |
true |
Denotes the bank where the account is held. |
RaiffeisenLUConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
RaiffeisenSKConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RaiffeisenSKConnectorSettings;
ApiClient apiClient = new ApiClient(
new RaiffeisenSKConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RaiffeisenSKConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('RaiffeisenSK', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for RaiffeisenSK
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
RegioBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RegioBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new RegioBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.RegioBankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('RegioBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Regio Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
Renta4BancoConnectorSettings
Settings for initializing ApiClient for [Renta4Banco]
Credentials can be obtained at Renta 4 Banco Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
ResursConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ResursConnectorSettings;
ApiClient apiClient = new ApiClient(
new ResursConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.xApiKey("put-your-x-api-key-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
true |
User interface desired language |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
xApiKey |
string |
true |
API client key (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. Test certificate (for accessing sandbox environment) can be downloaded from Open Banking Market. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
RevolutBusinessConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country |
clientId |
string |
true |
API client ID (obtained from Revolut Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
jwsTan |
string |
false |
Value to be used for JWS-TAN header. Should be set to a domain where Client's JWKS is hosted |
RevolutConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country |
clientId |
string |
true |
API client ID (obtained from Revolut Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
jwsTan |
string |
false |
Value to be used for JWS-TAN header. Should be set to a domain where Client's JWKS is hosted |
RiseFlemloeseSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RiseFlemloeseSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new RiseFlemloeseSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Rise Flemløse Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
RockerConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RockerConnectorSettings;
ApiClient apiClient = new ApiClient(
new RockerConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.apiKey("your-api-key")
.sandbox(true));
import enablebanking
connector_settings = enablebanking.RockerConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
api_key="your-api-key"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
apiKey: "your-api-key"
}
const apiClient = new enablebanking.ApiClient('Rocker', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Rocker
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
apiKey |
string |
true |
API client key. |
RoendeSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.RoendeSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new RoendeSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Rønde Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SBABConnectorSettings
Settings for initializing ApiClient for SBAB.
Credentials can obtained at SBAB Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required for sandbox and production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required for production. |
SDCConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SDCConnectorSettings;
ApiClient apiClient = new ApiClient(
new SDCConnectorSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for SDC (sandbox only version).
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possible values: |
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
false |
Path to QWAC certificate in PEM format. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SEBBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBConnectorSettings;
ApiClient apiClient = new ApiClient(
new SPankkiConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.clientId("put-your-client-id-here")
.country("EE")
.sandbox(true));
Settings for initializing ApiClient for SEB Estonia, SEB Latvia and SEB Lithuania.
Credentials can obtained at SEB Developer Portal for Baltic countries.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID (obtained from SEB Developer Portal) |
SEBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBConnectorSettings;
ApiClient apiClient = new ApiClient(
new SPankkiConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.clientId("put-your-client-id-here")
.country("EE")
.sandbox(true));
Settings for initializing ApiClient for SEB Estonia, SEB Latvia and SEB Lithuania.
Credentials can obtained at SEB Developer Portal for Baltic countries.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID (obtained from SEB Developer Portal) |
SEBDenmarkConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SEBKortBankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBKortBankBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new SEBKortBankBusinessConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.brand("brand")
.sandbox(true));
Settings for initializing ApiClient for SEBKortBankBusiness.
Credentials can obtained at SEB Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SEB to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SEB in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
brand |
string |
true |
Bank brand. Allowed values are |
SEBKortBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBKortBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SEBKortBankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.brand("brand")
.sandbox(true));
Settings for initializing ApiClient for SEB Sweden.
Credentials can obtained at SEB Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SEB to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SEB in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
brand |
string |
true |
Bank brand. Allowed values are |
SEBSwedenBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBSwedenBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new SEBSwedenBusinessConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.language("sv")
.sandbox(true));
Settings for initializing ApiClient for SEB Sweden (for business users).
Credentials can obtained at SEB Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from SEB Developer Portal), corporate entry to be used in production |
clientSecret |
string |
true |
API client secret (obtained from SEB Developer Portal), corporate entry to be used in production |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
language |
string |
false |
Language of authentication user interface. |
SEBSwedenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBSwedenConnectorSettings;
ApiClient apiClient = new ApiClient(
new SEBSwedenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.language("sv")
.sandbox(true));
Settings for initializing ApiClient for SEB Sweden.
Credentials can obtained at SEB Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from SEB Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from SEB Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
language |
string |
false |
Language of authentication user interface. |
SKBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SKBConnectorSettings;
ApiClient apiClient = new ApiClient(
new SKBConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SKBConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('SKB', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for SKB
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SNSBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SNSBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SNSBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SNSBankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('SNSBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for SNS Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
SPankkiConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SPankkiConnectorSettings;
ApiClient apiClient = new ApiClient(
new SPankkiConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.xApiKey("put-your-x-api-key-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.sandbox(true));
Settings for initializing ApiClient for S-Pankki (Finland).
Credentials can obtained at Open Banking Market
operated by Crosskey Banking Solutions.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
language |
string |
true |
User interface desired language |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
xApiKey |
string |
true |
API client key (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
ST1FinanceConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ST1FinanceConnectorSettings;
ApiClient apiClient = new ApiClient(
new ST1FinanceConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.apiKey("your-api-key")
.sandbox(true));
import enablebanking
connector_settings = enablebanking.ST1FinanceConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial",
api_key="your-api-key"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial",
apiKey: "your-api-key"
}
const apiClient = new enablebanking.ApiClient('ST1Finance', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for ST1Finance
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
apiKey |
string |
true |
API client key. |
SaastopankkiConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (sandbox client id obtained from Samlink Developer Portal, for production equals organizationIdentifier from eIDAS QSEAL certificate subject) |
clientSecret |
string |
false |
API client secret (sandbox client secret obtained from Samlink Developer Portal, not used in production) |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. Required for production, not used in sandbox. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath (not used in sandbox, required for production) |
signCertPath |
string |
false |
Path to QSEAL public certificate in PEM format. Required for production, not used in sandbox. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Samlink Developer Portal, "Ocp-Apim-Subscription-Key" in sandbox, "Api Key" in production) |
SabadellConnectorSettings
Settings for initializing ApiClient for [Banco de Sabadell] (https://www.bancsabadell.com)
Credentials can be obtained at Banco de Sabadell Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
SafraBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SantanderBankPolskaConnectorSettings
Settings for initializing ApiClient for Santander Bank Polska
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
client ID |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
none |
signFingerprint |
string |
false |
none |
signCertUrl |
string |
false |
none |
SantanderConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SantanderConnectorSettings;
ApiClient apiClient = new ApiClient(
new SantanderConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SantanderConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Santander', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Santander
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SantanderConsumerBankNorwayConnectorSettings
Settings for initializing ApiClient for Santander Consumer Bank (NO)
Credentials can obtained at Santander Consumer Bank (NO) Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Santander Consumer Bank (NO) Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Santander Consumer Bank (NO) Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
SantanderLUConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SantanderTottaConnectorSettings
Settings for initializing ApiClient for BANCO SANTANDER TOTTA, SA (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
SaxoConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SaxoConnectorSettings;
ApiClient apiClient = new ApiClient(
new SaxoConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SaxoConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret"
}
const apiClient = new enablebanking.ApiClient('Saxo', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Saxo
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank's country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
SbankenBusinessConnectorSettings
Settings for initializing ApiClient for Sbanken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SbankenConnectorSettings
Settings for initializing ApiClient for Sbanken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SebLUConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SebNOConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SelbuSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SelbuSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SelbuSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Selbu Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SelfBankConnectorSettings
Settings for initializing ApiClient for [SelfBank]
Credentials can be obtained at SelfBank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
SiauliuBankas2ConnectorSettings
Settings for initializing ApiClient for [Šiaulių Bankas] (https://sb.lt/)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
SiauliuBankasConnectorSettings
Settings for initializing ApiClient for [Šiaulių Bankas] (https://sb.lt/)
Credentials can be obtained at Šiaulių Bankas Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
postbackUri |
string |
false |
URL to receive a post request with list of accounts in the request body |
SignetBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
xApiKey |
string |
true |
API client key (obtained in developer portal) |
country |
string |
true |
Bank`s country |
certPath |
string |
false |
Path to QWAC certificate in PEM format |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SkandiaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SkandiaConnectorSettings;
ApiClient apiClient = new ApiClient(
new SkandiaConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.sandbox(true));
Settings for initializing ApiClient for Skandia.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
SkjernBankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SkjernBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SkjernBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for SkjernBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
SkjernBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SkjernBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SkjernBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for SkjernBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
SkudenesAakraSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Skudenes & Aakra Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SkudenesAakraSparebankConnectorSettings
Settings for initializing ApiClient for Skudenes & Aakra Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SlovenskaSporitelnaConnectorSettings
Settings for Slovenska Sporitelna (Bank in Slovak Republic)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
apiKey |
string |
true |
API key (obtained from the API Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SocieteGeneraleEntreprisesConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SocieteGeneraleEntreprisesConnectorSettings;
ApiClient apiClient = new ApiClient(
new SocieteGeneraleEntreprisesConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SocieteGeneraleEntreprisesConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('SocieteGeneraleEntreprises', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Société Générale Entreprises
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
SocieteGeneraleLUConnectorSettings
Settings for initializing ApiClient for Societe Generale bank in Luxembourg
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID |
clientSecret |
string |
true |
API client secret |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SocieteGeneraleParticuliersConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SocieteGeneraleParticuliersConnectorSettings;
ApiClient apiClient = new ApiClient(
new SocieteGeneraleParticuliersConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SocieteGeneraleParticuliersConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('SocieteGeneraleParticuliers', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Société Générale Particuliers
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
SocieteGeneraleProfessionnelsConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SocieteGeneraleProfessionnelsConnectorSettings;
ApiClient apiClient = new ApiClient(
new SocieteGeneraleProfessionnelsConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SocieteGeneraleProfessionnelsConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('SocieteGeneraleProfessionnels', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Société Générale Professionnels
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
SoenderhaaHoerstedSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SoenderhaaHoerstedSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new SoenderhaaHoerstedSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sønderhå - Hørsted Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SogneOgGreipstadSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Sogne og Greipstad Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SogneOgGreipstadSparebankConnectorSettings
Settings for initializing ApiClient for Sogne og Greipstad Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SolarisbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SolarisbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SolarisbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SolarisbankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('Solarisbank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Solarisbank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SparNordBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SparbankenSydConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparbankenSydConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparbankenSydConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparbanken Syd.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SpareBank1BusinessConnectorSettings
Settings for initializing ApiClient for Sparebank 1
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
brand |
string |
true |
Denotes the bank where the account is held. |
SpareBank1ConnectorSettings
Settings for initializing ApiClient for Sparebank 1
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
brand |
string |
true |
Denotes the bank where the account is held. |
Sparebank68NordConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.Sparebank68NordConnectorSettings;
ApiClient apiClient = new ApiClient(
new Sparebank68NordConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparebank 68 Nord.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SparebankenDINConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparebankenDINConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparebankenDINConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparebanken DIN.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SparebankenMoreBusinessConnectorSettings
Settings for initializing ApiClient for Sparebanken Møre
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenMoreConnectorSettings
Settings for initializing ApiClient for Sparebanken Møre
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenOstBusinessConnectorSettings
Settings for initializing ApiClient for Sparebanken Øst
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenOstConnectorSettings
Settings for initializing ApiClient for Sparebanken Øst
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenSognOgFjordaneBusinessConnectorSettings
Settings for initializing ApiClient for Sparebanken Sogn og Fjordane
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenSognOgFjordaneConnectorSettings
Settings for initializing ApiClient for Sparebanken Sogn og Fjordane
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenSorBusinessConnectorSettings
Settings for initializing ApiClient for Sparebanken Sør
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenSorConnectorSettings
Settings for initializing ApiClient for Sparebanken Sør
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparebankenVestConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparebankenVestConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparebankenVestConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SparebankenVestConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('SparebankenVest', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Sparebanken Vest
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SparekasseBredebroConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekasseBredebroConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekasseBredebroConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparekasse Bredebro.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SparekassenBallingConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenBallingConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenBallingConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparekassen Balling.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SparekassenDanmarkConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenDanmarkConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenDanmarkConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparkassen Danmark.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SparekassenDjurslandConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenDjurslandConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenDjurslandConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparekassen Djursland.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SparekassenKronjyllandConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenKronjyllandConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenKronjyllandConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparekassen Kronjylland.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SparekassenSjaellandFynBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenSjaellandFynConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenSjaellandFynConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Sparekassen Sjælland-Fyn
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
SparekassenSjaellandFynConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenSjaellandFynConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenSjaellandFynConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Sparekassen Sjælland-Fyn
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
SparekassenThyConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenThyConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenThyConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Sparekassen Thy.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SpareskillingsbankenBusinessConnectorSettings
Settings for initializing ApiClient for Spareskillingsbanken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SpareskillingsbankenConnectorSettings
Settings for initializing ApiClient for Spareskillingsbanken
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SparkasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparkasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparkasseConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true)
.brand("Berliner Sparkasse");
import enablebanking
connector_settings = enablebanking.SparkasseConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
brand="Berliner Sparkasse"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
brand: "Name of Sparkasse cooperating Savings Bank (e.g. Berliner Sparkasse)"
}
const apiClient = new enablebanking.ApiClient('Sparkasse', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Sparkasse
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
brand |
string |
true |
Name of Sparkasse cooperating Savings Bank (e.g. Berliner Sparkasse). |
SparkasseSIConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparkasseSIConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparkasseSIConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SparkasseSIConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('SparkasseSI', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for SparkasseSI
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SpuerkeessConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
StadilSparekasseConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.StadilSparekasseConnectorSettings;
ApiClient apiClient = new ApiClient(
new StadilSparekasseConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Stadil Sparekasse.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
StadsbygdSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.StadsbygdSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new StadsbygdSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Stadsbygd Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
StorebrandConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.StorebrandConnectorSettings;
ApiClient apiClient = new ApiClient(
new StorebrandConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Storebrand.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SuduroyarSparikassiConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SuduroyarSparikassiConnectorSettings;
ApiClient apiClient = new ApiClient(
new SuduroyarSparikassiConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Suðuroyar Sparikassi.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SumUpConnectorSettings
Settings for initializing ApiClient for Sum Up
Credentials can obtained at Sum Up Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Sum Up Developer Portal) |
clientSecret |
string |
true |
API api key (obtained from Sum Up Developer Portal) |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
language |
string |
false |
Language of authentication user interface. The default language is |
SurnadalSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SurnadalSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SurnadalSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Surnadal Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SveaBankBusinessConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SveaBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SwedbankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SwedbankBusinessConnectorSettings;
ApiClient apiClient = new ApiClient(
new SwedbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.country("SE")
.redirectUri("scheme://uri/to/redirect/after/payment")
.sandbox(true));
Settings for initializing ApiClient for access to business accounts in Swedbank (Sweden and Baltic coutries).
Credentials can obtained at Swedbank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required in production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required in production. |
signKeyPath |
string |
false |
Path to QSeal certificate private key in PEM format. Required for PIS functionality in production. |
signPubKeySerial |
string |
false |
Serial Number of the TPPs certificate declared in Developer Portal and CA name. |
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
brand |
string |
false |
Name of Swedbank cooperating Savings Bank (e.g. Bergslagens Sparbank). If not set defaults to Swedbank. |
SwedbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SwedbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SwedbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.country("SE")
.redirectUri("scheme://uri/to/redirect/after/payment")
.sandbox(true));
Settings for initializing ApiClient for Swedbank (Sweden and Baltic coutries).
Credentials can obtained at Swedbank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
language |
string |
false |
Language of authentication user interface. |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required in production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required in production. |
signKeyPath |
string |
false |
Path to QSeal certificate private key in PEM format. Required for PIS functionality in production. |
signPubKeySerial |
string |
false |
Serial Number of the TPPs certificate declared in Developer Portal and CA name. |
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
brand |
string |
false |
Name of Swedbank cooperating Savings Bank (e.g. Bergslagens Sparbank). If not set defaults to Swedbank. |
SwedbankDenmarkConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SwedbankNorgeBusinessConnectorSettings
Settings for initializing ApiClient for Swedbank Norge
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SwedbankNorgeConnectorSettings
Settings for initializing ApiClient for Swedbank Norge
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SweepBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SweepBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SweepBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.hmac("your-hmac")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.SweepBankConnectorSettings(
country="your-country",
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
hmac="your-hmac"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
hmac: "your-hmac"
}
const apiClient = new enablebanking.ApiClient('SweepBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for SweepBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
hmac |
string |
true |
none |
SwissquoteBankEuropeConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SydbankBusinessConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SydbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SydbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Sydbank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
SydbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SydbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SydbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Sydbank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
SydbankFlensburgConnectorSettings
Settings for initializing ApiClient for Sydbank A/S Filiale Flensburg (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
TakarekbankConnectorSettings
Settings for initializing ApiClient for Takarebank(Hungary)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
TargoBankConnectorSettings
Settings for initializing ApiClient for TARGOBANK AG (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
TargobankESConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.TargobankESConnectorSettings;
ApiClient apiClient = new ApiClient(
new TargobankESConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.TargobankESConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('TargobankES', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for TargobankES
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
TatrabankaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.TatrabankaConnectorSettings;
ApiClient apiClient = new ApiClient(
new TatrabankaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.TatrabankaConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
cert_path="your-cert-path",
key_path="your-key-path",
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
certPath: "your-cert-path",
keyPath: "your-key-path",
}
const apiClient = new enablebanking.ApiClient('Tatrabanka', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Tatrabanka
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ThreeSMoneyConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
TolgaOsSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.TolgaOsSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new TolgaOsSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Tolga-Os Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
TomamosImpulsoConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.TomamosImpulsoConnectorSettings;
ApiClient apiClient = new ApiClient(
new TomamosImpulsoConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.TomamosImpulsoConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('TomamosImpulso', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Tomamos impulso
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
TotalbankenConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
TriodosBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.TriodosBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new TriodosBankConnectorSettings()
.country("your-country")
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.signCertPath("your-sign-cert-path")
.signKeyPath("your-sign-key-path")
.signPubKeySerial("your-sign-pub-key-serial")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.TriodosBankConnectorSettings(
client_id="your-client-id",
client_secret="your-client-secret",
sign_cert_path="your-sign-cert-path",
sign_key_path="your-sign-key-path",
sign_pub_key_serial="your-sign-pub-key-serial"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
clientSecret: "your-client-secret",
signCertPath: "your-sign-cert-path",
signKeyPath: "your-sign-key-path",
signPubKeySerial: "your-sign-pub-key-serial"
}
const apiClient = new enablebanking.ApiClient('TriodosBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for TriodosBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
none |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required for production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required for production. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
TriodosBankESConnectorSettings
Settings for initializing ApiClient for TriodosBankES
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
UBPConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate private key in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
UBSConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UBSConnectorSettings;
ApiClient apiClient = new ApiClient(
new UBSConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UBSConnectorSettings(
country="your-country",
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UBS', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UBS
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UlsterBankConnectorSettings
Settings for initializing ApiClient for Ulster Bank (Ireland).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained during onboarding to the bank) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
false |
The path to the certificate for signing. |
signPubKeySerial |
string |
true |
The key id of sign certificate. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
UniCreditBankAustriaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UniCreditBankAustriaConnectorSettings;
ApiClient apiClient = new ApiClient(
new UniCreditBankAustriaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.business(false)
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UniCreditBankAustriaConnectorSettings(
business=False,
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
business: false,
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UniCreditBankAustria', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UniCredit Bank Austria
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
business |
boolean |
true |
none |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UniCreditBankCZSKConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UniCreditBankCZSKConnectorSettings;
ApiClient apiClient = new ApiClient(
new UniCreditBankCZSKConnectorSettings()
.redirectUri("https://your-redirect-uri")
.country("your-country")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UniCreditBankCZSKConnectorSettings(
country="your-country",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
country: "your-country",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UniCreditBankCZSK', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UniCredit Bank Czech Republic and Slovakia
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UniCreditBankHungaryConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UniCreditBankHungaryConnectorSettings;
ApiClient apiClient = new ApiClient(
new UniCreditBankHungaryConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UniCreditBankHungaryConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UniCreditBankHungary', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UniCredit Bank Hungary
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UniCreditBankRomaniaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UniCreditBankRomaniaConnectorSettings;
ApiClient apiClient = new ApiClient(
new UniCreditBankRomaniaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UniCreditBankRomaniaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UniCreditBankRomania', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UniCredit Bank Romania
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UniCreditBankSloveniaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UniCreditBankSloveniaConnectorSettings;
ApiClient apiClient = new ApiClient(
new UniCreditBankSloveniaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UniCreditBankSloveniaConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UniCreditBankSlovenia', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UniCredit Bank Slovenia
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UniCreditBulbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UniCreditBulbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new UniCreditBulbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UniCreditBulbankConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UniCreditBulbank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UniCredit Bulbank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UniCreditSPAConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UniCreditSPAConnectorSettings;
ApiClient apiClient = new ApiClient(
new UniCreditSPAConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UniCreditSPAConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('UniCreditSPA', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UniCredit S.p.A.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
UnicajaBancoConnectorSettings
Settings for initializing ApiClient for [UnicajaBanco]
Credentials can be obtained at Unicaja Banco Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
UnicreInstituicaoFinanceiraConnectorSettings
Settings for initializing ApiClient for Unicre - Instituição Financeira de Crédito S.A (Portugal)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Two-letter country code |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC private key in PEM format |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signPubKeySerial |
string |
true |
Serial number of a QSeal certificate from signKeyPath field |
clientId |
string |
true |
The client Id |
UnipolPayConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.UnipolPayConnectorSettings;
ApiClient apiClient = new ApiClient(
new UnipolPayConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("your-cert-path")
.keyPath("your-key-path")
.clientId("your-client-id")
.clientSecret("your-client-secret")
.business(false)
.sandbox(true);
import enablebanking
connector_settings = enablebanking.UnipolPayConnectorSettings(
cert_path="your-cert-path",
key_path="your-key-path",
client_id="your-client-id",
client_secret="your-client-secret",
business=False
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
certPath: "your-cert-path",
keyPath: "your-key-path",
clientId: "your-client-id",
clientSecret: "your-client-secret",
business: false
}
const apiClient = new enablebanking.ApiClient('UnipolPay', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for UnipolPay
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID. |
clientSecret |
string |
true |
API client secret. |
business |
boolean |
true |
Account type to access to. If true business, if false personal. |
VDKBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.VDKBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new VDKBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.VDKBankConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('VDKBank', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for VDKBank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
VUBBankaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.VUBBankaConnectorSettings;
ApiClient apiClient = new ApiClient(
new VUBBankaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("your-client-id")
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.VUBBankaConnectorSettings(
client_id="your-client-id",
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
clientId: "your-client-id",
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('VUBBanka', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for VUB Banka
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
VanLanschotConnectorSettings
Settings for initializing ApiClient for Van Lanschot Kempen N.V.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
ocpApimSubscriptionKey |
string |
true |
Subscription key which provides access to this API. |
clientId |
string |
true |
API client ID. |
VestjyskBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
VikSparebankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.VikSparebankConnectorSettings;
ApiClient apiClient = new ApiClient(
new VikSparebankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Vik Sparebank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
VolkswagenBankConnectorSettings
Settings for initializing ApiClient for Volkswagen Bank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country, two-letter country code. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. |
business |
boolean |
true |
none |
VolvofinansConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.VolvofinansConnectorSettings;
ApiClient apiClient = new ApiClient(
new VolvofinansConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Sydbank
Credentials can obtained at Volvofinans Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
clientId |
string |
true |
API client ID (obtained from Volvofinans Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Volvofinans Developer Portal) |
xApiKey |
string |
true |
API client key (obtained from Volvofinans Developer Portal) |
VossSparebankBusinessConnectorSettings
Settings for initializing ApiClient for Voss Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
VossSparebankConnectorSettings
Settings for initializing ApiClient for Voss Sparebank
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSeal certificate in PEM format. |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
VossVekselOgLandmandsbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.VossVekselOgLandmandsbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new VossVekselOgLandmandsbankConnectorSettings()
.certPath("path-to-qwac-cert")
.keyPath("path-to-qwac-key")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for Voss Veksel- og Landmandsbank.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
WiZinkConnectorSettings
Settings for initializing ApiClient for [WiZink]
Credentials can be obtained at WiZink Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
Company id in the format PSD{country_code}-{NCA_code}-{PSP identifier} Eg. PSDFI-FINFSA-29884997 |
signKeyPath |
string |
true |
Path to QSeal private key in PEM format |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
certPath |
string |
false |
Path to QWAC certificate in PEM format (production only) |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format (production only) |
WiseConnectorSettings
Settings for initializing ApiClient for TriodosBank (BE)
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country |
clientId |
string |
true |
API client ID. |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required for production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required for production. |
signKeyPath |
string |
true |
Path to QSEAL certificate private key in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. |
YaConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
language |
string |
true |
User interface desired language |
clientId |
string |
true |
none |
xApiKey |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
ZagrebackaBankaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ZagrebackaBankaConnectorSettings;
ApiClient apiClient = new ApiClient(
new ZagrebackaBankaConnectorSettings()
.redirectUri("https://your-redirect-uri")
.business(false)
.certPath("your-cert-path")
.keyPath("your-key-path")
.sandbox(true);
import enablebanking
connector_settings = enablebanking.ZagrebackaBankaConnectorSettings(
business=False,
cert_path="your-cert-path",
key_path="your-key-path"
)
api_client = ApiClient(connector_settings)
const enablebanking = require('enablebanking');
const CONNECTOR_SETTINGS = {
sandbox: true,
consentId: null,
accessToken: null,
redirectUri: "https://your-redirect-uri",
business: false,
certPath: "your-cert-path",
keyPath: "your-key-path"
}
const apiClient = new enablebanking.ApiClient('ZagrebackaBanka', CONNECTOR_SETTINGS)
Settings for initializing ApiClient for Zagrebacka banka
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
business |
boolean |
true |
none |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
ZiraatBankConnectorSettings
Settings for initializing ApiClient for ZIRAAT BANK INTERNATIONAL AG Hauptverwaltung (Germany).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country. |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signCertPath |
string |
true |
Path to QSEAL public certificate in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
Platform
Java
public abstract class AbstractConnectorSettingsFactory
Base class for connector settings factory, which can be used for creating instances of connector-specific settings classes (derived from ConnectorSettings class)
Code sample
public class SampleConnectorSettingsFactory {
@Override
public String getConnectorName() {
// Returning connector identifier
return "Nordea";
}
@Override
public Boolean getSandbox() {
// Returning value of `sandbox` parameter (common for all connectors)
return true;
}
@Override
public String getAccessToken() {
// Returning "not set" for `accessToken` parameter (common for all connectors)
return null;
}
@Override
public String getConsentId() {
// Returning "not set" for `consentId` parameter (common for all connectors)
return null;
}
@Override
public String getRedirectUri() {
// Returning value of `redirectUri` parameter (common for all connectors)
return "https://redirect-uri";
}
public String getClientId() {
// Returning value of `clientId` parameter (Nordea specific)
return "api-client-id";
}
public String getClientSecret() {
// Returning value of `clientSecret` parameter (Nordea specific)
return "api-client-secret";
}
public String getCertPath() {
// Returning value of `certPath` parameter (Nordea specific)
return "/path/to/qwac/cert";
}
public String getKeyPath() {
// Returning value of `keyPath` parameter (Nordea specific)
return "/path/to/qwac/key";
}
}
abstract protected String getConnectorName()
- Returns: Connector identifier
abstract protected Boolean getSandbox()
- Returns:
true
- sandbox settings,false
- production settings
abstract protected String getConsentId()
- Returns: Consent identifier (
null
if consent is not set)
abstract protected String getSandboxConsentId()
- Returns: Sandbox consent identifier (
null
if consent is not set)
abstract protected String getAccessToken()
- Returns: User access token (
null
if access token is not set)
abstract protected String getRefreshToken()
- Returns: User refresh token (
null
if refresh token is not set)
abstract protected String getSandboxClientSecret()
- Returns: Sandbox client secret (
null
if client secret is not set)
public ConnectorSettings createConnectorSettings()
Creates connector settings for a connector with identifier returned from getConnectorName
method. The created connector settings instance will contain data returned by the
corresponding methods of the factory. For example, if getConnectorName
returns "Nordea"
instance of NordeaConnectorSettings
class will be created and data like client ID and
client secret will be set (corresponding methods including getClientId
and
getClientSecret
must be implemented in the factory, otherwise runtime exception will be
thrown).
- Returns: Instance of a connector settings class
public abstract class AbstractPlatformExtension
Base class for customazing low-level functionality to the requirements of a target platform
- Package: com.enablebanking
abstract protected PlatformApiResponse makeRequest(PlatformApiRequest request, boolean followRedirects, Integer timeout)
Executes API request and returns an object containing response details.
- Parameters:
request
— Request details including parameters for establishing (M)TLS connectionfollowRedirects.
— Specifies if HTTP request should follow redirects
- Returns: Object containing response details
abstract protected String getCertHashAlgorithm(String certPath)
Gets the hash algorithm from the provided certificate
- Parameters:
certPath
— Path to the certificate - Returns: String containing hash algorithm
abstract protected String signWithKeyString(String data, String keyString, String hashAlgorithm, String cryptoAlgorithm)
Generates signature of the provided data
.
Signing algorithm is determined by the key
- Parameters:
data
— String to be signedkeyString
— Key string used for signing {@code data} parameterhashAlgorithm
— Hash algorithm to use (optional). If not provided then SHA256 will be usedcryptoAlgorithm
— Cryptographic algorithm to use. Default RS used if algorithm is not provided
- Returns: String containing signature
abstract protected String signWithKey(String data, String keyPath, String hashAlgorithm, String cryptoAlgorithm)
Generates signature of the provided data
.
Signing algorithm is determined by the key
- Parameters:
data
— String to be signedkeyPath
— URI of the key used for signing {@code data} parameterhashAlgorithm
— Hash algorithm to use (optional). If not provided then SHA256 will be usedcryptoAlgorithm
— Cryptographic algorithm to use. Default RS used if algorithm is not provided
- Returns: String containing signature
abstract protected boolean verifySignature(String signature, String message, PlatformJWKCertificate cert, String hashAlgorithm, String cryptoAlgorithm)
- Parameters:
signature
— Base64 urlsafe encoded signature to verifymessage
— Message to verify againstcert
— Instance of a PlatformJWKCertificatehashAlgorithm
— Hash algorithm to use (optional). If not provided then SHA256 will be usedcryptoAlgorithm
— Cryptographic algorithm to use. Default RS used if algorithm is not provided
- Returns: boolean showing if signature is valid
abstract protected String loadPEM(String path)
Load PEM content.
- Parameters:
path
— {String} — Path to PEM - Returns: String – PEM content
abstract protected String encryptWithCertString(byte[] data, String certString, String hashAlgorithm, String cryptoAlgorithm)
Encrypts the provided data
using the provided certificate.
- Parameters:
data
— String to be encryptedcertString
— String Public key content stringhashAlgorithm
— String. Hash algorithm to use. Default SHA256 used if algorithm is not providedcryptoAlgorithm
— CryptoAlgorithm. Cryptographic algorithm to use. Default RSA-OAEP used if algorithm is not provided
- Returns: String containing encrypted data
abstract protected com.enablebanking.KeyPair generateKeyPair(String cryptoAlgorithm, int keySize)
Generate Key Pair.
- Parameters:
cryptoAlgorithm
— {String} — Cryptographic algorithm to usekeySize
— {int} — key size
- Returns: KeyPair – Generated public and private PEM
abstract protected String encryptWithAEAD(String data, byte[] key, byte[] vector, byte[] aad, String algorithm)
Authenticated Encryption with Associated Data (AEAD)
- Parameters:
data
— String. Data to encode.key
— byte[]. Content Encryption Key (CEK) - a symmetric key for the AEAD algorithm used to encrypt the plaintext to produce the ciphertext and the Authentication Tag.vector
— byte[]. UTF-8 BytesData encoded Initialization Vector value used when encrypting the plaintext.aad
— byte[]. UTF-8 BytesData encoded Additional Authenticated Data - an input to an AEAD operation that is integrity protected but not encrypted.algorithm
— String. Algorithm to use. Right now only AESGCM is supported.
- Returns: String. Dot-separated, base64 urlencoded encrypted data with tag. Format: "{encryptedData}.{tag}"
@Builder public class PlatformApiRequest
Internal representation of API request
- Package: com.enablebanking
public PlatformApiRequest(String method, String origin, String path, List<Map.Entry<String, String>> headers, List<Map.Entry<String, String>> query, String body, TlsSettings tls)
Creates instance of the class with all necessary parameters.
- Parameters:
method
— HTTP method: GET, POST, PUT, etcorigin
— HTTP origin (i.e.<scheme> "://" <hostname> [ ":" <port> ]
)path
— Path part of a requested URLheaders
— HTTP headers as a list of key-value pairsquery
— Query part of a requested URL as a list of key-value pairsbody
— HTTP bodytls
— Parameters for establishing (M)TLS connection
public PlatformApiRequest(String method, String origin, String path, List<Map.Entry<String, String>> headers, List<Map.Entry<String, String>> query, String body)
Creates instance of the class with all parameters except (M)TLS settings.
- Parameters:
method
— HTTP method: GET, POST, PUT, etcorigin
— HTTP origin (i.e.<scheme> "://" <hostname> [ ":" <port> ]
)path
— Path part of an URLheaders
— HTTP headers as a list of key-value pairsquery
— Query part of an URL as a list of key-value pairsbody
— HTTP body
public String getMethod()
- Returns: HTTP method of the request
public String getOrigin()
- Returns: HTTP origin of the request
public String getPath()
- Returns: Path part of the requested URL
public List<Map.Entry<String, String>> getHeaders()
- Returns: HTTP headers of the request
public List<Map.Entry<String, String>> getQuery()
- Returns: Query part of the requested URL
public String getBody()
- Returns: Body of the request
public TlsSettings getTls()
- Returns: Parameters for establishing (M)TLS connection for the request
public String findHeader(String header)
- Parameters:
header
— Name of an HTTP header - Returns: First entry of the HTTP header with a given name or null if not found
@Builder @NoArgsConstructor public class TlsSettings
Internal representation of the parameters used to establish (M)TLS connection
- Package: com.enablebanking
public TlsSettings(String certPath, String keyPath)
Creates instance of the class with all necessary parameters.
- Parameters:
certPath
— URI for identifying client certificate for MTLS connectionkeyPath
— URI for identifying private key for MTLS client certificate
public String getCertPath()
- Returns: URI for identifying client certificate for MTLS connection
public String getKeyPath()
- Returns: URI for identifying private key for MTLS client certificate
@Override public boolean equals(Object obj)
Checks if this object equals to another.
- Parameters:
obj
— Object to compare with - Returns: true when {@code obj} is considered equal to {@code this}, otherwise false
@Builder public class PlatformApiResponse
Internal representation of API response
- Package: com.enablebanking
public PlatformApiResponse(int statusCode, List<Map.Entry<String, String>> headers, String body)
Creates instance of the class with all necessary parameters.
- Parameters:
statusCode
— HTTP response codeheaders
— HTTP response headers as list of key-value pairsbody
— Body of HTTP response
public int getStatusCode()
- Returns: HTTP code of the response
public List<Map.Entry<String, String>> getHeaders()
- Returns: HTTP headers of the response
public String getBody()
- Returns: Body of the response
public String findHeader(String header)
- Parameters:
header
— Name of an HTTP header - Returns: First entry of the HTTP header with a given name or null if not found
Changelog
0.14.3 - Unreleased
Changed
- AuthUrlType is updated. New values are: REDIRECT, IFRAME, APP, IMAGE_LINK, IMAGE_RENDER, IMAGE_B64
- AuthDataItem is updated. New values are: otpIndex, message, APP, IMAGE_LINK, IMAGE_RENDER, IMAGE_B64
- New fields added to MakeTokenException and PaymentConfirmationException: url and authData
Connectors changed
- [EpirusBank][GreeceBaseConnector] fix authorization request
- [OP] return debtor account and name in getPaymentRequest
- [Wizink] rollback to api version v1
- [OrangeBank] fix the TPP-Redirect-URI header
- [SaltEdge] fix NPE in validateDataRetrievalResponse
- [WorldlineBase] fix the paymentId
- [KH] disable response id validation
- [OKQ8Bank] Fix accounts datamapping
- [Atruvia] improve error validation
- [Redsys] improve error validation
0.14.2 - 2024-04-21
Fixed
- [Python] Fixed logging initialisation creating multiple loggers and causing memory leak
Connectors added
- [RBSInternational] ais integration
Connectors changed
- [AbancaBase] fix the date header
- [AbancaBusiness] contract index for contract selection
- [AbancaBusiness] fix the signature
- [ActivoBank][SantanderTotta] set default dateFrom if not present
- [AktiaContingency] Limit transaction details fetching to 30s
- [AktiaContingency] set remittanceInformationRequired to true for SEPA payments
- [Alandsbanken] added remittance information and reference number validation for FI SEPA
- [Atruvia] Sandbox origin and path prefix are changed and payment URLs are corrected
- [Bankinter] fix duplicate request id problem
- [BNPParibasFortisBusiness] Fix token request
- [BNPParibasFR] Fix client_credentials token request for SEPA payments
- [BNPParibasPoland] upgrade to API version 2.1.5
- [BRDBusiness][BRD] Fix credentials description
- [BredBanquePopulaire] Add Content-Type to AIS and PIS requests headers
- [BredBanquePopulaire] Change Content-Type to a required header
- [DanskeBankBase] fix handling invalid transactions interval
- [DanskeBankPersonal][DanskeBankBusiness] add nbf claim to jwt
- [DanskeBankPersonal][DanskeBankBusiness] return debtor name in getPaymentRequest
- [DiPocket] sandbox integration fix
- [DNBBusiness] for avoidance of confusion changed title of the COMPANYID credential to "User ID"
- [DNBBusiness] update companyId template
- [DNBBase] handle invalid credentials error
- [EikaBusiness][EikaPersonal] fix domain url mapping
- [ING][INGWholesaleBanking] improve debtor bic parsing
- [INGPoland][MillenniumBank][PKO] improve error response handling
- [INGPoland] disable fetching single account details in getAccounts call
- [KH] fix response id validation
- [KH] Set Accept header to '/' for AIS calls
- [Luminor] improve session expiration in case of updating certificates due to re-onboarding
- [Lunar] added support for Sweden and Norway
- [Lunar] add transactions pagination
- [MBHBank] improved account id parsing
- [MedicinosBankas] brand changed to Urbo, updated integration to use new endpoints
- [Morrow] removed PSU type business as the bank does not serve business customers
- [NovoBanco][SIBSBaseV3] improve continuation key handling
- [OP] Improve reference number validation
- [OP] Only one of reference number or remittance information should be set for SEPA payments
- [PayPal] upgraded API version to the latest
- [Postbank] fix issue with invalid value date
- [Qonto] fix parsing iban issue
- [Redsys] disable response id validation
- [Redsys] upgraded to version 1.1
- [Redsys][SIBS] enabled support of 180 days max consent validity
- [Redsys][BancaMarch] fix handling of already valid consent
- [SEBSweden] add fallback on
validateResponse
invalidateMakeToken
- [SPankki] added remittance information and reference number validation for FI SEPA
- [Swedbank] add balanceAfterTransaction field mapping
- [UlsterBank] fixed authorization issue
- [UnicajaBanco] cleaning balanceAfterTransaction values as the bank returns values containing spaces
Connectors removed
- [AndebuSparebank] connector removed, because the bank doesn't operate anymore
- [FidorBank] connector removed, because the bank doesn't operate anymore
- [Fornebubanken] connector removed, because the bank doesn't operate anymore
- [KBCIreland] connector removed, because the bank doesn't operate anymore
- [OerskogSparebank] connector removed, because the bank doesn't operate anymore
- [RomsdalSparebank] connector removed, because the bank doesn't operate anymore
0.14.1 - 2024-03-19
Added
- Add creditor/debtor IBAN validation for payments
Connectors changed
- [Abanca][AbancaBusiness] fix the remittance information mapping
- [AbancaBusiness] add a separate connector for business channel
- [BPCE] no "?" char in the successfulReportUrl
- [BPCE] creationDateTime format fix
- [BredBanquePopulaire] Fix modifying consent path
- [Citadele] enabled support of 180 days max consent validity
- [DanskeBankPersonal][DanskeBankBusiness] Defined supported reference number schemas for SEPA payments
- [Handelsbanken][HandelsbankenBusiness] Defined supported reference number schemas for SEPA payments
- [KH] Fix IBAN regexp
- [Luminor] enabled support of 180 days max consent validity
- [MediobancaPremier] fix the redirect_uri
- [MedicinosBankas] enabled support of 180 days max consent validity
- [MBHBank] added support for
Access.validUntil
, improved expired consent validation - [N26] enabled support of 180 days max consent validity
- [Nordea][NordeaBusiness] Defined supported reference number schemas for SEPA payments
- [Nordea][NordeaBusiness] added support for DK domestic payments
- [NordeaCorporate] fixed authorization flow handling when only one approval is required
- [Nordea][NordeaBusiness] initiate multiple signing basket payments in parallel (previously they were initiated consecutively)
- [OmaSp] Defined supported reference number schemas for SEPA payments
- [OP] added support for BULK_SEPA payments
- [OP] Defined supported reference number schemas for SEPA payments
- [OTPBank] fix the validation for refreshing token
- [PermanentTSB] added the self-generated
entryReference
- [POPPankki] Defined supported reference number schemas for SEPA payments
- [POSTEPAY] Fix account transactions request
- [Saastopankki] Defined supported reference number schemas for SEPA payments
- [SBAB] removed sign from transaction amounts
- [SBAB] improved data mapping for remittance information and internal notes
- [SEB] enabled support of 180 days max consent validity (for EE, LT and LV)
- [SiauliuBankas2] enabled support of 180 days max consent validity
- [UniCreditBankRomania][UniCreditBulbank] enabled production environment
- [VanLanschot] fix the validator for refreshing token
0.14.0 - 2024-02-22
Added
- HalPaymentRequest model is extended with paymentRequest field.
- Added psuIpAddressV6Supported meta flag.
- Added the PaymentRequestTransaction function for fetching payment transaction details.
- Added PaymentRequestResourceDetails, CreditTransferTransactionDetails and HalPaymentRequestTransaction models, paymentRequest field in the HalPaymentRequest model is using PaymentRequestResourceDetails model instead.
- Added pisAccountsAvailable meta flag.
Changed
- (Breaking Change) added optional
timeout
parameter formakeRequest
, which isnull
by default. Used for setting custom timeouts per request. - Field transaction.resourceId nulled for connectors where is not possible to retrieve transaction details.
Connectors added
- [LKU] AIS & PIS integration
Connectors changed
- [Abanca] Make iban as the resourceId because of the changing resourceId
- [AktiaContingency] Added meta information indicating that the maximum consent validity is 1 hour
- [AktiaContingency] Missing BIC code added
- [AmExNew] Update revoke token
- [Andbank] fixed the consent
- [ArkeaBase] fixed the signature
- [ArkeaBase] fixed the signature
- [ArkeaBase] Remove size from accounts call query parameters
- [BancoSantander] Migrated to API v1.1
- [BNPParibasPoland][MBank][SantanderBankPolska] handle rate limit response
- [Boursorama] added SEPA payment
- [BPCE] Fix payment request body
- [BPCE] requestedExecutionDate format fix
- [CreditAgricoleFR] Handle Invalid status dateFrom error
- [DanskeBankBusiness][DanskeBankPersonal] setting entryReference to TransactionId when TransactionReference contains only zeros
- [DjurslandsBank][JyskeBank][Kreditbanken][Landbobank][NordfynsBank][SkjernBank] [SparekassenSjaellandFyn][Sydbank] reduced transactions page size to avoid timeouts
- [DKB] fix the no extra sca flow
- [DNB] handle getting card accounts error
- [DNBBase] end_to_end_id limited to 35 characters
- [DNBBase] Remove PSU-HTTP-METHOD header
- [DNBBase] Send SEPA payment body
- [DNBBusiness] Add IBAN to supported debtorAccountSchemas for DOMESTIC payments
- [DNBBusiness] Send companyID in PSU-ID header
- [DNBBusiness] update credentials template
- [Handelsbanken] improve parsing of card balances
- [Handelsbanken][HandelsbankenBusiness] charge bearer values removed from meta for SEPA payments in FI
- [Handelsbanken][HandelsbankenBusiness] maximum consent validity is now 180 days
- [IbercajaBanco] set PSU-Accept header to application/json
- [ICABanken] update API urls
- [ING] improve parsing creditor agent bic
- [ING] removed FR from list of supported countries
- [ING][INGWholesaleBanking] Extracting creditor IDs
- [ING][INGWholesaleBanking] Extracting reference numbers only if they follow ISO 11649
- [ING][INGWholesaleBanking][Sparkasse] Maximum allowed AIS consent validity period is set to 180 days
- [KeytradeBank] Add the TPP-Redirect-URI header in the authorizations endpoint
- [KH] Fix transaction status mapping
- [KH] Improve app token parsing
- [KH] Improve app token parsing
- [LaBanquePostale] upgrade to v2
- [Lansforsakringar][LansforsakringarBusiness] Missing BIC code added
- [LHV] fix handling expired refresh token
- [LHV][LHVBusiness] Missing BIC code added
- [LHVBase] Fixed the consent request body
- [LHVBusiness] fix creating consent
- [LHVBusiness] fix creating consent
- [Luminor] Missing BIC code added
- [Lunar] improve transactions response data mapping
- [Max] remove the Max connector
- [MBHBank][Takarekbank] handle RateLimit exception
- [MBHBank][Takarekbank] improved refresh token handling
- [N26] handle CONSENT_INVALID error
- [N26] handle transaction period exception
- [NLB] added the required psu headers
- [Nordea] SEPA payments in Finland use single SCA approach when debtor account is provided
- [NordeaBase] added the signing basket payment
- [NordeaCorporate] add invalid credentials exception
- [NordeaCorporate] improved handling of pending transactions
- [NordeaCorporate] improved transaction entry reference mapping
- [OP] improved scope requested when creating accounts consent
- [OTPBank] handle expired consent in cases when new authorization deactivates previously created authorization
- [PermanentTSB] remove the validation for consentID in the IdToken
- [Pleo] fix populating transaction entry reference
- [POPPankki][OmaSp][Saastopankki] explicit accounts provided during authorization are now ignored, as this is not supported by the banks.
- [Qonto] fix parsing scheme name
- [Qonto] Fixed the scope for AIS
- [RedsysBase] fixed an issue when call would fail if using compressed IPv6
- [RedsysBase] modifyConsents adds state to the redirectUri
- [Renta4Banco] improve expired token error handling
- [Sabadell] improved expired refresh token handling
- [Samlink] payment debtor information is provided in the getPaymentRequest response
- [SEB][SEBBusiness] Missing BIC code added
- [SEBSweden][SEBSwedenBusiness] Missing BIC code added
- [Swedbank] fix getting over 90 days transactions
- [Swedbank] fix requested execution date and reference number for SE SEPA
- [Swedbank] in SEPA payments setting debtor account when it's provided
- [TietoEvryBusiness] Remove PSU-Context header
- [TietoEvryBusiness] Send PSU-CONTEXT header to receive PSU-CORPORATE-ID param
- [TietoEvryBusiness] Send PSU-Corporate-Id header
- [TomamosImpulso] adding new brand Targobank
- [TriodosBank] fix the continuationKey
- [UK] improved bank transaction code data mapping
- [UK][Danske] improve InterimCleared balance mapping
- [AIB][AmExNew][AionBank2][BancaMediolanum][BanqueCPH][BanqueTransatlantique][BankDeKremer] [BankVanBreda][Bankinter][BNPParibasFRBusiness][BNLBusiness][Boursorama][BpostBank] [BredBanquePopulaire][CajamarCajaRural][CIC][Comdirect][CreditMutuel][CreditMutuelBaseConnector] [DeutscheBankBusinessES][DeutscheBankES][DynamicMockASPSP][ErsteSteiermarkischeBank] [Finecobank][HelloBankFR][KeytradeBank][Kvika][LCL][Nagelmackers][OpenBank][PayPalBase] [SiauliuBankas2][SocieteGeneraleBase][Solarisbank][SweepBank][TomamosImpulso][TriodosBank] [UniCreditSPA] The connectors from now on are considered stable (i.e. have sufficient traffic and no ongoing issues) and thus beta flag is removed
- [VolkswagenBank] changed to another platform provider
- [Wise] enabled in all EEA countries
- [Wise] Missing BIC code added
Connectors extended
- [ArkeaBase] added PIS integration (SEPA)
- [Bankart] added PIS integration (SEPA)
- [BNPParibasFR] added PIS integration (SEPA and INSTANT_SEPA)
- [BPCE] added PIS integration (SEPA)
- [BredBanquePopulaire] added PIS integration (SEPA)
- [CaisseDEparnge] added PIS integration (SEPA)
- [CreditAgricoleFR] added PIS integration (SEPA)
- [DNBBase] added BULK_DOMESTIC
- [LaBanquePostale] added PIS integration (SEPA)
- [LCL] added PIS integration (SEPA)
- [NordeaCorporate] added DOMESTIC and DOMESTIC_SE_GIRO payment integrations
0.13.10 - 2023-11-16
Added
- TransactionPeriodException is extended with dateFrom and dateTo fields
- transactionHistoryDays field is added to the connector's meta information. It contains the maximum number of days for which transactions can be fetched.
Connectors changed
- [AIB] Maximum allowed AIS consent validity period is set to 180 days
- [AIB] Validate response data
- [BNPParibasFRPersonal] Handle empty response on balances call
- [DynamicMockASPSP] supported filtering and pagination
- [DanskeBankPersonal][DanskeBankBusiness] remittance information is set required for SEPA
- [LCL] Handle wrong transactions period error
- [CreditAgricoleFR] Handle rate limit error
- [Lunar] fixed refresh token handling
- [Nordea][NordeaBusiness] Not returning resourceId for transactions as fetching details isn't possible
- [Nordea][NordeaBusiness] Nulling account name if its value is the same as the product
- [NordeaBusiness] Using account_holder field as name if it's available (seems to be only in Finland)
- [RedsysBase] Patching IPv6 PSU-IP-Address for making sandbox to work
- [RedsysBase][BBVA] token and transaction data validation
- [KBC] Fixed refresh token
- [BankVerlag] handle PERIOD_INVALID transactions fetching error
- [DeVolksbank] Fixed sepa payment body
- [Rabobank] fixed the sepa payment body
- [DNBBusiness] update credentials template
- [Holvi] improved/fixed transactions pagination
- [Qonto] improved transactions data mapping
- [SIBSBaseV3] set dateFrom param by default
- [Finecobank] add transaction entry reference
- [Kvika] handle account not accessible error
- [Islandsbanki] improve fetching list of accounts and account details
- [Kvinesdal][Gildeskål][Valle][Romerike][Agder] banks migrated from SDC platform provider to TietoEvry
- [SBAB] handle expired access token
- [SEBSwedenBase] Get transaction ID from transaction details link
- [DanskeBank] Split into DanskeBankPersonal and DanskeBankBusiness
- [SEBBusiness] fixed reference number handling for DOMESTIC_SE_GIRO
- [Luminor] fix creating consent with duplicated accounts
- [ActivoBankES] [Andbank] [Kutxabank] [LaboralKutxa] [OpenBank] [Renta4Banco] [Sabadell] [SelfBank] [UnicajaBanco] [WiZink] disable bulk payment for some unsupported banks
- [KH] Fix accounts parsing
- [Euroabnk] upgrade to new API
- [OpenBank] extract data from transaction remittance information
- [KH] Return empty array when resource not authorized
- [KH] Fix transactions response parsing
- [NordeaCorporate] add multiple signers authorization support
- [DNBBusiness] DE, DK, FI & SE are enabled
Connectors extended
- [CreditMutuel] added PIS integration (BULK_SEPA, SEPA)
- [RevolutBusiness] added BULK_SEPA payment type
Connectors removed
- [Komplett]
0.13.9 - 2023-09-13
Added
- Added
referenceNumberSchemas
to list the reference number schemas supported by a payment method - Added
BULK_SEPA
as a newPaymentType
to support the bulk payment - Norwegian reference number validation
Connectors added
- [AndbankLU][BankinterLU][BanqueDeLuxembourg][BanqueDeLuxembourgBE][BanqueDePatrimoinesPrives] [BanqueTransatlantiqueBE][BanqueTransatlantiqueLU][EastWestUnitedBank][EurobankPrivateBank] [FideuramBank][IntesaSanpaoloWealthManagement][JuliusBaer][PostLU][RaiffeisenLU][SafraBank] [SantanderLU][SebLU][SebNO][Spuerkeess][SwissquoteBankEurope][ThreeSMoney][UBP] account information service
- [EpirusBank] account information service
- [LPB] account information service
- [NordeaCommercialCards] account information service
- [ProCreditBank] account information service
Connectors changed
- [Abanca] fixed the currency and the contract id field
- [ANBAMRO] changed sandbox auth url
- [ActivoBankES][Andbank][BBVA][BancoInversis][BancoMediolanum][BancoSantanderCorporate][Bankinter] [CaixaBank][CajamarCajaRural][CajasurBanco][EurocajaRural][IbercajaBanco][Kutxabank][OpenBank] [Renta4Banco][Sabadell][SelfBank][WiZink] Maximum allowed AIS consent validity period is set to 180 days
- [ArkeaBase] fixed signature generation
- [Atruvia] fixed content type for some requests
- [BPI][SIBSBaseV4] added retry on received consent
- [BredBanquePopulaire] request signature fix
- [DNBBase] added support of reference number for domestic payments
- [FidorBank] Add custom transactions entry references
- [GranitBank] enabled production for account information service
- [KH] Fix consentId header
- [Landsbankinn][LandsbankinnBusiness][SaltEdge] fixed checking account refresh status
- [NBG] added entryReference field to transactions response
- [Nordea] improved parsing of card accounts
- [OTPBank] changed the auth origin
- [OTPBank] implemented continuation key in transactions fetching
- [Pleo] fixed parsing account details
- [SBAB] upgraded to v2 of authorization API
- [SEBSweden] add transaction details call
- [Skandia] payment initiation fixes
- [SpareBank1Business] meta information fix
- [Swedbank] passing state to payment confirmation SCA URL and using TPP-Nok-Redirect-URI with error=access_denied
- [Swedbank] improved data mapping for offline transactions
- [Swedbank] fixed fetching pending transactions
- [Tatrabanka] fixed refresh token
- [TietoEvryBase] add support of reference number (KID) for domestic payments
- [UnicreditBankHungary] add additional information mapping
Connectors extended
- [DeutscheBankBE][DeutscheBankDE][DeutscheBankES][DeutscheBankIT][DeutscheBankLU] added payment initiation service (SEPA payments)
- [ActivoBankES][Andbank][BBVA][BancoInversis][BancoMediolanum][BancoSantanderCorporate][Bankinter] [CaixaBank][CajamarCajaRural][CajasurBanco][EurocajaRural][IbercajaBanco][Kutxabank][OpenBank] [Renta4Banco][Sabadell][SelfBank][WiZink] added payment initiation service (SEPA and BULK_SEPA payments)
- [Sparkasse] added payment initiation service (SEPA payments)
- [EikaBusiness][EikaPersonal] Added brands: Aurskog Sparebank, Berg Sparebank, Birkenes Sparebank, Eidsberg Sparebank, Etnedal Sparebank, Evje og Hornnes Sparebank, Grong Sparebank, Hjartdal og Gransherad Sparebank, Larvikbanken, Oslofjord Sparebank, Romsdalsbanken, Rørosbanken, Sogn Sparebank, Soknedal Sparebank, Tinn Sparebank, Totens Sparebank, Valdres Sparebank, Vekekselbanken, Ørskog Sparebank
Connectors removed
- [BirkenesSparebank][BergSparebank][EidsbergSparebank][EtnedalSparebank][Larvikbanken] [ValdresSparebank] brands supported by the connectors moved to EikaBusiness and EikaPersonal
0.13.8 - 2023-08-05
Added
- Added public
validateCredentials
method inAuthApi
class modifyConsents
can now return optionalAuthData
array (containing information necessary for PSU to complete authorisation)- Added public
getTransactionDetails
method inAispApi
class
Connectors added
- [AlphaBankGR][AlphaBankRO][AlphaBankCY] account information service, payment initiation service
- [DiPocket] account information service
- [Eurobank] account information service
- [JTBanka] account information service
- [Kvika] account information service
- [NBG] account information service, payment initiation service
- [PiraeusBank] account information service
- [Pleo] account information service
- [Saxo] account information service (experimental)
- [SumUp] account information service
Connectors changed
- [AmExNew] Handle expired refresh token
- [BancaMediolanum][BankData][Belfius][CBIGlobe][Crelan][CSOBSK][DanskeBank][KH][LCL][Nordea] [NordeaBusiness][OmaSp][OP][POPPankki][RaiffeisenIT][SaastoPankki][Swedbank][SwedbankBusiness] Maximum allowed AIS consent validity period is set to 180 days
- [BBVA] Using transactionId as entryReference
- [KH] Request two separate consents (Identification consent, transaction history consent)
- [KH] Fix transaction history consent request
- [KH] Request transaction history token
- [MBHBank] Corrected origin for access token request
- [NordeaBusiness] fixed domestic SE giro payment
- [OP] fixed issue with empty reference number in v2 version of payments API
- [Postbank] Embedded Approach is no longer supported and the default SCA Approach is Redirect
- [RedsysBase] Fixed transactions' continuation key in case next page link is doesn't contain prefix
- [Sabadell] upgraded to API version v1.1.
- [SDCBase] DK DOMESTIC payments
- [SDCBase] fix transactions continuation key handling
- [SIBSBase] fix getting default headers
- [Solarisbank] add the entryReference
- [SparbankenSyd] Fixed extraction of clearing number and member id
- [SparbankenSyd] Add SE PIS DOMESTIC
- [Sparkasse] SEPA payments
- [SveaBank][SveaBankBusiness] Fixed removal of SVEA prefix from BBANs
- [Swedbank] improved fetching of pending transactions
- [TomamosImpulso] change the origin
- [Europabank] add the entryReference
Connectors extended
- [BankDataBase] add DOMESTIC payment type
0.13.7 - 2023-07-03
Changed
Connectors added
- [AddikoBankHR] account information service
- [CreditAgricoleITBusiness] Credit Agricole IT business accounts only
- [CreditAgricoleITPersonal] Credit Agricole IT personal accounts only
- [BancoCaminos][BancoCetelem][BancoEuropeoDeFinanzas][BancoSantanderCorporate][ActivoBankES][Bancofar] account information service
- [ErsteBankHUCorporate][ErsteBankHURetail] account information service
Connectors changed
- [ABNAMROBase] Updated production URLs
- [Atruvia] Brand "Volks- und Raiffeisenbank" changed to "VR Bank Mecklenburg"
- [AXA][Crelan][WorldlineBase] Requesting allPsd2 scope of access in case either balances or transactions are requested
- [BancoSantander][CajaRural][CajamarCajaRural][OpenBank] Added synthetic transaction entry reference
- [BankNorwegian] add handling of expired consent error
- [Belfius] change source field used for transaction entry reference
- [BNPParibasFortis] Allowing authorisation with card numbers starting with 4871
- [Boursorama] requesting extended_transaction_history scope of access
- [CreditAgricoleFR] Raise ExpiredConsentException when Consent expires
- [CreditAgricoleIT] Remove FriulAdria brand (Closed down)
- [DeutscheBankBase] Retrying makeToken on 503 response for authorisation status request
- [Handelsbanken] support crossborder payments in Sweden
- [Holvi] handle the error in case when account is disabled
- [ICABanken] fixed payment signing authentication link generation
- [KBC] Not requesting balances when fetching list of accounts
- [KH] fix authorization
- [Komplett] Komplett connector is replaced by Morrow connector.
- [LaBanquePostale] Raise TransactionPeriodException when requested period is not allowed
- [Landsbankinn] Fix for initiation of accounts data refresh before fetching balances and transactions
- [N26] fix getting consent status
- [OP] Migrated PIS to v2 API
- [OTPBank] passing state to the auth redirect URL and nulling "undefined" state from callback URLs
- [RaiffeisenHR] Enable production environment
- [RaiffeisenHR] Fix accounts data calls
- [RaiffeisenHR] Raise InvalidCredentialsException when wrong OIB provided
- [RaiffeisenHU] Added transaction entryReference
- [RaiffeisenIT] Improve authentication
- [RaiffeisenSK] Update to use the new PSD2 APIs
- [SEBSwedenBusiness] add crossborder payments
- [Swedbank] api version updated from 4 to 5
- [Swedbank] add crossborder payments
- [Swedbank] Fixed default end-to-end ID for bulk SEPA payments in EE, LT and LV
- [Tatrabanka] Update to use the new PSD2 APIs
- Following banks are migrated from SDC platform provider to Tieto Evry: Trøgstad Sparebank, Evje og Hornnes Sparebank, Hjartdal og Gransherad Sparebank, Soknedal Sparebank, Totens Sparebank, Oslofjord Sparebank, Romsdalsbanken, Rørosbanken, Tinn Sparebank, Aurskog Sparebank", Ørskog Sparebank
- [UniCreditBankHungary] Fixed the 62 days limit and add entryReference
Connectors removed
- [EikaKredittbank] removed
0.13.6 - 2023-06-04
Added
- Added a gzipCompress function in the platform
- Meta information about support of bulk payments (
maxTransactions
)
Changed
- HalPaymentRequest.paymentInformationStatus field is now optional, as the status is not returned in case payment is not yet (fully) initiated
Connectors added
- [GronlandsBANKEN] account information service
- [Cecabank] account information service (the same as the TriodosBankES)
- [Isybank] account information service (Banca5 migrated to a new bank Isybank)
- [MBHBank] account information service
- [SparekassenDanmark] because Sparekassen Vendsyssel rebranded to Sparekassen Danmark
Connectors changed
- [AktiaContingency] Improved account details data mapping
- [Alandsbanken] Fixed DOMESTIC_SE_GIRO payment type
- [BBVA] Handling the error related to refresh token
- [BredBanquePopulaire] Fix authentication
- [ConsorsBank] Added function to handle the html response
- [CreditMutuel] raised an exception for expired refresh token
- [Crelan][Nagelmackers] Added additional information into remittance information array
- [DeutscheBankIT] both transactionStatus not supported
- [ASNBank][ReggioBank][SNSBank] add handling of expired refresh token
- [Fidorbank] add handling of expired refresh token
- [Handelsbanken] FI SEPA, setting remittance information when reference number is provided
- [Handelsbanken] FI SEPA payment no longer requires creditor country to be set (creditorCountryRequired=false)
- [Handelsbanken] improved accounts list fetching behavior
- [ICABanken] Fixed BBAN from IBAN extraction (PIS only)
- [ICABanken] Removed invalid bookingDate for pending transactions
- [ING] fix the currency query param
- [KBC] Fixed generation of authorisation URL (using S256 value for the code_challenge_method param)
- [KH] Keeping only redirect auth method and removed userId credential as not required
- [KH] Fixes in the authorisation for access to account information
- [Knab] Account details mapping improved
- [Landsbankinn] Initiating accounts data refresh before fetching balances and transactions
- [Lunar] Fix account transaction conversion
- [MarginalenBank] add support of sha512 signature hash algorithm
- [MBank] Fix the mapping issue
- [MockASPSP] header psuGeoLocation is made optional
- [NordeaBase] Fixed BBAN from IBAN extraction (PIS only)
- [NordeaBase] using either
paymentStatus
orpayment_status
field from the response when checking payment status - [Postbank] Authentication using Postbank ID instead of account number
- [RaiffeisenHU] Fixed usage of Consent Id during authorisation
- [RaiffeisenHU] Fix accounts data calls
- [SaltEdge] Handling 'tppmessages' Filed Parsing Error
- [SantanderBankPolska] Fix the mapping issue
- [SBAB] Add function to get account holder name
- [SiauliuBankas2] handled the expired refresh token
- [Solarisbank] Make sure the parameter is presented if delta access or entryReferenceFrom is not supported
- [SparbankenSyd] Add clearning number and member id
- [Swedbank] default end-to-end identification for SEPA payments is limited to 32 characters
- [Swedbank] filling up BBAN to 15 digits when possible
- [Swedbank] SE sandbox environment fix
- [SweepBank][FerratumBank] add handling of expired refresh token
- [UBS] Generate the authorization url on our side
Connectors extended
- [ABNAMRO] add the bulk payment
- [AlmBrand][AndelskassenFaelleskassen][ArbejdernesLandsbank][BILDanmark][CoopBankDenmark] [DanskeAndelskassersBank][DenJyskeSparekasse][DjurslandsBank][FasterAndelskasse][FrorupAndelskasse] [FroslevMollerupSparekasse][FynskeBank][HandelsbankenDenmark][HvidbjergBank][JyskeBank][Kreditbanken] [LaegernesBank][Landbobank][LollandsBank][MajBank][MerkurAndelskasse][MonsBank][NordfynsBank] [NykreditBank][PenSam][SEBDenmark][SallingBank][SkjernBank][SparNordBank][SparekassenSjaellandFyn] [SwedbankDenmark][Sydbank][Totalbanken][VestjyskBank] SEPA and DOMESTIC payment integration
- [Alandsbanken] Added SE DOMESTIC payment type
- [DanskeBank] Added DOMESTIC_SE_GIRO payment type
- [DeVolksbank] add bulk payments support
- [ING] add the bulk payment
- [NordeaBusiness] add crossborder payments support
- [Swedbank] bulk payments support for SEPA in EE, LT, LV
Connectors removed
- [Banca5] replaced with Isybank (as Banca 5 transformed into Isybank)
- [BancaCarige][BancaRegionaleDiSviluppo] Banca Carige and Banca del Monte di Lucca became BPER Banca
- [MeridianBank] removed (as the bank migrated to new PSD2 APIs)
- [PrivatBank] removed (as the bank does not exist anymore)
0.13.5 - 2023-05-01
Added
- Added connectors: PermanentTSB, TriodosBankES, RaiffeisenHR
- [Swedbank][SEB] added option to select the language of SCA URL.
- [DNB][DNBBusiness] setting current consent id in the make token function.
- [MarginalenBank] Card accounts integration
- [ING] Extracting "Id Mandato" from unstructured remittance information for Italy
maximumConsentValidity
field is added to a connector's meta information- Added PIS datamapping for connectors: OP
- 'ACWP' payment status code added to the PaymentInformationStatusCode enum.
- [ArionBanki] added language support
Changed
- [CBIGlobe] Retrieve transactions 60 days in the past, with 1 month period batches
- Hemne Sparebank, JBF, Oppdalsbanken, Orkla Sparebank, Rindal Sparebank, Sunndal Sparebank, Tysnes Sparebank and Sparebanken Narvik are migrated from SDC platform provider to TietoEvry
- [SiauliuBankas2] API version updated from 1 to 2
- [Islandsbanki] Upgrade api to v2
- [ZagrebackaBanka] enable production
- [SEBSweden][SEBSwedenBusiness] maximumConsentValidity set to 180 days
Fixed
- [NordeaCorporate] Refresh token fix
- [Ya] Authorization fix
- [Qonto] Handle account not accessible exceptions
- [SaltEdge] Handle exception caused by fetching transaction entries for more than 90 days
- [N26] fix deleting consent, fix transactions entry reference, handle resource unknown error
- [SiauliuBankas2] handle wrong transactions interval error
- [OTPBank] fix the authCode parsing and datamapping
- [SEBKortBank][SEBKortBankBusiness] requesting psd2_payments scope in order to workaround 403 error when fetching list of accounts
- [SEB] handle expired consent exception
- [Nordea] handle account not found error
- [Holvi] handle withdrawn consent error
- [SDC] handle expired token error
- [Ya] Authorization
- [Qonto] Handle account not accessible exceptions.
- [SaltEdge] Handle exception caused by fetching transaction entries for more than 90 days.
- [N26] fix deleting consent
- [SiauliuBankas] handle wrong transactions interval error
- [N26] fix transactions entry reference
- [Knab] [Redsys] add an exception for unavailable account
- [DNBBase] Removed unsupported headers
- [Handelsbanken] fix the getAccountBalances function and the path in the getAccountTransactions
- [HandelsbankenBusiness] Not requesting card accounts for Finland
- [AmExNew] Fix transactions datamapping
- [MarginalenBank] Fix getAccounts concatenation
- [ABNAMRO][ABNAMROBusiness] Fix balance amount sign
- [CBIGlobe] fix the future dateTo
- [CreditMutuelBase] fix the dateTo that transactions are not included within the result in dateTo
- [Belfius] Handle account not accessible exception
- [BNBank] MakePaymentRequestConfirmation datamapping
- [DanskeBank] Handle access expired error
- [CBIGlobe] Booking status updated according to the restriction of each bank
- [CreditAgricoleFR] Send bank areas in modifyConsent body for users with several online banking areas
- [Luminor] added support for reference number and requestedExecutionDate in SEPA payments
- [TietoEvryBase] Set requestedExecutionDate to UTC+2
- [FidorBank] Fix the mapping of Entry Reference
- [N26] handle expired consent error
- [Sabadell] handle error showing a message to a PSU
- [CIBBank] fixed the creditDebitIndicator and added the bankTransactionCode
- [Arionbanki] Fix data mapping for transaction code and entry reference
- [Bunq] handle MonetaryAccountExternal in getAccounts call
- [Belfius] fixed account details handling
- [TietoEvryBase] Preserve paymentType in makePaymentRequestConfirmation
- [TietoEvryBase] Call getPaymentRequest after 2nd redirect
- [BNPParibasFortis] Handle not accessible account error
- [Nordea] Handle not accessible account error
- [SEBKortBank] handle empty card number
- [OP] fix the async signature
- [KH] Await getAuthHeaders
- [KH] Handle empty authorization response
- [RaiffeisenHU] Format validUntil
- [RaiffeisenHU] Add query parameters to the return sca Url
- [KH] Change selectScaMethod request body model
- [Handelsbanken] improved payment status mapping
- [MedicinosBankas] improved Creditor Agent bic mapping; added auth method for payments initiation.
- [Landsbankinn] fetching accounts status moved from getAccounts to makeToken to prevent timeout exception
- Following banks are migrated from SDC provider to Eika/TietoEvry: Bank 2, Bien Sparebank, Den Gule Banken, Hegra Sparebank, Høland og Setskog Sparebank, Marker Sparebank, Skagerrak Sparebank, Strømmen Sparebank, Trøgstad Sparebank
- [TietoEvryBase] Fix the holder name datamapping
- [TietoEvryBase] Fix details datamapping
- [SiauliuBankas2] removed unnecessary PSU headers
Removed
- [BNG] PSU Geo Location header requirement removed
- [Beobank] The max length limit of name in the BalanceResource was removed
0.13.4 - 2023-02-19
Changed
- [SEBKortBank] update the brands, Quintessentially Mastercard was removed in DK and SE, Circle K EXTRA Mastercard was added in DK, Landkreditt Mastercard was added in NO, and Scandic Mastercard was added in SE
- [SDCBase] Migrated AIS from API v1 to v2 for NO and DK banks using SDC as the PSD2 APIs provider
- Removed parameters
credentials
andmethod
from validatePaymentRequest function - Migrated AIS from API v1 to v2 for NO and DK banks using SDC as the PSD2 APIs provider
- [PolishAPI] Polished the API to get the account information, allowed getting possible account holder's name and address besides the basic account information
- [SEBKortBank] update the brands, Quintessentially Mastercard was removed in DK and SE, Circle K EXTRA Mastercard was added in DK, Landkreditt Mastercard was added in NO, and Scandic Mastercard was added in SE
- [DanskeBank] Extract recipient name and reference number from the remittance information
- [BNPParibasFortis][BNPParibasFortisBusiness] V3 API
- [Holvi] add a 6-month limit for the historical transactions
- [DNB][DNBBusiness] passing consent id through auth env
- [Atruvia] brands (Karlsruhe Baden-Baden and Pforzheim) were merged to be one brand (pur)
- [BNL] Removed BNL Corporate from the list of brands
- Allow using IBAN as debtor account for SE DOMESTIC and DOMESTIC_SE_GIRO payment types.
Fixed
- [Landsbankinn] workaround for requesting account-list consent
- [LHV] PISP authorization header
- [ConnectPay] Balances parsing
- [BPCE] Handle empty response
- [MedicinosBankas] fix credentials validation
- [Swedbank] Fix payment status mapping
- [PayPal] continuation key handling, transaction date field mapping
- [KH] Request consent for one service access (transactions)
- [BPCE] Pagination
- [ErsteBankAT] accounts list consent and accounts list retrieval
- [ConnectPay] ConnectPay transactions parsing and datamapping test
- [CheBanca] AIS consent and SCA flow
- [Storebrand] domestic payment initiation
- [LCL] DateTo should be before today
- [CheBanca] fixed the datamapping and continuationKey
- [CBIGlobe] authorisation fix
- [RaiffeisenIT] authorisation fix
- [Belfius] Improve the validator to handle the "Access is not ok for this account" error
- [MarginalenBank] Add the function to handle the issue that the account has no transaction data within the specified period
- [BankNorwegian] Improve the validator to handle the "Consent has expired" error
- [Bunq] Improve the validator to handle the "Insufficient authentication" error
- [SPankki] Improve the validator to handle the "Expired refresh token" error
- [MillenniumBank] Add Ratelimit exception to handle CONSENT_LIMIT_EXCEEDED error
- [ABNAMROBase] Date from must not be older than 18 months
- [OP] handing error parameter in redirect URL when it's in query
- [Belfius] Improve the validator to handler the "Access is not ok for this account" error
- [MarginalenBank] Add the function to handle the issue that the account has no transaction data within the specified period
- [Befius] Rvise the get account function to traverse all the pages and get whole accounts list
- [Bred] Fixed authorization
- [ABNAMRO] Inprove the validator grant revoked due to idle timeout error
- [CBIGlobe] Accounts datamapping fix
- [BredBanquePopulaire] (created) and (expired) signature headers not supported
- [DanskeBank] Improve the validator to handle the "The PSU does not have access to the requested account or it doesn't exist" error
- [BancaMediolanum] calling modifyConsents before fetching list of accounts
- [CreditMutuelBase] fixed the query and the continuationKey
- [AmExNew] Remittance information mapping fixed
Added
- Added connectors: HSBC, BRDBusiness, Finecobank, BNLBusiness.
- Added the bankTransactionCode.description field in the transaction
- [PayPal] personal payments, business payments
- [Handelsbanken] OCR number parsing for Sweden
- Added new payment type: INTERNAL for cases when a payment is done within the same ASPSP
- [TriodosBank] filling in transaction entry references
- Added I18n.t function for marking strings, which may appear in a user interface and need to be translated. Marked values of title and description fields in credentials for all connectors. Also added gulp task "core-i18n" for exporting translatable strings from the core's code.
- skipDebtorAccountValidation parameter to the validatePaymentRequest function
- [CreditMutuelBase] Added entryReference field for the transaction information
- [DeutscheBankBase] Add the entryReference field for the transaction information
- Added new exception: AccountNotAccessibleException
- [SveaBank] BBAN parsing
- [Swedbank][SEB] Added option to select the language of SCA URL.
- [Commerzbank] Added entryReference field to transaction data.
- [Handelsbanken] Card accounts API integration
0.13.3 - 2023-01-12
Changed
- Added default currency for Polish bank accounts as "XXX"
- Iterating through all transactions when filtering by date in the OP connector.
- Using BOOK status instead of OTHR for card transactions in the OP connector, because only booked transactions are returned by the bank.
- [MedicinosBankas] update account-list url
Added
- Added transaction filtering by date for the Holvi connector.
- SEPA payments for AktiaContingency, Revolut.
- Added the exchangeRate fields for card account transactions in the OP connector.
- Added NO domestic payments in the Nordea connector.
- [POPPankki][Säästöpankki][Oma Säästöpankki] Added support for fetching both transaction statuses at the same time
- [DNBBusiness] Added possibility to provide Company ID starting with TX
- [SiauliuBankas] SEPA and instant SEPA payments
- [Citadele] instant SEPA payment
- [DeVolksbank] [ASNBank] [SNSBank] [RegioBank] pisp integration
- [MedicinosBankas] SEPA payments
- [FerratumBank|SweepBank] SEPA payments
- [N26] SEPA payments
- Added connectors: Konist.
- [SDCBase] NO PIS
Fixed
- Throwing UnauthorizedException on suspicion of expired access token in the AmExNew connector.
- Fixed parseRedirectUrl to work without missing id_token verification in the KBCIreland connector.
- [Handelsbanken] Handle expired refresh token error
- SEPA payments for FI market in the Handelsbanken connector.
- SparebankenVest transaction parsing when date is not present
- [KBC] Handle expired refresh token error
- Improved handling of 408 HTTP response code
- [KBCIreland] fix the datamapping
- Handling of Berlin Group rate limit (ACCESS_EXCEEDED) error
- [CheBanca] Added the redirectUri header in ModifyConsents function
- [KomplettBank] Fixed authentication
- [SEBSweden] fixed the issue when payment confirmation step would be stuck for too long on authorization status retrieval
- [Boursorama] fixed the signature
- [Landsbankinn] fixed getAccounts method
- [UnicajaBanco] fixed the booking status
- [Kontist] fixed the exchange rate mapping
- [CreditAgricoleFR] Fixed transactions pagination
- [DanskeBank] fixed SEPA payment towards same country accounts
- [TietoEvryBase] fixed the datamapping of accounts
- [BankNorwegian] setting correct account type (CARD/SVGS) based on product name
- [KBCIreland] Throwing UnauthorizedException for token expired, TransactionPeriodException for invalid date
- [Nordea] proper handling of expired refresh token error
- [LHV] sandbox environment and SEPA payments
- [Islandsbanki] fixed pagination for account transactions, raise TransactionPeriodException for invalid date range, adjusted transaction mapping
- [MillenniumBank] fixed modifyConsent to update and not override the consentId
- [TietoEvry] fixed payment status call and async calls (getDefaultHeaders/getPispHeaders)
- [SpareBank1] [SpareBank1Business] SpareBank 1 Telemark and SpareBank 1 Modum merged with SpareBank 1 BV and renamed to SpareBank 1 Sørøst-Norge; SpareBank 1 Nordvest renamed into SpareBank 1 Nordmøre.
- [BredBanquePopulaire] ModifyConsent before accounts
0.13.2 - 2022-12-06
Changed
- Improved mapping of transaction fields in the SEB (EE, LT and LV) and SveaBank (SE) connectors.
- Migrated to use v8 AIS API in the SEBSweden and SEBSwedenBusiness connectors.
- Caisse d'Epargne brands moved from BPCE to the new CaisseDEpargne connector.
- Enabled support of CURDEPOSIT and LOCDEPOSIT accounts in the SparebankenVest connector.
Added
- Added connectors: ApoBank, CaisseDEpargne, KBCIreland.
- Extracting reference number from unstructured remittance information in the BancoSantander connector.
- Added
entryReference
for connectors derived from the TietoEvry connectors (many banks in Norway). - Added
entryReference
for LaBanquePostale connector. - Added creditor/debtor IDs mapping for Sparkasse connector.
- Added SEPA payments support in the ABNAMRO connector.
- Added DOMESTIC payments support in the DNB connector.
- Added request for account holder name in the Swedbank connector (newly available feature in the Swedbank's API for SE market).
- Samlink (POP Pankki, Säästöpankki, Oma Säästöpankki) added support for getting card accounts
Added
- Added connectors: KBC Ireland.
Fixed
- Fixed PGNR account type handling for DOMESTIC_SE_GIRO payment type in the Nordea connector.
- Fixed payment confirmation in the ICABanken connector to adjust to the upcoming API breaking changes.
- Fixed ID token verification in the AIB connector.
- Small improvements and fixes in the IE integrations (AIB, BOI and UlserBank connectors).
- Fixed handling of continuation key in the SocieteGeneraleParticuliers and Solarisbank connectors.
- Fixed payment initiation in the SEB connector (EE, LT and LV).
- Authentication fix in the Resurs connector (FI).
- Handling expired refresh token error in the Qonto connector.
- Multiple fixes and improvements in the CheBanca and LCL connectors.
- Fixed transaction amount parsing in the AmExNew connector.
- Fixed handling of "deleted" accounts in the SEBSweden and SEBSwedenBusiness connectors.
- Switched to SEPA payments v2 in DNB.
Removed
- Removed OnvistaBank connector.
0.13.1 - 2022-11-03
Changed
- Production environment enabled for the following connectors: CreditMunicipal, Islandsbanki, LazardFreresBanque, MilleisBanque, NeuflizeOBC, OrangeBank.
- AIS beta flag removed from BBVA, BancoSantander, Bankinter, CaixaBank, CajaRural, CajamarCajaRural, DeutscheBankBusinessES, DeutscheBankES, OpenBank, PayPal, Qonto and Sabadell connectors.
- Changed ASPSP ID in the KeytradeBank connector.
Added
- Added
entryReference
for Atruvia, CIBBank, Handelsbanken and HandelsbankenBusiness connectors. - Support of
requestedExecutionDate
field (standing orders) for DanskeBank, Nordea and NordeaBusiness connectors. - Retrieval of additional transaction details for DjurslandsBank, DjurslandsBankBusiness, JyskeBank, JyskeBankBusiness, Kreditbanken, KreditbankenBusiness, Landbobank, LandbobankBusiness, NordfynsBank, NordfynsBankBusiness, SEBSweden, SEBSwedenBusiness, SkjernBank, SkjernBankBusiness, SparekassenSjaellandFyn, SparekassenSjaellandFynBusiness, Sydbank, SydbankBusiness.
- Added connectors: AmExNew, BPCE (access to banks of the Banque Populaire group and Caisse d'Epargne in France), BOI, UlsterBank, DeutscheBankLU.
- Pisp integration: ING, Rabobank, AXA, BpostBank, Comdirect, Commerzbank, CreditEuropeBank, CreditMunicipal KeytradeBank, MilleisBanque, Nagelmackers, NeuflizeOBC, OrangeBank, VDKBank
Fixed
- Fixed
getPaymentRequest
unable to fetch payment status for POPPankki, OmaSp and Saastopankki connectors. - Expired consent handling improved for the folloing connectors: BNPParibasFRBusiness, SiauliuBankas2, Sparkasse.
- Multiple fixes and improvements in the AIB and CreditAgricoleFR connectors.
- Fixed transactions retrieval problem in the SocieteGeneraleEntreprises, SocieteGeneraleParticuliers and SocieteGeneraleProfessionnels connectors.
- Fixed handling of PHOTO_OTP for the Atruvia connector (access to the banks of the German Cooperative Financial Group and some other banks in Germany).
- Fixed certificate loading for the MagNetBank connector.
- Fixed authorisation handling for CIBBank and CIBBankBusiness connectors.
- Meta data information fixes for DOMESTIC_SE_GIRO for the Nordea and NordeaBusiness connectors.
- Fixed SEPA payment initiation for OP connector in case the amount has only one decimal place.
- Transaction fields mapping improvements in the PayPal connector.
- Fixed duplicated transactions issue in the AionBank2 connector.
- Fixed transaction creditDebitIndicator in the TriodosBank connector.
- Fixed request signing in the LCL connector.
- Fixed auth URL in the CreditEuropeBank connector.
- Fixed accounts list retrieval in the Landsbankinn connector.
Removed
- Removed Bankia and Bankoa connectors (due to merge of the banks with other banks).
- Removed AionBank connector, because the old APIs were shut down by the bank; AionBank2 connector is to be used.
0.13.0 - 2022-10-11
Changed
- Base connector implementing integration towards APIs following Open Banking UK specification now
maps
Data.Account[].Description
maps toproduct
andData.Account[].Nickname
todetails
(this mapping was missing). This changes behaviour for Revolut, Wise, S-Pankki and a few more integrations.
Added
- [Java] Added a method
protected KeyStore getKeystore()
inDefaultPlatformExtension
which can be overridden with custom keystore. - Added
validatePaymentRequest
method inPispApi
class. It has exactly the same method signature and does the same validation as inmakePaymentRequest
without making calls to a bank. - MetaApi.getConnectors added optional parameter
connector
, which allows to filter connectors by name. - If maximum daily access exceeded then the
RateLimitException
is raised. All responses will be validated. - Added connectors: Abanca, Addiko, AIB, BKSBankSI, BNPParibasFRBusiness, BNPParibasFRPersonal, BRD, CreditAgricoleFR, DBS, DelavskaHranilnica, FerratumBank, GBKR, HelloBankFR, ISPSlovenia, LCL, LON, NLB, NovaKBM, PayPal, PHV, RaiffeisenHU, SKB, SparkasseSI, Takarekbank, VUBBanka, BPCE, BredBanquePopulaire.
- Added method for getting account details (
/accounts/{accountResourceId}
) - New cashAccountType OTHR added to handle cases when it's not possible to reliably determine type of a payment account.
- Added sepa payments for ABNAMRO.
Fixed
- Decoupled authentication for Swedbank and SwedbankBusiness connectors.
- Added retry for SEBSweden retrieval of signing basket status.
- Token refresh in Swedbank connector for getPaymentRequest.
- In Swedbank connector Swish phone numbers removed from bankTransactionCode and moved to [creditorAccount|debtorAccount].other.
- Balance types in OP connector.
0.12.0 - 2022-07-25
Changed
- Access model data structure: fields
balances
andtransactions
are now boolean. ThetransactionsLimitDays
field is moved to the top level. Thepayments
filed is renamed topaymentInitiation
and it is now boolean as well. AccountResource.name
is now optional. Previously this field was populated with empty string if it was not provided by a bank.
Added
- Added support for reference number in payments. Added
referenceNumberSupported
meta flag.true
if an ASPSP supports reference number handling. In caseremittanceInformationRequired
istrue
andreferenceNumberSupported
is true, eitherreferenceNumber
orremittanceInformation
can be provided. In case bothreferenceNumber
andremittnceInformation
are provided in a credit transfer transaction and reference number is supported by an ASPSP, the reference number will be used. - Reference number support for the connectors: DanskeBank, Handelsbanken, HandelsbankenBusiness, Nordea, NordeaBusiness, OP.
- Added connectors: ArionBanki, UBS, BAWAG, RaiffeisenCZ, DinersClub, VolkswagenBank, BuddyBank, Berenberg, Boursorama, CheBanca, Sloarisbank, AionBank2, Ellou, CIBBank, RaiffeisenIT, BancaMediolanum, CSOBCZ, CSOBSK, Tatrabanka, RaiffeisenSK, BNPParibasFR.
- Added tppName to connectorSettings.
- Added DOMESTIC_SE_GIRO payment type for Swedish Domestic Giro payments. (Added support for Giro payments for following connectors: DanskeBank, SEBSweden, Swedbank, Lansforsakringar, ICABanken, Nordea, Alandsbanken, Handelsbanken)
- Added
validatePaymentRequest
method inPispApi
class. It has exactly the same method signature and does the same validation as inmakePaymentRequest
without making calls to a bank. - Added BancaCarige, BancaPassadore, BancoBPM, BancaPopolareDiSondrio, Findomestic, Banca5, CreditoEmiliano, BNL, BPERBanca, CreditAgricoleIT, IccreaBanca, UnipolPay, BancaPopolareDellAltoAdige, BancaCredifarma, BancaRegionaleDiSviluppo, AllianzBankFinancialAdvisors, Mooney, IgeaDigitalBank, MPS, NEXI, POSTEPAY, CheBancaCorporate, IntesaSanpaolo, BancaFideuram, IntesaSanpaoloPrivate connectors.
Fixed
- Account holder name for following connectors: BankData, Samlink, TietoEvry.
- Cut-off times handling for SE DOMESTIC payments in SEBSweden connector.
- Passing credentials to getAuth and makePaymentRequest (not converting null to empty string and handling special characters correctly).
Removed
- LuminorFallback connector
0.11.1 - 2022-05-16
Added
- Meta about remittance information lines of the credit transfer transaction.
- BIC codes added for some ASPSPs integrated earlier.
- New connectors: UniCreditBankAustria, UniCreditBankCZSK, Beobank, CIC, CreditMutuel, TomamosImpulso, Monabanq, Creatis, BanqueTransatlantique, BanqueEuropeenneDE, BanqueEuropeenneFR, CICBanquePrivee, DNBBusiness, DKB, Santander
- Transaction.credtorAgent and Transaction.debtorAgent fields.
Fixed
- Confirmation handling for SE domestic payments.
- Payment initiation with existing tokens for SEBSweden and SEBSwedenBusiness connetors.
Removed
- Banxe connector removed
- Nordnet connector
- Platform.encryptWithCert method
- AuthInfo.authData field
0.11.0 - 2022-04-14
Changed
Access
structure is updated -- addedpayments
field to specify access to paymentsgetCurrentConsent
doesn't raiseCoreException
when consent ID is not set, just returnsConsent
data structure with nullconsentId
field.
Added
- Platform method
encryptWithCert
, which encrypts provided data with public certificate refreshToken
parameter to all connectors constructors- Handling connector refreshToken in makePaymentRequest in makePaymentRequestConfirmation in the connectors: ICABanken, Lansforsakringar, Nordea, SEBSweden, Swedbank
- Interface of
modifyConsents
function is changed: addedstate
parameter and addedenv
response field. - New connectors: AionBank, AmEx, Atruvia, BankDeKremer, BankVanBreda, BanqueCPH, BpostBank, Comdirect, CreditAgricolePoland, CreditEuropeBank, CreditMunicipal, DeutscheBankBE, DeutscheBankBusinessES, DeutscheBankBusinessEU, DeutscheBankBusinessIT, DeutscheBankDE, DeutscheBankES, DeutscheBankIT, Europabank, IkanoBankDK, KeytradeBank, LazardFreresBanque, MilleisBanque, MillenniumBank, Nagelmackers, NeuflizeOBC, Norisbank, OnvistaBank, OrangeBank, Postbank, Sparkasee, VDKBank, VanLanschot, RaiffeisenBank.
Fixed
- Improved data mapping for ING connector.
- Improved handling of expired refresh tokens.
0.10.5 - 2022-02-28
Changed
- DanskeBank connector - setting default charge bearer and instruction priority for SEPA payments.
- [JS][Python] Internal changes related to asynchronous code execution. Platform methods
signWithKey
andloadPem
are now also asynchronous. CountryAuthInfo.defaultMethod
changed toCountryAuthInfo.hiddenMethod
with inverted value.- Commerzbank production connector (DE).
- Sparkasse production connector (DE).
Fixed
- SEBKortBank and SEBKortBankBusiness connectors - outdated endpoints replaced, accounts parsing.
- CoopPank, SignetBank, PrivatBank and MeridianBank connectors - transaction data mapping fixes.
- BancoSantander - fixed issue with PSU-Http-Method.
- TriodosBankBE and TriodosBankNL connectors - correct domain in the authentication URL.
- Nordea and NordeaBusiness connectors - parseRedirectUrl returns error and error_description.
- Alior - it is now possible to request access for all accounts without specifying them. Removed the need to use modifyConsent in AIS flow.
- Holvi connector supports masked IBANs.
Added
- Wise connector AISP.
- Islandsbanki connector.
- Commerzbank connector (DE).
- HypoVereinsbank connector (DE).
- Added
DOMESTIC
payment type for Swedish connectors: Nordea, Handelsbanken, ICABanken, DanskeBank, Swedbank, Lansforsakringar. - Payment type meta flag
creditorAgentClearingSystemMemberIdRequired
. - Added
DOMESTIC
payment type for Swedish connectors.(Nordea, Handelsbanken, ICABanken, DanskeBank, Swedbank) - Rocker connector.
- ST1Finance connector.
- OPBalticsEE connector.
- OPBalticsLV connector.
- OPBalticsLT connector.
- Nordnet connector AISP.
MIBN
scheme name for masked IBANs.
Removed
- SallingBank connector (the bank announced merger with Sparekassen Vendsyssel).
- DenJyskeSparekasse connector (the bank announced merger with Vestjysk Bank A/S).
- OPBaltics connector (EE, LV, LT).
0.10.4 - 2022-01-27
Added
- Added public method
getSDKVersion
to theApiClient
class which returns current version with hash appended. (e.g. "0.10.4-abcdef0") - Personal code and company code verification for LHV and LHVBusiness; Added handling of "CONSENT_REPLACED" error.
- Added automatic token refresh in PISP flow.
- Added MarginalenBank connector (SE).
- Enabled Andbank and BancoInversis connectors.
- SBAB - Added handling of KYC error.
- BankNorwegian - added handling of invalid consent error.
- Revolut - added handling of psu type;
- Added properties
regulatoryReportingCodes
andregulatoryReportingCodeRequired
into payment type meta information. - In Handelsbanken and ICABanken connectors added the possibility to pass regulatory reporting codes for SEPA payment type in Sweden.
- Citadele payments.
- Added Meta.betaAisp flag showing whether connector's AISP implementation is in beta state
- Added Meta.betaPisp flag showing whether connector's PISP implementation is in beta state
- Added TriodosBank connector (BE, GB, NL)
- Added Crelan connector (BE)
- Personal code and company code verification for SEB and SEBBusiness
- Personal code verification for Luminor
Changed
- Upgraded SEBSweden accounts API version from v6 to v7
- LHV connector split into two: LHV and LHVBusiness.
- Improved error handling for BerlinGroup connectors; improved mapping of current account.
- Improved flow for SEBSweden payments.
- Upgraded Citadele accounts API version to v9.
- Swedbank connector uses Swedbank's AIS and PIS APIs v4 instead of v3 APIs retiring in January 2022.
- Field
regulatoryReportingCodes
of theCreditTransferTransaction
data structure has been changed toregulatoryReporting
, which is an array ofRegulatoryReporting
elements containing extended information necessary for passing regulatory reporting details. - Nordea connector - using single SCA method for SEPA payments in Finland.
- SEB connector split into two: SEB and SEBBusiness
Fixed
- ICABanken connector - handling payment initiation "Due Date has passed" error occurring on weekends.
- SantanderBankPolska AISP fixes and improvements.
- Lansforsakringar connector - extracting local account number from debtorAccount BBAN.
- Fixed account consent creation for Spanish banks by Redsys provider. Also, improved header generation logic.
- TriodosBank - fixed issue with token creation.
- BNPParibasPoland - fixed issue with token refresh; fixed issue with missing client id.
- SEBKortBank - fixed available authorization methods.
- Polish banks - improved mapping for merchant category code; fixed entry reference mapping.
- Pekao - fixed issue with missing default values on transactions request.
- Volvofinans - fixed issue with missing content type on accounts request.
- Mbank - fixed issue with account resource id; fixed validUntil issue.
- Alandsbanken - fixed debtor account schema in meta information.
- Argent - sandbox fixes
- SEBSweden connector - fixed makePaymentRequestConfirmation for SEPA payment type and migrated to PIS API v7.
- Citadele - fixed issue with dateFrom parameter not being set.
- Refresh token handing in getPaymentRequest for ICABanken, Lansforsakringar, SEBSweden and Swedbank connectors.
Removed
- Lansforsakringar connector - IBAN has been removed from the list of supported debtorAccountSchemas as in fact it is not supported by the bank (an error occurs during payment confirmation).
0.10.3
Added
- Added Knab connector (NL), Sparebanken Vest connector (NO).
- [Java] Added BrokerPlatformExtension for offload of cryptographic functionality (mTLS & signing) to Open Banking eIDAS Broker
- Added debtorContactEmailRequired and debtorContactPhoneRequired properties to payment type meta information.
- Authentication method MOBILE_ID added to connector SEBSweden to provide the possibility of decoupled authentication using Mobile BankID. This method is not allowed for PISP.
- Transliteration of extended latin characters in creditor details and remittance information during payment initiation for FI, NO and SE connectors.
- Added validation for creditor bicFi in
makePaymentRequest
. - Added PsuActionRequiredException for cases when there are actions required from an end user.
Changed
- Improved exceptions inheritance (HttpException is now a subclass of ResponseValidationException)
Fixed
- DanskeBank connector - Fixed
makePaymentRequestConfirmation
not returning payment status; transliteration of text fields in payment resource. - DNB connector - skipping PSU-Accept header, which may contain unsupported characters; using default debtor BIC; improved payment status mapping.
- Handelsbanken connector - extracting "kontonummer" from BBAN; clearing up postalAddress by replacing extended latin characters and removal of unallowed symbols; state for payment authorization.
- ICABanken connector - Setting priority "SEPA" in case of the "SepaCreditTransfers" payment product,
using signing basket in
makePaymentRequestConfirmation
and refresh tokens forgetPaymentRequest
. - Lansforsakringar connector - Fixed
getPaymentRequest
causing an error in case of calling it beforemakePaymentRequestConfirmation
; Added creditorbicFi
anddebtorCurrency
as a required fields inpaymentRequest
. - SpareBank1 connector - API origin changed for SpareBank1 Helgeland.
- Sbanken connector - List of accounts fix.
- Swedbank connector - normalizing IBAN when making payment request confirmation.
Now in this case it returns null
paymentInformationStatus
. - Fixed an issue where calling
getPaymentRequest
would cause an error if payment has not been initiated on bank's side yet.
0.10.2
Added
- Added payment status code
ACCC
(AcceptedCreditSettlementCompleted, Settlement on the creditor's account has been completed) added into thePaymentInformationStatusCode
enum. - Added optional parameters
credentials
andmethod
tomakePaymentRequest
function in order to provide the possibility to use different payment authorization methods and pass credentials provided by a PSU. - Added optional field
authData
(possibly containing information necessary for PSU to complete payment authorisation) toHalPaymentRequestCreation
data structure. - Added
signKeyPath
andsignPubKeySerial
parameters to the Swedbank connector. Those parameters are used in the payments flow. - Added support of SEPA payment type for ICABanken connector.
- Added Skandia connector.
- Added CARDREADER authentication method for Handelsbanken and HandelsbankenBusiness connectors, because it required for authorization of SEPA payments.
- Added the
contactDetails
field into thePartyIdentification
data structure.
Changed
- The fields
paymentInformationStatus
andstatusReasonInformation
has been moved to the top level ofHalPaymentRequest
data structure in order to makePaymentRequestResource
data structure only as an input data structure for payment initiation. - The field
paymentTypes
of payment meta data structureCountryPaymentTypes
is changed to be an array ofPaymentTypeInfo
elements describing each payment type available for the country.
Removed
- Removed field
appliedAuthenticationApproach
fromHalPaymentRequestCreation
data structure returned frommakePaymentRequest
function. - Remove optional field
paymentRequest
fromPaymentRequestConfirmation
data structure passed tomakePaymentRequestConfirmation
function as unused. - Removed fields
resourceId
,creationDateTime
,initiatingParty
,paymentInformationStatus
,statusReasonInformation
,fundsAvailability
andbooking
fromPaymentRequestResource
data structure as unused.
Fixed
- Various account information fetching fixes and improvements in BNPParibasFortis, Resurs, SEBKortBank, SEBKortBankBusiness and Volvofinans.
- Decouple authentication flow in SBAB connector.
- Refresh token handling in PKO connector.
- [Java] Fixed empty response handling.
0.10.1
Added
- Production connectors for banks in Belgium: AXA, Belfius, KBC
- Production connectors for banks in Denmark: AlmBrand, AndelskassenFaelleskassen, ArbejdernesLandsbank, BILDanmark, CoopBankDenmark, DanskeAndelskassersBank, DenJyskeSparekasse, DjurslandsBank, FasterAndelskasse, FrorupAndelskasse, FroslevMollerupSparekasse, FynskeBank, HandelsbankenDenmark, HvidbjergBank, JyskeBank, Kreditbanken, LaegernesBank, Landbobank, LollandsBank, MajBank, MerkurAndelskasse, MonsBank, NordfynsBank, NykreditBank, PenSam, SEBDenmark, SallingBank, SkjernBank, SparNordBank, SparekassenSjaellandFyn, SwedbankDenmark, Sydbank, Totalbanken, VestjyskBank.
- Production connectors in Lithuania: Paysera
- Production connectors for banks in Netherlands: ABNAMRO, Rabobank, ING, INGWholesaleBanking
- Production connectors for banks in Norway: Komplett, Ya
- Production connectors for banks in Poland: PKO, Pekao, INGPoland, BNPParibasPoland, Alior
- Enabled business psuType for OmaSp and POPPankki connectors.
- Added
approach
field to theCountryAuthInfo
model. This field displays used authentication approachREDIRECT
orDECOUPLED
to help choose suitable authentication method.
Fixed
- Various bugfixes for connectors: AXA, Alior, Argenta, BIB, Belfius, Citadele, DNB, Handelsbanken, Holvi, ING, KBC, Lansforsakringar, Luminor, MedicinosBankas, Nordea, OP, Rabobank, Revolut, SEBSweden, SiauliuBankas, Swedbank
- [Python] Fixed issue with request urlencoding in async version.
- Fixed issue with length restriction in AccountResource.product
0.10.0
Added
- Norwegian and Swedish banks: BN Bank ASA, Etne Sparebank, Fana Sparebank, Flekkefjord Sparebank, Handelsbanken, Haugesund Sparebank, KLP Banken, Landkreditt Bank AS, Lillesands Sparebank, Luster Sparebank, Nordea Direct, OBOS-banken AS, OKQ8, Pareto Bank ASA, Sbanken, Skudenes & Aakra Sparebank, SpareBank 1 BV, SpareBank 1 Gudbrandsdal, SpareBank 1 Hallingdal Valdres, SpareBank 1 Helgeland, SpareBank 1 Lom og Skjåk, SpareBank 1 Modum, SpareBank 1 Nord-Norge, SpareBank 1 Nordvest, SpareBank 1 Ringerike Hadeland, SpareBank 1 SMN, SpareBank 1 SR-Bank, SpareBank 1 Søre Sunnmøre, SpareBank 1 Telemark, SpareBank 1 Østlandet, Sparebanken Møre, Sparebanken Sogn og Fjordane, Sparebanken Sør, Sparebanken Øst, Spareskillingsbanken, Swedbank, Søgne og Greipstad Sparebank, Voss Sparebank, DNB.
- [Python] Asynchronous version of the library.
Changed
- Removed field
bicFi
fromAccountResource
and added new fieldaccountServicer
containingbicFi
. - Major internal refactoring providing the possibility to use async/await syntax in the core.
- BREAKING CHANGE:
code
parameter inAuthApi.make_token
method is now optional - [Python] cryptography library version is updated to the latest 3.4.7
Fixed
- Holvi: continuation key internal behaviour
- OP: default consent transactions validity period now calculated properly
- Länsförsäkringar: fixed method
getAccountTransactions
- Other fixes related to returned fields
0.9.2
Added
- Setting
business
added to SEB connector in order to support different authentication URLs. - Added
Meta.transactionsInfo
field with bank-specific meta information related to transactions. - Improved IBAN validation.
- Possibility to fetch over 3 months old transactions in Swedbank connector.
- Added
formatTimestamp
method into all platforms.
Fixed
- Improved fields mapping in ICABanken connector.
- Handelsbanken and HandelsbankenBusiness connectors passing correct content-type header when sending authorization requests.
- Improved logic for setting toBookingDateTime in connectors to API following UK OB standard.
- [Java] Including jakarta.xml.bind and org.glassfish.jaxb into the build in order to support javax.databind depreciated in 9, 10 and removed in 11+
0.9.1
Added
- HandelsbankenBusiness connector and NL support for Handelsbanken connector.
- [Javascript] Possibility pass connector settings as an object with arbitrary order of the keys when initializing ApiClient.
- Improved error handling for connectors to APIs based on Berlin Group NextGenPSD2 specification.
Changed
- Connector setting
business
removed from Nordea and NordeaBusiness as unused. - Optional connector setting
psuId
removed from Handelsbanken.
Fixed
- Access handling in Swedbank connector.
- Decoupled authentication in Holvi connector.
- User ID pattern for Sweden.
0.9.0
Added
- Breaking change: Added support for different kinds of user credentials in
getAuth
(e.g.personalCode
,phoneNumber
,companyId
. Previously onlyuserId
andpassword
were supported). Added support for selection of authentication methods (for banks which support that). Because of that changed method signature forgetAuth
. Now it acceptscredentials
parameter (array of strings of user credentials) andmethod
(selected authentication method).userId
andpassword
were moved tocredentials
. Updated meta information reflecting these changes: added fieldcredentials
toAuthInfo
structure, if it is present then user credentials are required by the bank to log in and this field contains info about required credentials. - New connectors: BNBank, EtneSparebank, FanaSparebank, FlekkefjordSparebank, HandelsbankenNorway, HaugesundSparebank, HelgelandSparebank, KLPBanken, LandkredittBank, LillesandsSparebank, LuminorFallback, LusterSparebank, NordeaDirect, OBOSBanken, OKQ8Bank, ParetoBank, Sbanken, SkudenesAakraSparebank, SogneOgGreipstadSparebank, SpareBank1, SparebankenMore, SparebankenOst, SparebankenSognogFjordane, SparebankenSor, Spareskillingsbanken, SwedbankNorge, VossSparebank
- Added Transactions.referenceNumber field.
- Added new
state
parameter tomakePaymentRequest
method.
Changed
- Removed
Token.token_type
field - Removed
paymentAuthRedirectUri
andpaymentAuthState
parameters from most connectors.
Fixed
- Fixed UTC handling in JS SDK.
- Fixed
continuationKey
andremittanceInformation
fields ingetAccountTransactions
for OP connector. - Fixed handling of
referenceNumber
in Berlin Group connectors. - Fixed Handelsbanken redirect flow token issue.
0.8.1
Added
- New connectors: Rabobank NL, ABNAMRO NL, BNG NL, BNPParibasFortis BE (including Fintro and Hello Bank brands), DNB NO, KBC BE (KBC and CBC brands), AXA Bank, Belfius BE
- Added new method in meta connector settings
getSupportedPsuTypes
which returns array of supported account types (personal
,business
). - Support of business accounts for Nordea is moved into separate connector NordeaBusiness.
- TransactionPeriodError which is raised when wrong account transactions period is requested.
- Added fields
userIdFormat
anduserIdDescription
to the MetaApiAuthInfo
model. - Added
error
field to theAuthRedirect
model.
Fixed
- Fixes and improvements for production connectors (LHV, Nordea, ICABanken, Citadele, SEBSweden).
- General improvements for exception handling in the SDK.
0.8.0
Added
- Added parameter reference in
ParameterValidationException
, field and path references inModelValidationException
- [Java] Added getters for private fields for exceptions.
- [Java] Platform method
loadPEM
now can load certificates from any filesystem path (previously was loading only from resources). - Platform method
makeRequest
now has additional optional argumentfollowRedirects
(defaults totrue
). - Mock connector
MockASPSP
designed for testing common AISP flows with two countries - SE (mock decoupled flow with token polling and consent request required before callinggetAccounts
) and FI (mock redirect flow) - Production connectors for Revolut, LHV, Citadele, CoopPank, Swedbank LT
Changed
- Internal refactoring of SPankki, Alandsbanken, Resurs, Ya and Komplett connectors.
- Improved production integrations for SEB LT, Handelsbanken SE
- Removed
ClientInfoValidationException
(replaced withModelValidationException
)
0.7.1
Added
- New platform method
loadPEM
. It is responsible for loading PEM content into SDK. Overload it if you want to load your certificate from database/certificate storage.
Changed
- [Python] cryptography library version is updated to the latest 3.2.1
signCertString
connector settings is changed tosignCertPath
(Platform now properly loads its content thanks to new platform methodloadPEM
)- Changed interface of Platform.verifySignature:
certPath
parameter replaced withcert
parameter, accepting JWK class instance with two fields ("e" and "n").
Fixed
- Fixed
makeToken
issue for production Lansforsakringar connector. Added support for refresh token. - Removed
appId
connector setting in Handelsbanken connector.
0.7.0
Added
- Production connectors for SBAB, Lansforsakringar.
- Added
PSU-GEO-Location
validation. Expected input format for this header: comma separated latitude and longitude coordinates without spaces. (WithoutGEO:
prefix.)
Fixed
- Fixed authentication scopes and account transactions negative amount value for SEBSweden connector.
- Nordea appropriate error thrown (Unauthorized) if access token is expired
- Revolut fixed and improved account transaction fields mapping
Changed
- [Python] cryptography library version is updated to 3.1
- Breaking change: Internal improvements of handling Access model. Default values for Access.transactions.statuses and Access.balances.statuses are null. Widest possible consent requested by default.
0.6.6
Added
- ClientInfoValidation error is raised when a required client information is not set before sending requests to ASPSP.
- Production connector for SEB (Baltics)
Fixed
- Using correct value for Access.validUntil in Nordea connector.
- [Javascript] Fixed how core exceptions are rejecting async calls.
- Fixed makeToken issue in decoupled flow for Handelsbanken.
0.6.5
Added
- Revolut domestic payments
- Support for Handelsbanken SE decoupled authentication
- Sweden production connectors for Alandsbanken, DanskeBank, Handelsbanken, SEBSweden
Fixed
- Consent JWT payload for connectors SPankki, Alandsbanken, Resurs, Ya, Komplett
0.6.4
Added
- Parameter
brand
added to Swedbank connector settings. List of supported brands is available through the connector's meta.
Fixed
- Production API domain changed in DanskeBank connector.
0.6.3
Fixed
- Updated Swedbank connector to use v3 version of api.
- Generation of
Date
header is fixed in SEB connector.
0.6.2
Added
- Added PS signature algorithm verification in
Platform.verifySignature
method. Added optionalcryptoAlgorithm
parameter toPlatform.verifySignature
method.
Fixed
- Fixed Danske bank jwt signature algorithm and JWT fields
- [Python] Updated dependency library
cryptography
- [JavaScript] Updated ApiClient and Platform classes to conform other languages
0.6.1
Added
- Improved exception handling: now there are a lot less CoreExceptions raising. Improved exception messages in many cases.
- Added several new types of exceptions: ParameterValidationException, ModelValidationException, ResourceNotFoundException, InsufficientScopeException, InvalidResponseSignatureException, InvalidResponseIdException.
- Added validation for certificate/key paths.
- Production connectors for Holvi and Aktia Contingency API.
getAuth
returns an additional field for AktiaContingency connector -authData
that containsotpIndex
of code which user should pass tomakeToken
.- Connectors meta authInfo now has two additional fields:
passwordRequired
andauthData
. First shows if password is required ingetAuth
call, and second shows ifauthData
is returned ingetAuth
.
Fixed
- Fixed transaction fields mapping in Nordea, SPankki, DanskeBank and OP connectors.
- Fixed transaction amount mapping for Berlin Group connectors.
- Fixed incorrect headers in sandbox for POPPankki, OmaSp and Saastopankki connectors.
Changed
getAuth
now has optional parameterpassword
which is required for Holvi and AktiaContingency.
0.6.0
Added
- Added transaction pagination. To get next page of transaction, pass
HalTransactions.continuationKey
parameter togetAccountTransactions
.getAccountTransactions
other parameter must be the same as in the original call.continuationKey
is null if there are no more pages of transactions or if pagination is not supported by connector. - Production connectors for Finnish banks: Nordea, OP, POP Pankki, S-Pankki, Ålandsbanken, Resurs Bank, Handelsbanken, Säästöpankki, Oma Säästöpankki, Danske Bank.
- Field
brands
, containing list of end-user recognizable ASPSP's brands, added intoAuthInfo
data structure returned within connectors' meta. - CBPII API containing makeFundsConfirmation method added to support functionality needed for card-based payment instrument issuers.
- New connectors: Banxe, SantanderConsumerBankNorway
Fixed
- Fixed mappings for production connectors.
- Removed
sessionDuration
from Nordea connector settings, now usesAccess.validUntil
attribute instead. (passed togetAuth
) - Negative balance amount for banks using Open Banking UK API standard.
- Transaction filtering by date fixed for Alandsbanken, SPankki, Resurs, Danske connectors.
- Fixed setting session validity period (consent) for SPankki, Alandsbanken, Resurs, Danske, OmaSP, POPPankki, Saastopankki and Nordea.
0.5.2
Added
- PSU headers not supported by a connector now will be skipped instead of raising exception.
- [Java] fixed datetime parsing issue in DanskeBank, Alior and Pekao connectors.
- [Javascript] Possibility to initialize ApiClient using settings.
- [Javascript] Platform exposed through enablebanking module object.
- New connectors: AndelskassenFaelleskassen, BILDanmark, CoopBankDenmark, DanskeAndelskassersBank, DenJyskeSparekasse, FasterAndelskasse, FrorupAndelskasse, FroslevMollerupSparekasse, FynskeBank, HandelsbankenDenmark, HvidbjergBank, LaegernesBank, Lansforsakringar, LollandsBank, MajBank, MerkurAndelskasse, MonsBank, NykreditBank, PenSam, SallingBank, SEBDenmark, SparNordBank, SwedbankDenmark, Totalbanken, VestjyskBankArbejdernesLandsbank, Volvofinans.
Fixed
- Several connectors has been fixed (fixes are mostly related to date/time handling).
0.5.1
Added
- [Javascript] Possibility to instantiate model classes with data and support of the native date type.
- [Javascript] Better representation of the errors coming from the connectors.
- Optional parameter
cryptoAlgorithm
added to platform methodsignWithKey
in all platforms in order to enforce usage of a certain algorithm. - Support of
PS256
signature algorithm added on all platforms. - [Java] Added new dependency Bouncy Castle (
bcprov-jdk15on
) to supportPS256
signatures. - New connectors: AlmBrand, Citadele, DjurslandsBank, JyskeBank, Kreditbanken, Landbobank, Luminor, NordfynsBank, Revolut, SDC (NO), SiauliuBankas, SkjernBank, SparekassenSjaellandFyn, Sydbank.
Changed
- Resurs connector now requires
country
setting and in addition to Finland now supports Denmark, Norway and Sweden.
Fixed
- [Java] JDK 9+ compatibility bug is fixed
0.5.0
Added
getAuth
now returns an additional fieldAuth.env
, which contains additional authentication info to be passed tomakeToken
.makeToken
now has a new parameterauthEnv
(value has to be taken fromAuth.env
returned fromgetAuth
).- Field
refreshToken
in connector meta data, displaying refresh token support. - Added new fields to display party account's currency:
PaymentRequestResource.debtorCurrency
andBeneficiary.creditorCurrency
- New connectors: CoopPank, MeridianBank, PrivatBank, SignetBank
Changed
- Breaking change: moved
redirectUri
to base connector settings and removed it from getAuth, makeToken and modifyConsents parameters.redirectUri
is now required parameter for every connector. - Nordea connector settings field
keyPath
tosignKeyPath
in order to maintain consistency for QWAC/QSealC keys naming.
Removed
- Parameters
scope
andresponseType
removed fromgetAuth
. - Nordea connector setting
certPath
removed as QWAC not supported by Nordea.
0.4.4
Added
- Field
requiredPsuHeaders
containing array of mandatory PSU headers added toConnector
data structure. - Fields
modifyConsentsInfo
andauthInfo
containing additional information about usagemodifyConsents
andgetAuth
methods added toConnector
data structure.
0.4.3
Added
- Possibility for revoking PSU consent for several banks.
- Field
consentRevocation
in connector meta data, displaying consent revocation support. - Auth API now provides
setClientInfo
method for setting extra PSU headers such as PSU-IP-Address, PSU-IP-Port, PSU-Geo-Location etc. - [Python] Possibility to initialize
ApiClient
using a connector module imported from a separate package.
Changed
- psuIpAddress parameter removed from ConnectorSettings classes where it was present.
Method
setClientInfo
shall be used instead. - [Python] Platform classes require core module reference to be passed as the first argument of the constructor.
Fixed
- [Java] Top-level null deserialization is fixed.
- Payment request is fixed for Handelsbanken connector.
0.4.2
Added
- Auth API provides
parseRedirectUrl
method for parsing of the parameters returned in the callback URL, which a PSU is redirected to after successful authentication on the ASPSP's website. - [Python] Possibility to use connectors imported from another packages.
- Fields
creditorAccount
anddebtorAccount
added toTransaction
data structure to be able to return information about creditor and debtor accounts in the list of transactions when the information is returned by an ASPSP. - New connectors: DanskeBank, OmaSp and Saastopankki.
- Response signature verification for Pekao bank. If verification is failed then the
InvalidResponseSignatureException
is raised.
Changed
- Validation of
sandbox
setting is unified based on meta information (environment
field). - [Python]
enablebanking.CoreException
is thrown when unable to initialise a connector.
Fixed
- LHV connector fixes and improvements.
0.4.1
Added
- Builders of ConnectorSettings' subclasses now supports any order of parameter chaining.
- Fields
aspspIds
(list of supported ASPSPs identified by ECB's MFI codes) andenvironments
(sandbox/production) are added toConnector
data structure. - [Python] BrokerPlatform used for generating request signatures and making mTLS connections towards APSPSs through eIDAS broker service.
0.4.0
Added
- Optional parameter
hashAlgorithm
added to platform methodsignWithKey
in all platforms. Connector
data structure now has additionalpayments
field containing list of supported payment types (newPaymentTypes
data structure is added).
Changed
- Platform method
signPKCS1v15usingSHA256
renamed tosignWithKey
in all platforms. - Field
PaymentRequestResource.paymentTypeInformation
is used to identify payment type that is going to be executed.
0.3.1
Added
- Base connector for banks implementing PolishAPI on KIR platform.
Changed
- [Python] Capitalization of request headers removed to be able to support API backend with case-sensitive handling of the headers.
- Added Handelsbanken (AISP) connector for Finland and Sweden
- Updated OP connector. Changed connector settings
0.3.0
Changed
-
[Java] UnstructuredRemittanceInformation now uses ArrayList constructor.
-
Parameter
paymentRequestData
of PISP API methodmakePaymentRequestConfirmation
removed and the data is passed insideconfirmation
parameter (PaymentRequestConfirmation
data structure now has an option attributepaymentRequest
).
0.2.1
Added
- Added
DataRetrievalException
andInsufficientScopeException
derived fromHttpException
to represent particular cases.
0.2.0
Added
- [Java] Improved connector settings interface. Added
AbstractConnectorSettingsFactory
class with a factory methodcreateConnectorSettings
to simplify creation of connector settings.ApiClient
now has constructors takingConnectorSettings
instance instead of list of settings objects. - [Java]
AbstractPlatformExtension
class consisting ofsignPKCS1v15usingSHA256
andmakeRequest
methods is added in order to simplify customisation of Java platform. Also addedApiClient
constructor takingAbstractPlatformExtension
as a parameter. Transaction
data structure extended with fieldsbankTransactionCode
,creditor
anddebtor
andBankTransactionCode
data structure added.- Value
refresh_token
is supported forgrant_type
parameter ofmakeToken
method.
Changed
- [Java]
ApiClient
constructors accepting settings as a list of objects andJavaPlatform
class are changed toprotected
. - Changed order of connector settings. Parameters
consentId
,accessToken
andrefreshToken
are now always required (should be set tonull
if not present). - [Java] Standard Java SE 8 date-time classes are used instead of ThreeTen-Backport classes, thus Java SE 6 and 7 support is dropped.
- Field
remittanceInformation
ofTransaction
changed to not required (mainly to support credit card transactions, for which remittance info is usually not provided). Access
data structure changed:balances
andtransactions
fields contain details for requested access andaccounts
field contains list of accessed account IDs.
Fixed
- Field mapping for Open Banking UK standard is improved.
0.1.x
This version was created before the changelog has been introduced.