Introduction
Welcome to Enable Banking SDK developer documentation!
We are happy that you are interested our solution and hope that in this documentation you'll be able to find all information you need when using our SDK. If not, feel free to contact us at hello@enablebanking.com.
Get started by cloning our sample repository
$ git clone https://github.com/enablebanking/OpenBankingJavaExamples
$ git clone https://github.com/enablebanking/OpenBankingJSExamples
$ git clone https://github.com/enablebanking/OpenBankingPythonExamples
Enable Banking SDK provides native Java, Javascript and Python libraries for connecting directly from your code to a number of Open Banking APIs without intermediate aggregation services. The libraries provide unified interface for interacting with multiple ASPSPs (banks), harmonize data and implement all cryptographic functionality needed for interaction with PSD2 APIs, such as mTLS connection using eIDAS QWAC and request signing using eIDAS QSeal certificate.
It's the easiest to get started with the SDK by using our code samples. The complete code samples for Java, Javascript and Python are available under our Github account: https://github.com/enablebanking.
For starting you need to have a developer license, which you can order on our website. You will get your developer SDK, which is connected Open Banking API sandboxes.
For production usage please contact us via e-mail.
User guide
Authorization and user token refresh
This section describes a basic authorization flow and access token refreshing flow (if it is supported by an ASPSP).
In order to execute this flow correctly for a specific ASPSP (bank) connector, one should obtain connector's meta information and build your flow according to that information.
Below is the basic example of how such flow can be implemented.
0. Import library and choose connector
import com.enablebanking.ApiClient;
import com.enablebanking.api.AuthApi;
import com.enablebanking.api.MetaApi;
import com.enablebanking.model.*;
// Initializing meta interface.
MetaApi metaApi = new MetaApi(new ApiClient());
// Getting connectors for a country.
HalConnectors connectors = metaApi.getConnectors("FI");
// Finding meta data for a desired connector
Connector connectorMeta = null;
for (Connector conn : connectors.getConnectors()) {
if (conn.getName().equals('Sample')) {
connectorMeta = conn;
break;
}
}
const enablebanking = require('enablebanking');
// Initializing meta interface.
const metaApi = new enablebanking.MetaApi(new enablebanking.ApiClient());
// Getting connectors for a country.
const connectors = await metaApi.getConnectors({country: 'FI'});
// Finding meta data for a desired connector
let connectorMeta;
for (const conn of connectors.connectors) {
if (conn.name == 'Sample') {
connectorMeta = conn;
break;
}
}
import enablebanking
// Initializing meta interface.
meta_api = enablebanking.MetaApi(enablebanking.ApiClient())
// Getting connectors for a country.
connectors_info = meta_api.get_connectors(country='FI')
// Finding meta data for a desired connector
connector_meta = None
for conn in .connectors:
if conn.name == 'Sample':
connector_meta = conn
break
First of all we are getting the list of connectors for a country and choosing one connector, which we are going to used. In real-world case a list of banks accessed with the connectors, shall be shown to a user for making a choise. In the code sample we are just using 'Sample'.
1. Initialize API client and authentication interface
// Initializing settings; connector settings class to be replaced with a real one.
ConnectorSettings settings = new SampleConnectorSettings()
// other settings, including callback redirect URI, to be set here
.sandbox(true);
// Creating client instance.
ApiClient apiClient = new ApiClient(settings);
// Initializing authentication interface.
AuthApi authApi = new AuthApi(apiClient);
// Creating client instance; connector name to be replaced with a real one.
const apiClient = new enablebanking.ApiClient('Sample', [
true, // sandbox
/* other settings, including callback redirect URI, to be set here */
]);
// Initializing authentication interface.
const authApi = new enablebanking.AuthApi(apiClient);
// Creating client instance; connector name to be replaced with a real one.
api_client = enablebanking.ApiClient(
"Sample",
{
"sandbox": True,
# other settings, including callback redirect URI, to be set here
})
// Initializing authentication interface.
auth_api = enablebanking.AuthApi(api_client)
After the choise has been made, we need to initialize API client for the chosen connector and create the authentication interface.
Connector settings (i.e. set of parameters used when initializing API client) differ from one connector to another. Description of settings for every connector can be found here.
2. Initiate user authentication
// Checking if user ID is required when initiating user authentication.
String userId = null;
if (connectorMeta.getAuthInfo().get(0).getInfo().isUserIdRequired()) {
// Setting userId; in real case it is coming from a user.
userId = "someUserId";
}
// Checking if user consent can be received while user authentication.
Access access = null;
if (connectorMeta.getAuthInfo().get(0).getInfo().isAccess()) {
// Initializing access, i.e. scope of access, which user needs to consent with.
access = new Access(/* user consent parameters */);
}
// Initiating user authentication
Auth auth = authApi.getAuth(
"test", // state returned to callback redirect URI
userId,
access);
// Sending user to this URL for authentication
String authUrl = auth.url;
// Checking if user ID is required when initiating user authentication.
let userId;
if (connectorMeta.authInfo[0].info.userIdRequired) {
// Setting userId; in real case it is coming from a user.
userId = "someUserId";
}
// Checking if user consent can be received while user authentication.
let access;
if (connectorMeta.authInfo[0].info.access) {
// Initializing access, i.e. scope of access, which user needs to consent with.
access = new Access(/* user consent parameters */);
}
// Initiating user authentication
const auth = await authApi.getAuth({
state: 'test', // state returned to callback redirect URI
access: access,
userId: userId
});
// Sending user to this URL for authentication
const authUrl = auth.url;
# Checking if user ID is required when initiating user authentication.
user_id = None
if connector_meta.auth_info[0].info.user_id_required:
# Setting userId; in real case it is coming from a user.
user_id = "some_user_id"
# Checking if user consent can be received while user authentication.
access = None
if connector_meta.auth_info[0].info.access:
# Initializing access, i.e. scope of access, which user needs to consent with.
access = enablebanking.Access(
# user consent parameters
)
# Initiating user authentication
auth = auth_api.get_auth(
state='test', # state returned to callback redirect URI
user_id=user_id,
access=access)
# Sending user to this URL for authentication
url = auth.url
Some connectors require user ID to be set when initiating user authentication. This information is available in connector meta and user ID shall be requested from a user.
Also scope of access, which user needs to consent with, shall be set. It will be used if user consent can be received while user authentication. If not, modify consents shall be used later on. By default widest possible scope will be requested.
And after the parameters are set, user authentication shall be initiated.
3. Obtain authorization code and get an access token
// Extracting data from the URL where user was redirected to after authentication.
AuthRedirect redirectInfo = authApi.parseRedirectUrl("https://your.domain/callback");
// Obtaining user access token (and possibly refresh token as well).
Token token = authApi.makeToken(
"authorization_code", // grant type
redirectInfo.code, // authorization code
auth.env // authentication environment
);
// Extracting data from the URL where user was redirected to after authentication.
AuthRedirect redirectInfo = authApi.parseRedirectUrl('https://your.domain/callback');
// Obtaining user access token (and possibly refresh token as well).
const token = await authApi.makeToken(
'authorization_code', // grant type
redirectInfo.code, // authorization code
auth.env // authentication environment
)
# Extracting data from the URL where user was redirected to after authentication.
redirect_info = auth_api.parse_redirect_url("https://your.domain/callback")
# Obtaining user access token (and possibly refresh token as well).
token = auth_api.make_token(
"authorization_code", # grant type
code, # authorization code
auth.env)
After the user has authenticated (and is redirected back to the desired URL), we are parsing this callback redirect URL to obtain an authorization code and other data.
The authorization code is to be used when making user access token. Along with the access token, returned Token data structure contains lifetime of the access token in seconds and refresh token (if it is supported).
4. Refresh access token (when needed)
Token refreshedToken = null;
if (connectorMeta.isRefreshToken()) {
refreshedToken = authApi.makeToken(
"refresh_token", // grant type
token.getRefreshToken(), // refresh token
null // environment is not needed for token refresh
);
}
let refreshedToken;
if (connectorMeta.refreshToken) {
refreshedToken = authApi.makeToken(
"refresh_token", // grant type
token.refresh_token // refresh token
);
}
refreshed_token = None
if connector_meta.refresh_token:
refreshed_token = auth_api.make_token(
"refresh_token",
token.refresh_token
)
When supported, refresh token can be used for obtaining new user access token.
Information on whether refresh tokens are supported by a connector can be seen in the connector meta.
SDK reference
Select a language for code samples from the tabs above or the mobile navigation menu.
enable:Banking SDK consists of 4 interfaces:
- Meta provides information about available connectors;
- Auth provides PSU (bank user) authentication and token creation functionality;
- AISP provides functions for accessing account information, such as transactions and balances, on behalf of a PSU;
- PISP provides functions for initiating and conforming payment requests.
The same calls and data structures are used for interacting with different ASPSPs (banks). In order to use each of the interfaces corresponding instance needs to be created. When instantiating any of the interfaces except Meta, ASPSP connector name and settings need to be supplied. The settings differ from one connector to another (although many of them have the same properties) and are described in the Connectors section of the documentation.
enable:Banking SDK interfaces are based on STET PSD2 specification, but have been modified and extended in order to support usage scenarious beyond the original specification.
License: LicenseRef-LICENSE
Meta API
getConnectors
Code samples
const enablebanking = require('enablebanking');
const metaApi = new enablebanking.MetaApi(new enablebanking.ApiClient());
const connectors = await metaApi.getConnectors({
country: 'FI' // requesting connectors for Finland
});
import enablebanking
meta_api = enablebanking.MetaApi(enablebanking.ApiClient())
connectors = meta_api.get_connectors(
country='FI') # requesting connectors for Finland
import com.enablebanking.*;
import com.enablebanking.model.*;
import com.enablebanking.api.MetaApi;
MetaApi metaApi = new MetaApi(new ApiClient());
HalConnectors connectors = metaApi.getConnectors("FI"); // connectors for Finland
Retrieval of the available connectors
Parameters
Name | Type | Required | Description |
---|---|---|---|
country | string | false | Country ISO 3166-1 alpha-2 code |
Returned value
Data type: HalConnectors
List of connectors
Auth API
getAuth
Code samples
const enablebanking = require('enablebanking');
const authApi = new enablebanking.AuthApi(
new enablebanking.ApiClient('Bank name', [ /* Bank settings */ ]));
const { url } = await authApi.getAuth(
{
state: 'test' // state to pass to redirect URL
});
import enablebanking
auth_api = enablebanking.AuthApi(enablebanking.ApiClient(
'Bank name',
{
# Bank settings
})
url = auth_api.get_auth(
state='test', # state to pass to redirect URL
).url
import java.util.List;
import java.util.ArrayList;
import java.util.Arrays;
import com.enablebanking.ApiClient;
import com.enablebanking.model.*;
import com.enablebanking.api.AuthApi;
List bankSettings = new ArrayList();
// Filling in bank settings here...
AuthApi authApi = new AuthApi(new ApiClient("Bank name", bankSettings));
String url = authApi.getAuth(
"test", // state to pass to redirect URL
null, // user id
null, // password
null // not passing access request (bank's defaults will be used)
).url;
Request Authorization Data
Parameters
Name | Type | Required | Description |
---|---|---|---|
state | string | false | Arbitrary String to be returned from to redirect URI |
user_id | string | false | Identification of a PSU to be authenticated (used only for ASPSPs, which do not provide user interface for entering user ID on the authentication web page) |
password | string | false | Identification of a PSU to be authenticated (used only for ASPSPs, which do not provide user interface for entering user ID and password on the authentication web page) |
access | Access | false | Request for access to account information |
Detailed descriptions
access: Request for access to account information This parameter should be set to override defaults for the banks, which allow PSUs to give consent for account information sharing right after authentication.
Returned value
Data type: Auth
Authorization Data
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
makeToken
Code samples
const enablebanking = require('enablebanking');
const authApi = new enablebanking.AuthApi(
new enablebanking.ApiClient('Bank name', [ /* Bank settings */ ]));
const token = await authApi.makeToken(
'authorization_code', // grant type, MUST be set to "authorization_code"
'so43ls-3ldg03sd-hl4saa3l2sl5czfhl3'); // The code received in the query string when redirected from authorization
import enablebanking
auth_api = enablebanking.AuthApi(enablebanking.ApiClient(
'Bank name',
{
# Bank settings
})
token = auth_api.make_token(
'authorization_code', # grant type, MUST be set to "authorization_code"
'so43ls-3ldg03sd-hl4saa3l2sl5czfhl3') # The code received in the query string when redirected from authorization
import java.util.List;
import java.util.ArrayList;
import com.enablebanking.ApiClient;
import com.enablebanking.model.*;
import com.enablebanking.api.AuthApi;
List bankSettings = new ArrayList();
// Filling in bank settings here...
AuthApi authApi = new AuthApi(new ApiClient("Bank name", bankSettings));
Token token = authApi.makeToken(
"authorization_code", // grant type, MUST be set to "authorization_code"
"so43ls-3ldg03sd-hl4saa3l2sl5czfhl3" // The code received in the query string when redirected from authorization
);
Request Access Token
Parameters
Name | Type | Required | Description |
---|---|---|---|
grant_type | string | true | Value should be set to authorization_code or refresh_token depending on what |
code | string | true | Value of either the code received in the query string when redirected from |
auth_env | string | false | Additional environment data for makeToken. This parameter is only required for some connectors. |
Detailed descriptions
grant_type: Value should be set to authorization_code
or refresh_token
depending on what
passed to code
parameter.
code: Value of either the code received in the query string when redirected from authorization page or the refresh token used for renewing access token.
Enumerated Values
grant_type
Value | Description |
---|---|
authorization_code | Used to exchange an authorization code for an access token. After the user returns to the client via the redirect URL, the application will get the authorization code from the URL and use it to request an access token. |
refresh_token | Used to exchange a refresh token for an access token when the access token has expired. This allows clients to continue to have a valid access token without further interaction with the user. Please note that refresh token is likely to be changed when calling `makeToken` with `refresh_token` grant type. |
Returned value
Data type: Token
Authorisation token (Bearer)
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
getCurrentToken
Code samples
const enablebanking = require('enablebanking');
const authApi = new enablebanking.AuthApi(
new enablebanking.ApiClient('Bank name', [ /* Bank settings */ ]));
const token = await authApi.getCurrentToken();
import enablebanking
auth_api = enablebanking.AuthApi(enablebanking.ApiClient(
'Bank name',
{
# Bank settings
})
token = auth_api.get_current_token()
import java.util.List;
import java.util.ArrayList;
import com.enablebanking.ApiClient;
import com.enablebanking.model.*;
import com.enablebanking.api.AuthApi;
List bankSettings = new ArrayList();
// Filling in bank settings here...
AuthApi authApi = new AuthApi(new ApiClient("Bank name", bankSettings));
Token token = authApi.getCurrentToken();
Get current token data
Returned value
Data type: Token
Current token. Values for some fields might be missing if not used.
parseRedirectUrl
Parsing of redirect url during authorization
Parameters
Name | Type | Required | Description |
---|---|---|---|
redirectUrl | string | true | Redirect url |
Returned value
Data type: AuthRedirect
Structured data extracted from redirect URL
setClientInfo
Set extra PSU headers
Parameters
Name | Type | Required | Description |
---|---|---|---|
clientInfo | ClientInfo | false | ClientInfo object with PSU headers |
Returned value
Data type: None
Client Info was successfully set.
AISP API
getAccounts
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', [ /* Connector settings */ ]));
const accounts = await aispApi.getAccounts();
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
accounts = aisp_api.get_accounts()
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
HalAccounts accounts = aispApi.getAccounts();
Retrieval of the PSU accounts (AISP)
Description
This call returns all payment accounts that are relevant the PSU on behalf of whom the AISP is connected.Prerequisites
- Valid PSU access token shall be set in the connector.
- For ASPSPs requiring PSU consent for listing the accounts, consent ID shall be set in the connector.
Business flow
The TPP sends a request to the ASPSP for retrieving the list of the PSU payment accounts. The ASPSP computes the relevant PSU accounts and builds the answer as an accounts list. Each payment account will be provided with its characteristics returned by ASPSP, which may vary between ASPSPs and depending on the consent given by the PSU.Returned value
Data type: HalAccounts
Returned value consists of the list of the accounts that have been made available to the AISP by the PSU and HYPERMEDIA links to the related resources provided by ASPSP through the API.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
InvalidResponseSignatureException | none |
getAccountBalances
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', [ /* Connector settings */ ]));
const balances = await aispApi.getAccountBalances('203059694928560295396833');
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
balances = aisp_api.get_account_balances('203059694928560295396833')
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
HalBalances balances = aispApi.getAccountBalances("203059694928560295396833")
Retrieval of an account balances report (AISP)
Description
This call returns a set of balances for a given PSU account that is specified by the AISP through an account resource identification.- ASPSPs provide at least the accounting balance on the account.
- ASPSPs can provide other balance restitutions, e.g. instant balance, as well, if possible.
- Actually, from the PSD2 perspective, any other balances that are provided through the Web-Banking service of the ASPSP must also be provided by this ASPSP through the API.
Prerequisites
- Valid PSU access token shall be set in the connector.
- Consent ID shall be set in the connector.
- The TPP has previously retrieved the list of available accounts for the PSU.
Business flow
The AISP requests the ASPSP on one of the PSU’s accounts.The ASPSP answers by providing a list of balances on this account.
Parameters
Name | Type | Required | Description |
---|---|---|---|
accountResourceId | string | true | Identification of account resource to fetch |
Returned value
Data type: HalBalances
Returned value consists of the list of account balances and HYPERMEDIA links to the related resources provided by ASPSP through the API.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
InvalidResponseSignatureException | none |
getAccountTransactions
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', [ /* Connector settings */ ]));
const transactions = await aispApi.getAccountTransactions('203059694928560295396833');
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
transactions = aisp_api.get_account_transactions('203059694928560295396833')
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
HalTransactions transactions = aispApi.getAccountTransactions("203059694928560295396833");
Retrieval of an account transaction set (AISP)
Description
This call returns transactions for an account for a given PSU account that is specified by the AISP through an account resource identification. The request may use some filter parameter in order to restrict the query- on a given imputation date range
- past a given incremental technical identification
Prerequisites
- Valid PSU access token shall be set in the connector.
- Consent ID shall be set in the connector.
- The TPP has previously retrieved the list of available accounts for the PSU.
Business flow
The AISP requests the ASPSP on one of the PSU’s accounts. It may specify some selection criteria. The ASPSP answers by a set of transactions that matches the query.Parameters
Name | Type | Required | Description |
---|---|---|---|
accountResourceId | string | true | Identification of account resource to fetch |
dateFrom | string(date-time) | false | Inclusive minimal imputation date of the transactions. |
dateTo | string(date-time) | false | Exclusive maximal imputation date of the transactions. |
continuationKey | string | false | Specifies the value on which the result has to be computed. |
transactionStatus | TransactionStatus | false | Transactions having a transactionStatus equal to this parameter are included within the result. |
Detailed descriptions
dateFrom: Inclusive minimal imputation date of the transactions.
Transactions having an imputation date equal to this parameter are included within the result.
dateTo: Exclusive maximal imputation date of the transactions.
Transactions having an imputation date equal to this parameter are not included within the result.
continuationKey: Specifies the value on which the result has to be computed.
Enumerated Values
transactionStatus
Value | Description |
---|---|
BOOK | (ISO20022 ClosingBooked) Accounted transaction |
CNCL | Cancelled transaction |
HOLD | Account hold |
OTHR | Transaction with unknown status or not fitting the other options |
PDNG | (ISO20022 Expected) Instant Balance Transaction |
RJCT | Rejected transaction |
SCHD | Scheduled transaction |
Returned value
Data type: HalTransactions
Complete transactions response
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
DataRetrievalException | Raised when unable to retrieve data requested from ASPSP API. |
InsufficientScopeException | Raised when consent given by the PSU does not allow to retrieve requested data. |
InvalidResponseSignatureException | none |
modifyConsents
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', [ /* Connector settings */ ]));
const consent = await aispApi.modifyConsents({
access: {
balances: ['203059694928560295396833'],
transactions: [],
trustedBeneficiaries: false,
psuIdentity: true
}
});
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
consent = aisp_api.modify_consents(
access=enablebanking.Access(
# default access to balances
balances=enablebanking.BalancesAccess(),
# default access to transactions
transactions=enablebanking.TransacrionsAccess(),
trusted_beneficiaries=False,
psu_identity=True,
),
)
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
Consent consent = aispApi.modifyConsents(
new Access()
.balances(new BalancesAccess()) // default access to balances
.transactions(new TransactionsAccess()) // default access to transactions
.trustedBeneficiaries(false)
.psuIdentity(true));
Forwarding the PSU consent (AISP)
Description
This call requests ASPSP to set the consent of an authenticated PSU. In the mixed detailed consent on accounts- the AISP captures the consent of the PSU
- then it forwards this consent to the ASPSP
Prerequisites
- Valid PSU access token shall be set in the connector.
- For ASPSPs allowing consent to be give only for specified accounts, the list of accounts shall be retrieved.
Business flow
The PSU specifies to the AISP which of his/her accounts will be accessible and which functionalities should be available. The AISP forwards these settings to the ASPSP.Parameters
Name | Type | Required | Description |
---|---|---|---|
access | Access | false | List of consents granted to the AISP by the PSU. |
Returned value
Data type: Consent
Created or modified consent
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
getCurrentConsent
Code samples
const enablebanking = require('enablebanking');
const aispApi = new enablebanking.AISPApi(
new enablebanking.ApiClient('ConnectorName', [ /* Connector settings */ ]));
const consent = await aispApi.getCurrentConsent();
import enablebanking
aisp_api = enablebanking.AISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
consent = aisp_api.get_current_consent()
import com.enablebanking.ApiClient;
import com.enablebanking.api.AispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
AispApi aispApi = new AispApi(new ApiClient(connectorSettings));
Consent consent = aispApi.getCurrentConsent();
Returns current consent (AISP)
Description
This call returns the consent current being used by the ASPSP connector, i.e. either explicitly set when the connector is initialized or as side effect produced by `getAuth` call.Returned value
Data type: Consent
Current consent used for accessing AIS APIs.
deleteConsent
Deletes consent (AISP)
Description
Revokes PSU consent by specified consent ID. After the consent is revoked, it becomes invalid and can not be used for futher API requests.Business flow
The PSU signals that he/she wants to revoke his consent for TPP to access account information. The TPP makes a request to the ASPSP to revoke PSU consent. The consent becomes invalid and its ID can not be used for consequent calls.Parameters
Name | Type | Required | Description |
---|---|---|---|
consentId | string | true | Identification of consent to fetch |
Returned value
Data type: None
Consent successfully revoked
PISP API
makePaymentRequest
Code samples
const enablebanking = require('enablebanking');
const pispApi = new enablebanking.PISPApi(
new enablebanking.ApiClient('ConnectorName', [ /* Connector settings */ ]));
// Making payment request with credit transafer transaction of one element
const requestCreation = await pispApi.makePaymentRequest({
creditTransferTransaction: [{
instructedAmount: {
amount: '12.25', // amount
currency: 'EUR' // currency code
},
beneficiary: {
creditor: {
name: 'Creditor Name' // payee (merchant) name
},
creditorAccount: {
iban: 'FI4966010005485495' // payee account number
}
}
}],
debtor: {
name: 'Debtor Name' // payer (account holder) name
},
debtorAccount: {
iban: 'FI3839390001384700' // payer account number
}
});
import enablebanking
pisp_api = enablebanking.PISPApi(enablebanking.ApiClient(
'ConnectorName',
{
# Connector settings
}))
# Making payment request with credit transafer transaction of one element
request_creation = pisp_api.make_payment_request(
enablebanking.PaymentRequestResource(
credit_transfer_transaction=[
enablebanking.CreditTransferTransaction(
instructed_amount=enablebanking.AmountType(
amount='12.25', # amount
currency='EUR'), # currency code
beneficiary=enablebanking.Beneficiary(
creditor=enablebanking.PartyIdentification(
name='Creditor Name'), # payee (merchant) name
creditor_account=enablebanking.AccountIdentification(
iban='FI4966010005485495'), # payee account number
),
),
],
debtor=enablebanking.PartyIdentification(
name='Debtor Name'), # payer (account holder) name
debtor_account=enablebanking.AccountIdentification(
iban='FI3839390001384700'), # payer account number
))
import java.math.BigDecimal;
import java.util.Arrays;
import com.enablebanking.ApiClient;
import com.enablebanking.api.PispApi;
import com.enablebanking.model.*;
// To be replaced with real connector settings class and set properties
ConnectorSettings connectorSettings = new SomeBankConnectorSettings();
PispApi pispApi = new PispApi(new ApiClient(connectorSettings));
// Making payment request with credit transafer transaction of one element
HalPaymentRequestCreation requestCreation = pispApi.makePaymentRequest(
new PaymentRequestResource()
.creditTransferTransaction(Arrays.asList(
new CreditTransferTransaction()
.instructedAmount(
new AmountType()
.currency("EUR")
.amount(new BigDecimal("12.25")))
.beneficiary(
new Beneficiary()
.creditor(
new PartyIdentification()
.name("Creditor Name"))
.creditorAccount(
new AccountIdentification()
.iban("FI4966010005485495")))))
.debtor(
new PartyIdentification()
.name("Debtor Name"))
.debtorAccount(
new AccountIdentification()
.iban("FI3839390001384700")));
Payment request initiation (PISP)
Description
The following use cases can be applied:- payment request on behalf of a merchant
- transfer request on behalf of the account's owner
- standing-order request on behalf of the account's owner
Data content
A payment request or a transfer request might embed several payment instructions having- one single execution date or multiple execution dates,
- one single beneficiary or multiple beneficiaries.
Business flow
Payment Request use case
The PISP forwards a payment request on behalf of a merchant.The PSU buys some goods or services on an e-commerce website held by a merchant. Among other payment method, the merchant suggests the use of a PISP service. As there is obviously a contract between the merchant and the PISP, there is no need of such a contract between the PSU and this PISP to initiate the process.
Case of the PSU that chooses to use the PISP service:
- The merchant forwards the requested payment characteristics to the PISP and redirects the PSU to the PISP portal.
- The PISP requests from the PSU which ASPSP will be used.
- The PISP prepares the Payment Request and sends this request to the ASPSP.
- The Request can embed several payment instructions having different requested execution date.
- The beneficiary, as being the merchant, is set at the payment level.
Transfer Request use case
The PISP forwards a transfer request on behalf of the owner of the account.- The PSU provides the PISP with all information needed for the transfer.
- The PISP prepares the Transfer Request and sends this request to the relevant ASPSP that holds the debtor account.
- The Request can embed several payment instructions having different beneficiaries.
- The requested execution date, as being the same for all instructions, is set at the payment level.
Standing Order Request use case
The PISP forwards a Standing Order request on behalf of the owner of the account.- The PSU provides the PISP with all information needed for the Standing Order.
- The PISP prepares the Standing Order Request and sends this request to the relevant ASPSP that holds the debtor account.
- The Request embeds one single payment instruction with
- The requested execution date of the first occurrence
- The requested execution frequency of the payment in order to compute further execution dates
- An execution rule to handle cases when the computed execution dates cannot be processed (e.g. bank holydays)
- An optional end date for closing the standing Order
Authentication flows for all use cases
As the request posted by the PISP to the ASPSP needs a PSU authentication before execution, this request will include:- The specification of the authentication approaches that are supported by both the PISP and the ASPSP (any combination of "REDIRECT", "EMBEDDED" and "DECOUPLED" values).
- In case of possible REDIRECT or DECOUPLED authentication approach, one or two callback URLs will be passed to the ASPSP at the finalisation of the authentication and consent process. The URLs are set in the connector settings.
- In case of possible "EMBEDDED" or "DECOUPLED" approaches, the PSU identifier that can be processed by the ASPSP for PSU recognition must have been set within the [debtor] structure.
- A resource identification of the accepted request that can be further used to retrieve the request and its status information.
- The specification of the chosen authentication approach taking into account both the PISP and the PSU capabilities.
- In case of chosen REDIRECT authentication approach, the URL to be used by the PISP for redirecting the PSU in order to perform a authentication.
Redirect authentication approach
When the authentication approach within the ASPSP answer is set to "REDIRECT":- The PISP redirects the PSU to the ASPSP which authenticates the PSU.
- The ASPSP asks the PSU to give (or deny) his/her consent to the payment request
- The PSU chooses or confirms which of his/her accounts shall be used by the ASPSP for the future credit transfer.
- The ASPSP is then able to initiate the subsequent credit transfer.
- The ASPSP redirects the PSU to the PISP using one of the callback URLs.
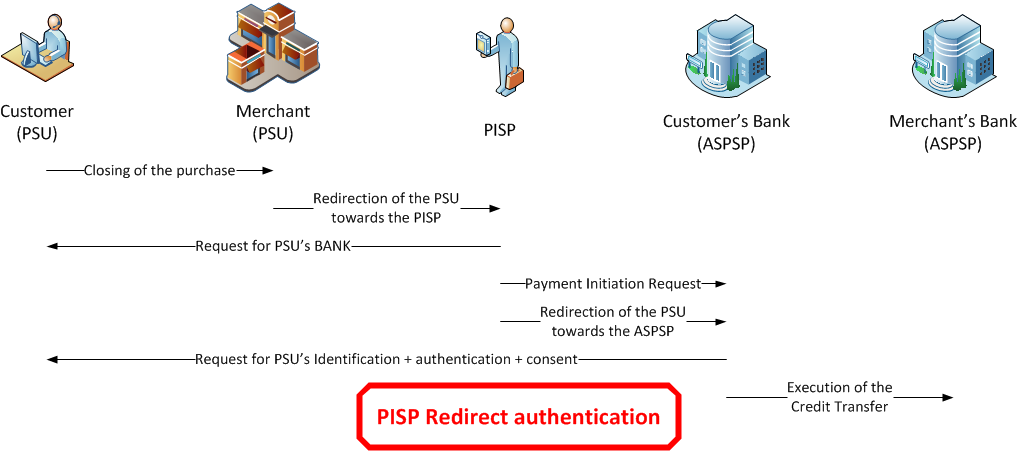
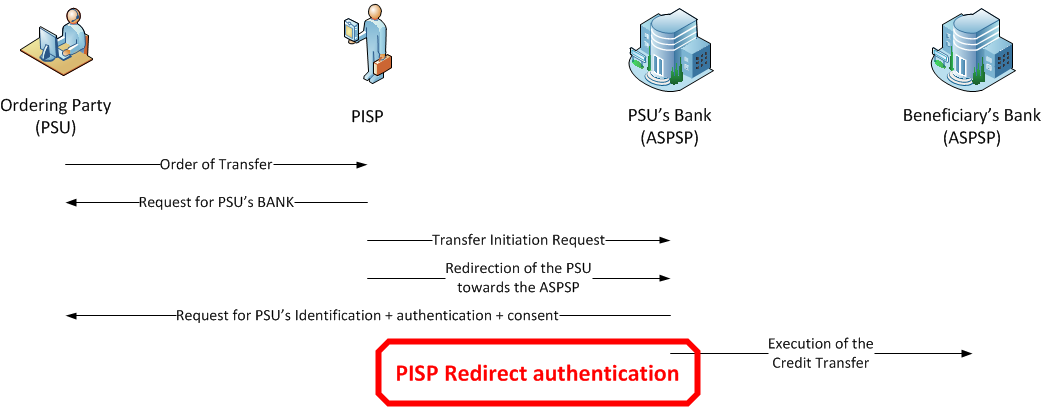
Decoupled authentication approach
When the authentication approach is "DECOUPLED":- Based on the PSU identifier provided within the payment request by the PISP, the ASPSP gives the PSU with the payment request details and challenges the PSU for a Strong Customer Authentication on a decoupled device or application.
- The PSU chooses or confirms which of his/her accounts shall be used by the ASPSP for the future credit transfer.
- The ASPSP is then able to initiate the subsequent credit transfer.
- The ASPSP notifies the PISP about the finalisation of the authentication and consent process by using one of the callback URLs.
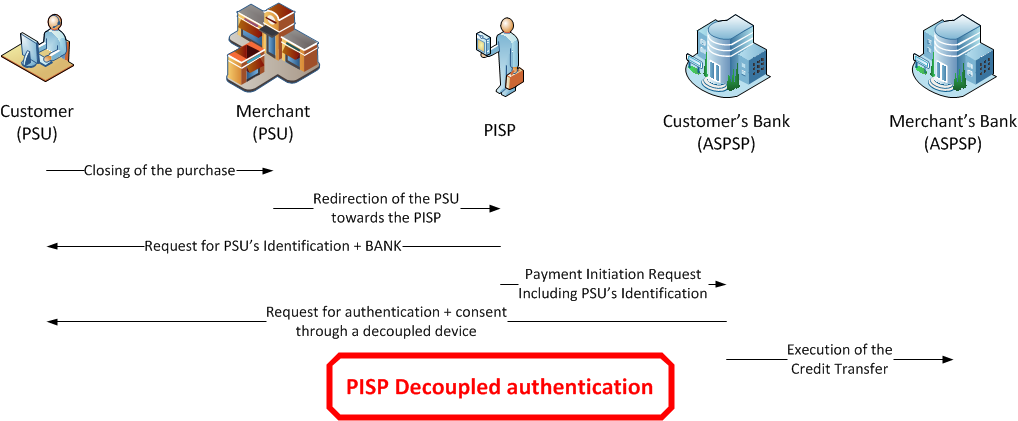
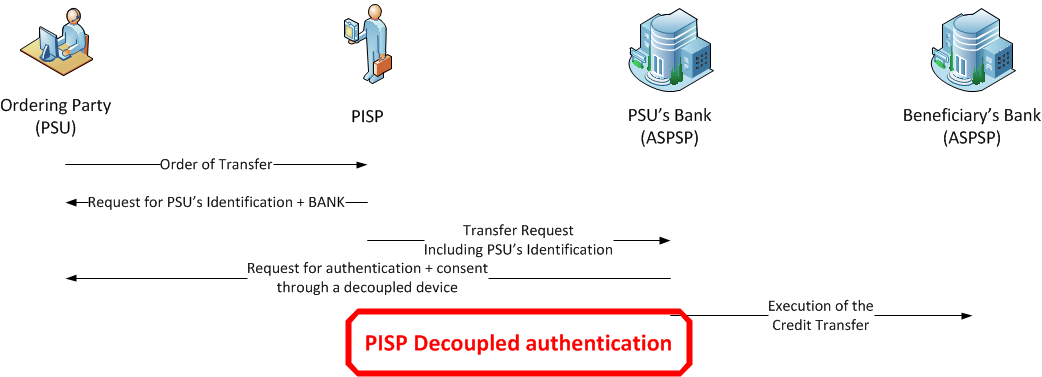
Embedded authentication approach
When the authentication approach within the ASPSP answers is set to "EMBEDDED":- The TPP informs the PSU that a challenge is needed for completing the Payment Request processing. This challenge will be one of the following:
- A One-Time-Password sent by the ASPSP to the PSU on a separate device or application.
- A response computed by a specific device on base of a challenge sent by the ASPSP to the PSU on a separate device or application.
- The PSU unlocks the device or application through a "knowledge factor" and/or an "inherence factor" (biometric), retrieves the payment request details and processes the data sent by the ASPSP.
- The PSU might choose to confirm which of his/her accounts shall be used by the ASPSP for the future credit transfer when the device or application allows it.
- When agreeing the payment request, the PSU enters the resulting authentication factor through the PISP interface.
- The PISP shall confirm the payment request using the authentication factor received from the PSU.
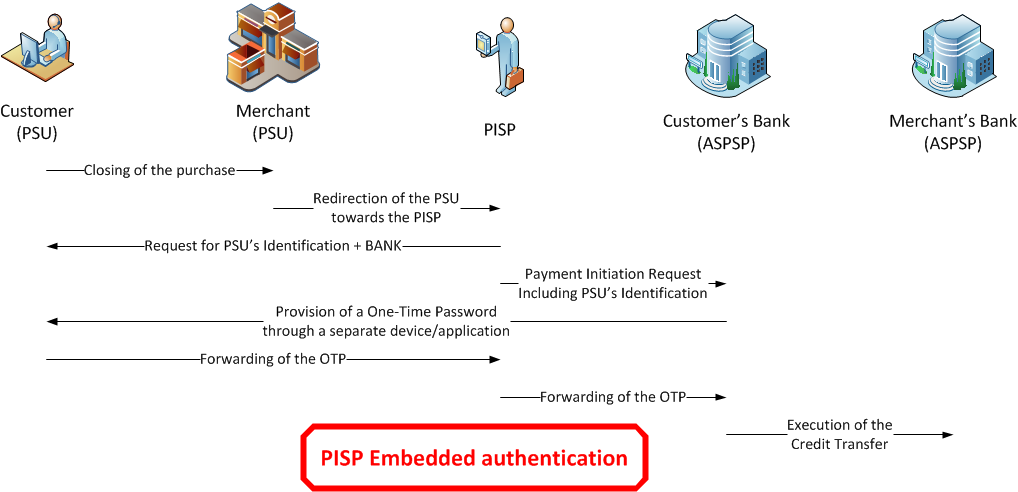
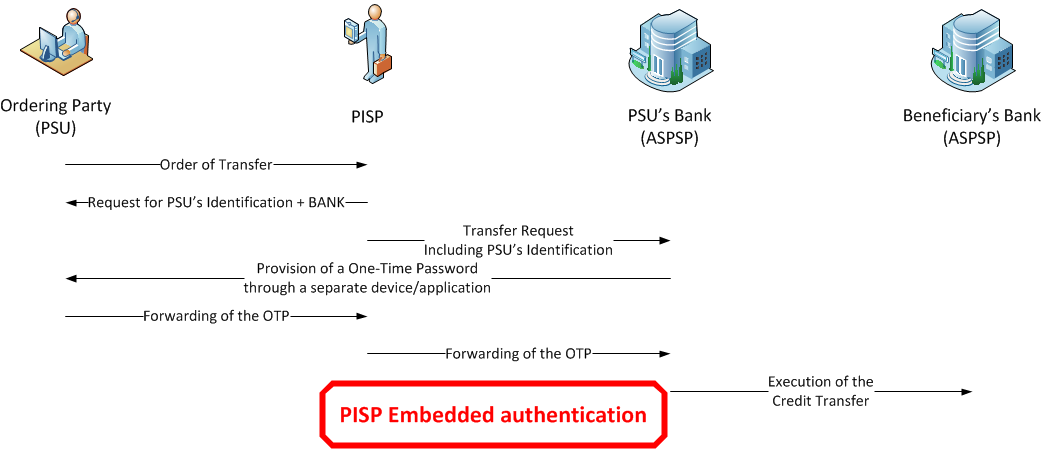
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequest | PaymentRequestResource | true | ISO20022 based payment Initiation Request |
Returned value
Data type: HalPaymentRequestCreation
The request has been created as a resource. The ASPSP must authenticate the PSU.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
getPaymentRequest
Retrieval of a payment request (PISP)
Description
The following use cases can be applied:- retrieval of a payment request on behalf of a merchant
- retrieval of a transfer request on behalf of the account's owner
- retrieval of a standing-order request on behalf of the account's owner
The ASPSP has registered the request, updated if necessary the relevant identifiers in order to avoid duplicates and returned the updated request.
The PISP gets the request that might have been updated with the resource identifiers, the status of the payment/transfer request and the status of the subsequent credit transfer.
Prerequisites
- The TPP has previously posted a payment request which has been accepted by the ASPSP.
Business flow
The PISP asks to retrieve the payment/transfer request that has been accepted by the ASPSP. The PISP uses the payment request identifier provided by the ASPSP in response to the payment request.The ASPSP returns the previously accepted payment/transfer request, which is enriched with:
- The resource identifiers given by the ASPSP
- The status information of the Payment Request and of the subsequent credit transfer
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
Returned value
Data type: HalPaymentRequest
Retrieval of the previously posted Payment Request
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
modifyPaymentRequest
Modification of a payment/transfer request (PISP)
Description
The PISP sent a payment/transfer request through a POST command.The ASPSP registered the Payment/Transfer Request, updated if necessary the relevant identifiers in order to avoid duplicates and returned the location of the updated Request.
The PISP got the Payment/Transfer Request that might have been updated with the resource identifiers, the status of the Payment/Transfer Request and the status of the subsequent credit transfer.
The PISP request for the payment cancellation or for some payment instructions cancellation
No other modification of the Payment/Transfer Request is allowed.
Prerequisites
- The TPP previously sent a payment/transfer request which was accepted by the ASPSP
- The TPP presented the payment/transfer request.
Business flow
The following cases can be applied:- Case of a payment with multiple instructions or a standing order, the PISP asks to cancel the whole payment/transfer or standing order request including all non-executed payment instructions by setting the [paymentInformationStatus] to "RJCT" and the relevant [statusReasonInformation] to "DS02" at payment level.
- Case of a payment with multiple instructions, the PISP asks to cancel one or several payment instructions by setting the [transactionStatus] to "RJCT" and the relevant [statusReasonInformation] to "DS02" at each relevant instruction level.
- The specification of the authentication approaches that are supported by the PISP (any combination of "REDIRECT", "EMBEDDED" and "DECOUPLED" values).
- In case of possible REDIRECT or DECOUPLED authentication approach, one or two call-back URLs to be used by the ASPSP at the finalisation of the authentication and consent process :
- The first call-back URL will be called by the ASPSP if the Transfer Request is processed without any error or rejection by the PSU
- The second call-back URL is to be used by the ASPSP in case of processing error or rejection by the PSU. Since this second URL is optional, the PISP might not provide it. In this case, the ASPSP will use the same URL for any processing result.
- Both call-back URLS must be used in a TLS-secured request, including mutual authentication based on each party’s TLS certificate.
- In case of possible "EMBEDDED" or "DECOUPLED" approaches, a PSU identifier that can be processed by the ASPSP for PSU recognition.
- The specification of the chosen authentication approach taking into account both the PISP and the PSU capabilities.
- In case of chosen REDIRECT authentication approach, the URL to be used by the PISP for redirecting the PSU in order to perform an authentication.
Authentication flows for both use cases
Redirect authentication approach
When the chosen authentication approach within the ASPSP answers is set to "REDIRECT":- The PISP redirects the PSU to the ASPSP which authenticates the PSU
- The ASPSP asks the PSU to give (or deny) his/her consent to the Payment Request
- The PSU chooses or confirms which of his/her accounts shall be used by the ASPSP for the future Credit Transfer.
- The ASPSP is then able to initiate the subsequent Credit Transfer
- The ASPSP redirects the PSU to the PISP using one of the call-back URLs provided within the posted Payment Request
Decoupled authentication approach
When the chosen authentication approach is "DECOUPLED":- Based on the PSU identifier provided within the Payment Request by the PISP, the ASPSP gives the PSU with the Payment Request details and challenges the PSU for a Strong Customer Authentication on a decoupled device or application.
- The PSU chooses or confirms which of his/her accounts shall be used by the ASPSP for the future Credit Transfer.
- The ASPSP is then able to initiate the subsequent Credit Transfer
- The ASPSP notifies the PISP about the finalisation of the authentication and consent process by using one of the call-back URLs provided within the posted Payment Request
Embedded authentication approach
When the chosen authentication approach within the ASPSP answers is set to "EMBEDDED":- The TPP informs the PSU that a challenge is needed for completing the Payment Request processing. This challenge will be one of the following:
- A One-Time-Password sent by the ASPSP to the PSU on a separate device or application.
- A response computed by a specific device on base of a challenge sent by the ASPSP to the PSU on a separate device or application.
- The PSU unlock the device or application through a "knowledge factor" and/or an "inherence factor" (biometric), retrieves the Payment Request details and processes the data sent by the ASPSP;
- The PSU might choose or confirm which of his/her accounts shall be used by the ASPSP for the future Credit Transfer when the device or application allows it.
- When agreeing the Payment Request, the PSU enters the resulting authentication factor through the PISP interface which will forward it to the ASPSP through a confirmation request (cf. § 4.7)
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
paymentRequest | PaymentRequestResource | true | ISO20022 based payment Initiation Request |
Returned value
Data type: HalPaymentRequestCreation
The modification request has been saved. The ASPSP must authenticate the PSU before committing the update.
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
makePaymentRequestConfirmation
Confirmation of a payment request or a modification request (PISP)
Description
The PISP confirms one of the following requests- payment request on behalf of a merchant
- transfer request on behalf of the account's owner
- standing-order request on behalf of the account's owner
Prerequisites
- The TPP has previously set a request which has been accepted by the ASPSP.
- The TPP has retrieved the accpeted request in order to get the relevant resource IDs.
- The TPP provided the PSU necessary information and means for SCA required before the confirmation.
Business flow
Once the PSU has been authenticated, it is the due to the PISP to confirm the Request to the ASPSP in order to complete the process flow.In REDIRECT and DECOUPLED approach, this confirmation is not a prerequisite to the execution of the Credit Transfer.
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId | string | true | Identification of the Payment Request Resource |
confirmation | PaymentRequestConfirmation | false | Data needed for confirmation of the Payment Request, especially in EMBEDDED approach |
Detailed descriptions
confirmation: Data needed for confirmation of the Payment Request, especially in EMBEDDED approach
Returned value
Data type: HalPaymentRequest
retrieval of the Payment Request enriched with the status report
Exceptions
Data type | Description |
---|---|
HttpException | Raised when ASPSP API responses with unspecified error. |
CBPII API
makeFundsConfirmation
Payment coverage check request (CBPII)
Description
The CBPII can ask an ASPSP to check if a given amount can be covered by the liquidity that is available on a PSU cash account or payment card.Business flow
The CBPII requests the ASPSP for a payment coverage check against either a bank account or a card primary identifier.The ASPSP answers with a structure embedding the original request and the result as a Boolean.
Parameters
Name | Type | Required | Description |
---|---|---|---|
paymentCoverage | PaymentCoverageRequestResource | true | parameters of a payment coverage request |
Returned value
Data type: HalPaymentCoverageReport
payment coverage request
Data types
Access
Requested access services.
Fields
Name | Type | Required | Description |
---|---|---|---|
accounts |
false |
List of accounts access to which is requested. If not set behaviour depends on the bank: some banks allow users to choose list of accessible accounts through their access consent UI, while other may provide access to all accounts or just access to the list of accounts. |
|
balances |
false |
Defines how balance information is going to be accessed. If not set balances are not explicitly requested |
|
transactions |
false |
Defines how transactions are going to be accessed. If not set transactions are not explicitly requested |
|
trustedBeneficiaries |
boolean |
false |
Indicator that access to the trusted beneficiaries list was granted or not to the AISP by the PSU
|
psuIdentity |
boolean |
false |
Indicator that access to the PSU identity, first name and last name, was granted or not to the AISP by the PSU
|
accountDetails |
boolean |
false |
Indicates if access to account details if explicitly requested |
recurringIndicator |
boolean |
false |
|
frequencyPerDay |
integer |
false |
This field indicates the requested maximum frequency for an access without PSU involvement per day. For a one-off access, this attribute is set to "1". The frequency needs to be greater equal to one. |
validUntil |
string(date-time) |
false |
This parameter is requesting a valid until date for the requested consent. The content is the local ASPSP date in ISO-Date Format, e.g. 2017-10-30. Future dates might get adjusted by ASPSP. |
AccountIdentification
Unique and unambiguous identification for the account between the account owner and the account servicer.
Fields
Name | Type | Required | Description |
---|---|---|---|
iban |
string |
false |
ISO20022: International Bank Account Number (IBAN) - identification used internationally by financial institutions to uniquely identify the account of a customer. Further specifications of the format and content of the IBAN can be found in the standard ISO 13616 "Banking and related financial services - International Bank Account Number (IBAN)" version 1997-10-01, or later revisions. |
other |
false |
ISO20022: Unique identification of an account, a person or an organisation, as assigned by an issuer. |
AccountResource
PSU account that is made available to the TPP
Fields
Name | Type | Required | Description |
---|---|---|---|
resourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
bicFi |
string |
false |
ISO20022: Code allocated to a financial institution by the ISO 9362 Registration Authority as described in ISO 9362 "Banking - Banking telecommunication messages - Business identification code (BIC)". |
accountId |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
name |
string |
true |
Label of the PSU account In case of a delayed debit card transaction set, the name shall specify the holder name and the imputation date |
details |
string |
false |
Specifications that might be provided by the ASPSP
|
linkedAccount |
string |
false |
Case of a set of pending card transactions, the APSP will provide the relevant cash account the card is set up on. |
usage |
string |
false |
Specifies the usage of the account |
cashAccountType |
string |
true |
Specifies the type of the account |
product |
string |
false |
Product Name of the Bank for this account, proprietary definition |
currency |
true |
Currency used for the account |
|
balances |
false |
list of balances provided by the ASPSP |
|
psuStatus |
string |
false |
Relationship between the PSU and the account - Account Holder - Co-account Holder - Attorney |
_links |
true |
links that can be used for further navigation when browsing Account Information at one |
Enumerated Values
usage
Value | Description |
---|---|
PRIV |
private personal account |
ORGA |
professional account |
cashAccountType
Value | Description |
---|---|
CACC |
Account used to post debits and credits when no specific account has been nominated |
CASH |
Account used for the payment of cash |
CARD |
List of card based transactions (non ISO 20022) |
LOAN |
Loan account |
AddressType
ISO20022: Identifies the nature of the postal address.
Base type
string
Enumerated Values
Value | Description |
---|---|
Business |
— |
Correspondence |
— |
DeliveryTo |
— |
MailTo |
— |
POBox |
— |
Postal |
— |
Residential |
— |
Statement |
— |
AmountType
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used.
API: only instructed amount can be used
Fields
Name | Type | Required | Description |
---|---|---|---|
currency |
true |
Specifies the currency of the amount or of the account. |
|
amount |
string(number) |
true |
ISO20022: Amount of money to be moved between the debtor and creditor, before deduction of charges, expressed in the currency as ordered by the initiating party. |
Auth
Fields
Name | Type | Required | Description |
---|---|---|---|
url |
string |
false |
URL for authorization. Used for REDIRECT authorization flow |
env |
string |
false |
Additional authorization environment data. To be passed to makeToken. |
authData |
false |
[structure used for returning auth parameters to be passed to user] |
AuthDataItem
structure used for returning auth parameters to be passed to user
Fields
Name | Type | Required | Description |
---|---|---|---|
type |
string |
false |
Type of the auth data item |
value |
string |
false |
Value of auth data item |
Enumerated Values
type
Value | Description |
---|---|
otpIndex |
— |
AuthInfo
Information about usage of getAuth
method
Fields
Name | Type | Required | Description |
---|---|---|---|
access |
boolean |
true |
Shows if access parameter is supported in |
userIdRequired |
boolean |
true |
Shows if it is necessary to provide user id in |
passwordRequired |
boolean |
false |
Shows if it is necessary to provide user password in |
authData |
boolean |
false |
Shows if |
brands |
[Brand] |
false |
List of brands used by the ASPSP (in a certain country) |
AuthRedirect
structure used for returning parameters of redirect url
Fields
Name | Type | Required | Description |
---|---|---|---|
code |
string |
false |
redirect code received from a bank |
id_token |
string |
false |
Id of token received from a bank |
state |
string |
false |
state specified by TPP |
AuthenticationApproach
The ASPSP, based on the authentication approaches proposed by the PISP, choose the one that it can processed, in respect with the preferences and constraints of the PSU and indicates in this field which approach has been chosen
Base type
string
Enumerated Values
Value | Description |
---|---|
REDIRECT |
the PSU is redirected by the TPP to the ASPSP which processes identification and authentication |
DECOUPLED |
the TPP identifies the PSU and forwards the identification to the ASPSP which processes the authentication through a decoupled device |
EMBEDDED |
the TPP identifies the PSU and forwards the identification to the ASPSP which starts the authentication. The TPP forwards one authentication factor of the PSU (e.g. OTP or response to a challenge) |
BalanceResource
Structure of an account balance
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
true |
Label of the balance |
balanceAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
balanceType |
true |
Type of balance |
|
lastChangeDateTime |
string(date-time) |
false |
Timestamp of the last change of the balance amount |
referenceDate |
string(date) |
false |
Reference date for the balance |
lastCommittedTransaction |
string |
false |
Identification of the last committed transaction. This is actually useful for instant balance. |
BalanceStatus
Type of balance
Base type
string
Enumerated Values
Value | Description |
---|---|
CLBD |
(ISO20022 ClosingBooked) Accounting Balance |
FWAV |
(ISO20022 ForwardAvailable) Balance of money that is at the disposal of the account owner on the date specified |
ITAV |
(ISO20022 InterimAvailable) Available balance calculated in the course of the day |
ITBD |
(ISO20022 InterimBooked) Booked balance calculated in the course of the day |
OTHR |
Other Balance |
VALU |
Value-date balance |
XPCD |
(ISO20022 Expected) Instant Balance |
BalancesAccess
Defines what access to blanaces is requested
Fields
Name | Type | Required | Description |
---|---|---|---|
statuses |
false |
List of balance statuses, which are going to be accessed |
BankTransactionCode
ISO20022: Allows the account servicer to correctly report a transaction, which in its turn will help account owners to perform their cash management and reconciliation operations.
Fields
Name | Type | Required | Description |
---|---|---|---|
description |
string |
false |
Transaction type description |
code |
string |
false |
ISO20022: Specifies the family of a transaction within the domain |
subCode |
string |
false |
ISO20022: Specifies the sub-product family of a transaction within a specific family |
Beneficiary
Specification of a beneficiary
Fields
Name | Type | Required | Description |
---|---|---|---|
id |
string |
false |
Id of the beneficiary |
isTrusted |
boolean |
false |
The ASPSP having not implemented the trusted beneficiaries list must not set this flag. Otherwise, the ASPSP indicates whether or not the beneficiary has been registered by the PSU within the trusted beneficiaries list.
|
creditorAgent |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
creditor |
false |
API : Description of a Party which can be either a person or an organization. |
|
creditorAccount |
true |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
creditorCurrency |
string |
false |
Creditor account currency code |
BookingInformation
indicator that the payment can be immediately booked or not
- true: payment is booked
- false: payment is not booked
Base type
boolean
Brand
Information about ASPSP end-user recognizable brand
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
true |
End-customer facing name |
logos |
[Logo] |
false |
List of available logos |
CategoryPurposeCode
ISO20022: Specifies the high level purpose of the instruction based on a set of pre-defined categories. This is used by the initiating party to provide information concerning the processing of the payment. It is likely to trigger special processing by any of the agents involved in the payment chain.
Base type
string
Enumerated Values
Value | Description |
---|---|
BONU |
BonusPayment Transaction is the payment of a bonus. |
CASH |
CashManagementTransfer Transaction is a general cash management instruction. |
CBLK |
Card Bulk Clearing A Service that is settling money for a bulk of card transactions, while referring to a specific transaction file or other information like terminal ID, card acceptor ID or other transaction details. |
CCRD |
Credit Card Payment Transaction is related to a payment of credit card. |
CORT |
TradeSettlementPayment Transaction is related to settlement of a trade, eg a foreign exchange deal or a securities transaction. |
DCRD |
Debit Card Payment Transaction is related to a payment of debit card. |
DIVI |
Dividend Transaction is the payment of dividends. |
DVPM |
DeliverAgainstPayment Code used to pre-advise the account servicer of a forthcoming deliver against payment instruction. |
EPAY |
Epayment Transaction is related to ePayment. |
FCOL |
Fee Collection A Service that is settling card transaction related fees between two parties. |
GOVT |
GovernmentPayment Transaction is a payment to or from a government department. |
HEDG |
Hedging Transaction is related to the payment of a hedging operation. |
ICCP |
Irrevocable Credit Card Payment Transaction is reimbursement of credit card payment. |
IDCP |
Irrevocable Debit Card Payment Transaction is reimbursement of debit card payment. |
INTC |
IntraCompanyPayment Transaction is an intra-company payment, ie, a payment between two companies belonging to the same group. |
INTE |
Interest Transaction is the payment of interest. |
LOAN |
Loan Transaction is related to the transfer of a loan to a borrower. |
MP2B |
Commercial Mobile P2B Payment |
MP2P |
Consumer Mobile P2P Payment |
OTHR |
OtherPayment Other payment purpose. |
PENS |
PensionPayment Transaction is the payment of pension. |
RPRE |
Represented Collection used to re-present previously reversed or returned direct debit transactions. |
RRCT |
ReimbursementReceivedCreditTransfer Transaction is related to a reimbursement for commercial reasons of a correctly received credit transfer. |
RVPM |
ReceiveAgainstPayment Code used to pre-advise the account servicer of a forthcoming receive against payment instruction. |
SALA |
SalaryPayment Transaction is the payment of salaries. |
SECU |
Securities Transaction is the payment of securities. |
SSBE |
SocialSecurityBenefit Transaction is a social security benefit, ie payment made by a government to support individuals. |
SUPP |
SupplierPayment Transaction is related to a payment to a supplier. |
TAXS |
TaxPayment Transaction is the payment of taxes. |
TRAD |
Trade Transaction is related to the payment of a trade finance transaction. |
TREA |
TreasuryPayment Transaction is related to treasury operations. E.g. financial contract settlement. |
VATX |
ValueAddedTaxPayment Transaction is the payment of value added tax. |
WHLD |
WithHolding Transaction is the payment of withholding tax. |
ChargeBearerCode
ISO20022: Specifies which party/parties will bear the charges associated with the processing of the payment transaction.
Base type
string
Enumerated Values
Value | Description |
---|---|
SLEV |
Service level. Charges are to be applied following the rules agreed in the service level and/or scheme. |
SHAR |
Shared. |
DEBT |
The Payer (sender of the payment) will bear all of the payment transaction fees. |
CRED |
The Payee (recipient of the payment) will incur all of the payment transaction fees. |
ClearingSystemMemberIdentification
ISO20022: Information used to identify a member within a clearing system. API: to be used for some specific international credit transfers in order to identify the beneficiary bank
Fields
Name | Type | Required | Description |
---|---|---|---|
clearingSystemId |
string |
false |
ISO20022: Specification of a pre-agreed offering between clearing agents or the channel through which the payment instruction is processed. |
memberId |
string |
false |
ISO20022: Identification of a member of a clearing system. |
ClientInfo
Client Data.
Fields
Name | Type | Required | Description |
---|---|---|---|
psuIpAddress |
string |
false |
IP address used by the PSU's terminal when connecting to the TPP |
psuIpPort |
string |
false |
IP port used by the PSU's terminal when connecting to the TPP |
psuHttpMethod |
string |
false |
Http method for the most relevant PSU’s terminal request to the TTP |
psuDate |
string |
false |
Timestamp of the most relevant PSU’s terminal request to the TTP |
psuUserAgent |
string |
false |
"User-Agent" header field sent by the PSU terminal when connecting to the TPP |
psuReferer |
string |
false |
"Referer" header field sent by the PSU terminal when connecting to the TPP. Notice that an initial typo in RFC 1945 specifies that "referer" (incorrect spelling) is to be used. The correct spelling "referrer" can be used but might not be understood. |
psuAccept |
string |
false |
"Accept" header field sent by the PSU terminal when connecting to the TPP |
psuAcceptCharset |
string |
false |
"Accept-Charset" header field sent by the PSU terminal when connecting to the TPP |
psuAcceptEncoding |
string |
false |
"Accept-Encoding" header field sent by the PSU terminal when connecting to the TPP |
psuAcceptLanguage |
string |
false |
"Accept-Language" header field sent by the PSU terminal when connecting to the TPP |
psuGeoLocation |
string |
false |
Geographical location of the PSU as provided by the PSU mobile terminal if any to the TPP |
psuDeviceId |
string |
false |
UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of installation identification this ID need to be unaltered until removal from device. |
psuLastLoggedTime |
string |
false |
The time when the PSU last logged in with the TPP. |
Connector
Information about the connector used for interaction with ASPSPs
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
false |
Name of the connector |
environments |
[string] |
false |
List of supported environments (sandbox/production) |
countries |
[string] |
false |
List of supported countries supported |
scopes |
[string] |
false |
List of supported APIs (aisp/pisp) supported |
modifyConsentsInfo |
false |
Details about usage of |
|
authInfo |
false |
Details about usage of |
|
consentRevocation |
boolean |
false |
Flag showing if the consent revocation is supported in the connector |
refreshToken |
boolean |
false |
Flag showing if the |
payments |
false |
Supported payment types by country |
|
aspspIds |
[string] |
false |
List of all ASPSP IDs supported in the connector |
requiredPsuHeaders |
[string] |
false |
List of required PSU headers |
ConnectorSettings
Base settings for all connectors
Fields
Name | Type | Required | Description |
---|---|---|---|
sandbox |
boolean |
true |
Defines whether connect to sandbox or production APIs |
consentId |
string |
true |
User consent identifier |
accessToken |
string |
true |
User access token |
redirectUri |
string |
true |
URI where clients are redirected to after giving consent. |
connector |
string |
true |
Connector identifier (discriminator) |
ConnectorsLinks
links that can be used for further navigation when browsing connector information
Fields
Name | Type | Required | Description |
---|---|---|---|
self |
true |
hypertext reference |
Consent
User consent
Fields
Name | Type | Required | Description |
---|---|---|---|
consentStatus |
string |
false |
none |
consentId |
string |
false |
none |
_links |
false |
none |
CoopPankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
xApiKey |
string |
true |
API client key (obtained in developer portal) |
country |
string |
true |
Bank`s country |
CoreException
Base Exception class in SDK, raised for unexpected exceptions.
Fields
Name | Type | Required | Description |
---|---|---|---|
code |
integer(int32) |
true |
Error code |
message |
string |
true |
Error description |
CountryAuthInfo
Auxiliary data structure binding information about usage of getAuth
method to a country
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code |
info |
true |
Information about usage of |
CountryModifyConsentsInfo
Auxiliary data structure binding information about usage of modifyConsents
method
to a country
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code |
info |
true |
Information about usage of |
CountryPaymentTypes
Auxiliary data structure containing binding information about supported payment types to a country
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Country ISO 3166-1 alpha-2 code |
paymentTypes |
true |
List of payment types for the country |
CreationDateTime
ISO20022: Date and time at which a (group of) payment instruction(s) was created by the instructing party.
Base type
string(date-time)
CreditTransferTransaction
ISO20022: Payment processes required to transfer cash from the debtor to the creditor. API:
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentId |
false |
ISO20022: Set of elements used to reference a payment instruction. |
|
requestedExecutionDate |
false |
ISO20022: Date at which the initiating party requests the clearing agent to process the payment. |
|
endDate |
false |
The last applicable day of execution for a given standing order. |
|
executionRule |
false |
Execution date shifting rule for standing orders |
|
frequency |
false |
Frequency rule for standing orders. |
|
instructedAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
exchangeRateInformation |
false |
Provides details on the currency exchange rate and contract. |
|
ultimateDebtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
beneficiary |
true |
Specification of a beneficiary |
|
ultimateCreditor |
false |
API : Description of a Party which can be either a person or an organization. |
|
regulatoryReportingCodes |
false |
List of needed regulatory reporting codes for international payments |
|
remittanceInformation |
false |
ISO20022: Information supplied to enable the matching of an entry with the items that the transfer is intended to settle, such as commercial invoices in an accounts' receivable system. |
|
transactionStatus |
false |
ISO20022: Specifies the status of the payment information group. |
|
statusReasonInformation |
false |
ISO20022: Provides detailed information on the status reason. Can only be used in status equal to "RJCT". |
CurrencyCode
Specifies the currency of the amount or of the account. A code allocated to a currency by a Maintenance Agency under an international identification scheme, as described in the latest edition of the international standard ISO 4217 "Codes for the representation of currencies and funds".
Base type
string
EndDate
The last applicable day of execution for a given standing order. If not given, the standing order is considered as endless.
Base type
string(date-time)
ExchangeRate
Provides details on the currency exchange rate and contract.
Fields
Name | Type | Required | Description |
---|---|---|---|
unitCurrency |
false |
Currency in which the rate of exchange is expressed in a currency exchange. In the example 1GBP = xxxCUR, the unit currency is GBP. |
|
exchangeRate |
string(number) |
false |
The factor used for conversion of an amount from one currency to another. This reflects the price at which one currency was bought with another currency. |
rateType |
string |
false |
none |
contractIdentification |
string |
false |
Unique and unambiguous reference to the foreign exchange contract agreed between the initiating party/creditor and the debtor agent. |
Enumerated Values
rateType
Value | Description |
---|---|
SPOT |
Spot – Exchange rate applied is the spot rate. |
SALE |
Sale – Exchange rate applied is the market rate at the time of the sale. |
AGRD |
Agreed – Exchange rate applied is the rate agreed between the parties. |
ExecutionRule
Execution date shifting rule for standing orders This data attribute defines the behaviour when recurring payment dates falls on a weekend or bank holiday. The payment is then executed either the "preceding" or "following" working day. ASPSP might reject the request due to the communicated value, if rules in Online-Banking are not supporting this execution rule.
Base type
string
Enumerated Values
Value | Description |
---|---|
FWNG |
following |
PREC |
preceding |
FinancialInstitutionIdentification
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme.
Fields
Name | Type | Required | Description |
---|---|---|---|
bicFi |
string |
true |
ISO20022: Code allocated to a financial institution by the ISO 9362 Registration Authority as described in ISO 9362 "Banking - Banking telecommunication messages - Business identification code (BIC)". |
clearingSystemMemberId |
false |
ISO20022: Information used to identify a member within a clearing system. |
|
name |
string |
false |
Name of the financial institution |
postalAddress |
false |
ISO20022 : Information that locates and identifies a specific address, as defined by postal services. |
FrequencyCode
Frequency rule for standing orders. The following codes from the "EventFrequency7Code" of ISO 20022 are supported. However, each ASPSP might restrict these values into a subset.
Base type
string
Enumerated Values
Value | Description |
---|---|
DAIL |
Daily |
WEEK |
Weekly |
TOWK |
EveryTwoWeeks |
MNTH |
Monthly |
TOMN |
EveryTwoMonths |
QUTR |
Quarterly |
SEMI |
SemiAnnual |
YEAR |
Annual |
FundsAvailabilityInformation
indicator that the payment can be covered or not by the funds available on the relevant account
- true: payment is covered
- false: payment is not covered
Base type
boolean
GenericIdentification
ISO20022: Unique identification of an account, a person or an organisation, as assigned by an issuer. API: The ASPSP will document which account reference type it will support.
Fields
Name | Type | Required | Description |
---|---|---|---|
identification |
string |
true |
API Identifier |
schemeName |
string |
true |
Name of the identification scheme. Partially based on ISO20022 external code list |
issuer |
string |
false |
ISO20022: Entity that assigns the identification. this could a country code or any organisation name or identifier that can be recognized by both parties |
Enumerated Values
schemeName
Value | Description |
---|---|
CHID |
Clearing Identification Number |
GS1G |
GS1GLNIdentifier |
DUNS |
Data Universal Numbering System |
BANK |
BankPartyIdentification. Unique and unambiguous assignment made by a specific bank or similar financial institution to identify a relationship as defined between the bank and its client. |
TXID |
TaxIdentificationNumber |
CUST |
CorporateCustomerNumber |
EMPL |
EmployerIdentificationNumber |
OTHC |
OtherCorporate. Handelsbanken-specific code |
DRLC |
DriversLicenseNumber |
CUSI |
CustomerIdentificationNumberIndividual. Handelsbanken-specific code |
SOSE |
SocialSecurityNumber |
ARNU |
AlienRegistrationNumber |
CCPT |
PassportNumber |
TXII |
TaxIdentificationNumberIndividual. Handelsbanken-specific code |
EMPI |
EmployeeIdentificationNumberIndividual. Handelsbanken-specific code |
OTHI |
OtherIndividual. Handelsbanken-specific code |
COID |
CountryIdentificationCode. Country authority given organisation identification (e.g., corporate registration number) |
SREN |
The SIREN number is a 9 digit code assigned by INSEE, the French National Institute for Statistics and Economic Studies, to identify an organisation in France. |
SRET |
The SIRET number is a 14 digit code assigned by INSEE, the French National Institute for Statistics and Economic Studies, to identify an organisation unit in France. It consists of the SIREN number, followed by a five digit classification number, to identify the local geographical unit of that entity. |
NIDN |
NationalIdentityNumber. Number assigned by an authority to identify the national identity number of a person. |
OAUT |
OAUTH2 access token that is owned by the PISP being also an AISP and that can be used in order to identify the PSU |
CPAN |
Card PAN |
BBAN |
Basic Bank Account Number. Represents a country-specific bank account number. |
BG |
Swedish BankGiro account number. Used in domestic swedish giro payments |
PG |
Swedish PlusGiro account number. Used in domestic swedish giro payments |
HalAccounts
HYPERMEDIA structure used for returning the list of the available accounts to the AISP
Fields
Name | Type | Required | Description |
---|---|---|---|
connectedPsu |
string |
false |
Last name and first name that has granted access to the AISP on the accounts data This information can be retrieved based on the PSU's authentication that occurred during the OAUTH2 access token initialisation. |
accounts |
true |
List of PSU account that are made available to the TPP |
|
_links |
true |
Links that can be used for further navigation when browsing Account Information at top |
HalBalances
HYPERMEDIA structure used for returning the list of the relevant balances for a given account to the AISP
Fields
Name | Type | Required | Description |
---|---|---|---|
balances |
true |
List of account balances |
|
_links |
true |
links that can be used for further navigation when browsing Account Information at one |
HalBeneficiaries
HYPERMEDIA structure used for returning the list of the whitelisted beneficiaries
Fields
Name | Type | Required | Description |
---|---|---|---|
beneficiaries |
true |
List of trusted beneficiaries |
|
_links |
true |
links that can be used for further navigation when browsing Account Information at one |
HalConnectors
HYPERMEDIA structure used for returning the list of the connectors for given countries
Fields
Name | Type | Required | Description |
---|---|---|---|
connectors |
true |
List of connectors |
|
_links |
false |
links that can be used for further navigation when browsing connector information |
HalPaymentCoverageReport
HYPERMEDIA structure used for returning the payment coverage report to the CBPII
Fields
Name | Type | Required | Description |
---|---|---|---|
request |
true |
Payment coverage request structure. The request must rely either on a cash account or a payment card. |
|
result |
boolean |
true |
Result of the coverage check :
|
_links |
true |
links that can be used for further navigation to post another coverage request. |
HalPaymentRequest
HYPERMEDIA structure used for returning the original Payment Request to the PISP
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentRequest |
true |
ISO20022: The PaymentRequestResource message is sent by the Creditor sending party to the Debtor receiving party, directly or through agents. It is used by a Creditor to request movement of funds from the debtor account to a creditor. |
|
_links |
true |
links that can be used for further navigation when having post a Payment Request in order |
HalPaymentRequestCreation
data forwarded by the ASPSP to the PISP after creation of the Payment Request resource creation
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentRequestResourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
appliedAuthenticationApproach |
false |
The ASPSP, based on the authentication approaches proposed by the PISP, choose the one that it can processed, in respect with the preferences and constraints of the PSU and indicates in this field which approach has been chosen |
|
_links |
false |
Links that can be used for further navigation, especially in REDIRECT approach |
HalTransactions
HYPERMEDIA structure used for returning the list of the transactions for a given account to the AISP
Fields
Name | Type | Required | Description |
---|---|---|---|
transactions |
true |
List of transactions |
|
continuationKey |
string |
false |
Value to be passed to getAccountTransactions to retrieve next page of transactions. Null if there are no more pages. Only valid in current session. |
_links |
true |
links that can be used for further navigation when browsing Account Information at one |
InvalidResponseIdException
This exception is raised when ASPSP API response id does not match request id in.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
requestId |
string |
true |
Request id |
InvalidResponseSignatureException
This exception is raised when ASPSP API response signature verification failed.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
jws |
string |
true |
JSON web signature |
LocalInstrumentCode
ISO20022: User community specific instrument. Usage: This element is used to specify a local instrument, local clearing option and/or further qualify the service or service level.
Base type
string
Logo
ASPSP logo, which can be used for showing to an end-user
Fields
Name | Type | Required | Description |
---|---|---|---|
url |
string |
true |
Logo URL |
MeridianBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
xApiKey |
string |
true |
API client key (obtained in developer portal) |
country |
string |
true |
Bank`s country |
ModifyConsentsInfo
Information about usage of modifyConsents
method
Fields
Name | Type | Required | Description |
---|---|---|---|
beforeAccounts |
boolean |
true |
Shows if |
extendsDetails |
boolean |
true |
Shows if bank returns extended information about accounts from method |
accountsRequired |
boolean |
true |
Shows if |
PartyIdentification
API : Description of a Party which can be either a person or an organization.
Fields
Name | Type | Required | Description |
---|---|---|---|
name |
string |
false |
ISO20022: Name by which a party is known and which is usually used to identify that party. |
postalAddress |
false |
ISO20022 : Information that locates and identifies a specific address, as defined by postal services. |
|
organisationId |
false |
Unique and unambiguous way to identify an organisation. |
|
privateId |
false |
Unique and unambiguous identification of a person. |
PaymentCoverageRequestResource
Payment coverage request structure. The request must rely either on a cash account or a payment card.
Fields
Name | Type | Required | Description |
---|---|---|---|
paymentCoverageRequestId |
string |
true |
Identification of the payment Coverage Request |
payee |
string |
false |
The merchant where the card is accepted as information to the PSU. |
instructedAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
accountId |
true |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
PaymentIdentification
ISO20022: Set of elements used to reference a payment instruction.
Fields
Name | Type | Required | Description |
---|---|---|---|
resourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
instructionId |
string |
false |
ISO20022: Unique identification as assigned by an instructing party for an instructed party to unambiguously identify the instruction. API: Unique identification shared between the PISP and the ASPSP |
endToEndId |
string |
false |
ISO20022: Unique identification assigned by the initiating party to unambiguously identify the transaction. This identification is passed on, unchanged, throughout the entire end-to-end chain. API: Unique identification shared between the merchant and the PSU |
PaymentInformationId
ISO20022 : Reference assigned by a sending party to unambiguously identify the payment information block within the message.
Base type
string
PaymentInformationStatusCode
ISO20022: Specifies the status of the payment information.
Base type
string
Enumerated Values
Value | Description |
---|---|
ACCP |
AcceptedCustomerProfile. Preceding check of technical validation was successful. Customer profile check was also successful. |
ACSC |
AcceptedSettlementCompleted. Settlement on the debtor's account has been completed. |
ACSP |
AcceptedSettlementInProcess. All preceding checks such as technical validation and customer profile were successful. Dynamic risk assessment is now also successful and therefore the Payment Request has been accepted for execution. |
ACTC |
AcceptedTechnicalValidation. Authentication and syntactical and semantical validation are successful. |
ACWC |
AcceptedWithChange. Instruction is accepted but a change will be made, such as date or remittance not sent. |
ACWP |
AcceptedWithoutPosting. Payment instruction included in the credit transfer is accepted without being posted to the creditor customer’s account. |
PART |
PartiallyAccepted. A number of transactions have been accepted, whereas another number of transactions have not yet achieved 'accepted' status. |
RCVD |
Received. Payment initiation has been received by the receiving agent. |
PDNG |
Pending. Payment request or individual transaction included in the Payment Request is pending. Further checks and status update will be performed. |
RJCT |
Rejected. Payment request has been rejected. |
PaymentRequestConfirmation
Contains data for confirmation of payment request
Fields
Name | Type | Required | Description |
---|---|---|---|
psuAuthenticationFactor |
string |
false |
Authentication factor forwarded by the TPP to the ASPSP in order to fulfil the strong customer authentication process |
paymentRequest |
false |
Original payment request used to initiate the payment |
PaymentRequestResource
ISO20022: The PaymentRequestResource message is sent by the Creditor sending party to the Debtor receiving party, directly or through agents. It is used by a Creditor to request movement of funds from the debtor account to a creditor. API: Information about the creditor (Id, account and agent) might be placed either at payment level or at instruction level. Thus multi-beneficiary payments can be handled. The requested execution date can be placed either at payment level when all instructions are requested to be executed at the same date or at instruction level. The latest case includes:
- multiple instructions having different requested execution dates
- standing orders settings
Fields
Name | Type | Required | Description |
---|---|---|---|
resourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
paymentInformationId |
false |
ISO20022 : Reference assigned by a sending party to unambiguously identify the payment information block within the message. |
|
creationDateTime |
false |
ISO20022: Date and time at which a (group of) payment instruction(s) was created by the instructing party. |
|
initiatingParty |
false |
API : Description of a Party which can be either a person or an organization. |
|
paymentTypeInformation |
false |
ISO20022: Set of elements used to further specify the type of transaction. |
|
debtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
debtorAccount |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
debtorAgent |
false |
ISO20022: Unique and unambiguous identification of a financial institution, as assigned under an internationally recognised or proprietary identification scheme. |
|
debtorCurrency |
string |
false |
Debtor account currency code |
purpose |
false |
ISO20022: Underlying reason for the payment transaction, as published in an external purpose code list. |
|
chargeBearer |
false |
ISO20022: Specifies which party/parties will bear the charges associated with the processing of the payment transaction. |
|
paymentInformationStatus |
false |
ISO20022: Specifies the status of the payment information. |
|
statusReasonInformation |
false |
ISO20022: Provides detailed information on the status reason. Can only be used in status equal to "RJCT". |
|
fundsAvailability |
false |
indicator that the payment can be covered or not by the funds available on the relevant account |
|
booking |
false |
indicator that the payment can be immediately booked or not |
|
creditTransferTransaction |
true |
ISO20022: Payment processes required to transfer cash from the debtor to the creditor. API: Each ASPSP will specify a maxItems value for this field taking into accounts its specificities about payment request handling |
PaymentTypeInformation
ISO20022: Set of elements used to further specify the type of transaction.
Fields
Name | Type | Required | Description |
---|---|---|---|
instructionPriority |
false |
ISO20022: Indicator of the urgency or order of importance that the instructing party would like the instructed party to apply to the processing of the instruction. |
|
serviceLevel |
false |
ISO20022: Agreement under which or rules under which the transaction should be processed. Specifies a pre-agreed service or level of service between the parties, as published in an external service level code list. |
|
categoryPurpose |
false |
ISO20022: Specifies the high level purpose of the instruction based on a set of pre-defined categories. This is used by the initiating party to provide information concerning the processing of the payment. It is likely to trigger special processing by any of the agents involved in the payment chain. |
|
localInstrument |
false |
ISO20022: User community specific instrument. |
PostalAddress
ISO20022 : Information that locates and identifies a specific address, as defined by postal services.
Fields
Name | Type | Required | Description |
---|---|---|---|
addressType |
false |
ISO20022: Identifies the nature of the postal address. |
|
department |
string |
false |
Identification of a division of a large organisation or building. |
subDepartment |
string |
false |
Identification of a sub-division of a large organisation or building. |
streetName |
string |
false |
Name of a street or thoroughfare. |
buildingNumber |
string |
false |
Number that identifies the position of a building on a street. |
postCode |
string |
false |
Identifier consisting of a group of letters and/or numbers that is added to a postal address to assist the sorting of mail. |
townName |
string |
false |
Name of a built-up area, with defined boundaries, and a local government. |
countrySubDivision |
string |
false |
Identifies a subdivision of a country such as state, region, county. |
country |
string |
true |
ISO20022: Country in which a person resides (the place of a person's home). In the case of a company, it is the country from which the affairs of that company are directed. |
addressLine |
[string] |
false |
Unstructured address. The two lines must embed zip code and town name |
PriorityCode
ISO20022: Indicator of the urgency or order of importance that the instructing party would like the instructed party to apply to the processing of the instruction.
Base type
string
Enumerated Values
Value | Description |
---|---|
HIGH |
High priority |
NORM |
Normal priority |
EXPR |
Express priority. Polish-specific priority code |
PrivatBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
xApiKey |
string |
true |
API client key (obtained in developer portal) |
country |
string |
true |
Bank`s country |
PurposeCode
ISO20022: Underlying reason for the payment transaction, as published in an external purpose code list.
Base type
string
Enumerated Values
Value | Description |
---|---|
ACCT |
Funds moved between 2 accounts of same account holder at the same bank. |
CASH |
general cash management instruction, may be used for Transfer Initiation. |
COMC |
Transaction is related to a payment of commercial credit or debit. |
CPKC |
General Carpark Charges Transaction is related to carpark charges. |
TRPT |
Transport RoadPricing Transaction is for the payment to top-up pre-paid card and electronic road pricing for the purpose of transportation. |
RegulatoryReportingCode
Information needed due to regulatory and statutory requirements. Economical codes to be used are provided by the National Competent Authority
Base type
string
RegulatoryReportingCodes
List of needed regulatory reporting codes for international payments
Base type
RequestedExecutionDate
ISO20022: Date at which the initiating party requests the clearing agent to process the payment. API: This date can be used in the following cases:
- the single requested execution date for a payment having several instructions. In this case, this field must be set at the payment level.
- the requested execution date for a given instruction within a payment. In this case, this field must be set at each instruction level.
- The first date of execution for a standing order. When the payment cannot be processed at this date, the ASPSP is allowed to shift the applied execution date to the next possible execution date for non-standing orders. For standing orders, the [executionRule] parameter helps to compute the execution date to be applied.
Base type
string(date-time)
ResourceId
Identifier assigned by the ASPSP for further use of the created resource through API calls
Base type
string
ServiceLevelCode
ISO20022: Agreement under which or rules under which the transaction should be processed. Specifies a pre-agreed service or level of service between the parties, as published in an external service level code list.
Base type
string
Enumerated Values
Value | Description |
---|---|
BKTR |
Book Transaction – Payment through internal book transfer. |
G001 |
TrackedCustomerCreditTransfer – Tracked Customer Credit Transfer |
G002 |
TrackedStopAndRecall – Tracked Stop and Recall |
G003 |
TrackedCorporateTransfer – Tracked Corporate Transfer |
G004 |
TrackedFinancialInstitutionTransfer – Tracked Financial Institution Transfer |
NUGP |
Non-urgent Priority Payment – Payment must be executed as a non-urgent transaction with priority settlement. |
NURG |
Non-urgent Payment – Payment must be executed as a non-urgent transaction, which is typically identified as an ACH or low value transaction. |
PRPT |
EBAPriorityService – Transaction must be processed according to the EBA Priority Service. |
SDVA |
SameDayValue – Payment must be executed with same day value to the creditor. |
SEPA |
SingleEuroPaymentsArea – Payment must be executed following the Single Euro Payments Area scheme. |
SVDE |
Domestic Cheque Clearing and Settlement – Payment execution following the cheque agreement and traveller cheque agreement of the German Banking Industry Committee (Die Deutsche Kreditwirtschaft - DK) and Deutsche Bundesbank – Scheck Verrechnung Deutschland |
URGP |
Urgent Payment – Payment must be executed as an urgent transaction cleared through a real-time gross settlement system, which is typically identified as a wire or high value transaction. |
URNS |
Urgent Payment Net Settlement – Payment must be executed as an urgent transaction cleared through a real-time net settlement system, which is typically identified as a wire or high value transaction. |
SignetBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
xApiKey |
string |
true |
API client key (obtained in developer portal) |
country |
string |
true |
Bank`s country |
StatusReasonInformation
ISO20022: Provides detailed information on the status reason. Can only be used in status equal to "RJCT".
Base type
string
Enumerated Values
Value | Description |
---|---|
AC01 |
IncorectAccountNumber. the account number is either invalid or does not exist |
AC04 |
ClosedAccountNumber. the account is closed and cannot be used |
AC06 |
BlockedAccount. the account is blocked and cannot be used |
AG01 |
Transaction forbidden. Transaction forbidden on this type of account |
CH03 |
RequestedExecutionDateOrRequestedCollectionDateTooFarInFuture. The requested execution date is too far in the future |
CUST |
RequestedByCustomer. The reject is due to the debtor (refusal or lack of liquidity) |
DS02 |
OrderCancelled. An authorized user has cancelled the order |
FF01 |
InvalidFileFormat. The reject is due to the original Payment Request which is invalid (syntax, structure or values) |
FRAD |
FraudulentOriginated. the Payment Request is considered as fraudulent |
MS03 |
NotSpecifiedReasonAgentGenerated. No reason specified by the ASPSP |
NOAS |
NoAnswerFromCustomer. The PSU has neither accepted nor rejected the Payment Request and a time-out has occurred |
RR01 |
MissingDebtorAccountOrIdentification. The Debtor account and/or Identification are missing or inconsistent |
RR03 |
MissingCreditorNameOrAddress. Specification of the creditor’s name and/or address needed for regulatory requirements is insufficient or missing. |
RR04 |
RegulatoryReason. Reject from regulatory reason |
RR12 |
InvalidPartyID. Invalid or missing identification required within a particular country or payment type. |
Token
Fields
Name | Type | Required | Description |
---|---|---|---|
access_token |
string |
false |
The access token value |
token_type |
string |
false |
Type of the token is set to "Bearer" |
expires_in |
integer(int32) |
false |
The lifetime in seconds of the access token |
refresh_token |
string |
false |
The refresh token value |
scope |
string |
false |
Scopes of the token |
Enumerated Values
token_type
Value | Description |
---|---|
Bearer |
— |
Transaction
structure of a transaction
Fields
Name | Type | Required | Description |
---|---|---|---|
resourceId |
false |
Identifier assigned by the ASPSP for further use of the created resource through API calls |
|
entryReference |
string |
false |
Technical incremental identification of the transaction. |
transactionAmount |
true |
ISO20022: structure aiming to carry either an instructed amount or equivalent amount. Both structures embed the amount and the currency to be used. |
|
creditor |
false |
API : Description of a Party which can be either a person or an organization. |
|
creditorAccount |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
debtor |
false |
API : Description of a Party which can be either a person or an organization. |
|
debtorAccount |
false |
Unique and unambiguous identification for the account between the account owner and the account servicer. |
|
bankTransactionCode |
false |
ISO20022: Allows the account servicer to correctly report a transaction, which in its turn will help account owners to perform their cash management and reconciliation operations. |
|
creditDebitIndicator |
string |
true |
Accounting flow of the transaction |
status |
true |
Type of Transaction |
|
bookingDate |
string(date) |
false |
Booking date of the transaction on the account |
valueDate |
string(date) |
false |
Value date of the transaction on the account |
transactionDate |
string(date) |
false |
Date used for specific purposes:
|
remittanceInformation |
false |
ISO20022: Information supplied to enable the matching of an entry with the items that the transfer is intended to settle, such as commercial invoices in an accounts' receivable system. |
Enumerated Values
creditDebitIndicator
Value | Description |
---|---|
CRDT |
Credit type transaction |
DBIT |
Debit type transaction |
TransactionIndividualStatusCode
ISO20022: Specifies the status of the payment information group.
Base type
string
Enumerated Values
Value | Description |
---|---|
RCVD |
Payment request or individual transaction included in the Payment Request has been received by the receiving agent. |
RJCT |
Payment request or individual transaction included in the Payment Request has been rejected. |
PDNG |
Pending. Payment request or individual transaction included in the Payment Request is pending. Further checks and status update will be performed. |
ACSP |
All preceding checks such as technical validation and customer profile were successful and therefore the Payment Request has been accepted for execution. |
ACSC |
Settlement on the debtor's account has been completed. |
TransactionStatus
Type of Transaction
Base type
string
Enumerated Values
Value | Description |
---|---|
BOOK |
(ISO20022 ClosingBooked) Accounted transaction |
CNCL |
Cancelled transaction |
HOLD |
Account hold |
OTHR |
Transaction with unknown status or not fitting the other options |
PDNG |
(ISO20022 Expected) Instant Balance Transaction |
RJCT |
Rejected transaction |
SCHD |
Scheduled transaction |
TransactionsAccess
Defines what access to transactions is requested
Fields
Name | Type | Required | Description |
---|---|---|---|
statuses |
false |
List of transaction statuses, which are going to be accessed |
|
details |
boolean |
false |
Indicator that access to transaction details is requested |
limitDays |
integer |
false |
Number of days in the past to access transactions |
UnstructuredRemittanceInformation
ISO20022: Information supplied to enable the matching of an entry with the items that the transfer is intended to settle, such as commercial invoices in an accounts' receivable system. API: Only one occurrence is allowed
Fields
Name | Type | Required | Description |
---|---|---|---|
remittanceLine |
string |
false |
Relevant information to the transaction |
HAL
AccountLinks
links that can be used for further navigation when browsing Account Information at one account level
Fields
Name | Type | Required | Description |
---|---|---|---|
balances |
false |
link to the balances of a given account |
|
transactions |
false |
link to the transactions of a given account |
AccountsLinks
Links that can be used for further navigation when browsing Account Information at top level
Fields
Name | Type | Required | Description |
---|---|---|---|
self |
true |
link to the list of all available accounts |
|
beneficiaries |
false |
hypertext reference |
|
first |
false |
hypertext reference |
|
last |
false |
hypertext reference |
|
next |
false |
hypertext reference |
|
prev |
false |
hypertext reference |
BalancesLinks
links that can be used for further navigation when browsing Account Information at one account level
Fields
Name | Type | Required | Description |
---|---|---|---|
self |
true |
link to the balances of a given account |
|
parent-list |
false |
link to the list of all available accounts |
|
transactions |
false |
link to the transactions of a given account |
BeneficiariesLinks
links that can be used for further navigation when browsing Account Information at one account level
Fields
Name | Type | Required | Description |
---|---|---|---|
self |
true |
link to the beneficiaries |
|
parent-list |
false |
link to the list of all available accounts |
|
first |
false |
link to the first page of the beneficiaries result |
|
last |
false |
link to the last page of the beneficiaries result |
|
next |
false |
link to the next page of the beneficiaries result |
|
prev |
false |
link to the previous page of the beneficiaries result |
ConsentLinks
Fields
Name | Type | Required | Description |
---|---|---|---|
redirect |
false |
hypertext reference |
|
self |
false |
hypertext reference |
|
status |
false |
hypertext reference |
GenericLink
hypertext reference
Fields
Name | Type | Required | Description |
---|---|---|---|
href |
string |
true |
URI to be used |
templated |
boolean |
false |
specifies "true" if href is a URI template, i.e. with parameters. Otherwise, this property is absent or set to false |
PaymentCoverageReportLinks
links that can be used for further navigation to post another coverage request.
Fields
Name | Type | Required | Description |
---|---|---|---|
self |
true |
hypertext reference |
PaymentRequestLinks
links that can be used for further navigation when having post a Payment Request in order to get the relevant status report.
Fields
Name | Type | Required | Description |
---|---|---|---|
self |
false |
hypertext reference |
|
confirmation |
false |
This link shall not been provided when the confirmation has already been posted. |
PaymentRequestResourceCreationLinks
Links that can be used for further navigation, especially in REDIRECT approach
Fields
Name | Type | Required | Description |
---|---|---|---|
consentApproval |
false |
URL to be used by the PISP in order to start the ASPSP authentication and consent management process |
|
confirmation |
false |
Link to the payment confirmation API endpoint |
TransactionsLinks
links that can be used for further navigation when browsing Account Information at one account level
Fields
Name | Type | Required | Description |
---|---|---|---|
self |
true |
link to the transactions of a given account |
|
parent-list |
false |
link to the list of all available accounts |
|
balances |
false |
link to the balances of a given account |
|
first |
false |
link to the first page of the transactions result |
|
last |
false |
link to the last page of the transactions result |
|
next |
false |
link to the next page of the transactions result |
|
prev |
false |
link to the previous page of the transactions result |
Exceptions
AuthorizationCancelledException
This exception is raised when authorization was cancelled either by ASPSP or by user.
Base type
DataRetrievalException
This exception is raised when unable to retrieve data requested from ASPSP API.
Base type
HttpException
Code samples
{}
This exception is raised when ASPSP API responses with unspecified error. Base exception for HTTP related errors
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
responseHeaders |
[object] |
true |
Headers of http response |
responseBody |
string |
true |
Body of http response |
InsufficientScopeException
This exception is raised when consent given by the PSU does not allow to retrieve
requested data.
Base type
MakeTokenException
This exception is raised when ASPSP API is not ready yet. Flag retry
shows if it is required to retry method call.
Base type
ModelValidationException
This exception is raised when model validation failed.
Base type
NotImplementedException
This exception is raised when called method is not implemented in SDK(either not supported by ASPSP API or integration is not ready yet).
Base type
ParameterValidationException
This exception is raised when function parameter validation failed.
Base type
ResourceNotFoundException
This exception is raised when unable to load certificate/private key from path.
Base type
ResponseValidationException
This exception is raised when ASPSP API response validation failed.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
responseHeaders |
[object] |
true |
Headers of http response |
responseBody |
string |
true |
Body of http response |
UnauthorizedException
This exception is raised when unauthorized to retrieve data requested from ASPSP API (e.g. access token expired).
Base type
ValidationException
Base class for validation related exceptions.
Base type
Connectors
AktiaConnectorSettings
Settings for initializing ApiClient for Aktia (Finland).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Aktia Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Aktia Developer Portal) |
sandboxPaymentId |
string |
false |
ID of the payment request used in sandbox (sandbox only) |
sandboxSafeguard |
boolean |
false |
Flag whether to fix malformed sandbox data or not (sandbox only) |
AktiaContingencyConnectorSettings
Settings for initializing ApiClient for Aktia Contingency API (Finland).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
authorizationHeader |
string |
true |
Authorization header obtained from Aktia onboarding(without "Basic") |
AlandsbankenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AlandsbankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new AlandsbankenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.xApiKey("put-your-x-api-key-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for Ålandsbanken (Finland).
Credentials can obtained at Open Banking Market operated by Crosskey Banking Solutions.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
xApiKey |
string |
true |
API client key (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. Test certificate (for accessing sandbox environment) can be downloaded from Open Banking Market. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be presented as a decimal string. The following command can be use for extracting it from QSeal certificate in PEM format: |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
AliorConnectorSettings
Settings for initializing ApiClient for Alior Bank (Poland).
Credentials can obtained at Alior Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Alior Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Alior Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded with padding) |
signCertUrl |
string |
true |
Public URL where QSeal certificate can be found |
paymentAuthRedirectUri |
string |
false |
Payment redirect URI |
paymentAuthState |
string |
false |
Payment authentication state |
AlmBrandConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.AlmBrandConnectorSettings;
ApiClient apiClient = new ApiClient(
new AlmBrandConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for AlmBrand
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
AndelskassenFaelleskassenConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
ArbejdernesLandsbankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
BIBConnectorSettings
Settings for initializiong ApiClient for [Baltic International Bank] (https://www.bib.eu/)
Credentials can be obtained at Baltic International Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank`s country |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL public certificate string |
BILDanmarkConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
BNPParibasPolandConnectorSettings
Settings for initializing ApiClient for BNP Paribas Bank Polska (Poland).
Credentials can obtained at BNP Paribas Poland Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from BNP Paribas Poland Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from BNP Paribas Poland Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded without padding) |
signCertUrl |
string |
true |
Public URL where QSeal certificate can be found |
tppId |
string |
true |
ID assigned to the TPP by an authority (format: PSDXX-YYYY-ZZZZZZZZ) |
paymentAuthRedirectUri |
string |
false |
Payment redirect URI |
paymentAuthState |
string |
false |
Payment authentication state |
BanxeConnectorSettings
Settings for initializing ApiClient for Banxe (LT)
Credentials can obtained at Banxe (LT) Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Banxe Developer Portal ("Key")) |
clientSecret |
string |
true |
API client secret (obtained from Banxe Developer Portal ("Secret")) |
paymentAuthRedirectUri |
string |
false |
Payment redirect URI |
paymentAuthState |
string |
false |
Payment authentication state |
CitadeleConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
sandboxConsentId |
string |
false |
Static consent id with approved status used in sandbox |
CoopBankDenmarkConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SydbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SydbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
DanskeAndelskassersBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
DanskeBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DanskeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new DanskeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for DanskeBank
Credentials can obtained at Danske Bank Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
DenJyskeSparekasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
DjurslandsBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.DjurslandsBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new DjurslandsBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for DjurslandsBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
FasterAndelskasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
FrorupAndelskasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
FroslevMollerupSparekasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
FynskeBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
HandelsbankenConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client id (obtained from Handelsbanken developer portal) |
certPath |
string |
true |
Path to a QWAC public certificate |
keyPath |
string |
true |
Path to a QWAC private key |
country |
string |
true |
Bank`s country |
paymentAuthRedirectUri |
string |
false |
Redirect uri for payment (PISP) authorization flow |
paymentAuthState |
string |
false |
State for payment (PISP) authorization flow |
HandelsbankenDenmarkConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
HolviConnectorSettings
Settings for initializing ApiClient for Holvi (Finland).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Holvi onboarding) |
clientSecret |
string |
true |
API client secret (obtained from Holvi onboarding) |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
HvidbjergBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
ICABankenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ICABankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new ICABankenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.sandbox(true));
Settings for initializing ApiClient for ICA Banken.
Credentials can obtained at ICA Banken Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from ICA Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from ICA Developer Portal) |
certPath |
string |
false |
Path to QWAC certificate in PEM format. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. |
tppId |
string |
false |
none |
INGPolandConnectorSettings
Settings for initializing ApiClient for ING Silesian Bank (Poland).
Credentials can obtained at ING Silesian Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from ING Silesian Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signFingerprint |
string |
true |
Fingerprint of the QSeal certificate (base64 encoded without padding) |
signCertUrl |
string |
true |
Public URL where QSeal certificate can be found |
tppId |
string |
true |
ID assigned to the TPP by an authority (format: PSDXX-YYYY-ZZZZZZZZ) |
paymentAuthRedirectUri |
string |
false |
Payment redirect URI |
paymentAuthState |
string |
false |
Payment authentication state |
JyskeBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.JyskeBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new JyskeBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for JyskeBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
KIRConnectorSettings
Setting for the connector to PolishAPI implementation provided by KIR.
Originally implemented for openbankinghackathon.pl
Sandbox only
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
origin |
string |
true |
API origin (scheme://domain:port) |
clientId |
string |
true |
TPP ID to be put here. KIR sandbox certificates use PSDPL-KNF-1234567890 |
certPath |
string |
true |
Path to sandbox QWAC certificate |
keyPath |
string |
true |
Path to sandbox key used for QWAC |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. If not set request signing will be |
signSubjectKeyIdentifier |
string |
false |
Subject Key Identifier (2.5.29.14) field of QSeal certificate as hex string |
signFingerprint |
string |
false |
Fingerprint of the QSeal certificate (base64 encoded without padding) |
signCertUrl |
string |
false |
Public URL where QSeal certificate can be found |
paymentAuthRedirectUri |
string |
false |
Payment redirect URI |
paymentAuthState |
string |
false |
Payment authentication state |
KomplettConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
none |
xApiKey |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
paymentAuthRedirectUri |
string |
false |
none |
paymentAuthState |
string |
false |
none |
KreditbankenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.KreditbankenConnectorSettings;
ApiClient apiClient = new ApiClient(
new KreditbankenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for Kreditbanken
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
LHVConnectorSettings
Settings for initializing ApiClient for LHV (Estonia).
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
caCertPath |
string |
true |
Path to CA (QWAC issuer) certificate in PEM format |
clientId |
string |
true |
API client ID |
LaegernesBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
LandbobankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LandbobankConnectorSettings;
ApiClient apiClient = new ApiClient(
new LandbobankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for Landbobank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
LansforsakringarConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.LansforsakringarConnectorSettings;
ApiClient apiClient = new ApiClient(
new LansforsakringarConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.sandbox(true));
Settings for initializing ApiClient for Lansforsakringar.
Credentials can obtained at Lansforsakringar Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from ICA Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from ICA Developer Portal) |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Optional in sandbox, but required in production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Optional in sandbox, but required in production. |
nokRedirectUri |
string |
false |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. Required for production. |
LollandsBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
LuminorConnectorSettings
Settings for initializing ApiClient for Luminor Estonia, Luminor Latvia, Luminor Lithuania.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID |
clientSecret |
string |
true |
API client secret |
companyName |
string |
true |
Company name displayed to the PSU during authorization |
MajBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
MedicinosBankasConnectorSettings
Settings for initializiong ApiClient for [Medicinos Bankas] (https://www.medbank.lt/)
Credentials can be obtained at Medicinos Bankas Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
MerkurAndelskasseConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
MonsBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
NordeaConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordeaConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordeaConnectorSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.signKeyPath("path/to/qseal-key.pem")
.country("FI")
.language("fi")
.redirectUri("https://your-redirect-uri")
.sandbox(true));
Settings for initializing ApiClient for Nordea (all countries).
Credentials can obtained at Nordea Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
API client ID (obtained from Nordea Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Nordea Developer Portal) |
signKeyPath |
string |
true |
Path to Qseal private key in PEM format. |
language |
string |
false |
Language of authentication user interface. If country is |
paymentAuthRedirectUri |
string |
false |
State for payment (PISP) authorization flow |
paymentAuthState |
string |
false |
Redirect uri for payment (PISP) authorization flow |
NordfynsBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.NordfynsBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new NordfynsBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for NordfynsBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
NykreditBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
OPConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
apiKey |
string |
true |
API key |
clientId |
string |
true |
Client id |
clientSecret |
string |
true |
Client secret |
certPath |
string |
true |
Path to a QWAC |
keyPath |
string |
true |
Path to a QWAC private key |
signKeyPath |
string |
true |
Path to a public QSEAL certificate |
signPubKeySerial |
string |
true |
Public QSEAL certificate serial number |
paymentAuthState |
string |
false |
Redirect uri for payment (PISP) authorization flow |
paymentAuthRedirectUri |
string |
false |
State for payment (PISP) authorization flow |
OmaSpConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Samlink Developer Portal) |
clientSecret |
string |
false |
API client secret (sandbox client secret obtained from Samlink Developer Portal, not used in production) |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. Required for production, not used in sandbox. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath (not used in sandbox, required for production) |
signCertString |
string |
false |
QSEAL certificate string. Required for production, not used in sandbox. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Samlink Developer Portal, "Ocp-Apim-Subscription-Key" in sandbox, "Api Key" in production) |
PKOConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PKOConnectorSettings;
ApiClient apiClient = new ApiClient(
new PKOConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-key.pem")
.signPubKeySerial("qseal-cert-serial")
.signFingerprint("qseal-cert-fingerprint")
.signCertUrl("qseal-cert-public-url")
.tppId("your-tpp-id")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
none |
signFingerprint |
string |
true |
none |
signCertUrl |
string |
true |
none |
tppId |
string |
true |
none |
paymentAuthRedirectUri |
string |
false |
none |
paymentAuthState |
string |
false |
none |
POPPankkiConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Samlink Developer Portal) |
clientSecret |
string |
false |
API client secret (sandbox client secret obtained from Samlink Developer Portal, not used in production) |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. Required for production, not used in sandbox. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath (not used in sandbox, required for production) |
signCertString |
string |
false |
QSEAL certificate string. Required for production, not used in sandbox. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Samlink Developer Portal, "Ocp-Apim-Subscription-Key" in sandbox, "Api Key" in production) |
PekaoConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.PekaoConnectorSettings;
ApiClient apiClient = new ApiClient(
new PekaoConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-key.pem")
.signPubKeySerial("qseal-cert-serial")
.signFingerprint("qseal-cert-fingerprint")
.signCertUrl("qseal-cert-public-url")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
none |
clientSecret |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
none |
signFingerprint |
string |
true |
none |
signCertUrl |
string |
true |
none |
paymentAuthRedirectUri |
string |
false |
none |
paymentAuthState |
string |
false |
none |
serverSignCertPath |
string |
false |
Path/URI for a server public pem certificate which is used for verification of a signature in a response received from a server. |
serverSignCertThumbprint |
string |
false |
Thimbprint of a server signature pem certificate provided in the |
PenSamConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
ResursConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.ResursConnectorSettings;
ApiClient apiClient = new ApiClient(
new ResursConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.xApiKey("put-your-x-api-key-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
xApiKey |
string |
true |
API client key (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. Test certificate (for accessing sandbox environment) can be downloaded from Open Banking Market. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
paymentAuthRedirectUri |
string |
false |
none |
paymentAuthState |
string |
false |
none |
RevolutConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Revolut Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
SBABConnectorSettings
Settings for initializing ApiClient for SBAB.
Credentials can obtained at SBAB Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required for production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required for production. |
sandboxCertString |
string |
false |
QWAC certificate string. Required for sandbox. |
paymentAuthRedirectUri |
string |
false |
Redirect uri for payment (PISP) authorization flow |
SDCConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SDCConnectorSettings;
ApiClient apiClient = new ApiClient(
new SDCConnectorSettings()
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.ocpApimSubscriptionKey("Ocp-Apim-Subscription-Key)
.sandbox(true));
Settings for initializing ApiClient for SDC.
Credentials can obtained at SDC Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
clientId |
string |
true |
Client reference for the bank that you are calling to obtain data. Issued by SDC to TPP in onboarding process |
clientSecret |
string |
true |
Client secret obtained from SDC in onboarding process |
certPath |
string |
false |
Path to QWAC certificate in PEM format. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from SDC Developer Portal) |
SEBConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBConnectorSettings;
ApiClient apiClient = new ApiClient(
new SPankkiConnectorSettings()
.redirectUri("https://your-redirect-uri")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.clientId("put-your-client-id-here")
.country("EE")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.sandbox(true));
Settings for initializing ApiClient for SEB Estonia, SEB Latvia and SEB Lithuania.
Credentials can obtained at SEB Developer Portal for Baltic countries.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
clientId |
string |
true |
API client ID (obtained from SEB Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
SEBDenmarkConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SEBSwedenConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SEBSwedenConnectorSettings;
ApiClient apiClient = new ApiClient(
new SEBSwedenConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.sandbox(true));
Settings for initializing ApiClient for SEB Sweden.
Credentials can obtained at SEB Developer Portal.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from SEB Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from SEB Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
SPankkiConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SPankkiConnectorSettings;
ApiClient apiClient = new ApiClient(
new SPankkiConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.xApiKey("put-your-x-api-key-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.signKeyPath("path/to/qseal-crt.pem")
.signPubKeySerial("qseal-cert-public-serial-here")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for S-Pankki (Finland).
Credentials can obtained at Open Banking Market
operated by Crosskey Banking Solutions.
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
xApiKey |
string |
true |
API client key (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format. |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
SaastopankkiConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (sandbox client id obtained from Samlink Developer Portal, for production equals organizationIdentifier from eIDAS QSEAL certificate subject) |
clientSecret |
string |
false |
API client secret (sandbox client secret obtained from Samlink Developer Portal, not used in production) |
signKeyPath |
string |
false |
Path to sandbox key used for QSeal certificate. Required for production, not used in sandbox. |
signPubKeySerial |
string |
false |
Public serial key of the QSeal certificate located in signKeyPath (not used in sandbox, required for production) |
signCertString |
string |
false |
QSEAL certificate string. Required for production, not used in sandbox. |
ocpApimSubscriptionKey |
string |
true |
API subscription key (obtained from Samlink Developer Portal, "Ocp-Apim-Subscription-Key" in sandbox, "Api Key" in production) |
SallingBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SantanderConsumerBankNorwayConnectorSettings
Settings for initializing ApiClient for Santander Consumer Bank (NO)
Credentials can obtained at Santander Consumer Bank (NO) Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Santander Consumer Bank (NO) Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Santander Consumer Bank (NO) Developer Portal) |
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
SiauliuBankasConnectorSettings
Settings for initializiong ApiClient for [Šiaulių Bankas] (https://sb.lt/)
Credentials can be obtained at Šiaulių Bankas Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
SkjernBankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SkjernBankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SkjernBankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for SkjernBank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
SparNordBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SparekassenSjaellandFynConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SparekassenSjaellandFynConnectorSettings;
ApiClient apiClient = new ApiClient(
new SparekassenSjaellandFynConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for Sparekassen Sjælland-Fyn
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
SwedbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SwedbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SwedbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.country("SE")
.redirectUri("scheme://uri/to/redirect/after/payment")
.sandbox(true));
Settings for initializing ApiClient for Swedbank (all countries except Norway).
Credentials can obtained at Swedbank Developer Portal
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
country |
string |
true |
Bank country (only clients from the specified will be able to authenticate). Possilbe values: |
certPath |
string |
false |
Path to QWAC certificate in PEM format. Required for production. |
keyPath |
string |
false |
Path to QWAC certificate private key in PEM format. Required for production. |
clientId |
string |
true |
API client ID (obtained at the developer portal) |
clientSecret |
string |
true |
API client secret (obtained at the developer portal) |
brand |
string |
false |
Name of Swedbank cooperating Savings Bank (e.g. Bergslagens Sparbank). If not set defaults to Swedbank. |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
SwedbankDenmarkConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
SydbankConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.SydbankConnectorSettings;
ApiClient apiClient = new ApiClient(
new SydbankConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.certPath("path/to/qwac-crt.pem")
.keyPath("path/to/qwac-key.pem")
.xApiKey("x-api-key")
.paymentAuthRedirectUri("scheme://uri/to/redirect/after/payment")
.paymentAuthState("payment-auth-state-value-here")
.sandbox(true));
Settings for initializing ApiClient for Sydbank
Credentials can obtained at BankData Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained at Open Banking Market) |
certPath |
string |
true |
Path to QWAC certificate in PEM format. |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format. |
xApiKey |
string |
true |
API client key (obtained from BankData Developer Portal) |
paymentAuthRedirectUri |
string |
false |
URI where clients are redirected to after payment authorization. |
paymentAuthState |
string |
false |
This value returned to paymentAuthRedirectUri after payment authorization. |
TotalbankenConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
VestjyskBankConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
certPath |
string |
true |
Path to QWAC certificate in PEM format |
keyPath |
string |
true |
Path to QWAC certificate private key in PEM format |
signKeyPath |
string |
true |
Path to QSeal certificate in PEM format |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath |
signCertString |
string |
true |
QSEAL certificate string |
nokRedirectUri |
string |
true |
URI of the TPP, where the transaction flow shall be redirected instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. |
VolvofinansConnectorSettings
Code samples
package com.eb.sample;
import com.enablebanking.ApiClient;
import com.enablebanking.model.VolvofinansConnectorSettings;
ApiClient apiClient = new ApiClient(
new VolvofinansConnectorSettings()
.redirectUri("https://your-redirect-uri")
.clientId("put-your-client-id-here")
.clientSecret("put-your-client-secret-here")
.xApiKey("x-api-key")
.sandbox(true));
Settings for initializing ApiClient for Sydbank
Credentials can obtained at Volvofinans Developer Site
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
API client ID (obtained from Volvofinans Developer Portal) |
clientSecret |
string |
true |
API client secret (obtained from Volvofinans Developer Portal) |
xApiKey |
string |
true |
API client key (obtained from Volvofinans Developer Portal) |
YaConnectorSettings
Base type
Fields
Name | Type | Required | Description |
---|---|---|---|
clientId |
string |
true |
none |
xApiKey |
string |
true |
none |
certPath |
string |
true |
none |
keyPath |
string |
true |
none |
signKeyPath |
string |
true |
none |
signPubKeySerial |
string |
true |
Public serial key of the QSeal certificate located in signKeyPath. The serial must be |
paymentAuthRedirectUri |
string |
false |
none |
paymentAuthState |
string |
false |
none |
Platform
Java
public abstract class AbstractConnectorSettingsFactory
Base class for connector settings factory, which can be used for creating instances of connector-specific settings classes (derived from ConnectorSettings class)
Code sample
public class SampleConnectorSettingsFactory {
@Override
public String getConnectorName() {
// Returning connector identifier
return "Nordea";
}
@Override
public Boolean getSandbox() {
// Returning value of `sandbox` parameter (common for all connectors)
return true;
}
@Override
public String getAccessToken() {
// Returning "not set" for `accessToken` parameter (common for all connectors)
return null;
}
@Override
public String getConsentId() {
// Returning "not set" for `consentId` parameter (common for all connectors)
return null;
}
@Override
public String getRedirectUri() {
// Returning value of `redirectUri` parameter (common for all connectors)
return "https://redirect-uri";
}
public String getClientId() {
// Returning value of `clientId` parameter (Nordea specific)
return "api-client-id";
}
public String getClientSecret() {
// Returning value of `clientSecret` parameter (Nordea specific)
return "api-client-secret";
}
public String getCertPath() {
// Returning value of `certPath` parameter (Nordea specific)
return "/path/to/qwac/cert";
}
public String getKeyPath() {
// Returning value of `keyPath` parameter (Nordea specific)
return "/path/to/qwac/key";
}
}
abstract protected String getConnectorName()
- Returns: Connector identifier
abstract protected Boolean getSandbox()
- Returns:
true
- sandbox settings,false
- production settings
abstract protected String getConsentId()
- Returns: Consent identifier (
null
if consent is not set)
abstract protected String getSandboxConsentId()
- Returns: Sandbox consent identifier (
null
if consent is not set)
abstract protected String getAccessToken()
- Returns: User access token (
null
if access token is not set)
public ConnectorSettings createConnectorSettings()
Creates connector settings for a connector with identifier returned from getConnectorName
method. The created connector settings instance will contain data returned by the
corresponding methods of the factory. For example, if getConnectorName
returns "Nordea"
instance of NordeaConnectorSettings
class will be created and data like client ID and
client secret will be set (corresponding methods including getClientId
and
getClientSecret
must be implemented in the factory, otherwise runtime exception will be
thrown).
- Returns: Instance of a connector settings class
public abstract class AbstractPlatformExtension
Base class for customazing low-level functionality to the requirements of a target platform
- Package: com.enablebanking
abstract protected PlatformApiResponse makeRequest(PlatformApiRequest request)
Executes API request and returns an object containing response details.
- Parameters:
request
— Request details including parameters for establishing (M)TLS connection - Returns: Object containing response details
abstract protected String signWithKey(String data, String keyPath, String hashAlgorithm, String cryptoAlgorithm)
Generates signature of the provided data
.
Signing algorithm is determined by the key
- Parameters:
data
— String to be signedkeyPath
— URI of the key used for signing {@code data} parameterhashAlgorithm
— Hash algorithm to use (optional). If not provided then SHA256 will be usedcryptoAlgorithm
— Cryptographic algorithm to use. Default RS used if algorithm is not provided
- Returns: String containing signature
abstract protected boolean verifySignature(String signature, String message, String certPath, String hashAlgorithm, String cryptoAlgorithm)
- Parameters:
signature
— Base64 urlsafe encoded signature to verifymessage
— Message to verify againstcertPath
— Path to a public certificate to use for verificationhashAlgorithm
— Hash algorithm to use (optional). If not provided then SHA256 will be usedcryptoAlgorithm
— Cryptographic algorithm to use. Default RS used if algorithm is not provided
- Returns: boolean showing if signature is valid
public class PlatformApiRequest
Internal representation of API request
- Package: com.enablebanking
public PlatformApiRequest(String method, String origin, String path, List<Map.Entry<String, String>> headers, List<Map.Entry<String, String>> query, String body, TlsSettings tls)
Creates instance of the class with all necessary parameters.
- Parameters:
method
— HTTP method: GET, POST, PUT, etcorigin
— HTTP origin (i.e.<scheme> "://" <hostname> [ ":" <port> ]
)path
— Path part of a requested URLheaders
— HTTP headers as a list of key-value pairsquery
— Query part of a requested URL as a list of key-value pairsbody
— HTTP bodytls
— Parameters for establishing (M)TLS connection
public PlatformApiRequest(String method, String origin, String path, List<Map.Entry<String, String>> headers, List<Map.Entry<String, String>> query, String body)
Creates instance of the class with all parameters except (M)TLS settings.
- Parameters:
method
— HTTP method: GET, POST, PUT, etcorigin
— HTTP origin (i.e.<scheme> "://" <hostname> [ ":" <port> ]
)path
— Path part of an URLheaders
— HTTP headers as a list of key-value pairsquery
— Query part of an URL as a list of key-value pairsbody
— HTTP body
public String getMethod()
- Returns: HTTP method of the request
public String getOrigin()
- Returns: HTTP origin of the request
public String getPath()
- Returns: Path part of the requested URL
public List<Map.Entry<String, String>> getHeaders()
- Returns: HTTP headers of the request
public List<Map.Entry<String, String>> getQuery()
- Returns: Query part of the requested URL
public String getBody()
- Returns: Body of the request
public TlsSettings getTls()
- Returns: Parameters for establishing (M)TLS connection for the request
public String findHeader(String header)
- Parameters:
header
— Name of an HTTP header - Returns: First entry of the HTTP header with a given name or null if not found
public class TlsSettings
Internal representation of the parameters used to establish (M)TLS connection
- Package: com.enablebanking
public TlsSettings(String certPath, String keyPath, String caCertPath, String keyPassword)
Creates instance of the class with all necessary parameters.
- Parameters:
certPath
— URI for identifying client certificate for MTLS connectionkeyPath
— URI for identifying private key for MTLS client certificatecaCertPath
— URI for identifying server certificatekeyPassword
— Password used to get access to the private key (optional)
public TlsSettings(String certPath, String keyPath, String caCertPath)
Creates instance of the class with all parameters except key password (set to null).
- Parameters:
certPath
— URI for identifying client certificate for MTLS connectionkeyPath
— URI for identifying private key for MTLS client certificatecaCertPath
— URI for identifying server certificate
public TlsSettings(String certPath, String keyPath)
Creates instance of the class with all parameters except key password and server certificate (set to null).
- Parameters:
certPath
— URI for identifying client certificate for MTLS connectionkeyPath
— URI for identifying private key for MTLS client certificate
public String getCertPath()
- Returns: URI for identifying client certificate for MTLS connection
public String getKeyPath()
- Returns: URI for identifying private key for MTLS client certificate
public String getCaCertPath()
- Returns: URI for identifying server certificate (null if not set)
public String getKeyPassword()
- Returns: Password used to get access to the private key (null if not set)
@Override public boolean equals(Object obj)
Checks if this object equals to another.
- Parameters:
obj
— Object to compare with - Returns: true when {@code obj} is considered equal to {@code this}, otherwise false
public class PlatformApiResponse
Internal representation of API response
- Package: com.enablebanking
public PlatformApiResponse(int statusCode, List<Map.Entry<String, String>> headers, String body)
Creates instance of the class with all necessary parameters.
- Parameters:
statusCode
— HTTP response codeheaders
— HTTP response headers as list of key-value pairsbody
— Body of HTTP response
public int getStatusCode()
- Returns: HTTP code of the response
public List<Map.Entry<String, String>> getHeaders()
- Returns: HTTP headers of the response
public String getBody()
- Returns: Body of the response
public String findHeader(String header)
- Parameters:
header
— Name of an HTTP header - Returns: First entry of the HTTP header with a given name or null if not found
Changelog
0.5.2
Added
- PSU headers not supported by a connector now will be skipped instead of raising exception.
- [Java] fixed datetime parsing issue in DanskeBank, Alior and Pekao connectors.
- [Javascript] Possibility to initialize ApiClient using settings.
- [Javascript] Platform exposed through enablebanking module object.
- New connectors: AndelskassenFaelleskassen, BILDanmark, CoopBankDenmark, DanskeAndelskassersBank, DenJyskeSparekasse, FasterAndelskasse, FrorupAndelskasse, FroslevMollerupSparekasse, FynskeBank, HandelsbankenDenmark, HvidbjergBank, LaegernesBank, Lansforsakringar, LollandsBank, MajBank, MerkurAndelskasse, MonsBank, NykreditBank, PenSam, SallingBank, SEBDenmark, SparNordBank, SwedbankDenmark, Totalbanken, VestjyskBankArbejdernesLandsbank, Volvofinans.
Fixed
- Several connectors has been fixed (fixes are mostly related to date/time handling).
0.5.1
Added
- [Javascript] Possibility to instantiate model classes with data and support of the native date type.
- [Javascript] Better representation of the errors coming from the connectors.
- Optional parameter
cryptoAlgorithm
added to platform methodsignWithKey
in all platforms in order to enforce usage of a certain algorithm. - Support of
PS256
signature algorithm added on all platforms. - [Java] Added new dependency Bouncy Castle (
bcprov-jdk15on
) to supportPS256
signatures. - New connectors: AlmBrand, Citadele, DjurslandsBank, JyskeBank, Kreditbanken, Landbobank, Luminor, NordfynsBank, Revolut, SDC (NO), SiauliuBankas, SkjernBank, SparekassenSjaellandFyn, Sydbank.
Changed
- Resurs connector now requires
country
setting and in addition to Finland now supports Denmark, Norway and Sweden.
Fixed
- [Java] JDK 9+ compatibility bug is fixed
0.5.0
Added
getAuth
now returns an additional fieldAuth.env
, which contains additional authentication info to be passed tomakeToken
.makeToken
now has a new parameterauthEnv
(value has to be taken fromAuth.env
returned fromgetAuth
).- Field
refreshToken
in connector meta data, displaying refresh token support. - Added new fields to display party account's currency:
PaymentRequestResource.debtorCurrency
andBeneficiary.creditorCurrency
- New connectors: CoopPank, MeridianBank, PrivatBank, SignetBank
Changed
- Breaking change: moved
redirectUri
to base connector settings and removed it from getAuth, makeToken and modifyConsents parameters.redirectUri
is now required parameter for every connector. - Nordea connector settings field
keyPath
tosignKeyPath
in order to maintain consistency for QWAC/QSealC keys naming.
Removed
- Parameters
scope
andresponseType
removed fromgetAuth
. - Nordea connector setting
certPath
removed as QWAC not supported by Nordea.
0.4.4
Added
- Field
requiredPsuHeaders
containing array of mandatory PSU headers added toConnector
data structure. - Fields
modifyConsentsInfo
andauthInfo
containing additional information about usagemodifyConsents
andgetAuth
methods added toConnector
data structure.
0.4.3
Added
- Possibility for revoking PSU consent for several banks.
- Field
consentRevocation
in connector meta data, displaying consent revocation support. - Auth API now provides
setClientInfo
method for setting extra PSU headers such as PSU-IP-Address, PSU-IP-Port, PSU-Geo-Location etc. - [Python] Possibility to initialize
ApiClient
using a connector module imported from a separate package.
Changed
- psuIpAddress parameter removed from ConnectorSettings classes where it was present.
Method
setClientInfo
shall be used instead. - [Python] Platform classes require core module reference to be passed as the first argument of the constructor.
Fixed
- [Java] Top-level null deserialization is fixed.
- Payment request is fixed for Handelsbanken connector.
0.4.2
Added
- Auth API provides
parseRedirectUrl
method for parsing of the parameters returned in the callback URL, which a PSU is redirected to after successful authentication on the ASPSP's website. - [Python] Possibility to use connectors imported from another packages.
- Fields
creditorAccount
anddebtorAccount
added toTransaction
data structure to be able to return information about creditor and debtor accounts in the list of transactions when the information is returned by an ASPSP. - New connectors: DanskeBank, OmaSp and Saastopankki.
- Response signature verification for Pekao bank. If verification is failed then the
InvalidResponseSignatureException
is raised.
Changed
- Validation of
sandbox
setting is unified based on meta information (environment
field). - [Python]
enablebanking.CoreException
is thrown when unable to initialise a connector.
Fixed
- LHV connector fixes and improvements.
0.4.1
Added
- Builders of ConnectorSettings' subclasses now supports any order of parameter chaining.
- Fields
aspspIds
(list of supported ASPSPs identified by ECB's MFI codes) andenvironments
(sandbox/production) are added toConnector
data structure. - [Python] BrokerPlatform used for generating request signatures and making mTLS connections towards APSPSs through eIDAS broker service.
0.4.0
Added
- Optional parameter
hashAlgorithm
added to platform methodsignWithKey
in all platforms. Connector
data structure now has additionalpayments
field containing list of supported payment types (newPaymentTypes
data structure is added).
Changed
- Platform method
signPKCS1v15usingSHA256
renamed tosignWithKey
in all platforms. - Field
PaymentRequestResource.paymentTypeInformation
is used to identify payment type that is going to be executed.
0.3.1
Added
- Base connector for banks implementing PolishAPI on KIR platform.
Changed
- [Python] Capitalization of request headers removed to be able to support API backend with case-sensitive handling of the headers.
- Added Handelsbanken (AISP) connector for Finland and Sweden
- Updated OP connector. Changed connector settings
0.3.0
Changed
-
[Java] UnstructuredRemittanceInformation now uses ArrayList constructor.
-
Parameter
paymentRequestData
of PISP API methodmakePaymentRequestConfirmation
removed and the data is passed insideconfirmation
parameter (PaymentRequestConfirmation
data structure now has an option attributepaymentRequest
).
0.2.1
Added
- Added
DataRetrievalException
andInsufficientScopeException
derived fromHttpException
to represent particular cases.
0.2.0
Added
- [Java] Improved connector settings interface. Added
AbstractConnectorSettingsFactory
class with a factory methodcreateConnectorSettings
to simplify creation of connector settings.ApiClient
now has constructors takingConnectorSettings
instance instead of list of settings objects. - [Java]
AbstractPlatformExtension
class consisting ofsignPKCS1v15usingSHA256
andmakeRequest
methods is added in order to simplify customisation of Java platform. Also addedApiClient
constructor takingAbstractPlatformExtension
as a parameter. Transaction
data structure extended with fieldsbankTransactionCode
,creditor
anddebtor
andBankTransactionCode
data structure added.- Value
refresh_token
is supported forgrant_type
parameter ofmakeToken
method.
Changed
- [Java]
ApiClient
constructors accepting settings as a list of objects andJavaPlatform
class are changed toprotected
. - Changed order of connector settings. Parameters
consentId
,accessToken
andrefreshToken
are now always required (should be set tonull
if not present). - [Java] Standard Java SE 8 date-time classes are used instead of ThreeTen-Backport classes, thus Java SE 6 and 7 support is dropped.
- Field
remittanceInformation
ofTransaction
changed to not required (mainly to support credit card transactions, for which remittance info is usually not provided). Access
data structure changed:balances
andtransactions
fields contain details for requested access andaccounts
field contains list of accessed account IDs.
Fixed
- Field mapping for Open Banking UK standard is improved.
0.1.x
This version was created before the changelog has been introduced.